Python Super Function: Inheritence Super Guide
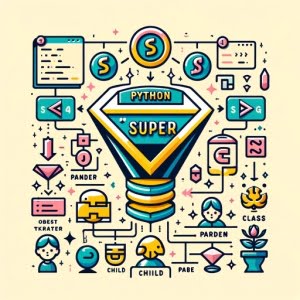
Are you finding it challenging to understand and use Python’s super function? You’re not alone. Many developers find themselves puzzled when it comes to utilizing this powerful function in Python. Think of Python’s super function as a master key – it can unlock powerful functionality in your object-oriented Python code.
This guide will walk you through the process of understanding and effectively using the super function in Python, from the basics to more advanced techniques. We’ll cover everything from the basic use in single inheritance, advanced use in multiple inheritance, as well as alternative approaches.
Let’s dive in and start mastering Python’s super function!
TL;DR: How Do I Use the Super Function in Python?
The super function in Python is used to call a method from a parent class in a child class. It’s a powerful tool in object-oriented programming that allows you to avoid hardcoding class names in your methods, making your code more maintainable and flexible.
Here’s a simple example:
class Parent:
def greet(self):
print('Hello from Parent')
class Child(Parent):
def greet(self):
super().greet()
print('Hello from Child')
child = Child()
child.greet()
# Output:
# 'Hello from Parent'
# 'Hello from Child'
In this example, we have a Parent class with a method greet()
. We then create a Child class that inherits from the Parent class and overrides the greet()
method. Inside the Child’s greet()
method, we use super().greet()
to call the Parent’s greet()
method. When we create an instance of the Child class and call its greet()
method, it first prints ‘Hello from Parent’ (from the Parent’s greet()
method) and then prints ‘Hello from Child’ (from the Child’s greet()
method).
This is a basic way to use the super function in Python, but there’s much more to it. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Python Super Function: The Basics
- Python Super Function in Multiple Inheritance
- Exploring Alternative Approaches to Python Super Function
- Troubleshooting Python Super Function: Common Issues and Solutions
- Digging Deeper: Python’s Class Inheritance and MRO
- Going Beyond Python Super Function
- Wrapping Up: Mastering Python Super Function
Understanding Python Super Function: The Basics
The super()
function in Python is a built-in function designed for use in object-oriented programming. It’s a key part of Python’s class inheritance mechanism and is used to call a method from a parent class in a child class.
How Does the Super Function Work in Python?
To understand how super()
works, let’s start with a basic example of Python’s class inheritance:
class Parent:
def greet(self):
print('Hello from Parent')
class Child(Parent):
def greet(self):
super().greet()
print('Hello from Child')
child = Child()
child.greet()
# Output:
# 'Hello from Parent'
# 'Hello from Child'
In this example, we have two classes: Parent
and Child
. The Child
class is a subclass of the Parent
class, which means it inherits all of the Parent
class’s attributes and methods. In the Child
class, we override the greet()
method, but we still want to use the Parent
class’s greet()
method. This is where super()
comes in.
When we call super().greet()
in the Child
class, Python looks for a greet()
method in the Parent
class and executes it. This is why ‘Hello from Parent’ is printed first, followed by ‘Hello from Child’.
Advantages of Using Super Function
Using super()
has several advantages. It makes your code more maintainable by avoiding hardcoding the parent class name in your methods. This is especially useful if you ever decide to change the name of the parent class or the inheritance hierarchy of your classes.
Potential Pitfalls
While super()
is powerful, it’s not without its pitfalls. It’s important to remember that super()
doesn’t just call the parent class’s method—it follows the method resolution order (MRO), which is a specific order Python follows when looking for methods. If you’re not careful, this can lead to unexpected results, especially when dealing with multiple inheritance. But don’t worry, we’ll cover this in more detail in the ‘Advanced Use’ section.
Python Super Function in Multiple Inheritance
As you delve deeper into Python’s object-oriented programming, you’ll likely encounter scenarios involving multiple inheritance. This is where a class inherits from multiple parent classes. The super()
function plays a crucial role in these scenarios.
Using Super in Multiple Inheritance
Let’s take a look at an example:
class Parent1:
def greet(self):
print('Hello from Parent1')
class Parent2:
def greet(self):
print('Hello from Parent2')
class Child(Parent1, Parent2):
def greet(self):
super().greet()
print('Hello from Child')
child = Child()
child.greet()
# Output:
# 'Hello from Parent1'
# 'Hello from Child'
In this example, our Child
class inherits from two parent classes, Parent1
and Parent2
. Both parent classes have a greet()
method. However, when we call super().greet()
in the Child
class, only the greet()
method from Parent1
is executed. Why is that?
This is due to Python’s Method Resolution Order (MRO). When dealing with multiple inheritance, Python follows a specific order to determine which method to call. In our case, Parent1
comes before Parent2
in the MRO, so Parent1
‘s greet()
method is called.
Understanding Method Resolution Order (MRO)
In Python, you can check the MRO of a class using the mro()
method. Let’s check the MRO for our Child
class:
print(Child.mro())
# Output:
# [<class '__main__.Child'>, <class '__main__.Parent1'>, <class '__main__.Parent2'>, <class 'object'>]
As you can see, Parent1
comes before Parent2
in the MRO, which is why Parent1
‘s greet()
method is called when we use super().greet()
in the Child
class.
Best Practices
When using super()
in multiple inheritance, it’s important to be aware of the MRO and how it affects which methods are called. If you’re finding the MRO confusing or it’s not giving you the results you want, you may need to reconsider your class hierarchy or the design of your classes. Remember, super()
is a powerful tool, but it requires careful use to avoid unexpected results.
Exploring Alternative Approaches to Python Super Function
While super()
is a powerful tool in Python’s object-oriented programming, it’s not the only way to call parent class methods. There are alternative approaches you can use, depending on your specific needs and the complexity of your class hierarchy.
Direct Parent Class Method Calling
One such alternative is to directly call the parent class method. This can be done by explicitly specifying the parent class name when calling the method. Let’s take a look at an example:
class Parent:
def greet(self):
print('Hello from Parent')
class Child(Parent):
def greet(self):
Parent.greet(self)
print('Hello from Child')
child = Child()
child.greet()
# Output:
# 'Hello from Parent'
# 'Hello from Child'
In this example, instead of using super().greet()
, we use Parent.greet(self)
. This directly calls the greet()
method from the Parent
class.
Advantages and Disadvantages
Direct parent class method calling can be useful in certain scenarios. It gives you more control over which parent class method is called, which can be handy in complex inheritance hierarchies. However, it comes with its own set of drawbacks. It can make your code less maintainable, as you have to manually update the parent class name in all method calls if it ever changes. It also breaks the encapsulation principle of object-oriented programming, as the child class needs to know the implementation details of the parent class.
Recommendations
While these alternative approaches can be useful, it’s generally recommended to use the super()
function whenever possible. It makes your code more maintainable and flexible, and it adheres to the principles of object-oriented programming. However, understanding these alternatives can give you more tools in your programming toolbox and help you make more informed design decisions.
Troubleshooting Python Super Function: Common Issues and Solutions
While the super()
function in Python is a powerful tool, it’s not without its challenges. Let’s discuss some common issues you may encounter when using super()
, and how to resolve them.
Incorrect Inheritance Order
One common issue is incorrect inheritance order. This can occur in multiple inheritance scenarios where the order of parent classes matters.
Let’s consider an example:
class Parent1:
def greet(self):
print('Hello from Parent1')
class Parent2:
def greet(self):
print('Hello from Parent2')
class Child(Parent2, Parent1):
def greet(self):
super().greet()
print('Hello from Child')
child = Child()
child.greet()
# Output:
# 'Hello from Parent2'
# 'Hello from Child'
In this case, we’ve switched the order of Parent1
and Parent2
in the Child
class’s inheritance list. Now, super().greet()
calls Parent2
‘s greet()
method instead of Parent1
‘s. This is because Python follows the order of parent classes from left to right when looking for methods.
Problems with Method Resolution Order (MRO)
Another common issue is problems with the Method Resolution Order (MRO). This can occur when the inheritance hierarchy is complex and the MRO isn’t what you expect.
To avoid these issues, it’s important to understand the MRO and check it using the mro()
method if necessary. You may also need to rethink your class hierarchy or method names to ensure they work as expected with the MRO.
Tips for Using Super Function
Here are some tips for using the super()
function effectively:
- Understand the MRO: The MRO determines which method
super()
calls. Make sure you understand how it works and check it using themro()
method if necessary. Be careful with multiple inheritance: Multiple inheritance can make the MRO more complex. Be careful with the order of parent classes and consider whether multiple inheritance is the best solution for your needs.
Use clear, distinct method names: This can help avoid unexpected method calls due to the MRO.
Remember, while super()
is a powerful tool, it requires careful use and a good understanding of Python’s class inheritance system.
Digging Deeper: Python’s Class Inheritance and MRO
To fully grasp the power and functionality of the super()
function, it’s important to understand two fundamental concepts in Python’s object-oriented programming: class inheritance and the method resolution order (MRO).
Python’s Class Inheritance
In Python, class inheritance is a mechanism that allows one class (the child or subclass) to inherit the attributes and methods of another class (the parent or superclass). This promotes code reusability and makes it easier to create and maintain complex applications.
Let’s consider a simple example:
class Parent:
def greet(self):
print('Hello from Parent')
class Child(Parent):
pass
child = Child()
child.greet()
# Output:
# 'Hello from Parent'
In this example, the Child
class inherits from the Parent
class. This means it gets access to the Parent
class’s greet()
method, even though we didn’t define it in the Child
class. When we create an instance of the Child
class and call the greet()
method, it prints ‘Hello from Parent’.
Method Resolution Order (MRO)
The method resolution order (MRO) is the order in which Python looks for methods in a class hierarchy. It’s a key part of how the super()
function works, especially in scenarios involving multiple inheritance.
The MRO follows a specific algorithm that takes into account both the order of parent classes in the class definition and the inheritance hierarchy. You can check the MRO of a class using the mro()
method, as we’ve seen in previous examples.
Understanding both class inheritance and the MRO is crucial to effectively using the super()
function in Python. It allows you to call parent class methods in a flexible and maintainable way, while avoiding common pitfalls and unexpected results.
Going Beyond Python Super Function
The super()
function is a vital part of Python’s object-oriented programming. However, it’s just one piece of the puzzle. To become proficient in Python, especially in object-oriented programming, it’s essential to explore related concepts.
Exploring Polymorphism and Encapsulation
Two such related concepts are polymorphism and encapsulation. Polymorphism is a principle in object-oriented programming that allows objects of different classes to be treated as objects of the same class. It’s closely related to the super()
function and class inheritance, as it often involves overriding methods in a subclass.
Encapsulation, on the other hand, is about hiding the internal details of how an object works. It’s about creating a clear interface for interacting with an object, while keeping the implementation details private. This can make your code more maintainable and less prone to errors.
Further Resources for Mastering Python OOP
To deepen your understanding of Python’s object-oriented programming, here are some resources that you might find helpful:
- IOFlood’s Guide to Python OOP – Learn how to use encapsulation in Python to restrict access to certain parts of a class and prevent data modification.
Understanding the Object in Python – Explore Python object-oriented programming principles and their applications.
Exploring Inheritance in Python – Master Python inheritance concepts for creating relationships between classes.
Real Python’s guide on Object-Oriented Programming (OOP) in Python 3 provides a thorough introduction to OOP in Python.
Python’s official documentation on classes – The official documentation on Python’s features.
Python OOP Tutorial – This video tutorial covers everything from basic to advanced topics.
Remember, mastering Python’s object-oriented programming, including the super()
function, is a journey. Don’t rush it. Take your time to understand the concepts, practice with real code, and don’t hesitate to seek help when you need it.
Wrapping Up: Mastering Python Super Function
In this comprehensive guide, we’ve explored the ins and outs of the super()
function in Python, a powerful tool in object-oriented programming.
We began with the basics, learning how to use the super()
function in single inheritance scenarios. We then delved into more advanced usage, such as handling multiple inheritance and understanding the method resolution order (MRO). Along the way, we tackled common issues you might encounter when using super()
, such as incorrect inheritance order and problems with the MRO, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to calling parent class methods, such as direct parent class method calling. Here’s a quick comparison of these methods:
Method | Flexibility | Maintainability |
---|---|---|
super() function | High | High |
Direct parent class method calling | Moderate | Low |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your object-oriented programming skills, we hope this guide has given you a deeper understanding of the super()
function in Python and its capabilities.
With its balance of flexibility and maintainability, the super()
function is a powerful tool for object-oriented programming in Python. Happy coding!