Python OOP: Mastering Object-Oriented Programming
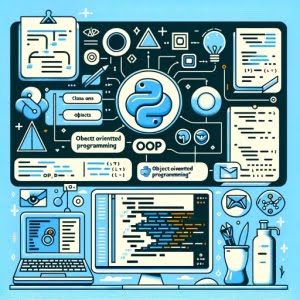
Feeling a bit lost when it comes to object-oriented programming (OOP) in Python? You’re not alone. Many programmers find this concept a bit confusing at first. But don’t worry, just like building blocks, OOP allows you to construct large, complex programs with ease. It’s a powerful tool in your programming arsenal.
In this guide, we’ll walk you through the basics of OOP in Python. We’ll start with creating simple classes and gradually move on to more complex concepts like inheritance and polymorphism. By the end of this guide, you’ll have a solid understanding of Python’s OOP and how to use it effectively in your projects.
TL;DR: What is Object-Oriented Programming in Python?
Object-oriented programming (OOP) in Python is a programming paradigm that uses ‘objects’—instances of classes—which are capable of having properties and behaviors. In other words, it’s a way to structure your Python code using concepts like classes and objects.
Here’s a simple example of a class in Python:
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return 'Woof!'
my_dog = Dog('Fido')
print(my_dog.bark())
# Output:
# 'Woof!'
In this example, we define a Dog
class with a bark
method. We then create an instance of the Dog
class (an object), and call its bark
method. The output is ‘Woof!’, as expected.
This is just a basic introduction to OOP in Python. There’s much more to learn about classes, objects, and other OOP concepts. Continue reading for a more detailed understanding of OOP in Python.
Table of Contents
- Python OOP Basics: Creating Classes and Objects
- Python OOP: Understanding Methods
- Advanced OOP Concepts: Inheritance, Encapsulation, and Polymorphism
- Alternative Programming Paradigms in Python
- Troubleshooting Python OOP: Common Pitfalls and Solutions
- Unpacking Python OOP: Classes, Objects, and More
- Python OOP: Beyond the Basics
- Wrapping Up: Python’s OOP and Beyond
Python OOP Basics: Creating Classes and Objects
In Python, the term ‘class’ is used to define a new type of object. A class serves as a blueprint for creating instances or objects of that class. Here’s a simple example of how to define a class in Python:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
In this example, Car
is the class with two properties: brand
and model
. The __init__
method is a special method that Python calls when a new instance of the class is created. This method sets the initial state of the object.
Now, let’s create an object of the Car
class:
my_car = Car('Toyota', 'Corolla')
print(my_car.brand)
print(my_car.model)
# Output:
# Toyota
# Corolla
Here, my_car
is an object (or instance) of the Car
class. We can access the properties of the Car
class using the dot notation.
Python OOP: Understanding Methods
Methods are functions that are defined within a class. They represent the behaviors that an object of the class can perform. Let’s add a method to our Car
class:
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def honk(self):
return 'Beep Beep!'
my_car = Car('Toyota', 'Corolla')
print(my_car.honk())
# Output:
# Beep Beep!
In this example, honk
is a method that returns ‘Beep Beep!’ when called. We can call this method on any object of the Car
class.
Understanding these basic concepts of defining classes, creating objects, and using methods is crucial to mastering Python’s OOP. They provide a structured way to organize your code, making it more readable and maintainable. However, keep in mind that each class should have a single responsibility. Avoid creating ‘god’ classes that do too much, as it can make your code hard to understand and maintain.
Advanced OOP Concepts: Inheritance, Encapsulation, and Polymorphism
As you get more comfortable with Python’s OOP, you’ll encounter more advanced concepts like inheritance, encapsulation, and polymorphism. Let’s dive into these concepts and how they can benefit your programming.
Inheritance: Building Upon Classes
Inheritance allows us to define a class that inherits all the methods and properties from another class. The original class is called the parent class, and the new class is called the child class.
class Vehicle:
def __init__(self, brand, model):
self.brand = brand
self.model = model
class Car(Vehicle):
def honk(self):
return 'Beep Beep!'
my_car = Car('Toyota', 'Corolla')
print(my_car.honk())
print(my_car.brand)
print(my_car.model)
# Output:
# Beep Beep!
# Toyota
# Corolla
In this example, Car
is a child class of Vehicle
and inherits its properties. We can also add new methods to the Car
class.
Encapsulation: Hiding the Inner Workings
Encapsulation is a mechanism of wrapping the data (variables) and the code acting on the data (methods) into a single unit. In Python, we denote private attributes using a single underscore _
before the attribute name.
class Car:
def __init__(self, brand, model):
self._brand = brand
self._model = model
def get_brand(self):
return self._brand
my_car = Car('Toyota', 'Corolla')
print(my_car.get_brand())
# Output:
# Toyota
Here, _brand
and _model
are private attributes. We use a getter method get_brand
to access _brand
.
Polymorphism: One Interface, Multiple Functions
Polymorphism is an OOP concept that allows us to use a single type entity (method, operator or object) to represent different types in different scenarios.
class Car:
def honk(self):
return 'Beep Beep!'
class Truck:
def honk(self):
return 'Honk Honk!'
def sound_horn(vehicle):
print(vehicle.honk())
my_car = Car()
my_truck = Truck()
sound_horn(my_car)
sound_horn(my_truck)
# Output:
# Beep Beep!
# Honk Honk!
In this example, Car
and Truck
classes have a method with the same name but different functionality. The sound_horn
function can take any object that has a honk
method and call that method.
These advanced concepts of Python’s OOP provide a way to structure your code in a reusable and organized manner. They make it easier to manage and scale your code, especially in large projects.
Alternative Programming Paradigms in Python
While object-oriented programming (OOP) is a powerful tool in Python, it’s not the only programming paradigm available. Python also supports functional and procedural programming, each with its own strengths and use cases.
Functional Programming: Pure Functions and Immutable Data
Functional programming is a paradigm where programs are constructed by applying and composing functions. It emphasizes the use of pure functions (functions that produce the same output for the same input and have no side effects) and immutable data.
def add(x, y):
return x + y
result = add(5, 3)
print(result)
# Output:
# 8
In this example, add
is a pure function. It takes two arguments and returns their sum. The function does not change any state or have any side effects.
Procedural Programming: Step-by-Step Instructions
Procedural programming is a paradigm based upon the concept of procedure calls. Procedures, also known as routines, subroutines, or functions, simply contain a series of computational steps to be carried out.
def greet(name):
print(f'Hello, {name}!')
greet('Alice')
# Output:
# Hello, Alice!
In this example, greet
is a procedure that prints a greeting. The program follows a step-by-step instruction to execute the procedure.
When to Use OOP, Functional, or Procedural Programming
Each of these paradigms has its place. OOP is great when you have a complex system with lots of objects interacting with each other, as it provides a structure to manage these interactions. Functional programming shines when you need to perform data transformations and can benefit from its features like higher-order functions and lazy evaluation. Procedural programming is straightforward and can be a good choice for simple scripts and programs where a step-by-step execution model is appropriate.
Remember, Python is a multi-paradigm language, which means you can use these paradigms in conjunction with each other. The key is to understand each paradigm’s strengths and use the right tool for the job.
Troubleshooting Python OOP: Common Pitfalls and Solutions
As you delve deeper into Python’s OOP, you might encounter some common issues. Here, we’ll discuss these problems and provide solutions and workarounds.
Understanding ‘self’ in Python OOP
One common point of confusion among beginners is the ‘self’ keyword used in Python classes. ‘self’ is a reference to the instance of the class and is used to access variables and methods associated with that instance.
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def display(self):
print(f'Car Brand: {self.brand}, Model: {self.model}')
my_car = Car('Toyota', 'Corolla')
my_car.display()
# Output:
# Car Brand: Toyota, Model: Corolla
In this example, ‘self’ is used to access the ‘brand’ and ‘model’ attributes within the ‘display’ method.
Dealing with Inheritance Issues
Inheritance is a powerful feature of OOP, but it can lead to issues if not used carefully. One common issue is the Diamond Problem, which occurs when a class inherits from two or more classes that have a common superclass.
To solve this issue, Python uses a method resolution order (MRO) algorithm that linearizes the inheritance graph. It ensures that each class is visited once before its parents, and the parent classes are visited in the order they are specified.
class A: pass
class B(A): pass
class C(A): pass
class D(B, C): pass
print(D.mro())
# Output:
# [<class '__main__.D'>, <class '__main__.B'>, <class '__main__.C'>, <class '__main__.A'>, <class 'object'>]
In this example, the MRO of class D is D, B, C, A. This order ensures that class A (the common superclass) is visited only once.
Remember, understanding these issues and how to solve them is crucial to effectively using Python’s OOP. It’s part of the journey to becoming a proficient Python programmer.
Unpacking Python OOP: Classes, Objects, and More
To fully grasp Python’s object-oriented programming (OOP), we need to understand its core principles: classes, objects, methods, inheritance, encapsulation, and polymorphism. These principles form the foundation of OOP in Python.
Classes: The Blueprints
In OOP, a class is a blueprint for creating objects. It’s a user-defined prototype that contains variables and methods that an object of this class will have.
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
In this example, Car
is a class with two variables brand
and model
, and one method __init__
.
Objects: Instances of Classes
Objects are instances of a class. When a class is defined, no memory is allocated but when it is instantiated (i.e., an object is created), memory is allocated.
my_car = Car('Toyota', 'Corolla')
Here, my_car
is an object of the Car
class.
Methods: Behaviors of Objects
Methods are functions defined within a class. They define the behaviors of the objects of the class.
class Car:
def __init__(self, brand, model):
self.brand = brand
self.model = model
def honk(self):
return 'Beep Beep!'
In this example, honk
is a method of the Car
class.
Inheritance: Reusing and Extending Code
Inheritance is a way of creating a new class using details of an existing class without modifying it. The newly formed class is a derived class (or child class). The existing class is a base class (or parent class).
Encapsulation: Hiding Data
Encapsulation is the mechanism of hiding data (variables) and methods within a class from direct access which is also known as data hiding.
Polymorphism: One Interface, Many Implementations
Polymorphism is an OOP concept that allows us to define methods in the child class with the same name as defined in their parent class. As the name suggests, polymorphism gives a way to use a class exactly like its parent so there’s no need to modify a class to use its behavior.
These principles form the backbone of Python’s OOP and are crucial to becoming an effective Python programmer.
Python OOP: Beyond the Basics
Object-oriented programming (OOP) in Python is not just a theoretical concept. It’s a practical tool that you can use to structure your code, especially in larger projects. It’s widely used in real-world applications and can make your code more readable, maintainable, and reusable.
OOP in Larger Projects
In larger projects, OOP can help you manage complexity and keep your code organized. You can define classes that encapsulate data and methods, and create objects that interact with each other. This can make it easier to reason about your code and debug issues.
class Project:
def __init__(self, name):
self.name = name
self.tasks = []
def add_task(self, task):
self.tasks.append(task)
def display_tasks(self):
for task in self.tasks:
print(task)
my_project = Project('My Project')
my_project.add_task('Task 1')
my_project.add_task('Task 2')
my_project.display_tasks()
# Output:
# Task 1
# Task 2
In this example, we define a Project
class that encapsulates data (name and tasks) and methods (add_task and display_tasks). We can create a Project
object and interact with it.
Exploring Related Concepts
Once you’re comfortable with Python’s OOP, you can explore related concepts like design patterns and SOLID principles. Design patterns are reusable solutions to common problems in software design. SOLID principles are a set of guidelines for designing software that is easy to maintain and extend.
Further Resources for Mastering Python OOP
To deepen your understanding of Python’s OOP, you can explore the following resources:
- Python Inheritance: Extending Classes – Explore Python inheritance for code reuse and hierarchy creation.
Python OOP Tutorial – A comprehensive tutorial on Python’s OOP.
Python Design Patterns – An overview of design patterns in Python.
SOLID Principles in Python – An introduction to SOLID principles in Python.
Remember, mastering Python’s OOP is not an overnight process. It requires practice and patience. But once you get the hang of it, it can significantly improve your programming skills.
Wrapping Up: Python’s OOP and Beyond
In this comprehensive guide, we’ve delved into the concept of object-oriented programming (OOP) in Python.
We’ve explored the core principles of OOP, including classes, objects, methods, inheritance, encapsulation, and polymorphism. We’ve also looked at how to define classes, create objects, and use methods in Python, with inline code examples
to illustrate these concepts.
We’ve touched upon some common issues you might encounter when using OOP in Python, such as understanding ‘self’ and dealing with inheritance issues. We’ve provided solutions and workarounds for each issue, helping you navigate the world of Python’s OOP more effectively.
We’ve also discussed alternative programming paradigms in Python, such as functional and procedural programming. Understanding these paradigms can give you more tools to tackle different programming tasks.
Here’s a quick comparison of the discussed methods:
Method | Use Case | Example |
---|---|---|
OOP | Complex systems with interacting objects | Defining a Car class with brand and model attributes |
Functional Programming | Data transformations, pure functions, and immutable data | Using a pure add function to add two numbers |
Procedural Programming | Simple scripts and programs with step-by-step execution | Using a greet procedure to print a greeting |
Remember, mastering Python’s OOP takes time and practice. But once you get the hang of it, it can significantly improve your programming skills and open up new opportunities for you. Happy coding!