JUnit Testing in Java: Your Ultimate Guide
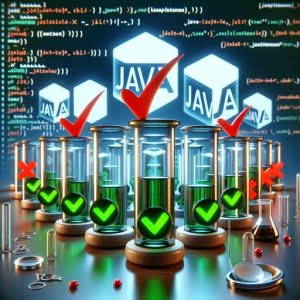
Are you finding JUnit testing in Java a bit of a struggle? You’re not alone. Many developers find themselves in a maze when it comes to JUnit testing. But consider JUnit as your detective, helping you uncover the hidden bugs in your code, ensuring your software’s robustness and reliability.
JUnit is a powerful tool in the Java ecosystem, providing a systematic way to test your code, making it a must-have skill for every Java developer.
In this guide, we’ll walk you through the process of JUnit testing in Java, from the basics to more advanced techniques. We’ll cover everything from creating your first test case, writing tests, running them, to understanding and implementing more complex aspects of JUnit testing. We’ll also introduce you to alternative testing frameworks and discuss common issues and their solutions.
So, let’s dive in and start mastering JUnit testing in Java!
TL;DR: How Do I Perform JUnit Testing in Java?
JUnit testing
in Java involves creating a separate .java file (the test case), where you write methods (the tests) that assert certain conditions in your code. You will need to import the necessary JUnit classes with,import org.junit.jupiter.api.*;
. You can then code test classes with simple lines such as
import static org.junit.jupiter.api.Assertions.assertEquals;assertEquals(2, 1 + 1);
. You can think of these tests as a series of checks to ensure your code behaves as expected.
Here’s a simple example:
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
class MyFirstJUnitTest {
@Test
void addition() {
assertEquals(2, 1 + 1);
}
}
In this example, we import the necessary JUnit classes and create a test case MyFirstJUnitTest
. Inside this class, we define a test method addition()
that checks if the sum of 1 and 1 equals 2 using the assertEquals()
method. If the condition holds true, the test passes; otherwise, it fails.
This is a basic way to perform JUnit testing in Java, but there’s much more to learn about creating and managing tests effectively. Continue reading for a more detailed guide on JUnit testing in Java.
Table of Contents
- Crafting Your First JUnit Test Case
- Advantages and Disadvantages of JUnit Testing
- Diving Deeper: Advanced JUnit Testing
- Exploring Alternatives: TestNG and Mockito
- Solving Common JUnit Testing Challenges
- Understanding the Principles of Unit Testing
- The Role of JUnit in Unit Testing
- The Importance of Unit Testing in Software Development
- JUnit Testing in the Broader Software Testing Context
- Wrapping Up: JUnit Testing in Java
Crafting Your First JUnit Test Case
Creating a JUnit test case is the first step in your JUnit testing journey. This is a separate .java file where you’ll write methods that test specific functionalities in your code.
Let’s create a simple JUnit test case:
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
class BasicJUnitTest {
@Test
void checkProduct() {
assertEquals(4, 2 * 2);
}
}
In this example, we’re testing a simple multiplication operation. The checkProduct()
method is annotated with @Test
, which signals that it’s a test method. Inside this method, we use assertEquals()
to check if the produc of 2 and 2 equals 4.
To run this test, you can use an IDE like Eclipse or IntelliJ, or a build tool like Maven or Gradle. If the test passes, it means the condition in assertEquals()
holds true.
Advantages and Disadvantages of JUnit Testing
Like any tool, JUnit testing has its pros and cons.
Benefits of JUnit Testing
- Identify Bugs Early: JUnit tests help you catch bugs and issues early in the development cycle, making them easier and cheaper to fix.
- Improve Code Quality: Writing tests forces you to think about your code’s design and quality. This often leads to better, more maintainable code.
- Facilitate Changes and Simplify Integration: With a good set of tests, you can make changes to your code with confidence. The tests will tell you if your changes have broken anything.
Drawbacks of JUnit Testing
- Time-Consuming: Writing tests can be time-consuming. It’s an investment that pays off in the long run, but it can slow down initial development.
- Learning Curve: JUnit has a learning curve, especially when it comes to more advanced features. However, once you master it, you’ll find it an invaluable tool.
- Doesn’t Catch Every Bug: While JUnit tests are great for catching many types of bugs, they’re not a silver bullet. Other types of testing (like integration testing and system testing) are also necessary.
Diving Deeper: Advanced JUnit Testing
As you become more comfortable with JUnit testing, you’ll want to explore its more advanced features. These include testing exceptions, using setup and teardown methods, and grouping tests into test suites.
Testing Exceptions
JUnit allows you to test if your code throws the expected exceptions. Here’s an example:
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertThrows;
class ExceptionTest {
@Test
void exceptionTesting() {
Exception exception = assertThrows(ArithmeticException.class, () -> {
int result = 10 / 0;
});
assertEquals("/ by zero", exception.getMessage());
}
}
In this example, we’re testing if dividing by zero throws an ArithmeticException
. The assertThrows()
method checks if the execution of the lambda expression (10 / 0)
throws an exception of type ArithmeticException
. If it does, the test passes.
Setup and Teardown Methods
Setup and teardown methods are used to prepare and clean up the test environment before and after each test. JUnit provides @BeforeEach
and @AfterEach
annotations for this purpose.
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
class SetupTeardownTest {
private int sum;
@BeforeEach
void setUp() {
sum = 0;
}
@Test
void addition() {
sum = 1 + 1;
assertEquals(2, sum);
}
@AfterEach
void tearDown() {
sum = 0;
}
}
In this example, the setUp()
method is run before each test, and the tearDown()
method is run after each test.
Grouping Tests into Test Suites
JUnit allows you to group related tests into test suites. This can make your tests easier to manage and run.
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
class MathTestSuite {
@Nested
class AdditionTests {
@Test
void addition() {
assertEquals(2, 1 + 1);
}
}
@Nested
class SubtractionTests {
@Test
void subtraction() {
assertEquals(0, 1 - 1);
}
}
}
In this example, we have a test suite MathTestSuite
that contains two nested classes AdditionTests
and SubtractionTests
. Each nested class contains related tests.
Exploring Alternatives: TestNG and Mockito
While JUnit is a powerful tool for unit testing in Java, it’s not the only one. Other testing frameworks like TestNG and Mockito also offer robust testing capabilities. Let’s take a look at these alternatives and see how they compare with JUnit.
TestNG: A Powerful Testing Framework
TestNG (Test Next Generation) is a testing framework inspired by JUnit but introduces some new functionalities that make it more powerful and easier to use. It supports parallel test execution, flexible test configuration, and powerful test scenarios.
Here’s a simple TestNG test case:
import org.testng.Assert;
import org.testng.annotations.Test;
public class TestNGExample {
@Test
public void addition() {
int sum = 1 + 1;
Assert.assertEquals(sum, 2);
}
}
// Output:
// PASSED: addition
In this example, we’re testing an addition operation, similar to our JUnit example. The syntax is slightly different, but the concept is the same.
Mockito: A Mocking Framework
Mockito is a mocking framework that you can use to write unit tests for Java applications. While JUnit tests can cover the unit testing, Mockito can be used in conjunction with JUnit to mock the behavior of complex dependencies and speed up the testing process.
Here’s a simple Mockito test case:
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.mockito.Mockito.*;
public class MockitoExample {
@Test
public void testQuery() {
// Create a mock instance of a class
MyClass test = mock(MyClass.class);
// Define the return value for method getUniqueId()
when(test.getUniqueId()).thenReturn(43);
// Use mock in test....
assertEquals(test.getUniqueId(), 43);
}
}
// Output:
// PASSED: testQuery
In this example, we’re creating a mock instance of MyClass
and defining that getUniqueId()
should return 43
. This allows us to test methods that depend on MyClass
without actually having to use a real MyClass
instance.
JUnit vs. TestNG vs. Mockito: Making the Choice
Choosing between JUnit, TestNG, and Mockito depends on your specific needs:
- If you’re looking for a simple, widely-used unit testing framework, JUnit is a great choice.
- If you need more advanced features like parallel test execution and flexible test configuration, TestNG might be the better option.
- If you’re dealing with complex dependencies that you want to mock in your tests, Mockito is a powerful tool to have in your arsenal.
Remember, these frameworks aren’t mutually exclusive. Many developers use JUnit and Mockito together to get the best of both worlds.
Solving Common JUnit Testing Challenges
While JUnit testing is a powerful tool, it’s not without its challenges. Here, we’ll discuss common errors or obstacles you might encounter during JUnit testing and provide solutions. We’ll also share some best practices and optimization tips.
Common Errors and Solutions
Error: Test Didn’t Run
Sometimes, you might find that your test didn’t run at all. This could be due to a variety of reasons, such as the test method being private
or not being annotated with @Test
.
class TestDidntRun {
private void testMethod() {
// This method won't run as a test because it's private
}
}
To solve this, ensure that your test methods are public and correctly annotated with @Test
.
class TestDidntRun {
@Test
public void testMethod() {
// This method will run as a test
}
}
Error: Test Failed Unexpectedly
A test might fail unexpectedly if the condition in the assertion method doesn’t hold true. For example, assertEquals(2, 1 + 1)
will fail if the sum of 1 and 1 doesn’t equal 2.
@Test
void addition() {
assertEquals(2, 1 + 2); // This test will fail
}
In this case, check your assertion conditions and ensure they’re correct.
Best Practices and Optimization Tips
- Write Small, Focused Tests: Each test should focus on one specific functionality. This makes your tests easier to understand and maintain.
- Use Descriptive Test Method Names: Your test method names should describe what the test does. This makes your tests easier to read and understand.
- Don’t Ignore Failing Tests: If a test is failing, don’t ignore it. Investigate the cause of the failure and fix it. Ignoring failing tests can lead to more serious issues down the line.
- Keep Tests Independent: Each test should be independent and not rely on the output of other tests. This makes your tests more reliable and easier to manage.
Understanding the Principles of Unit Testing
Unit testing is a software testing method where individual units of source code—sets of one or more computer program modules together with associated control data, usage procedures, and operating procedures—are tested to determine if they are fit for use. It’s a fundamental practice in software development, allowing developers to ensure that individual parts of their program are working correctly.
@Test
public void whenAdding1and1_thenResult2() {
assertEquals(2, 1 + 1);
// Output: Test passes as the sum of 1 and 1 equals 2
}
In this simple unit test, we’re testing the functionality of the addition operation. If the sum of 1 and 1 equals 2, then the test passes, indicating that the addition operation is working as expected.
The Role of JUnit in Unit Testing
JUnit plays a critical role in unit testing in Java. It provides annotations to identify test methods and assertions to test the expected results. It also provides test runners to run tests. JUnit tests can be run automatically and they check their own results and provide immediate feedback. There’s no need to manually comb through a report of test results.
import org.junit.jupiter.api.*;
import static org.junit.jupiter.api.Assertions.assertEquals;
class JUnitTest {
@Test
void addition() {
assertEquals(2, 1 + 1);
// Output: Test passes as the sum of 1 and 1 equals 2
}
}
In this JUnit test, we’re using the @Test
annotation to identify the addition()
method as a test method. We’re also using the assertEquals()
assertion to check if the sum of 1 and 1 equals 2. The test runner will run this test and provide immediate feedback.
The Importance of Unit Testing in Software Development
Unit testing is crucial in software development for several reasons. It helps developers to identify and fix bugs early before the software goes into production, ensures that the code works as expected, and makes it easier to refactor code. Unit testing also helps to speed up the development process, as it provides quick feedback whenever a piece of code is changed. Overall, unit testing leads to higher quality code and more reliable software.
JUnit Testing in the Broader Software Testing Context
JUnit testing is a critical part of the software testing ecosystem. It allows developers to test individual units of code and ensure they’re working as expected. But it’s not the only type of testing you should be doing. In fact, JUnit testing is just one piece of a much larger testing puzzle.
Integration Testing: Checking the Bigger Picture
While unit testing focuses on individual units of code, integration testing looks at how these units work together. It’s about checking the bigger picture.
// A simple example of integration testing
// Class A
public class A {
public int methodA(){
return 1;
}
}
// Class B
public class B {
A a;
public B(A a){
this.a = a;
}
public int methodB(){
return a.methodA() + 1;
}
}
// Integration Test
@Test
public void whenUsingMethodBinB_thenResult2() {
A a = new A();
B b = new B(a);
assertEquals(2, b.methodB());
// Output: Test passes as the result of methodB() in class B equals 2
}
In this example, we have two classes, A
and B
. Class B
depends on class A
. In the integration test, we’re testing the methodB()
in class B
to see if it correctly uses methodA()
from class A
.
System Testing: Evaluating the Entire System
System testing is a level of testing where a complete and integrated software is tested. It’s about evaluating the system as a whole and making sure all the pieces work together in harmony.
Acceptance Testing: Verifying the System Meets Requirements
Acceptance testing is the final phase of testing, where you verify if the system meets the business requirements and is ready to be delivered.
Further Resources for JUnit Testing
To further your understanding of JUnit testing and its role in the broader software testing ecosystem, check out these resources:
- Exploring Java Code Testing Methods – Explore the JUnit framework for Java code testing and test automation.
Mockito Overview – Discover Mockito, a framework for Java that simplifies the creation of mock objects in unit tests.
Java Reflection Basics – Dive into Java reflection API for inspecting and manipulating class metadata at runtime.
JUnit 5 User Guide is a comprehensive resource for all things JUnit.
Java Unit Testing with JUnit 5 course from Udemy dives deep into JUnit testing.
JUnit Test Framework Tutorial by TutorialsPoint provides a comprehensive guide on using JUnit.
Wrapping Up: JUnit Testing in Java
In this comprehensive guide, we’ve explored the ins and outs of JUnit testing in Java, a crucial tool for ensuring the reliability and robustness of your software.
We began with the basics of creating a JUnit test case, writing tests, and running them. We then delved into more advanced aspects of JUnit testing, such as testing exceptions, using setup and teardown methods, and grouping tests into test suites. We also took a look at alternative testing frameworks like TestNG and Mockito, and discussed how they compare with JUnit.
Along the way, we tackled common challenges you might encounter when using JUnit, such as tests not running or failing unexpectedly, and provided solutions to help you overcome these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
JUnit | Widely used, robust | Has a learning curve |
TestNG | Advanced features, flexible configuration | Less beginner-friendly |
Mockito | Great for mocking complex dependencies | Requires understanding of mocking |
Whether you’re just starting out with JUnit testing or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of JUnit testing in Java and its role in software development.
With its balance of simplicity and power, JUnit is an invaluable tool for any Java developer. Happy testing!