Java Array Sorting: Methods, Tips, and Examples
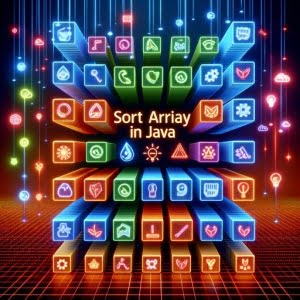
Ever felt like sorting an array in Java is a tricky task? You’re not alone. Many developers find Java array sorting a bit challenging. Think of Java’s array sorting as a puzzle – a puzzle that organizes your data in a specific order, making it easier to manage and understand.
Java provides powerful tools to sort arrays, making them extremely popular for organizing data in various applications.
This guide will walk you through the process of sorting arrays in Java, from the basics to more advanced techniques. We’ll cover everything from using the built-in Arrays.sort()
method, handling different types of arrays, to dealing with custom comparators and even troubleshooting common issues.
Let’s dive in and start mastering array sorting in Java!
TL;DR: How Do I Sort an Array in Java?
Java provides the
Arrays.sort()
method to sort an array. It’s as simple as calling this method with your array as the argument.
Here’s a simple example:
int[] arr = {3, 1, 4, 1, 5};
Arrays.sort(arr);
System.out.println(Arrays.toString(arr));
// Output:
// [1, 1, 3, 4, 5]
In this example, we’ve created an integer array arr
with some unsorted numbers. We then use the Arrays.sort()
method to sort the array. Finally, we print the sorted array, which outputs the numbers in ascending order.
This is just a basic way to sort an array in Java, but there’s much more to learn about sorting arrays, including handling different types of arrays, using custom comparators, and troubleshooting common issues. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Sorting Arrays in Java: The Basics
Java provides a built-in method, Arrays.sort()
, to sort an array. This method sorts the array’s elements into ascending order, applicable to different types of arrays such as integer, double, string, or custom objects. The Arrays.sort()
method uses a variation of the QuickSort algorithm, known for its efficiency in most common scenarios.
Let’s look at a simple code example:
int[] numArray = {20, 3, 5, 22, 10};
Arrays.sort(numArray);
System.out.println(Arrays.toString(numArray));
// Output:
// [3, 5, 10, 20, 22]
In this code, we have an integer array numArray
with unsorted numbers. We use the Arrays.sort()
method, which sorts the array in ascending order. We then print the sorted array, resulting in the numbers arranged in ascending order.
The Arrays.sort()
method is straightforward and efficient for sorting arrays. However, it’s important to note that it sorts the original array, meaning the array is sorted ‘in-place’. This can be a potential pitfall if you want to preserve the original array’s order.
Additionally, for arrays of custom objects, the Arrays.sort()
method requires the objects to implement the Comparable
interface. Without it, the method wouldn’t know how to sort the objects, resulting in a ClassCastException
.
Advanced Array Sorting in Java
Sorting with Custom Comparators
Java allows for custom sorting of arrays through the use of comparators. A comparator is a simple interface that you can implement in your class to define how objects of this class should be ordered.
String[] strArray = {"Java", "Python", "C++", "JavaScript"};
Comparator<String> lengthComparator = new Comparator<String>() {
@Override
public int compare(String str1, String str2) {
return Integer.compare(str1.length(), str2.length());
}
};
Arrays.sort(strArray, lengthComparator);
System.out.println(Arrays.toString(strArray));
// Output:
// [C++, Java, Python, JavaScript]
In this example, we created a comparator lengthComparator
that compares strings based on their lengths. We then sorted strArray
using this comparator, resulting in an array sorted by string length.
Sorting Objects
To sort an array of custom objects, the objects’ class must implement the Comparable
interface and override compareTo()
method. If you want a different sort order, you can create a Comparator
for that class.
Sorting Multi-Dimensional Arrays
Java can also sort multi-dimensional arrays. However, Arrays.sort()
only sorts the ‘first dimension’. If you want to sort by a ‘second dimension’, you’ll need to use a custom comparator.
int[][] multiArray = {{3, 5}, {1, 4}, {2, 6}};
Arrays.sort(multiArray, new Comparator<int[]>() {
@Override
public int compare(int[] entry1, int[] entry2) {
return Integer.compare(entry1[0], entry2[0]);
}
});
System.out.println(Arrays.deepToString(multiArray));
// Output:
// [[1, 4], [2, 6], [3, 5]]
In this example, we sorted multiArray
based on the first element of each sub-array. The Arrays.sort()
method, combined with a custom comparator, sorted the 2D array according to our needs.
Exploring Alternative Methods for Array Sorting in Java
Using Collections.sort()
for ArrayLists
While Arrays.sort()
is a powerful method for sorting arrays, it’s not applicable to ArrayLists. In such cases, Java provides Collections.sort()
method, which can efficiently sort an ArrayList.
ArrayList<Integer> arrayList = new ArrayList<>(Arrays.asList(3, 1, 4, 1, 5));
Collections.sort(arrayList);
System.out.println(arrayList);
// Output:
// [1, 1, 3, 4, 5]
In this example, we’ve created an ArrayList arrayList
and sorted it using Collections.sort()
. This method also sorts the ArrayList in-place, similar to Arrays.sort()
.
Implementing Your Own Sorting Algorithm
For advanced users seeking more control over the sorting process, implementing your own sorting algorithm is an option. This approach allows for custom sorting behaviors but requires a deep understanding of sorting algorithms and their complexities.
Here’s an example of implementing a simple Bubble Sort algorithm:
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n-1; i++) {
for (int j = 0; j < n-i-1; j++) {
if (arr[j] > arr[j+1]) {
// swap arr[j+1] and arr[j]
int temp = arr[j];
arr[j] = arr[j+1];
arr[j+1] = temp;
}
}
}
}
int[] arr = {64, 34, 25, 12, 22, 11, 90};
bubbleSort(arr);
System.out.println(Arrays.toString(arr));
// Output:
// [11, 12, 22, 25, 34, 64, 90]
In this example, we’ve implemented a Bubble Sort algorithm to sort an array. This method offers more control over the sorting process but can be more time-consuming and complex.
Each method has its advantages and disadvantages. Arrays.sort()
and Collections.sort()
are straightforward and efficient for most sorting tasks. Implementing your own sorting algorithm offers more control but requires a deep understanding of sorting algorithms. The choice depends on your specific needs and expertise.
Troubleshooting Java Array Sorting
Handling Null Values
One common issue when sorting arrays in Java is dealing with null values. If an array contains null values and you attempt to sort it using Arrays.sort()
, a NullPointerException
will be thrown.
A good practice is to check for null values before sorting. Here’s an example of how you can handle this:
String[] strArray = {"Java", null, "Python", "C++"};
Arrays.sort(strArray, Comparator.nullsLast(Comparator.naturalOrder()));
System.out.println(Arrays.toString(strArray));
// Output:
// [C++, Java, Python, null]
In this example, we used the Comparator.nullsLast()
method along with Arrays.sort()
to sort the array while placing null values at the end. This method prevents a NullPointerException
from being thrown.
Sorting Arrays of Custom Objects
Another common issue is sorting arrays of custom objects. If the objects’ class doesn’t implement the Comparable
interface, a ClassCastException
will be thrown when you try to sort the array.
To solve this issue, implement the Comparable
interface in your class and override the compareTo()
method. Alternatively, you can create a Comparator
for your class.
public class Person implements Comparable<Person> {
private String name;
// constructor, getters and setters
@Override
public int compareTo(Person person) {
return this.name.compareTo(person.getName());
}
}
Person[] persons = {new Person("John"), new Person("Adam"), new Person("Zoe")};
Arrays.sort(persons);
System.out.println(Arrays.toString(persons));
// Output:
// [Adam, John, Zoe]
In this example, we implemented Comparable
in the Person
class and sorted an array of Person
objects. The compareTo()
method compares Person
objects based on their name
attribute.
Understanding Sorting Algorithms in Java
QuickSort: The Backbone of Arrays.sort()
The Arrays.sort()
method in Java primarily uses a Dual-Pivot Quicksort algorithm for sorting primitives, like int
, char
, etc., which is a slightly modified version of the classic QuickSort algorithm.
QuickSort is a divide-and-conquer algorithm that divides the array into two smaller sub-arrays and then recursively sorts them. The two pivots in Dual-Pivot QuickSort speed up the sorting process by reducing the number of comparisons and swaps.
Here’s a simplified version of how QuickSort works:
// A simplified version of QuickSort for understanding
public static void quickSort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// This function takes last element as pivot, places the pivot element at its correct position in sorted array,
// and places all smaller (smaller than pivot) to left of pivot and all greater elements to right of pivot
public static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
// swap arr[i] and arr[j]
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
// swap arr[i+1] and arr[high] (or pivot)
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
int[] arr = {10, 7, 8, 9, 1, 5};
int n = arr.length;
quickSort(arr, 0, n - 1);
System.out.println(Arrays.toString(arr));
// Output:
// [1, 5, 7, 8, 9, 10]
This is a basic version of QuickSort. It’s not exactly how Java’s Arrays.sort()
works but it gives you a basic understanding of QuickSort’s logic.
Time Complexity and Space Complexity
Understanding time complexity and space complexity is crucial when dealing with sorting algorithms. Time complexity refers to the computational complexity that describes the amount of time an algorithm takes to run. Space complexity describes the amount of memory (space) an algorithm needs to run.
QuickSort has a worst-case time complexity of O(n^2), which occurs when the input array is already sorted or reverse sorted. However, in the average case, QuickSort performs well with a time complexity of O(n log n).
The space complexity of QuickSort is O(log n) because it needs space to store the recursive function calls. This makes QuickSort more space-efficient than other sorting algorithms like Bubble Sort or Selection Sort, which have a space complexity of O(1), but less time-efficient.
The Bigger Picture: Sorting Arrays in Real-World Projects
Sorting arrays in Java is not just a basic programming task but a crucial tool in larger projects. It plays a significant role in various domains like database management, data analysis, and machine learning.
Sorting in Database Management
In database management, sorting is often used to organize records based on certain fields, making data retrieval faster and more efficient. For instance, a database of customers can be sorted by names, allowing for quick search of customer information.
Sorting in Data Analysis
In data analysis, sorting can help identify patterns, trends, and outliers in a dataset. For example, sorting a dataset of sales records by the amount can instantly reveal the highest and lowest sales.
Exploring Related Concepts
Mastering array sorting is just the beginning. There’s a world of related concepts to explore, such as other types of data structures (lists, sets, maps, trees, etc.) and more complex algorithms. Understanding these concepts can help you write more efficient and effective code.
Further Resources for Mastering Java Array Sorting
To deepen your understanding of sorting arrays in Java, here are some valuable resources:
- Tips and Techniques for Using Arrays in Java – Discover how to implement dynamic resizing and dynamic arrays in Java.
How to Print Arrays in Java – Learn techniques for formatting and displaying array elements.
Converting Arrays to Strings in Java – Explore methods for customizing the string format of array elements.
Java Tutorials by Oracle cover all aspects of Java, including data structures and algorithms.
GeeksforGeeks’ Java Algorithms Section provides a wealth of information on various algorithms implemented in Java.
Baeldung Articles on Java offers many in-depth articles on Java, including array sorting and related topics.
These resources provide a wealth of information that can help you deepen your understanding of array sorting and related concepts in Java.
Wrapping Up: Sorting Arrays in Java
In this comprehensive guide, we’ve journeyed through the process of sorting arrays in Java, from the basics to more advanced techniques.
We started with the basics, learning how to use the built-in Arrays.sort()
method to sort different types of arrays. We then ventured into more advanced territory, exploring custom comparators, sorting objects, and handling multi-dimensional arrays.
Along the way, we tackled common challenges you might face when sorting arrays in Java, such as handling null values and sorting arrays of custom objects, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to sorting arrays in Java, such as using the Collections.sort()
method for ArrayLists and even implementing your own sorting algorithm. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Arrays.sort() | Fast, efficient, works with different types of arrays | Modifies original array, requires Comparable for custom objects |
Collections.sort() | Works with ArrayLists | Not applicable to arrays |
Implementing your own sorting algorithm | More control over sorting process | Requires deep understanding of sorting algorithms |
Whether you’re just starting out with sorting arrays in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of sorting arrays in Java and its capabilities.
Sorting arrays is a fundamental task in Java programming, and now you’re well-equipped to handle it. Happy coding!