What is an Object Java: A Detailed Exploration
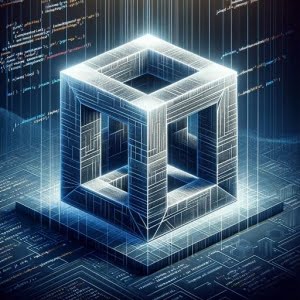
Are you finding it challenging to understand objects in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling objects in Java, but we’re here to help.
Think of an object in Java as a ‘worker’ that can perform tasks and store information. It’s like a tiny machine inside your code that can do a lot of work for you.
In this guide, we’ll help you understand what objects are in Java, how to create and use them, and why they are a fundamental part of Java programming. We’ll cover everything from the basics of creating and using objects to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start exploring objects in Java!
TL;DR: What is an Object in Java?
An object in Java is an instance of a class that can perform actions and store data, created with the syntax:
MyClass myObject = new MyClass()
. It’s a fundamental part of Java programming that allows you to encapsulate related data and behavior into a single entity.
Here’s a simple example of creating an object in Java:
class Vehicle {
// class body
}
Vehicle car = new Vehicle();
# Output:
# This code creates an instance of Vehicle class named car.
In this example, we define a class Vehicle
, then create a new object car
from the Vehicle
class.
This is a basic way to create and use objects in Java, but there’s much more to learn about object-oriented programming in Java. Continue reading for a more detailed explanation and advanced usage of objects in Java.
Table of Contents
Creating and Using Objects in Java
In Java, objects are created from classes. A class is a blueprint or prototype that defines the variables and methods common to all objects of a certain kind.
Here’s a simple example of creating an object in Java:
class MyClass {
// class body
}
MyClass myObject = new MyClass();
# Output:
# This code creates an instance of MyClass named myObject.
In the above example, MyClass
is a class and myObject
is an object of MyClass
. The new
keyword is used to create a new instance of a class.
Calling Methods on Objects
Objects in Java can have methods. Methods are functions that are defined inside a class. They describe the behaviors of an object.
Here’s how you can define a method in a class and call it on an object:
class MyClass {
void myMethod() {
System.out.println("Method called");
}
}
MyClass myObject = new MyClass();
myObject.myMethod();
# Output:
# Method called
In the above example, myMethod
is a method defined in MyClass
. We call this method on the myObject
object using the dot (.
) operator.
Accessing Object Attributes
Objects in Java can have attributes. Attributes are variables that are defined inside a class. They represent the state of an object.
Here’s how you can define an attribute in a class and access it from an object:
class MyClass {
String myAttribute = "Hello";
}
MyClass myObject = new MyClass();
System.out.println(myObject.myAttribute);
# Output:
# Hello
In the above example, myAttribute
is an attribute defined in MyClass
. We access this attribute from the myObject
object using the dot (.
) operator.
Advanced Object Usage in Java
As you delve deeper into Java programming, you’ll encounter more complex ways to use objects. Let’s explore some of these advanced uses.
Creating Multiple Objects
In Java, you can create multiple objects from the same class. Each object will have its own copy of the class’s attributes and methods.
class MyClass {
String myAttribute = "Hello";
}
MyClass myObject1 = new MyClass();
MyClass myObject2 = new MyClass();
System.out.println(myObject1.myAttribute);
System.out.println(myObject2.myAttribute);
# Output:
# Hello
# Hello
In the above example, we create two objects, myObject1
and myObject2
, from the MyClass
class. Each object has its own myAttribute
.
Objects as Method Parameters and Return Types
Objects can be used as method parameters and return types. This allows for more complex interactions between objects.
class MyClass {
String myAttribute;
MyClass(String attribute) {
myAttribute = attribute;
}
void displayAttribute(MyClass obj) {
System.out.println(obj.myAttribute);
}
MyClass returnObject() {
MyClass newObj = new MyClass("Goodbye");
return newObj;
}
}
MyClass myObject = new MyClass("Hello");
myObject.displayAttribute(myObject);
MyClass returnedObject = myObject.returnObject();
System.out.println(returnedObject.myAttribute);
# Output:
# Hello
# Goodbye
In the above example, the displayAttribute
method accepts an object as a parameter, and the returnObject
method returns an object.
Objects in Arrays and Collections
Objects can be stored in arrays and collections. This allows for efficient organization and manipulation of multiple objects.
class MyClass {
String myAttribute;
MyClass(String attribute) {
myAttribute = attribute;
}
}
MyClass[] myObjects = new MyClass[2];
myObjects[0] = new MyClass("Hello");
myObjects[1] = new MyClass("World");
for (MyClass obj : myObjects) {
System.out.println(obj.myAttribute);
}
# Output:
# Hello
# World
In the above example, we create an array of MyClass
objects and iterate over it using a for-each loop.
Exploring Alternative Object Usage in Java
Java offers a plethora of ways to use objects, giving you the flexibility to choose the most suitable approach for your specific needs. Let’s explore some of these alternative object usage techniques.
Anonymous Objects
In Java, you can create anonymous objects—objects that don’t have a reference variable. These objects are useful for one-time usage.
class MyClass {
void myMethod() {
System.out.println("Method called");
}
}
new MyClass().myMethod();
# Output:
# Method called
In the above example, we create an anonymous object of MyClass
and call the myMethod
method on it. This approach is beneficial when you need to use an object only once, but it’s not suitable for situations where the object needs to be referenced multiple times.
Nested Objects
In Java, you can nest objects within other objects. This allows for complex data structures and can improve code organization.
class OuterClass {
String outerAttribute = "Hello";
class InnerClass {
String innerAttribute = "World";
}
}
OuterClass outerObject = new OuterClass();
OuterClass.InnerClass innerObject = outerObject.new InnerClass();
System.out.println(outerObject.outerAttribute);
System.out.println(innerObject.innerAttribute);
# Output:
# Hello
# World
In the above example, we create an InnerClass
inside OuterClass
and create objects of both classes. The innerObject
is nested within outerObject
. This approach can lead to more organized and readable code, but it can also make the code more complex and harder to understand for beginners.
Troubleshooting Common Issues with Java Objects
Working with objects in Java can sometimes lead to issues, especially for beginners. Here, we’ll discuss some common problems and how to tackle them.
Null Pointer Exceptions
A common issue when working with objects in Java is the Null Pointer Exception. This occurs when you try to access a method or attribute of an object that hasn’t been initialized.
class MyClass {
String myAttribute;
}
MyClass myObject = null;
System.out.println(myObject.myAttribute);
# Output:
# Exception in thread "main" java.lang.NullPointerException
In the above example, we try to access myAttribute
from myObject
, which is null
. This results in a Null Pointer Exception.
To avoid this, always ensure your objects are properly initialized before you try to use them.
Class Casting Exceptions
Another common issue is the ClassCastException. This occurs when you try to cast an object of one type to another incompatible type.
class MyClass1 {}
class MyClass2 {}
MyClass1 myObject1 = new MyClass1();
MyClass2 myObject2 = (MyClass2) myObject1;
# Output:
# Exception in thread "main" java.lang.ClassCastException: MyClass1 cannot be cast to MyClass2
In the above example, we try to cast myObject1
of type MyClass1
to MyClass2
, which is not possible. This results in a ClassCastException.
To avoid this, always ensure that the object you’re casting is compatible with the new type.
By understanding these common issues when working with objects in Java, you can write more robust and error-free code.
Object-Oriented Programming in Java
Java is fundamentally an object-oriented programming (OOP) language. Understanding OOP is crucial to mastering Java objects, as it provides the framework within which objects operate.
Encapsulation
Encapsulation is the principle of bundling the data (attributes) and the methods that operate on the data into a single unit, i.e., a class. When an object is created from that class, it can access and manipulate the data.
class MyClass {
private String myAttribute;
public String getMyAttribute() {
return myAttribute;
}
public void setMyAttribute(String myAttribute) {
this.myAttribute = myAttribute;
}
}
MyClass myObject = new MyClass();
myObject.setMyAttribute("Hello");
System.out.println(myObject.getMyAttribute());
# Output:
# Hello
In the above example, myAttribute
is encapsulated in MyClass
. It’s marked as private
, so it can only be accessed and modified through the getMyAttribute
and setMyAttribute
methods.
Inheritance
Inheritance is the principle that allows a class to inherit attributes and methods from another class. This promotes code reusability and hierarchical classifications.
class ParentClass {
String parentAttribute = "Hello";
}
class ChildClass extends ParentClass {
String childAttribute = "World";
}
ChildClass myObject = new ChildClass();
System.out.println(myObject.parentAttribute + " " + myObject.childAttribute);
# Output:
# Hello World
In the above example, ChildClass
inherits parentAttribute
from ParentClass
. Thus, an object of ChildClass
can access both parentAttribute
and childAttribute
.
Polymorphism
Polymorphism is the principle that allows an object to take on many forms. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object.
class ParentClass {
void myMethod() {
System.out.println("Parent's method");
}
}
class ChildClass extends ParentClass {
void myMethod() {
System.out.println("Child's method");
}
}
ParentClass myObject = new ChildClass();
myObject.myMethod();
# Output:
# Child's method
In the above example, myObject
is of ParentClass
type but refers to an object of ChildClass
. When we call myMethod
, the child’s version of the method is called, demonstrating polymorphism.
Understanding these fundamental principles of OOP is essential to effectively create and use objects in Java.
Advanced Java Topics: The Importance of Objects
Mastering objects in Java is not just about understanding the basics. It opens the door to more advanced Java topics, including design patterns, frameworks, and APIs. Let’s delve into why understanding objects is crucial for these advanced topics.
Design Patterns and Objects
Design patterns in Java are standard solutions to common problems in software design. These patterns are templates that can be applied to real-world programming scenarios. A deep understanding of objects in Java is crucial for implementing these design patterns effectively. For example, the Singleton pattern relies on the concept of limiting object creation to a single instance.
Frameworks and Objects
Frameworks in Java, like Spring and Hibernate, heavily rely on objects and the principles of object-oriented programming. These frameworks use objects to represent and manipulate data, and understanding objects is key to utilizing these frameworks to their full potential.
APIs and Objects
Java APIs, like the Java Collections API and the Java Stream API, provide pre-defined classes and methods to perform various operations. These APIs are object-oriented, and understanding how objects work in Java is vital to using these APIs effectively.
Further Resources for Mastering Java Objects
To help you further enhance your understanding of objects in Java, here are some resources that you might find useful:
- Exploring Java Class Basics – Learn how Java classes encapsulate data and behavior.
Getter and Setter in Java – Understand the getter and setter methods in Java for accessing and modifying objects.
Oracle’s Java Tutorials provide explanations on various Java topics, including objects.
Java Design Patterns by JournalDev dives into various Java design patterns
Spring Framework Guides can help you get started with the popular Spring Framework, which heavily uses objects.
Remember, understanding objects in Java is a journey. The more you practice and learn, the more comfortable you’ll become with using objects in your Java programming.
Wrapping Up: Mastering Objects in Java
In this comprehensive guide, we’ve delved into the world of objects in Java, a fundamental aspect of Java programming.
We began with the basics, learning how to create and use objects in Java. We then ventured into more advanced territory, exploring complex uses of objects, such as creating multiple objects, using objects as method parameters and return types, and storing objects in arrays and collections.
We also explored alternative approaches to using objects in Java, such as creating anonymous objects and nesting objects within other objects. Along the way, we tackled common challenges you might face when working with objects in Java, such as null pointer exceptions and class casting exceptions, providing you with solutions to these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Basic Use | Simple and easy to understand | Limited functionality |
Advanced Use | More powerful and flexible | More complex and challenging to understand |
Alternative Approaches | Provides additional flexibility | May be more complex and less commonly used |
Whether you’re just starting out with Java or you’re looking to level up your object-oriented programming skills, we hope this guide has given you a deeper understanding of objects in Java and their importance in Java programming.
With a solid understanding of objects in Java, you’re well on your way to becoming a proficient Java programmer. Keep practicing and exploring, and you’ll continue to grow your skills. Happy coding!