Mockito for Java | Unit Testing with Mock Objects
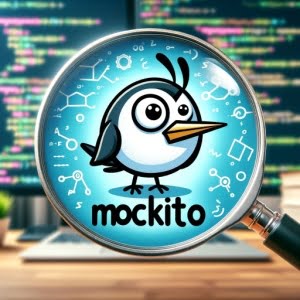
Are you finding it challenging to perform unit testing in Java? You’re not alone. Many developers find themselves grappling with this task, but there’s a tool that can make this process a breeze.
Like a skilled actor, Mockito can play any role in your code, making it easier to isolate and test individual components. These mocks can run on any system, even those without Java installed.
This guide will walk you through the basics of Mockito, from setting up your first mock to advanced techniques. We’ll explore Mockito’s core functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Mockito!
TL;DR: How Do I Use Mockito for Unit Testing in Java?
Mockito
is a powerful tool that allows you to create and configure mock objects for unit testing in Java. It can be imported with,import static org.mockito.Mockito.*;
and instantiated withList mockedList = mock(List.class);
. It simplifies the process of setting up and verifying mocks in your tests.
Here’s a simple example:
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
mockedList.add("one");
verify(mockedList).add("one");
# Output:
# No output. The test passes silently.
In this example, we import the necessary Mockito classes, create a mock List object, perform an operation (adding an element), and then verify that the operation was called on the mock object. If the operation wasn’t called, Mockito would throw an error, causing the test to fail.
This is just a basic way to use Mockito for unit testing in Java, but there’s much more to learn about creating and manipulating mocks, stubbing methods, and verifying behavior. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Creating and Using Mocks with Mockito
- Advanced Mockito: Argument Matchers, Stubbing Methods, and Spies
- Exploring Alternatives to Mockito: JMock and EasyMock
- Troubleshooting Mockito: Common Issues and Solutions
- The Importance of Unit Testing and Mocking
- Mockito: A Pillar in the Java Testing Landscape
- Mockito in Larger Projects and Different Types of Testing
- Wrapping Up: Mockito
Creating and Using Mocks with Mockito
Mockito is a powerful library that simplifies the process of creating and using mock objects in your Java unit tests. Here’s a step-by-step guide on how to create mocks with Mockito and use them in your tests.
Step 1: Creating Mocks
The first step in using Mockito is to create a mock object. Here’s an example of how to do this:
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
In this example, we’re creating a mock object of the List
class. The mock()
method creates a mock object of the specified class.
Step 2: Using Mocks in Tests
Once you’ve created a mock object, you can use it in your tests. Here’s an example:
mockedList.add("one");
This line of code adds an element to the mock list. Because it’s a mock, it won’t actually add an element to a list, but it will behave as if it did.
Step 3: Verifying Behavior
After you’ve used a mock object in a test, you can verify its behavior using Mockito’s verify()
method. Here’s an example:
verify(mockedList).add("one");
# Output:
# No output. The test passes silently.
This line of code verifies that the add()
method was called on the mockedList
object with the argument “one”. If the method wasn’t called with this argument, Mockito would throw an error, causing the test to fail.
Advantages and Pitfalls
Using Mockito for your Java unit tests has several advantages. It simplifies the process of setting up and verifying mocks, making your tests cleaner and easier to understand. It also allows you to isolate the behavior of the class or method you’re testing, making your tests more reliable.
However, there are potential pitfalls to be aware of when using Mockito. For example, if you’re not careful, you might end up overusing mocks, which can make your tests harder to understand and maintain. Additionally, Mockito can’t mock final classes or methods, which can be a limitation in some cases.
Advanced Mockito: Argument Matchers, Stubbing Methods, and Spies
As you become more comfortable with Mockito, you can start exploring its more advanced features. These include argument matchers, stubbing methods, and spying on real objects.
Argument Matchers
Argument matchers allow you to flexibly specify arguments when setting up your mocks. Here’s an example:
import static org.mockito.ArgumentMatchers.*;
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
when(mockedList.get(anyInt())).thenReturn("element");
System.out.println(mockedList.get(999));
# Output:
# "element"
In this example, we’re using the anyInt()
argument matcher to specify that the get()
method should return “element” for any integer argument. When we call mockedList.get(999)
, it returns “element”.
Stubbing Methods
Stubbing methods allow you to specify the behavior of your mock objects. Here’s an example:
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
when(mockedList.size()).thenReturn(100);
System.out.println(mockedList.size());
# Output:
# 100
In this example, we’re stubbing the size()
method to return 100. When we call mockedList.size()
, it returns 100.
Spying on Real Objects
Sometimes, you may want to use a real object but still be able to spy on its behavior. Mockito allows you to do this with spies. Here’s an example:
import static org.mockito.Mockito.*;
List list = new LinkedList();
List spy = spy(list);
spy.add("one");
spy.add("two");
System.out.println(spy.get(0));
# Output:
# "one"
In this example, we’re creating a spy on a real LinkedList
object. We can then interact with the spy just like we would with a real object, but we can also verify its behavior and stub its methods.
Exploring these advanced features of Mockito can help you write more flexible and powerful tests. However, remember that with great power comes great responsibility. Use these features judiciously to keep your tests clean and maintainable.
Exploring Alternatives to Mockito: JMock and EasyMock
While Mockito is a powerful tool for unit testing in Java, it’s not the only game in town. Other mocking frameworks, such as JMock and EasyMock, also offer robust features for creating and managing mock objects.
JMock: A Dynamic Mocking Framework
JMock is a library that supports flexible, automatic mocking of Java classes and interfaces. Here’s a simple example of how to use JMock:
import org.jmock.Expectations;
import org.jmock.Mockery;
import org.junit.Test;
public class JMockExample {
Mockery context = new Mockery();
List mockedList = context.mock(List.class);
@Test
public void test() {
context.checking(new Expectations() {{
oneOf(mockedList).add("one");
}});
mockedList.add("one");
}
}
# Output:
# No output. The test passes silently.
In this example, we’re creating a mock List object and setting an expectation that the add()
method will be called with the argument “one”. If this expectation is not met, the test will fail.
EasyMock: A High-Fidelity Mocking Framework
EasyMock is another popular choice for mocking in Java. Here’s an example of how to use EasyMock:
import org.easymock.EasyMock;
import org.junit.Test;
public class EasyMockExample {
List mockedList = EasyMock.createMock(List.class);
@Test
public void test() {
mockedList.add("one");
EasyMock.replay(mockedList);
mockedList.add("one");
EasyMock.verify(mockedList);
}
}
# Output:
# No output. The test passes silently.
In this example, we’re creating a mock List object, recording an expected behavior, and then replaying and verifying the behavior.
Comparison of Mockito, JMock, and EasyMock
Feature | Mockito | JMock | EasyMock |
---|---|---|---|
Ease of use | High | Medium | Medium |
Flexibility | High | High | Medium |
Support for Java 8+ | Yes | No | Yes |
Ability to mock final classes/methods | Yes (with limitations) | No | Yes |
While all three frameworks are powerful and flexible, Mockito’s ease of use and support for Java 8+ make it a popular choice. However, JMock and EasyMock can be useful alternatives in certain scenarios.
Troubleshooting Mockito: Common Issues and Solutions
While Mockito is a powerful tool that simplifies unit testing in Java, you may encounter some issues when using it. Let’s discuss some of these common problems and their solutions.
Unexpected Behavior
Sometimes, Mockito might not behave as you expect. This is often due to incorrect setup or misuse of the framework. For instance, let’s say you’ve set up a mock to throw an exception, but it’s not doing so:
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
when(mockedList.get(0)).thenThrow(new RuntimeException());
try {
mockedList.get(0);
} catch(RuntimeException e) {
System.out.println("Exception caught");
}
# Output:
# Exception caught
If the exception isn’t caught, you might be calling the method on the real object instead of the mock. Always ensure you’re interacting with the mock object in your tests.
Problems with Complex Mocks
Creating complex mocks with many stubbed methods or deep stubs can lead to tests that are hard to understand and maintain. To avoid this, try to keep your mocks simple and focused. Use the principle of ‘Don’t mock what you don’t own’ to decide what to mock.
Mockito’s Limitations
While Mockito is powerful, it has some limitations. For example, it can’t mock equals() or hashCode(), and it has limited support for mocking final classes or methods. If you need to mock these, consider using other tools like PowerMock.
Remember, the key to successful unit testing with Mockito is understanding how it works and using it effectively. Keep practicing, and don’t hesitate to consult the Mockito documentation or community if you encounter issues.
The Importance of Unit Testing and Mocking
Unit testing is a crucial part of software development. It involves testing individual units of source code to determine whether they’re fit for use. This helps ensure that each part of your program works correctly, leading to higher overall code quality.
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
public class CalculatorTest {
@Test
public void testAdd() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 2);
assertEquals(4, result);
}
}
# Output:
# Test passes silently
In this simple example, we’re testing a Calculator
class with a single add
method. The test checks whether the add
method correctly adds two numbers together. If the method works as expected, the test will pass; if not, it will fail, alerting us to a problem with our code.
However, unit testing becomes more complex when your code has external dependencies. That’s where mocking comes in.
Mockito: A Pillar in the Java Testing Landscape
Mocking is a technique used in unit testing to simulate the behavior of real objects. Mockito is a popular mocking framework in Java. It allows you to create and configure mock objects that mimic the behavior of complex, real objects, making it easier to isolate and test individual units of code.
import static org.mockito.Mockito.*;
List mockedList = mock(List.class);
when(mockedList.size()).thenReturn(100);
int size = mockedList.size();
System.out.println(size);
# Output:
# 100
In this example, we’re creating a mock List
object and stubbing its size
method to return 100
. When we call mockedList.size()
, it returns 100
, even though the list is actually empty. This allows us to test code that interacts with the list without having to set up a real list.
By providing a way to isolate code for testing, Mockito fits into the broader landscape of Java testing as a vital tool for creating robust, reliable tests.
Mockito in Larger Projects and Different Types of Testing
While Mockito is a powerful tool for unit testing, its usefulness extends to larger projects and different types of testing, such as integration testing and system testing.
Mockito in Integration Testing
Integration testing is a level of software testing where individual units are combined and tested as a group. Mockito can be used to mock the behavior of individual units to ensure the integration between units is working correctly.
import static org.mockito.Mockito.*;
Database mockDatabase = mock(Database.class);
when(mockDatabase.isConnected()).thenReturn(true);
Application app = new Application(mockDatabase);
System.out.println(app.canConnectToDatabase());
# Output:
# true
In this example, we’re creating a mock Database
object and using it to test an Application
object. The Application
object depends on the Database
object, but by using a mock, we can isolate the behavior of the Application
object and test it independently.
Mockito in System Testing
System testing is a level of software testing where a complete and integrated software is tested. Mockito can be used to mock external systems and services, allowing you to test your system as a whole without depending on external factors.
Exploring Test-Driven and Behavior-Driven Development
As you become more comfortable with Mockito and unit testing in general, you may want to explore related concepts like test-driven development (TDD) and behavior-driven development (BDD). These methodologies can further improve your testing practices and lead to more reliable, maintainable code.
Further Resources for Mockito Mastery
If you’re interested in learning more about Mockito and its applications, here are some resources that might help:
- IOFlood’s Java Code Testing Guide explains concepts in Java testing, like test fixtures and setup/teardown methods.
Using Mockito Spy – Learn how to use Mockito spy to mock specific methods of a real object.
JUnit Testing Overview – Dive into JUnit testing framework for writing and executing tests in Java.
Mockito’s Official Documentation is a comprehensive guide to Mockito’s features and usage.
Baeldung’s Guide to Mockito is a series of detailed tutorials on various aspects of Mockito.
Tutorialspoint’s Mockito Tutorial covers the basics and advanced features of Mockito.
Wrapping Up: Mockito
In this comprehensive guide, we’ve taken a deep dive into the world of Mockito, a robust framework for unit testing in Java.
We started with the basics, learning how to create, use, and verify mocks with Mockito. We then explored more advanced features, such as argument matchers, stubbing methods, and spying on real objects. Along the way, we tackled common issues you might encounter when using Mockito, providing you with solutions and workarounds for each problem.
We also looked at alternative approaches to mocking in Java, comparing Mockito with other frameworks like JMock and EasyMock. Here’s a quick comparison of these libraries:
Library | Ease of Use | Flexibility | Support for Java 8+ |
---|---|---|---|
Mockito | High | High | Yes |
JMock | Medium | High | No |
EasyMock | Medium | Medium | Yes |
Whether you’re just starting out with Mockito or looking to level up your unit testing skills, we hope this guide has given you a deeper understanding of Mockito and its capabilities.
With its balance of ease of use, flexibility, and support for modern Java versions, Mockito is a powerful tool for unit testing in Java. Now, you’re well equipped to enjoy those benefits. Happy testing!