Python Objects: Usage, Syntax, and Examples
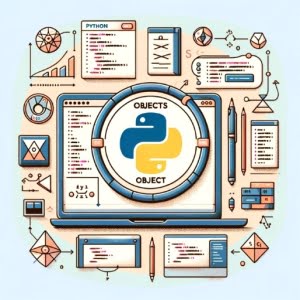
Ever found yourself puzzled over creating and manipulating objects in Python? You’re not alone. Many developers, especially those new to Python, find this aspect of the language a bit challenging.
In this guide, we’ll walk you through the process of creating and manipulating objects in Python, from the basics to more advanced techniques. We’ll cover everything from defining classes, instantiating objects, accessing methods and attributes, to more advanced object manipulation techniques.
So, whether you’re a beginner just starting out or an experienced developer looking to brush up your skills, there’s something in this guide for you. Let’s get started!
TL;DR: How Do I Create and Manipulate Objects in Python?
In Python, you create objects by defining a class and then instantiating an object of that class. You can manipulate the object by calling its methods or changing its attributes.
Here’s a simple example:
class MyClass:
def __init__(self, name):
self.name = name
my_object = MyClass('John')
print(my_object.name)
# Output:
# 'John'
In this example, we’ve defined a class MyClass
with a method __init__
that sets the attribute name
. We then create an object my_object
of the class MyClass
and print the name
attribute of the object, which outputs ‘John’.
This is a basic way to create and manipulate objects in Python, but there’s much more to learn about Python’s object-oriented programming. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Python Objects 101: Defining Classes and Instantiating Objects
- Advanced Python Object Manipulation Techniques
- Python Object Manipulation: Alternative Approaches
- Troubleshooting Python Object Creation and Manipulation
- Understanding Python Objects and Object-Oriented Programming
- Python Objects in Real-World Applications
- Wrapping Up: Mastering Python Objects
Python Objects 101: Defining Classes and Instantiating Objects
In Python, everything is an object. This includes integers, strings, lists, and even functions. Each object is an instance of a class, which is like a blueprint for creating objects. Let’s dive into how to define a class in Python, instantiate an object of that class, and access its methods and attributes.
Defining a Class
In Python, a class is defined using the class
keyword. Here’s how you can define a simple class:
class MyClass:
pass
In this example, MyClass
is a very basic class with no attributes or methods.
Creating an Object
Once a class is defined, you can create an object of that class by calling the class as if it were a function. Here’s how you can create an object of MyClass
:
my_object = MyClass()
In this example, my_object
is an object of the class MyClass
.
Accessing Attributes and Methods
Attributes and methods are accessed using the dot (.
) operator. Let’s define a class with an attribute and a method, create an object of that class, and access its attribute and method:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
return f'Hello, {self.name}!'
my_object = MyClass('Alice')
print(my_object.name)
print(my_object.greet())
# Output:
# 'Alice'
# 'Hello, Alice!'
In this example, MyClass
has an attribute name
and a method greet
. The __init__
method is a special method that gets called when an object is created. It’s used to initialize the attributes of the object. In the greet
method, we’re using the name
attribute to return a greeting.
Understanding how to define a class, create an object, and access its attributes and methods is the foundation of working with objects in Python. It allows you to encapsulate related data and functions into one entity, making your code more organized and reusable. However, it’s important to be aware of potential pitfalls such as trying to access an attribute or method that doesn’t exist, which can lead to errors.
Advanced Python Object Manipulation Techniques
Once you’ve grasped the basics of creating and manipulating objects in Python, it’s time to level up your skills with some more advanced techniques. This includes changing an object’s attributes, calling its methods, and even deleting an object.
Changing an Object’s Attributes
An object’s attributes can be changed just like you would change the value of a variable. Here’s how you can change the name
attribute of the my_object
object:
my_object.name = 'Bob'
print(my_object.name)
# Output:
# 'Bob'
In this example, we’ve changed the name
attribute of my_object
from ‘Alice’ to ‘Bob’.
Calling an Object’s Methods
An object’s methods can be called using the dot (.
) operator, just like accessing its attributes. Here’s how you can call the greet
method of the my_object
object:
print(my_object.greet())
# Output:
# 'Hello, Bob!'
In this example, calling the greet
method of my_object
now returns ‘Hello, Bob!’ because we changed the name
attribute to ‘Bob’.
Deleting an Object
You can delete an object using the del
statement. This removes the object and frees up the memory it was using. Here’s how you can delete the my_object
object:
del my_object
Note that trying to access my_object
after deleting it will raise a NameError
because my_object
no longer exists.
Mastering these advanced object manipulation techniques can give you more control over your Python code and help you write more efficient and effective programs. However, it’s important to be mindful of potential issues such as trying to access an object after deleting it, which can lead to errors.
Python Object Manipulation: Alternative Approaches
Python provides a variety of built-in functions and special methods for creating and manipulating objects. These alternatives can offer more flexibility and control in certain situations. Let’s explore some of these methods.
Built-in Functions: getattr() and setattr()
Python’s built-in getattr()
function allows you to access an object’s attribute by its name as a string. Similarly, setattr()
lets you set the value of an attribute. Here’s how you can use these functions:
print(getattr(my_object, 'name'))
setattr(my_object, 'name', 'Charlie')
print(getattr(my_object, 'name'))
# Output:
# 'Bob'
# 'Charlie'
In this example, getattr(my_object, 'name')
returns the value of the name
attribute of my_object
, and setattr(my_object, 'name', 'Charlie')
changes the name
attribute to ‘Charlie’.
Special Methods: str and del
Python objects also have special methods that provide default behaviors. The __str__
method returns a string representation of the object, and the __del__
method is called when the object is about to be destroyed. Here’s how you can define these methods in a class:
class MyClass:
def __init__(self, name):
self.name = name
def __str__(self):
return f'Object with name {self.name}'
def __del__(self):
print(f'Object with name {self.name} is being deleted')
my_object = MyClass('Charlie')
print(str(my_object))
del my_object
# Output:
# 'Object with name Charlie'
# 'Object with name Charlie is being deleted'
In this example, str(my_object)
calls the __str__
method of my_object
and returns ‘Object with name Charlie’, and del my_object
deletes my_object
and calls the __del__
method, which prints ‘Object with name Charlie is being deleted’.
These alternative approaches to creating and manipulating objects in Python can be very powerful, especially in more complex or specialized situations. However, they should be used judiciously as they can make your code harder to understand if overused.
Troubleshooting Python Object Creation and Manipulation
In the process of creating and manipulating Python objects, you may encounter some common issues. These include AttributeError
, TypeError
, and NameError
. Let’s discuss these errors, their causes, and how to solve them.
AttributeError
AttributeError
occurs when you try to access or modify an attribute that doesn’t exist. Here’s an example:
my_object = MyClass('Charlie')
print(my_object.age)
# Output:
# AttributeError: 'MyClass' object has no attribute 'age'
In this example, trying to print my_object.age
raises an AttributeError
because MyClass
doesn’t have an attribute named age
. To solve this, ensure that the attribute you’re trying to access or modify exists.
TypeError
TypeError
occurs when an operation or function is applied to an object of an inappropriate type. Here’s an example:
my_object = 'Charlie'
print(my_object.name)
# Output:
# TypeError: 'str' object has no attribute 'name'
In this example, trying to print my_object.name
raises a TypeError
because my_object
is a string, not an object of MyClass
. To solve this, ensure that the object you’re working with is of the correct type.
NameError
NameError
occurs when a name is not found in the local or global scope. Here’s an example:
print(my_object)
# Output:
# NameError: name 'my_object' is not defined
In this example, trying to print my_object
raises a NameError
because my_object
has not been defined. To solve this, ensure that the name you’re using has been defined.
Understanding these common issues and their solutions can help you debug your Python code more effectively. Remember, the best way to prevent errors is to write clean, understandable code and to test your code frequently.
Understanding Python Objects and Object-Oriented Programming
Python is an object-oriented programming language, which means it’s built around the concept of objects. An object is an entity that contains data along with associated functionalities. In Python, everything is an object – from basic types like numbers and strings to more complex types like classes and functions. Understanding the concept of objects is fundamental to mastering Python.
Classes, Methods, and Attributes
In Python, objects are instances of classes. A class is like a blueprint for creating objects. It defines a set of attributes and methods that its objects will have. Attributes are variables that hold data, and methods are functions that perform operations.
Here’s an example of a class with an attribute and a method:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
return f'Hello, {self.name}!'
In this example, MyClass
has an attribute name
and a method greet
. The __init__
method is a special method that gets called when an object is created. It’s used to initialize the attributes of the object.
Inheritance, Encapsulation, and Polymorphism
Inheritance, encapsulation, and polymorphism are three fundamental principles of object-oriented programming.
- Inheritance allows a class to inherit attributes and methods from another class. This promotes code reuse and is a way to form relationships between classes.
Encapsulation is the practice of hiding the internal details of objects and exposing only what’s necessary. This improves code security and simplicity.
Polymorphism allows one interface to be used for a general class of actions. This provides flexibility and makes it easier to add new classes.
Understanding these principles can help you write more efficient and effective Python code. Remember, the power of Python’s object-oriented programming lies in its simplicity and flexibility. So, don’t be afraid to experiment and explore!
Python Objects in Real-World Applications
Understanding how to create and manipulate objects in Python isn’t just a theoretical exercise. It’s a practical skill that you’ll use in real-world applications. Let’s explore how this knowledge can be applied in three key areas: game development, web development, and data analysis.
Python Objects in Game Development
In game development, objects can represent elements in the game like characters, items, or environments. Each object can have attributes like health, position, or color, and methods that define what the object can do. Understanding Python objects allows you to structure your game code effectively and intuitively.
Python Objects in Web Development
In web development, Python objects can represent elements like web pages, forms, or database records. For instance, in a web application built with Django (a popular Python web framework), each model (a Python class) can represent a table in the database, and each object of the model represents a record in the table.
Python Objects in Data Analysis
In data analysis, objects can represent datasets or statistical models. Python’s pandas library, for instance, provides the DataFrame object for handling structured data. Each DataFrame object has methods for filtering, grouping, and transforming data, making data analysis tasks more straightforward.
Further Resources for Mastering Python Objects
To continue your journey in mastering Python objects, here are some resources that provide more in-depth information:
- Beginner’s Guide to Python OOP Concepts – Explore the power of Python Object Oriented Programming to create code structures.
Abstract Class Usage in Python – Learn how to create abstract classes in Python.
Python super(): Accessing Parent Class Methods – Understand the usage of super() in Python for parent class methods.
Python’s official documentation on classes is a comprehensive guide on classes (and hence objects) in Python.
Real Python’s guide on object-oriented programming provides a practical introduction to Python OOP.
Python Classes Tutorial – Comprehensive guide to understanding and using classes in Python by W3Schools.
Mastering Python objects can open up a world of possibilities in your programming journey. So don’t stop here – keep learning, keep practicing, and keep exploring!
Wrapping Up: Mastering Python Objects
In this comprehensive guide, we’ve explored the ins and outs of creating and manipulating objects in Python, a fundamental aspect of Python’s object-oriented programming.
We began with the basics, learning how to define a class, instantiate an object, and access its methods and attributes. We then delved into more advanced techniques, such as changing an object’s attributes, calling its methods, and even deleting an object.
Along the way, we tackled common issues that you might encounter when working with Python objects, such as AttributeError
, TypeError
, and NameError
, providing solutions to help you navigate these challenges.
We also explored alternative approaches to creating and manipulating objects, such as using Python’s built-in getattr()
and setattr()
functions and special methods like __str__
and __del__
. Here’s a quick comparison of these methods:
Method | Flexibility | Complexity |
---|---|---|
Direct Attribute Access | Low | Low |
getattr() and setattr() | High | Moderate |
Special Methods | High | High |
Whether you’re a beginner just starting out with Python or an experienced developer looking to deepen your understanding of Python’s object-oriented programming, we hope this guide has given you a solid foundation in creating and manipulating Python objects. Happy coding!