Instanceof Java Operator: Guide to Validating Objects
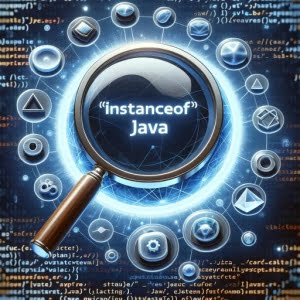
Ever felt like you’re wrestling with the ‘instanceof’ operator in Java? You’re not alone. Many developers find the ‘instanceof’ operator a bit daunting. Think of ‘instanceof’ as a detective – a detective that helps you determine an object’s type at runtime.
‘Instanceof’ is a powerful tool to check whether an object is an instance of a specific class or an interface. It’s an essential part of Java that can make your code more dynamic and flexible.
In this guide, we’ll walk you through the process of using ‘instanceof’ in Java, from the basics to more advanced techniques. We’ll cover everything from simple usage of ‘instanceof’, handling different types of objects, to dealing with common issues and even troubleshooting.
Let’s dive in and start mastering ‘instanceof’ in Java!
TL;DR: What is the ‘instanceof’ Operator in Java?
The ‘instanceof’ operator is a binary Java operator used in the form:
(object instanceof class)
It’s used to check whether an object is an instance of a specific class or an interface.
Here’s a simple example:
String s = 'Hello';
boolean result = s instanceof String;
System.out.println(result);
# Output:
# true
In this example, we have a string ‘Hello’ and we use the ‘instanceof’ operator to check if it’s an instance of the String class. The result is ‘true’, as ‘Hello’ is indeed a String.
This is just a basic usage of the ‘instanceof’ operator in Java. There’s much more to learn about it, including its advanced uses and alternatives. So, let’s dive deeper!
Table of Contents
- The Basics of ‘instanceof’ in Java
- Advanced ‘instanceof’ Usage: Subclasses and Interfaces
- Exploring Alternatives to ‘instanceof’ in Java
- Troubleshooting ‘instanceof’ in Java
- ‘instanceof’ and Object-Oriented Programming
- Expanding ‘instanceof’ Usage in Large-Scale Projects
- Wrapping Up: Mastering the ‘instanceof’ Operator in Java
The Basics of ‘instanceof’ in Java
The ‘instanceof’ operator in Java is a fundamental tool for any Java programmer. It’s a binary operator, meaning it requires two operands: an object and a class or interface. The operator checks if the object is an instance of the specified class or an implementation of the interface.
Let’s break down how to use ‘instanceof’ step-by-step:
- Declare and initialize an object.
String myString = 'Hello, Java!';
In this case, we’ve created a String object named ‘myString’.
- Use the ‘instanceof’ operator to check the type of the object.
boolean check = myString instanceof String;
System.out.println(check);
# Output:
# true
Here, we’re checking if ‘myString’ is an instance of the String class. The result is ‘true’, as ‘myString’ is indeed a String.
When and Why to Use ‘instanceof’
The ‘instanceof’ operator is most commonly used in situations where you need to verify the type of an object before performing operations on it. This can be particularly useful when dealing with collections of objects that may contain different types, or when implementing Java’s polymorphism and inheritance features.
For example, consider a List that contains a mix of String and Integer objects. Before performing a string-specific operation, you’d want to ensure the object is indeed a String. Using ‘instanceof’, you can avoid a ClassCastException.
Object myObject = list.get(i);
if (myObject instanceof String) {
String myString = (String) myObject;
// Perform string-specific operation
}
# Output:
# If myObject is a String, the operation is executed. If not, the program continues without throwing an exception.
In this example, ‘instanceof’ ensures we only attempt to cast and operate on ‘myObject’ if it’s a String, preventing potential runtime errors.
Advanced ‘instanceof’ Usage: Subclasses and Interfaces
As you gain more experience with Java, you’ll likely encounter situations where you need to check if an object is an instance of a subclass or an interface. Here, ‘instanceof’ truly shines.
Checking for Subclasses
In Java, a subclass is a class that inherits from another class, known as the superclass. The ‘instanceof’ operator can be used to check whether an object is an instance of a subclass.
Here’s an example:
// Define superclass and subclass
class SuperClass {}
class SubClass extends SuperClass {}
// Create an instance of SubClass
SubClass subInstance = new SubClass();
// Check if it's an instance of SuperClass
boolean result = subInstance instanceof SuperClass;
System.out.println(result);
# Output:
# true
In this example, ‘SubClass’ is a subclass of ‘SuperClass’. When we check if ‘subInstance’ (an instance of SubClass) is an instance of ‘SuperClass’, the result is ‘true’. This is because a subclass is considered an instance of its superclass.
Checking for Interface Implementation
Interfaces in Java are a way to achieve abstraction. They can have methods and variables, but the methods declared in interface are by default abstract. An interface can’t be instantiated, but it can be implemented by classes or extended by other interfaces.
The ‘instanceof’ operator can be used to check if an object’s class implements a specific interface. Let’s look at an example:
// Define an interface and a class that implements it
interface MyInterface {}
class MyClass implements MyInterface {}
// Create an instance of MyClass
MyClass myInstance = new MyClass();
// Check if it's an instance of MyInterface
boolean result = myInstance instanceof MyInterface;
System.out.println(result);
# Output:
# true
In this example, ‘MyClass’ implements the ‘MyInterface’ interface. When we check if ‘myInstance’ (an instance of MyClass) is an instance of ‘MyInterface’, the result is ‘true’. This is because a class that implements an interface is considered an instance of that interface.
In both these cases, ‘instanceof’ allows us to check for more than just direct class membership. It enables us to work with Java’s powerful inheritance and interface systems more effectively.
Exploring Alternatives to ‘instanceof’ in Java
While ‘instanceof’ is a powerful tool for type checking in Java, it’s not the only one. There are alternative approaches, such as using reflection or the ‘getClass()’ method. Let’s explore these alternatives in more detail.
Reflection in Java
Reflection is a feature in Java that allows an executing Java program to examine or ‘introspect’ upon itself, and manipulate internal properties of the program. It can be used to determine the class of an object.
Here’s an example:
String myString = 'Hello, Java!';
Class stringClass = myString.getClass();
System.out.println(stringClass.getName());
# Output:
# java.lang.String
In this example, we use the ‘getClass()’ method to obtain the Class object that represents the runtime class of ‘myString’. We then print the name of the class, which is ‘java.lang.String’.
While reflection can be powerful, it also has its drawbacks. It can be slower than other methods and can potentially compromise security and design principles.
The ‘getClass()’ Method
The ‘getClass()’ method returns the runtime class of an object. This can be used to check if two objects are of the same class.
Here’s an example:
String string1 = 'Hello, Java!';
String string2 = 'Hello, World!';
boolean result = string1.getClass() == string2.getClass();
System.out.println(result);
# Output:
# true
In this example, we have two String objects. We use the ‘getClass()’ method to compare their runtime classes. The result is ‘true’ because both objects are instances of the String class.
The ‘getClass()’ method is straightforward and fast, making it a good alternative to ‘instanceof’ in certain situations. However, it only checks for the exact class of an object, not its superclass or interfaces.
Making the Right Choice
Choosing between ‘instanceof’, reflection, and ‘getClass()’ depends on your specific use case. If you need to check for superclass or interface membership, ‘instanceof’ is the way to go. If you need to manipulate or obtain information about classes, methods, and fields at runtime, reflection is a powerful tool. If you simply need to check if two objects are of the same class, ‘getClass()’ can be a quick and easy solution.
As with all tools in programming, understanding the benefits and drawbacks of each option will help you make the best decision for your specific needs.
Troubleshooting ‘instanceof’ in Java
While ‘instanceof’ is a powerful tool, like any feature of a programming language, it comes with its own set of potential pitfalls. Let’s discuss common issues you might encounter while using ‘instanceof’ and how to solve them.
Dealing with Null References
One common issue is dealing with null references. If you try to use ‘instanceof’ on a null reference, it doesn’t throw a NullPointerException. Instead, it simply returns false. This is because null is not an instance of any class.
Here’s an example:
String myString = null;
boolean result = myString instanceof String;
System.out.println(result);
# Output:
# false
In this example, ‘myString’ is null. When we check if it’s an instance of the String class, the result is ‘false’.
Incorrect Type Casting
Another common issue is incorrect type casting. If you try to cast an object to a type that it’s not an instance of, Java throws a ClassCastException. You can use ‘instanceof’ to prevent this.
Here’s an example:
Object myObject = 'Hello, Java!';
if (myObject instanceof Integer) {
Integer myInteger = (Integer) myObject;
} else {
System.out.println('Cannot cast to Integer.');
}
# Output:
# Cannot cast to Integer.
In this example, ‘myObject’ is a String. We use ‘instanceof’ to check if it can be cast to an Integer. Since it can’t, we print a message instead of throwing a ClassCastException.
Best Practices
When using ‘instanceof’, it’s best practice to use it sparingly and always as a last resort. Overuse of ‘instanceof’ can lead to code that is hard to maintain and debug. It’s often better to use polymorphism and method overriding, which are more in line with object-oriented programming principles.
‘instanceof’ and Object-Oriented Programming
To fully grasp the ‘instanceof’ operator in Java, it’s crucial to understand some fundamental concepts of object-oriented programming (OOP), namely inheritance and polymorphism. Let’s delve into these concepts and see how they relate to ‘instanceof’.
Inheritance in Java
Inheritance is one of the four fundamental OOP concepts. The idea is that you can create new classes that are built upon existing classes. When you inherit from an existing class, you can reuse methods and fields from the parent class, and you can add new methods and fields to adapt your new class towards a specific need.
// Parent class
public class Animal {
public void eat() {
System.out.println('The animal eats.');
}
}
// Child class
public class Dog extends Animal {
public void bark() {
System.out.println('The dog barks.');
}
}
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
# Output:
# 'The animal eats.'
# 'The dog barks.'
In this example, ‘Dog’ is a subclass (or child class) of ‘Animal’. It inherits the ‘eat()’ method from ‘Animal’ and also defines a new method called ‘bark()’. An instance of ‘Dog’ can call both methods.
Polymorphism in Java
Polymorphism is another fundamental concept of OOP. It allows objects of different types to be accessed through the same interface. Each type can provide its own implementation of the interface. One of the key benefits of polymorphism is that it offers flexibility while still ensuring class independence.
public class Animal {
public void sound() {
System.out.println('The animal makes a sound.');
}
}
public class Dog extends Animal {
public void sound() {
System.out.println('The dog barks.');
}
}
public class Cat extends Animal {
public void sound() {
System.out.println('The cat meows.');
}
}
Animal myAnimal = new Dog();
myAnimal.sound();
myAnimal = new Cat();
myAnimal.sound();
# Output:
# 'The dog barks.'
# 'The cat meows.'
In this example, ‘Dog’ and ‘Cat’ are subclasses of ‘Animal’. They both override the ‘sound()’ method. An ‘Animal’ reference can be used to call the ‘sound()’ method, and the correct version of the method is called based on the actual object type.
‘instanceof’ in the Context of OOP
The ‘instanceof’ operator in Java fits right into the concept of inheritance and polymorphism. It allows you to check whether an object is an instance of a specific class or an interface. It can be used to ensure type compatibility before performing operations, to choose between different possible pathways in a program, or to do more complex type-related logic. It’s a powerful tool in the arsenal of any Java programmer, and understanding it deeply can help you write more robust and flexible code.
Expanding ‘instanceof’ Usage in Large-Scale Projects
The ‘instanceof’ operator is not just for simple type checking. Its utility extends to larger projects, especially when dealing with design patterns and exception handling.
‘instanceof’ in Design Patterns
Design patterns represent the best practices used by experienced object-oriented software developers. They are solutions to general problems that software developers faced during software development. These solutions are highly flexible and can be used in the context of ‘instanceof’.
For example, in the Factory pattern, ‘instanceof’ can be used to check the type of objects created by the factory before performing operations on them.
Exception Handling with ‘instanceof’
In Java, exceptions are events that disrupt the normal flow of program instructions. ‘instanceof’ can be used in exception handling to check the type of an exception and handle it accordingly.
Here’s an example:
try {
// Code that may throw an exception
} catch (Exception e) {
if (e instanceof IOException) {
System.out.println('Caught an IOException.');
} else {
System.out.println('Caught an exception.');
}
}
# Output:
# If an IOException is thrown, 'Caught an IOException.' is printed. Otherwise, 'Caught an exception.' is printed.
In this example, we use a try-catch block to handle exceptions. If an exception is thrown, we use ‘instanceof’ to check if it’s an IOException. Depending on the type of the exception, we print a different message.
Further Resources for Mastering ‘instanceof’ in Java
For more info on Java Objects and to learn how objects interact with each other in Java programs, Click Here!
To further your understanding of ‘instanceof’ in Java, consider exploring these resources:
- Exploring Java Instance Concepts – Learn how instances store the state of objects and provide access to their behavior.
Understanding Getter and Setter Methods – Learn how getter and setter methods ensure data encapsulation.
Oracle Java Documentation provides a comprehensive overview of the ‘instanceof’ operator.
Baeldung’s Java ‘instanceof’ Tutorial provides a deep dive into ‘instanceof’, including its usage and best practices.
GeeksforGeeks’ Java ‘instanceof’ Article covers ‘instanceof’, as well as more advanced topics like downcasting.
Wrapping Up: Mastering the ‘instanceof’ Operator in Java
In this comprehensive guide, we’ve delved into the ‘instanceof’ operator in Java, a powerful tool for determining an object’s type at runtime.
We started with the basics, learning how to use ‘instanceof’ to check whether an object is an instance of a specific class or interface. We then explored more advanced uses, such as checking for instances of subclasses or interfaces, and discussed alternative approaches like using reflection or the ‘getClass()’ method.
Throughout the guide, we addressed common issues that you might encounter when using ‘instanceof’, such as dealing with null references and incorrect type casting, and provided solutions to help you navigate these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity |
---|---|---|
‘instanceof’ | High | Low |
Reflection | Very High | High |
‘getClass()’ | Moderate | Low |
Whether you’re just starting out with Java or you’re an experienced programmer looking to deepen your understanding of type checking, we hope this guide has given you a comprehensive overview of the ‘instanceof’ operator and its uses.
Understanding ‘instanceof’ and other type checking tools in Java is crucial for writing robust and flexible code. With the knowledge you’ve gained from this guide, you’re well-equipped to handle a variety of programming challenges. Happy coding!