Utilizing Java Code Testers: A Detailed Walkthrough
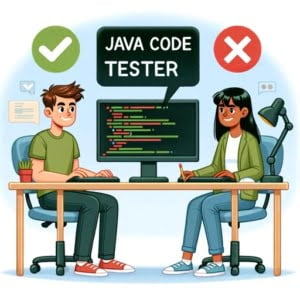
Are you grappling with testing your Java code? Like a meticulous proofreader, a Java code tester is an invaluable tool that can help you spot and fix errors in your code, ensuring your applications run smoothly and efficiently.
Java code testers are like the guardians of your code, diligently scanning through each line to ensure that everything is in order. They help you maintain the integrity of your code, making sure that your applications are robust and reliable.
This guide will take you through the top Java code testers and how to use them effectively. We’ll cover everything from the basics of setting up and using a Java code tester, to more advanced techniques and alternative approaches. So, let’s dive in and start mastering Java code testing!
TL;DR: How to use a Java Code Tester?
There are several excellent Java code testers available, but some of the most popular ones include
JUnit, Mockito, and Arquillian
. To start using a code tested you must import it with a statement such as:import org.junit.jupiter.api.*;
. Each code tester has its own strengths and weaknesses, so the best one for you will depend on your specific needs.
Here’s a simple example using JUnit:
import org.junit.jupiter.api.*;
public class SimpleTest {
@Test
public void testAddition() {
int a = 5;
int b = 10;
int expected = 15;
Assertions.assertEquals(expected, a + b);
}
}
// Output:
// Test successful
In this example, we’ve used JUnit, one of the most popular Java code testers. We’ve created a simple test case testAddition()
where we test if the addition of two numbers a
and b
equals the expected result. JUnit provides an assertEquals
method for this purpose. If the test passes, it means our addition operation works as expected.
This is just a basic way to use a Java code tester, but there’s much more to learn about creating and managing tests in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Java Code Testers
Java code testers are tools that help you ensure the correctness of your code. They allow you to write test cases that simulate different scenarios and check if your code behaves as expected. Let’s break down the basic steps of using a Java code tester.
Setting Up the Tester
The first step is to set up the tester. For our example, we’ll use JUnit, one of the most popular Java code testers. To add JUnit to your project, you’ll need to include it in your project’s dependencies. If you’re using a build tool like Maven, you can add the following to your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
This will download and add JUnit to your project, allowing you to use its features.
Writing Test Cases
Once you’ve set up JUnit, you can start writing test cases. A test case is a piece of code that checks if a certain part of your code works as expected. Here’s a simple test case that checks if the addition operation works correctly:
import org.junit.jupiter.api.*;
public class SimpleTest {
@Test
public void testAddition() {
int a = 5;
int b = 10;
int expected = 15;
Assertions.assertEquals(expected, a + b);
}
}
// Output:
// Test successful
In this test case, we’re checking if the sum of a
and b
equals expected
. If it does, the test passes. If it doesn’t, the test fails and JUnit will let us know.
Interpreting the Results
After running your tests, you’ll get a report detailing which tests passed and which failed. This will help you identify any issues in your code and fix them. Remember, the goal of testing is not to prove that your code works, but to find any places where it doesn’t.
Advanced Techniques in Java Code Testing
As you become more comfortable with basic testing, you can start exploring more advanced techniques. In this section, we’ll cover testing private methods, using mock objects, and testing database interactions.
Testing Private Methods
In Java, private methods are not directly accessible from outside the class they belong to. However, you can still test them using reflection. Here’s how you can do it:
import java.lang.reflect.Method;
import org.junit.jupiter.api.*;
public class PrivateMethodTest {
@Test
public void testPrivateMethod() throws Exception {
MyClass myClass = new MyClass();
Method method = MyClass.class.getDeclaredMethod("myPrivateMethod");
method.setAccessible(true);
int result = (Integer) method.invoke(myClass);
Assertions.assertEquals(42, result);
}
}
// Output:
// Test successful
In this code, we’re using the getDeclaredMethod
method to access the private method, and setAccessible(true)
to make it accessible. We then invoke the method and check if it returns the expected result.
Using Mock Objects
Mock objects are simulated objects that mimic the behavior of real objects in controlled ways. They are useful when the real objects are impractical to incorporate into the unit test.
In Java, we can use the Mockito library to create mock objects. Here’s an example:
import org.junit.jupiter.api.*;
import static org.mockito.Mockito.*;
public class MockTest {
@Test
public void testMock() {
// Create a mock object
List mockedList = mock(List.class);
// Use the mock object
mockedList.add("one");
mockedList.clear();
// Verify the behavior
verify(mockedList).add("one");
verify(mockedList).clear();
}
}
// Output:
// Test successful
In this code, we’re creating a mock List object, using it, and then verifying that it behaved as expected.
Testing Database Interactions
Testing database interactions is a crucial part of ensuring that your application works correctly. You can use a tool like JUnit and an in-memory database to test your database interactions. Here’s an example:
import org.junit.jupiter.api.*;
import java.sql.*;
public class DatabaseTest {
@Test
public void testDatabase() throws Exception {
Connection connection = DriverManager.getConnection("jdbc:h2:mem:");
Statement statement = connection.createStatement();
statement.execute("CREATE TABLE example (id INT, name VARCHAR)");
statement.execute("INSERT INTO example (id, name) VALUES (1, 'Test')");
ResultSet resultSet = statement.executeQuery("SELECT * FROM example");
resultSet.next();
Assertions.assertEquals(1, resultSet.getInt("id"));
Assertions.assertEquals("Test", resultSet.getString("name"));
}
}
// Output:
// Test successful
In this code, we’re creating an in-memory database, adding a table and a row to it, and then querying the database to check if the data is correct.
Exploring Alternative Testing Methods in Java
While using Java code testers like JUnit, Mockito, and Arquillian is common, there are other methods to test your Java code. These include manual testing, using different programming languages, or employing different testing methodologies like Test-Driven Development (TDD) or Behavior-Driven Development (BDD).
Manual Testing
Manual testing is the process of manually executing your code and checking if it behaves as expected. While it’s not as efficient as automated testing, it can be useful for exploratory testing or when automated testing is not feasible.
public class ManualTest {
public static void main(String[] args) {
int a = 5;
int b = 10;
int expected = 15;
System.out.println((a + b) == expected ? "Test successful" : "Test failed");
}
}
// Output:
// Test successful
In this example, we’re manually testing if the sum of a
and b
equals expected
. If it does, we print Test successful
. If it doesn’t, we print Test failed
.
Testing in Different Programming Languages
Sometimes, you might want to test your Java code using a different programming language. For example, you could use Python and a tool like PyUnit to test your Java code.
Test-Driven Development (TDD) and Behavior-Driven Development (BDD)
TDD and BDD are methodologies that guide the way you write and test your code. In TDD, you write your tests before your code, which helps ensure that your code is testable and that all important behaviors are tested. In BDD, you write your tests in a language that’s easy for non-developers to understand, which helps ensure that your code meets business requirements.
Here’s an example of a TDD approach using JUnit:
import org.junit.jupiter.api.*;
public class TddTest {
@Test
public void testAddition() {
// Write the test before the actual code
Calculator calculator = new Calculator();
Assertions.assertEquals(15, calculator.add(5, 10));
}
}
// Output:
// Test fails because the Calculator class and add method don't exist yet
In this example, we’re following the TDD approach of writing the test before the actual code. The test fails initially because the Calculator
class and add
method don’t exist yet. We would then write the Calculator
class and add
method to make the test pass.
Troubleshooting Java Code Testers
Like any tool, Java code testers can sometimes present challenges. Let’s explore some common issues you might encounter when using a Java code tester, along with solutions and workarounds.
Unexpected Test Case Failures
Sometimes, a test case might fail even though you expect it to pass. This could be due to a bug in your code, or it could be that your test case is not correctly set up. Here’s an example:
import org.junit.jupiter.api.*;
public class UnexpectedFailureTest {
@Test
public void testAddition() {
int a = 5;
int b = 10;
int expected = 16;
Assertions.assertEquals(expected, a + b);
}
}
// Output:
// Test failed: expected:<16> but was:<15>
In this example, the test case fails because the expected sum is incorrect. The solution is to correct the expected sum to match the actual sum of a
and b
.
Tests Taking Too Long
If your tests are taking too long to run, it could be that your code is inefficient, or that your test cases are too complex. Consider refactoring your code or breaking down your test cases into smaller, more manageable parts.
Issues with Setting Up the Testing Environment
Setting up the testing environment can sometimes be tricky. For example, you might encounter issues when trying to add JUnit to your project’s dependencies. If you’re using Maven, make sure your pom.xml
file is correctly set up:
<dependencies>
<dependency>
<groupId>org.junit.jupiter</groupId>
<artifactId>junit-jupiter-api</artifactId>
<version>5.7.0</version>
<scope>test</scope>
</dependency>
</dependencies>
If you’re still encountering issues, consider searching for solutions online or asking for help on a platform like Stack Overflow.
The Importance of Testing in Software Development
Software testing is a crucial aspect of any development process. It provides a safety net, ensuring that your code performs as expected and helping to prevent bugs from making their way into the final product. It’s like the quality control phase in manufacturing, catching errors before they reach the end user.
Different Types of Testing
There are several types of testing, each with its own purpose and use case. Let’s explore a few of them:
Unit Testing
Unit testing involves testing individual units of code (like functions or methods) in isolation. This helps ensure that each part of your code works correctly on its own.
import org.junit.jupiter.api.*;
public class UnitTest {
@Test
public void testAddition() {
int a = 5;
int b = 10;
int expected = 15;
Assertions.assertEquals(expected, a + b);
}
}
// Output:
// Test successful
In this example, we’re testing a simple addition operation. The unit test checks if the sum of a
and b
equals expected
. If it does, the test passes. If it doesn’t, the test fails.
Integration Testing
Integration testing involves testing how different parts of your code work together. This helps ensure that your code modules interact properly.
import org.junit.jupiter.api.*;
public class IntegrationTest {
@Test
public void testIntegration() {
Calculator calculator = new Calculator();
int result = calculator.add(5, 10);
Assertions.assertEquals(15, result);
}
}
// Output:
// Test successful
In this example, we’re testing the add
method of a Calculator
object. The integration test checks if the method works correctly when called with certain arguments.
Java Code Testers: How They Work
Java code testers, like JUnit or Mockito, provide a framework for writing and running your tests. They provide annotations to define test methods (like @Test
), assertions to check results (like assertEquals
), and runners to execute tests and report results.
import org.junit.jupiter.api.*;
public class JunitTest {
@Test
public void testAddition() {
int a = 5;
int b = 10;
int expected = 15;
Assertions.assertEquals(expected, a + b);
}
}
// Output:
// Test successful
In this example, we’re using JUnit to write a unit test. The @Test
annotation tells JUnit that testAddition
is a test method, and the assertEquals
method checks if the sum of a
and b
equals expected
.
The Bigger Picture: Java Code Testing and Software Development
Java code testing is not an isolated process. It fits into a larger context of software development and testing methodologies. Understanding this context can help you make the most of your Java code testing efforts.
Java Code Testing and Continuous Integration
Continuous Integration (CI) is a development practice where developers integrate code into a shared repository frequently, preferably several times a day. Each integration is then verified by an automated build and automated tests.
Java code testers play a crucial role in this process. They can be integrated into your CI pipeline to automatically test your code whenever you make changes. This helps catch issues early and ensures that your code is always in a releasable state.
# Example of a CI pipeline script that runs JUnit tests
mvn clean install
mvn test
In this example, we’re using Maven (a build automation tool) to clean, build, and test a Java project. The mvn test
command runs all JUnit tests in the project.
Test-Driven Development and Agile Methodologies
Test-Driven Development (TDD) is a software development methodology where you write tests before you write the actual code. Agile methodologies, on the other hand, emphasize adaptability and collaboration in the development process.
Java code testers are essential tools in both TDD and Agile methodologies. They provide a quick and reliable way to test your code, allowing you to iterate rapidly and adapt to changes.
// Example of a TDD approach using JUnit
@Test
public void testAddition() {
// Write the test before the actual code
Calculator calculator = new Calculator();
Assertions.assertEquals(15, calculator.add(5, 10));
}
// Output:
// Test fails because the Calculator class and add method don't exist yet
In this example, we’re following the TDD approach of writing the test before the actual code. The test fails initially because the Calculator
class and add
method don’t exist yet. We would then write the Calculator
class and add
method to make the test pass.
Further Resources for Java Code Testing
If you’re interested in diving deeper into Java code testing, here are some resources that you might find helpful:
- Exploring Java Syntax – Learn essential syntax rules and conventions for writing Java code efficiently and effectively.
Introduction to JUnit Testing – Learn how to create test cases, perform assertions, and organize test suites using JUnit.
JUnit 5 User Guide is the official user guide for JUnit 5, one of the most popular Java code testers.
Mockito Documentation – the official documentation for Mockito, a popular library for creating mock objects in Java.
Java Code Testing with Spring Boot provides a detailed walkthrough of how to test a web application built with Spring Boot.
Wrapping Up: Java Code Testers
In this comprehensive guide, we’ve delved into the world of Java code testing. We’ve explored how to use popular Java code testers like JUnit, Mockito, and Arquillian to ensure the robustness and reliability of your Java applications.
We began with the basics, learning how to set up and use these testers, with a simple JUnit example demonstrating testing the addition of two numbers. We then ventured into more advanced territory, exploring complex testing techniques like testing private methods, using mock objects, and testing database interactions. We also highlighted alternative methods of testing Java code, from manual testing to using different programming languages and testing methodologies like TDD and BDD.
Along the way, we tackled common challenges you might encounter when using Java code testers, such as unexpected test case failures, tests taking too long to run, and issues with setting up the testing environment. We provided solutions and workarounds for each of these issues, equipping you with the tools to overcome these obstacles.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
JUnit | Robust, supports many libraries | May require troubleshooting for some programs |
Mockito | Simulates objects, useful for unit testing | Requires understanding of mock objects |
Arquillian | Powerful, can test in real environment | More complex than other testers |
Whether you’re just starting out with Java code testing or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Java code testing and its importance in maintaining the integrity of your code.
With its balance of robustness, flexibility, and depth, Java code testing is a powerful tool for any Java developer. Happy coding!