Adding Elements to an Array in Java: A How-To Guide
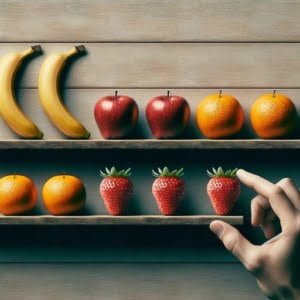
Do you find yourself wrestling with adding elements to an array in Java? You’re not alone. Many developers find this task a bit tricky. But think of Java arrays as a train that can add more carriages, accommodating more passengers, or in this case, elements.
This guide will walk you through the process of adding elements to an array in Java, from the basics to more advanced techniques. We’ll cover everything from using the Arrays.copyOf()
method, leveraging Java collections like ArrayList, to exploring alternative approaches for more flexible array manipulation.
So, let’s get started and master the art of adding elements to an array in Java!
TL;DR: How Do I Add Elements to an Array in Java?
In Java, arrays are fixed in size, so you can’t directly add elements to them. However, you can create a new, larger array and copy the old elements and the new element into it. This method utilizes the
.copyOf
method, with the syntax:newArray = Arrays.copyOf(oldArray, oldArray.length + 1);
. For more flexible array manipulation, consider using ArrayList or other Java collections.
Here’s a simple example:
char[] oldArray = {'a', 'b', 'c'};
char[] newArray = Arrays.copyOf(oldArray, oldArray.length + 1);
newArray[oldArray.length] = 'd';
// Output:
// newArray = {'a', 'b', 'c', 'd'}
In this example, we’ve created a new array that is one element larger than the old array. We then copied the elements from the old array into the new one using the Arrays.copyOf()
method. Finally, we added the new element at the end of the new array.
This is a basic way to add elements to an array in Java, but there’s much more to learn about array manipulation in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Basic Use: Adding Elements with Arrays.copyOf()
When you’re just starting out with Java, the most straightforward way to add elements to an array is by using the Arrays.copyOf()
method. This method allows you to create a new array with a larger size, then copy the elements from the old array to the new one.
Here’s a simple example:
int[] oldArray = {1, 2, 3};
int[] newArray = Arrays.copyOf(oldArray, oldArray.length + 1);
newArray[oldArray.length] = 4;
// Output:
// newArray = {1, 2, 3, 4}
In this code block, we first define an old array with three elements. We then create a new array that is one element larger. The Arrays.copyOf()
method is used to copy the old array into the new one. Finally, we add a new element to the end of the new array.
Advantages and Pitfalls of Arrays.copyOf()
While this method is simple and effective for small arrays or occasional use, it has its limitations. The main advantage is its simplicity and readability: the code is easy to understand even for beginners.
However, the downside is that it’s not efficient for large arrays or frequent additions. Each time you add an element, a new array is created, which can be memory-intensive and slow. Additionally, arrays in Java are fixed in size, so you can’t add or remove elements from an existing array without creating a new one.
Advanced Use: Embracing ArrayList for Flexibility
As you grow more comfortable with Java, you’ll find that the ArrayList
class provides a more flexible way to handle arrays. Unlike standard arrays, ArrayLists are dynamic and can easily accommodate additional elements without the need to create a new array.
Here’s an example of how you can use ArrayList to add elements to an array:
import java.util.ArrayList;
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
arrayList.add(4);
// Output:
// arrayList = [1, 2, 3, 4]
In this code block, we first import the ArrayList
class. We then create an ArrayList and add elements to it using the add()
method. The add()
method allows us to add elements to the ArrayList without worrying about its size.
The Power of ArrayList: Differences and Best Practices
The primary difference between using ArrayList
and standard arrays is the dynamic nature of ArrayLists. They can grow and shrink during runtime, providing a more flexible approach to handling arrays.
However, ArrayLists do come with their own set of considerations. While they offer more flexibility, they can be slower than standard arrays due to the overhead of object methods. Therefore, if performance is a concern and you know the size of your array upfront, a standard array might be a better choice.
In general, it’s best to use ArrayLists when you need a flexible and easy-to-use data structure for storing elements, and standard arrays when dealing with a fixed number of elements or when performance is a critical factor.
Alternative Approaches: Exploring Beyond the Basics
While Arrays.copyOf()
and ArrayList
are common methods to add elements to an array in Java, there are other advanced techniques that can provide more control or efficiency. Let’s delve into these alternative approaches.
Using Java’s System.arraycopy()
Java’s System.arraycopy()
method is another way to add elements to an array. This method copies an array from the specified source array to the destination array. Here’s an example:
int[] oldArray = {1, 2, 3};
int[] newArray = new int[oldArray.length + 1];
System.arraycopy(oldArray, 0, newArray, 0, oldArray.length);
newArray[oldArray.length] = 4;
// Output:
// newArray = {1, 2, 3, 4}
In this code block, we first define an old array and a new array that is one element larger. We then use System.arraycopy()
to copy the elements from the old array to the new one. Finally, we add a new element to the end of the new array.
Leveraging Third-Party Libraries
Third-party libraries, such as Apache Commons Lang, also provide methods to add elements to an array. The ArrayUtils.add()
method from Apache Commons Lang is a popular choice. However, this requires adding the library to your project, which may not always be desirable.
// Requires: import org.apache.commons.lang3.ArrayUtils;
int[] oldArray = {1, 2, 3};
int[] newArray = ArrayUtils.add(oldArray, 4);
// Output:
// newArray = {1, 2, 3, 4}
In this example, we use the ArrayUtils.add()
method to add a new element to the array. The method automatically creates a new array that includes the new element.
Weighing the Pros and Cons
While these alternative methods offer more options, they each come with their own benefits and drawbacks. System.arraycopy()
provides a fast and native way to copy arrays, but it doesn’t inherently add elements to an array. On the other hand, third-party libraries like Apache Commons Lang offer easy-to-use methods, but they add extra dependencies to your project.
In conclusion, the best method to add elements to an array in Java depends on your specific needs and constraints. Whether it’s the simplicity of Arrays.copyOf()
, the flexibility of ArrayList
, the control of System.arraycopy()
, or the convenience of third-party libraries, Java offers a variety of ways to achieve your goal.
Troubleshooting Java Array Additions
While adding elements to an array in Java might seem straightforward, it can sometimes lead to unexpected issues. Let’s discuss some common problems and their solutions.
Handling ArrayIndexOutOfBoundsException
One common issue when working with arrays is the ArrayIndexOutOfBoundsException
. This exception is thrown to indicate that an array has been accessed with an illegal index. The index is either negative or greater than or equal to the size of the array.
For example, consider the following code:
int[] array = {1, 2, 3};
array[3] = 4;
// Output:
// Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 3 out of bounds for length 3
In this code block, we try to add an element to the array at index 3. However, the array’s length is only 3, meaning valid indices are 0, 1, and 2. Thus, trying to access index 3 throws an ArrayIndexOutOfBoundsException
.
To avoid this, always ensure that the index at which you’re trying to add an element is within the array’s bounds.
Tips for Successful Array Manipulation
When adding elements to an array in Java, keep the following tips in mind:
- Always check the array’s length before trying to add an element to avoid
ArrayIndexOutOfBoundsException
. - Consider using
ArrayList
or other Java collections for more flexible and efficient array manipulation. - When performance is a concern, consider using
System.arraycopy()
or similar methods for faster array copying. - Don’t forget to import necessary classes or packages, like
java.util.ArrayList
ororg.apache.commons.lang3.ArrayUtils
, before using them.
By understanding the common issues and their solutions, you can add elements to a Java array efficiently and effectively.
Understanding Java Arrays and Collections
Before we delve further into adding elements to an array in Java, it’s important to understand the basics of arrays in Java and how Java collections can offer more flexibility.
The Nature of Java Arrays
In Java, an array is a static data structure, meaning its size is fixed once it’s created. Each element in the array has an index, starting from 0 and going up to the array’s length minus one.
Here’s a simple example of declaring and initializing an array in Java:
int[] array = new int[3];
array[0] = 1;
array[1] = 2;
array[2] = 3;
// Output:
// array = {1, 2, 3}
In this example, we create an array of integers with a length of 3 and then populate it with the numbers 1, 2, and 3. Note that the indices of the array start at 0 and go up to 2, which is the length of the array (3) minus one.
The static nature of arrays in Java means that you can’t add or remove elements from an existing array without creating a new one. This is where Java collections come into play.
The Flexibility of Java Collections
Java collections, like ArrayList, are dynamic data structures that can grow and shrink at runtime, offering much more flexibility than standard arrays.
Here’s an example of declaring and initializing an ArrayList in Java:
import java.util.ArrayList;
ArrayList<Integer> arrayList = new ArrayList<>();
arrayList.add(1);
arrayList.add(2);
arrayList.add(3);
// Output:
// arrayList = [1, 2, 3]
In this example, we create an ArrayList and add the numbers 1, 2, and 3 to it. The add()
method allows us to add elements to the ArrayList without worrying about its size.
While ArrayLists and other Java collections offer more flexibility than standard arrays, they can be slower due to the overhead of object methods. Therefore, the choice between using arrays or collections in Java often depends on your specific needs and constraints.
The Impact of Array Manipulation in Java Programming
Array manipulation, including adding elements to an array in Java, is more than just a programming exercise. It’s an integral part of many real-world applications in data processing, algorithms, and more.
Array Manipulation in Data Processing
In data processing, arrays are used to store and manipulate large amounts of data efficiently. Adding elements to an array can be a common operation in tasks like data cleaning, where new data is constantly being incorporated.
The Role of Arrays in Algorithms
Arrays also play a crucial role in algorithms. They provide a way to store multiple values in a single variable, which can be accessed and manipulated as needed. This makes arrays a powerful tool in the development of sophisticated algorithms.
Exploring Related Concepts
Once you’re comfortable with adding elements to an array in Java, there are many related concepts to explore. Multi-dimensional arrays, for example, are arrays of arrays, which can be used to represent complex data structures like matrices. Java collections, such as ArrayList, offer more flexibility and functionality than standard arrays.
Further Resources for Mastering Java Arrays
To deepen your understanding of arrays in Java, here are some valuable resources:
- IOFlood’s Java Arrays Guide explains how to iterate through arrays using loops and iterators in Java.
Essential Array Methods in Java – Master utilizing array methods to streamline array processing tasks in Java.
Printing an Array in Java – Understand different approaches for printing arrays in Java.
Oracle’s Java Tutorials provide a comprehensive introduction to arrays in Java.
GeeksforGeeks’ Java Array Guide offers a deep dive into Java arrays, including various methods to manipulate them.
Baeldung’s Guide on Java Arrays explores more advanced topics, including multi-dimensional arrays and popular array methods.
By understanding the fundamental concepts and practicing with real-world examples, you can master the art of adding elements to an array in Java and beyond.
Wrapping Up:
In this comprehensive guide, we’ve journeyed through the process of adding elements to an array in Java, exploring various methods and their implications.
We began with the basics, learning how to add elements to an array using the Arrays.copyOf()
method. We then delved into more advanced techniques, such as using the ArrayList
class for more flexible array manipulation. Along the way, we explored alternative approaches, like using System.arraycopy()
method or leveraging third-party libraries like Apache Commons Lang.
We also tackled common challenges that you might encounter when adding elements to an array in Java, such as ArrayIndexOutOfBoundsException
, and offered solutions to these issues. Furthermore, we discussed the fundamental concepts of arrays and collections in Java, providing a solid foundation for understanding array manipulation.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Arrays.copyOf() | Simple, readable | Not efficient for large arrays or frequent additions |
ArrayList | Flexible, dynamic size | Slower than standard arrays due to overhead of object methods |
System.arraycopy() | Fast, native | Does not inherently add elements to an array |
Third-party libraries (e.g., Apache Commons Lang) | Easy-to-use methods | Adds extra dependencies to your project |
Whether you’re a beginner just starting out with Java or an experienced developer looking to sharpen your skills, we hope this guide has deepened your understanding of adding elements to an array in Java.
Adding elements to an array is a fundamental operation in Java programming, and mastering it will undoubtedly enhance your data manipulation abilities. Now, you’re well equipped to tackle array manipulations in Java. Happy coding!