What is an ‘Instance’ in Java? | Guide to Creating Objects
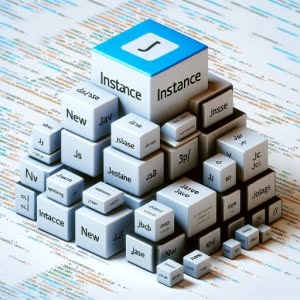
Are you finding it challenging to understand instances in Java? You’re not alone. Many developers, especially beginners, find the concept of instances in Java a bit tricky. But don’t worry, we’ve got you covered.
Think of an instance in Java as a unique copy of a class, each with its own set of variables and methods. It’s like a blueprint that defines the characteristics and behaviors of an object of a specific class.
This guide will walk you through the concept of instances in Java, from their creation to usage. We’ll start with the basics of creating an instance and accessing its variables and methods, then move on to more complex uses, such as creating multiple instances and using instance methods and variables. We’ll also discuss related concepts like static methods and variables, and provide tips for best practices and optimization.
So, let’s dive in and start mastering instances in Java!
TL;DR: What is an Instance in Java?
An
instance
in Java is an object created from a class, along with'new'
keyword followed by the classconstructor
.
Here’s a simple example:
class MyClass {
// class body
}
MyClass obj = new MyClass();
// Output:
// This creates an instance of MyClass named obj.
In this example, we’ve created a class named MyClass
. Then, we create an instance of MyClass
named obj
using the ‘new’ keyword and the class constructor. This is a basic way to create an instance in Java.
But Java’s instance creation and usage capabilities go far beyond this. Continue reading for more detailed examples and advanced usage techniques.
Table of Contents
- Creating and Using Instances in Java: A Beginner’s Guide
- Advanced Instance Creation and Usage in Java
- Exploring Alternative Approaches: Static Methods and Variables
- Navigating Common Errors and Obstacles
- Unpacking Java’s Object-Oriented Programming
- Instances in Java: Beyond the Basics
- Wrapping Up: Mastering Instances in Java
Creating and Using Instances in Java: A Beginner’s Guide
Creating an instance in Java is a straightforward process. First, you define a class, and then you create an instance (or an object) of that class. Let’s start with a simple example:
// Defining a class
class Car {
String color;
String model;
}
// Creating an instance of Car
Car myCar = new Car();
// Output:
// This creates an instance of Car named myCar.
In this example, we defined a class named Car
with two variables: color
and model
. Then, we created an instance of Car
named myCar
using the ‘new’ keyword and the class constructor.
Now that we have an instance of Car
, we can access its variables and modify them:
// Accessing variables of the instance
myCar.color = "Red";
myCar.model = "Sedan";
System.out.println("Car color: " + myCar.color);
System.out.println("Car model: " + myCar.model);
// Output:
// Car color: Red
// Car model: Sedan
In this code block, we assigned the color of myCar
as ‘Red’ and the model as ‘Sedan’. Then, we printed the color and model of myCar
to the console. The output shows the color and model of myCar
as ‘Red’ and ‘Sedan’, respectively.
This is the basic usage of instances in Java: creating an instance, and accessing and modifying its variables. In the next section, we’ll go over some more complex uses of instances.
Advanced Instance Creation and Usage in Java
As you become more comfortable with instances in Java, you can start exploring more complex uses. Let’s discuss creating multiple instances, using instance methods, and working with instance variables.
Creating Multiple Instances
You can create multiple instances of a class, each with its own set of variables and methods. Here’s an example:
// Creating multiple instances of Car
Car myCar1 = new Car();
Car myCar2 = new Car();
myCar1.color = "Red";
myCar1.model = "Sedan";
myCar2.color = "Blue";
myCar2.model = "SUV";
System.out.println("Car1 color: " + myCar1.color + ", Model: " + myCar1.model);
System.out.println("Car2 color: " + myCar2.color + ", Model: " + myCar2.model);
// Output:
// Car1 color: Red, Model: Sedan
// Car2 color: Blue, Model: SUV
In this code block, we created two instances of the Car
class: myCar1
and myCar2
. We assigned different colors and models to each car and then printed their properties. The output shows that each instance has its own set of variables.
Using Instance Methods
Instance methods are methods that belong to an instance of a class. They can access instance variables and perform operations. Here’s an example of using instance methods:
// Adding an instance method to Car
class Car {
String color;
String model;
void display() {
System.out.println("Car color: " + color + ", Model: " + model);
}
}
Car myCar = new Car();
myCar.color = "Red";
myCar.model = "Sedan";
myCar.display();
// Output:
// Car color: Red, Model: Sedan
In this code block, we added a method display()
to the Car
class. This method prints the color and model of the car. We created an instance of Car
, assigned values to its variables, and then used the display()
method to print its properties. The output shows the color and model of the car as defined in the method.
These are some of the more advanced uses of instances in Java. As you continue exploring, you’ll find that instances are a fundamental part of object-oriented programming in Java.
Exploring Alternative Approaches: Static Methods and Variables
In Java, not all methods and variables require an instance for access. Static methods and variables belong to the class itself, not any specific instance. This presents an alternative way of structuring your code.
Static Variables
A static variable is shared by all instances of a class. Any changes made to a static variable are reflected across all instances. Here’s an example:
// Defining a class with a static variable
class Car {
static int carCount;
Car() {
carCount++;
}
}
Car myCar1 = new Car();
Car myCar2 = new Car();
System.out.println("Total Cars: " + Car.carCount);
// Output:
// Total Cars: 2
In this code block, we defined a class Car
with a static variable carCount
. Each time a new instance of Car
is created, carCount
is incremented. We created two instances of Car
, and the output shows carCount
as 2, reflecting the total number of Car
instances.
Static Methods
Like static variables, static methods belong to the class itself and can be called without creating an instance. Here’s an example:
// Defining a class with a static method
class Car {
static void displayCarCount(int carCount) {
System.out.println("Total Cars: " + carCount);
}
}
Car.displayCarCount(2);
// Output:
// Total Cars: 2
In this code block, we defined a class Car
with a static method displayCarCount()
. This method takes an integer argument and prints the total number of cars. We can call this method directly using the class name, without creating an instance of Car
.
Static methods and variables provide an alternative approach to instance-based methods and variables. They can be beneficial when you need to share data across instances or when you want to perform operations that don’t depend on instance variables. However, they also come with drawbacks, such as difficulty in testing and increased risk of data corruption due to shared access. It’s important to carefully consider these factors when deciding to use static methods and variables.
Working with instances in Java is not without its challenges. Let’s discuss some common errors and obstacles you may encounter, along with their solutions.
Incorrect Instance Creation
A common mistake beginners make is forgetting to use the ‘new’ keyword when creating an instance. Here’s what happens if you do:
Car myCar;
myCar.color = "Red";
// Output:
// Error: java.lang.NullPointerException
In this code block, we tried to assign a color to myCar
without creating an instance. This results in a NullPointerException
. To fix this, you need to create an instance using the ‘new’ keyword:
Car myCar = new Car();
myCar.color = "Red";
// Output:
// No error
Accessing Non-Existent Variables or Methods
Another common error is trying to access a variable or method that doesn’t exist in the class. Here’s an example:
Car myCar = new Car();
myCar.price = 20000;
// Output:
// Error: java.lang.Error: Unresolved compilation problem: price cannot be resolved or is not a field
In this code block, we tried to assign a value to price
, which is not a variable in the Car
class. This results in a compilation error. To fix this, you need to ensure that the variable exists in the class:
class Car {
int price;
}
Car myCar = new Car();
myCar.price = 20000;
// Output:
// No error
Best Practices and Optimization
When working with instances in Java, it’s important to follow best practices for clean, efficient, and maintainable code. Here are some tips:
- Use meaningful names for your classes and instances. This makes your code easier to read and understand.
- Keep your classes small and focused. A class should represent a single concept or entity.
- Encapsulate your data. Make your instance variables private and provide public getter and setter methods to access and modify them.
- Avoid using static variables and methods unless necessary. They can make your code harder to test and maintain.
Working with instances in Java can be tricky, but with practice and understanding, you’ll be able to navigate these challenges and write efficient, instance-based code.
Unpacking Java’s Object-Oriented Programming
To fully grasp the concept of instances in Java, it’s crucial to understand the fundamentals of object-oriented programming (OOP) in Java. OOP is a programming paradigm that uses ‘objects’—instances of classes—to design applications and programs.
The Role of Classes
In Java, a class is like a blueprint for creating objects. It defines a data structure containing variables and methods, which the instances of the class (objects) will carry. Here’s a simple example:
// Defining a class
class Car {
String color;
String model;
}
// Output:
// This creates a class named Car with two variables: color and model.
In this code block, we defined a class Car
with two variables: color
and model
. This class can now be used to create instances.
Understanding Methods
Methods in Java are blocks of code that perform specific tasks. They can be associated with an instance of a class (instance methods) or with the class itself (static methods). Here’s an example of a method in the Car
class:
// Adding a method to Car
class Car {
String color;
String model;
void display() {
System.out.println("Car color: " + color + ", Model: " + model);
}
}
// Output:
// This adds a display() method to the Car class.
In this code block, we added a display()
method to the Car
class. This method can be used by instances of Car
to print their color and model.
The Power of Inheritance
Inheritance is a fundamental concept in OOP that allows one class to inherit the properties (variables and methods) of another. With inheritance, we can create a new class based on an existing one, leading to reusability of code and a logical, hierarchical class structure.
Understanding these fundamental concepts of OOP in Java is crucial to mastering instances. With this background, you can better understand how instances function and why they’re so important in Java programming.
Instances in Java: Beyond the Basics
As you become more comfortable with instances in Java, you’ll start to see their broader applications in larger Java projects. Instances are not standalone entities; they often interact with other concepts in Java, forming the backbone of object-oriented design.
Instances and Polymorphism
Polymorphism is a core concept in Java that allows objects of different types to be processed in a uniform way. When working with instances, you’ll often use polymorphism to create more flexible and reusable code.
// Using polymorphism with instances
class Car {
void display() {
System.out.println("This is a car");
}
}
class Sedan extends Car {
void display() {
System.out.println("This is a sedan");
}
}
Car myCar = new Sedan();
myCar.display();
// Output:
// This is a sedan
In this example, we created a Car
class and a Sedan
class that extends Car
. We then created an instance of Sedan
but assigned it to a Car
reference. When we call the display()
method, the method in Sedan
is executed, demonstrating polymorphism.
Instances and Interfaces
Interfaces in Java are used to achieve abstraction and multiple inheritance. They often work hand in hand with instances, allowing you to define a contract for classes without specifying how the class must fulfill it.
// Using interfaces with instances
interface Drivable {
void drive();
}
class Car implements Drivable {
public void drive() {
System.out.println("The car is driving");
}
}
Drivable myCar = new Car();
myCar.drive();
// Output:
// The car is driving
In this code block, we defined an interface Drivable
and a class Car
that implements Drivable
. We then created an instance of Car
and assigned it to a Drivable
reference. When we call the drive()
method, the method in Car
is executed.
Further Resources for Mastering Instances in Java
To delve deeper into instances in Java and related concepts, consider checking out these resources:
- Beginner’s Guide to Objects in Java – Discover how objects encapsulate data and behavior in Java.
Constructor Types in Java – Understand how constructors set initial values to object properties and perform initialization tasks.
instanceof in Java Overview – Explore the instanceof operator in Java for determining what an object is an instance of.
Oracle’s Java Tutorials offer comprehensive guides on instances and object-oriented programming in Java.
Instance Variable in Java blog post on MyGreatLearning provides a detailed explanation of Java instance variables.
Java Instance Variables by JavaTpoint offers a comprehensive tutorial on instance variables in Java.
With these resources and the information in this guide, you’re well on your way to mastering instances in Java and applying them in larger projects.
Wrapping Up: Mastering Instances in Java
In this comprehensive guide, we’ve delved into the concept of instances in Java, exploring their creation, usage, and related concepts. We’ve clarified what an instance in Java is, how it functions as an object created from a class, and how to create and manipulate these instances.
We began with the basics, learning how to create an instance and how to access and modify its variables. We then ventured into more advanced territory, discussing the creation of multiple instances, using instance methods, and working with instance variables. We also explored alternative approaches, such as static methods and variables, which do not require an instance to be accessed.
Along the way, we tackled common errors and obstacles you might face when working with instances in Java, providing you with solutions and best practices to overcome these challenges. We also unpacked the fundamentals of object-oriented programming in Java, as understanding this is crucial to mastering instances.
Here’s a quick comparison of instance usage at different levels:
Level | Instance Usage | Pros | Cons |
---|---|---|---|
Beginner | Basic instance creation and variable modification | Easy to understand | Limited functionality |
Intermediate | Multiple instances, instance methods, and variables | More functionality | Requires deeper understanding |
Expert | Static methods and variables | Can be accessed without an instance | Increased risk of data corruption |
Whether you’re just starting out with Java or you’re looking to deepen your understanding of instances, we hope this guide has illuminated the concept for you. Instances are a fundamental part of Java and understanding them is key to mastering the language. Happy coding!