Printing an Array in Java: A Guide For Printing to Screen
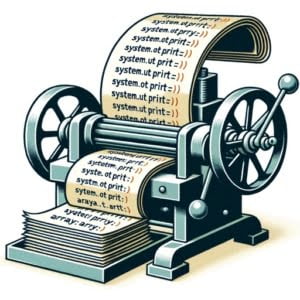
Are you finding it challenging to print arrays in Java? You’re not alone. Many developers find themselves in a maze when it comes to handling arrays in Java, but we’re here to help.
Think of printing an array in Java as unveiling a hidden treasure chest – each element revealing its own unique value, contributing to the overall worth of the array.
This guide will walk you through the process of printing an array in Java, from the basics to more advanced techniques. We’ll cover everything from simple array printing to handling multi-dimensional arrays, as well as alternative approaches.
Let’s dive in and start mastering array printing in Java!
TL;DR: How Do I Print an Array in Java?
To print an array in Java, you can use the
Arrays.toString()
method. This method converts the array into a string format that can be printed usingSystem.out.println()
.
Here’s a simple example:
int[] array = {1, 2, 3};
System.out.println(Arrays.toString(array));
# Output:
# [1, 2, 3]
In this example, we’ve created an integer array named ‘array’ with elements 1, 2, and 3. We then use the Arrays.toString()
method to convert this array into a string format that can be printed. The System.out.println()
method is then used to print this string to the console, resulting in the output ‘[1, 2, 3]’.
This is a basic way to print an array in Java, but there’s much more to learn about handling arrays in Java. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Unveiling Java Arrays with Arrays.toString()
- Navigating Multi-Dimensional Arrays with Arrays.deepToString()
- Exploring Alternatives: Java 8’s Stream API and For-Each Loop
- Navigating Common Pitfalls in Array Printing
- Understanding Arrays in Java
- Diving Into the Arrays Class
- The Bigger Picture: Arrays in Data Analysis and Algorithms
- Wrapping Up: Array Printing in Java
Unveiling Java Arrays with Arrays.toString()
The Arrays.toString()
method is your go-to tool for printing arrays in Java. This method belongs to the java.util.Arrays
class and is used to return a string representation of the contents of the specified array.
Here’s how you use it:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
System.out.println(Arrays.toString(array));
}
}
# Output:
# [1, 2, 3, 4, 5]
In this example, we’ve imported the java.util.Arrays
class, which contains various methods for manipulating arrays. We’ve then declared an integer array named ‘array’ with five elements. The Arrays.toString()
method is used to convert the array into a string format, and System.out.println()
prints this string to the console.
Advantages and Pitfalls of Arrays.toString()
The Arrays.toString()
method is straightforward and easy to use, making it ideal for beginners. It quickly converts the entire array into a string format, saving you the trouble of iterating over each element of the array.
However, it’s important to note that this method is best suited for one-dimensional arrays. When used with multi-dimensional arrays, Arrays.toString()
will not provide the desired output. Instead, it will print the internal memory addresses of the second level arrays, which is not typically useful.
For instance, observe the output when we try to print a two-dimensional array using Arrays.toString()
:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[][] array = {{1, 2, 3}, {4, 5, 6}};
System.out.println(Arrays.toString(array));
}
}
# Output:
# [[I@15db9742, [I@6d06d69c]
As you can see, the output is not the actual contents of the array but internal memory addresses. In the next section, we’ll cover how to correctly print multi-dimensional arrays in Java.
When dealing with multi-dimensional arrays in Java, Arrays.toString()
falls short. It prints out memory addresses instead of the array’s content. This is where Arrays.deepToString()
comes into play.
The Arrays.deepToString()
method is another handy tool from the java.util.Arrays
class. It’s designed to handle nested arrays effectively, making it the perfect solution for printing multi-dimensional arrays.
Here’s an example of how to use Arrays.deepToString()
:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[][] array = {{1, 2, 3}, {4, 5, 6}};
System.out.println(Arrays.deepToString(array));
}
}
# Output:
# [[1, 2, 3], [4, 5, 6]]
In this example, we’ve declared a two-dimensional integer array named ‘array’. Arrays.deepToString()
is used to convert the array into a string format, and System.out.println()
prints this string to the console. The output is the expected nested array structure, not memory addresses.
Arrays.deepToString() vs Arrays.toString()
The key difference between Arrays.deepToString()
and Arrays.toString()
lies in their handling of multi-dimensional arrays. Arrays.toString()
is sufficient for one-dimensional arrays but fails to correctly interpret nested arrays. On the other hand, Arrays.deepToString()
correctly prints all nested arrays, making it the preferred choice for multi-dimensional arrays.
While Arrays.deepToString()
can be a little slower due to its recursive nature, the difference is negligible unless you’re dealing with extremely large arrays. As a best practice, use Arrays.toString()
for one-dimensional arrays and Arrays.deepToString()
for multi-dimensional arrays.
Exploring Alternatives: Java 8’s Stream API and For-Each Loop
While Arrays.toString()
and Arrays.deepToString()
are the standard methods for printing arrays in Java, there are alternative approaches that offer more flexibility and control. Two of these are Java 8’s Stream API and the for-each loop.
Harnessing the Power of Java 8’s Stream API
Java 8 introduced the Stream API, a powerful tool that can be used to manipulate arrays and collections. The Stream API can be used to print an array in Java, like so:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
Arrays.stream(array).forEach(System.out::println);
}
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, Arrays.stream(array)
creates a stream of elements from the array. The forEach
method is then used with a method reference (System.out::println
) to print each element of the array on a new line.
Looping with the For-Each Loop
Another alternative is the for-each loop, which provides a simple, readable way to iterate over an array or collection.
Here’s an example of how to use a for-each loop to print an array in Java:
public class Main {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
for (int i : array) {
System.out.println(i);
}
}
}
# Output:
# 1
# 2
# 3
# 4
# 5
In this example, the for-each loop iterates over each element in the array, storing the current element in the variable ‘i’. The System.out.println(i)
statement is then used to print each element to the console.
Comparing the Alternatives
Method | Advantages | Disadvantages |
---|---|---|
Stream API | Concise and powerful, especially with large data sets | Overkill for simple tasks, can be slower with small data sets |
For-Each Loop | Simple and easy to read, good performance with small data sets | Less flexible than the Stream API, can be slower with large data sets |
In conclusion, the best method for printing an array in Java depends on the specific situation. For simple tasks with small arrays, the for-each loop is a good choice. For more complex tasks or large data sets, the Stream API provides more power and flexibility.
While printing an array in Java is a straightforward task, you may encounter some common issues. Let’s discuss these potential pitfalls and how to navigate them.
Dealing with ‘ArrayIndexOutOfBoundsException’
The ‘ArrayIndexOutOfBoundsException’ is a common error that occurs when you try to access an array element using an invalid index. This happens when the index is either negative or greater than or equal to the size of the array.
Here’s an example of how this error can occur:
public class Main {
public static void main(String[] args) {
int[] array = {1, 2, 3, 4, 5};
System.out.println(array[5]);
}
}
# Output:
# Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: Index 5 out of bounds for length 5
In this example, we’ve tried to access the element at index 5 of an array that only has five elements (with indices 0 to 4). This results in an ‘ArrayIndexOutOfBoundsException’. To avoid this error, always ensure that the index you’re using to access an array element is within the valid range.
Handling Null Values in Arrays
Another common issue when printing arrays in Java is dealing with null values. If your array contains null values, and you try to perform operations on these null values, a ‘NullPointerException’ could occur.
Consider this example:
public class Main {
public static void main(String[] args) {
String[] array = {"Hello", null, "World"};
for (String s : array) {
System.out.println(s.toUpperCase());
}
}
}
# Output:
# HELLO
# Exception in thread "main" java.lang.NullPointerException
In this example, we’ve tried to convert each string in the array to uppercase using the toUpperCase()
method. However, since one of the array elements is null, a ‘NullPointerException’ is thrown. To avoid this, you should always check if an array element is null before performing operations on it.
By being aware of these common issues and their solutions, you can avoid many of the pitfalls associated with printing arrays in Java.
Understanding Arrays in Java
In Java, an array is a container object that holds a fixed number of values of a single type. The length of an array is established when the array is created. Array elements are accessed using their integer index. The index of an array starts at 0 and ends at the array length minus one.
Here’s an example of declaring and initializing an array in Java:
int[] array = new int[5];
# The array now contains 5 elements, all initialized to 0
In this example, we’ve declared an integer array named ‘array’. The new
keyword is used to create the array, and int[5]
specifies that this array will hold five elements.
Diving Into the Arrays Class
The java.util.Arrays
class in Java provides various methods for manipulating arrays. It offers static methods for sorting and searching arrays, comparing arrays, and filling array elements. The Arrays.toString()
and Arrays.deepToString()
methods, which we’ve discussed earlier, are part of this class.
The Arrays
class is a member of the Java Collections Framework and inherits the java.lang.Object
class. It provides several utility methods that are used for common, often reoccurring, operations on arrays.
For example, to sort an array, you can use the Arrays.sort()
method:
import java.util.Arrays;
public class Main {
public static void main(String[] args) {
int[] array = {5, 3, 2, 4, 1};
Arrays.sort(array);
System.out.println(Arrays.toString(array));
}
}
# Output:
# [1, 2, 3, 4, 5]
In this example, we’ve used the Arrays.sort()
method to sort an integer array in ascending order. The Arrays.toString()
method is then used to print the sorted array.
Understanding the fundamentals of arrays and the Arrays
class in Java is crucial for effectively printing and manipulating arrays.
The Bigger Picture: Arrays in Data Analysis and Algorithms
Printing arrays in Java is not just about displaying values on the console. It’s a fundamental skill that has far-reaching implications in areas like data analysis and algorithms.
In data analysis, arrays often represent data sets. Printing an array can provide a quick snapshot of your data, helping you understand its structure and content. It can be the first step in exploratory data analysis, leading to insights that guide your data processing and modeling decisions.
In algorithms, arrays are a common data structure used to store and manipulate data. Printing arrays can be useful for debugging your algorithms, allowing you to track how your data changes at different stages of the algorithm.
Expanding Your Array Skills: Sorting and Searching
Once you’ve mastered printing arrays in Java, there are related concepts that you can explore to deepen your understanding and expand your skills. Two of these are array sorting and searching in arrays.
Array sorting is the process of arranging the elements in an array in a certain order (usually ascending or descending). Java provides built-in methods for array sorting, such as Arrays.sort()
, as well as more advanced sorting algorithms like quicksort and mergesort.
Searching in arrays involves finding a specific value or set of values in an array. Java provides methods for both linear search (searching each element in order) and binary search (a faster method that requires the array to be sorted).
Further Resources for Array Mastery in Java
To continue your journey towards mastering arrays in Java, here are some resources that provide more in-depth information and practical exercises:
- Java Arrays Mastery Simplified – Explore the role of arrays in sorting, searching, and filtering data in Java.
Add to Array in Java – Learn how to add elements to arrays dynamically in Java.
Sorting Arrays in Java – Learn about built-in sorting methods like Arrays.sort() for sorting arrays.
Oracle Java Arrays Documentation provides a thorough overview of declaring, initializing, and manipulating arrays arrays in Java.
Java Array Exercises offers a variety of practical exercises for working with arrays in Java.
Java Programming – Arrays tutorial provides a comprehensive guide to multi-dimensional arrays and the
Arrays
class.
Wrapping Up: Array Printing in Java
In this comprehensive guide, we’ve delved into the process of printing an array in Java. We’ve explored the various methods available, their usage, and the common issues that you might encounter, as well as their solutions.
We began with the basics, learning how to print an array using the Arrays.toString()
method. We then took a step further into handling multi-dimensional arrays with the Arrays.deepToString()
method.
Next, we ventured into alternative approaches, harnessing the power of Java 8’s Stream API and the for-each loop. We also navigated through common pitfalls you might encounter when printing arrays, such as ‘ArrayIndexOutOfBoundsException’ and handling null values, providing you with solutions and workarounds for each issue.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Arrays.toString() | Easy to use, ideal for one-dimensional arrays | Not suitable for multi-dimensional arrays |
Arrays.deepToString() | Handles multi-dimensional arrays effectively | Slightly slower due to its recursive nature |
Stream API | Powerful and flexible, especially with large data sets | May be overkill for simple tasks |
For-Each Loop | Simple and readable, good performance with small arrays | Less flexible than the Stream API |
Whether you’re just starting out with array printing in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the process and its intricacies.
With this knowledge, you’re now well equipped to handle array printing tasks in Java, whether you’re debugging your code, analyzing data, or implementing complex algorithms. Happy coding!