Using Java try-with-resources: A Try Statements Guide
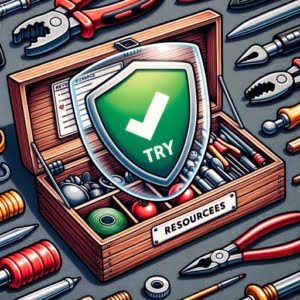
Are you finding it challenging to manage resources in Java? You’re not alone. Many developers grapple with this task, but there’s a feature that can make this process a breeze.
Like a responsible librarian, Java’s try-with-resources statement ensures every borrowed resource is returned properly. It’s a powerful tool that can help you manage resources effectively and avoid common pitfalls associated with resource leaks.
This guide will walk you through this powerful feature, from basic use to advanced techniques. So, let’s dive in and start mastering Java’s try-with-resources!
TL;DR: How Do I Use the Try-with-Resources in Java?
The try-with-resources statement in Java is a feature that automatically closes resources used within the try block, used with the syntax
try (FileReader fr = new FileReader("file.txt")){}
. This is a powerful tool that can help you manage resources effectively and avoid common pitfalls associated with resource leaks.
Here’s a simple example:
try (FileReader fr = new FileReader("file.txt")) {
// use the resource
}
// Output:
// The FileReader will be closed automatically after the try block.
In this example, we create a new FileReader instance within the parentheses of the try statement. This FileReader instance is a resource that needs to be closed after use. By declaring the resource within the try block, Java automatically closes the FileReader after the try block is exited, whether by normal execution flow or by an exception being thrown.
This is just a basic way to use the try-with-resources statement in Java, but there’s much more to learn about managing resources effectively. Continue reading for more detailed explanations and advanced usage scenarios.
Table of Contents
- Unpacking Java’s Try-With-Resources
- Leveraging Try-With-Resources with Multiple Resources
- Handling Exceptions in Try-With-Resources
- Exploring Alternative Methods for Resource Management
- Troubleshooting Java’s Try-With-Resources
- Understanding Resource Management in Java
- Expanding Your Java Horizons: The Bigger Picture
- Wrapping Up:
Unpacking Java’s Try-With-Resources
The try-with-resources statement is a remarkable feature in Java that simplifies working with resources such as files, network connections, or database connections. These resources must be closed after use to prevent resource leaks and other potential issues.
In Java, you can use the try-with-resources statement to automatically close these resources. This feature is available from Java 7 onwards.
Here’s a basic example of how the try-with-resources statement works:
try (FileReader fr = new FileReader("file.txt")) {
int i;
while ((i=fr.read()) !=-1) {
System.out.print((char) i);
}
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// Contents of the file.txt will be printed to the console. The FileReader will be closed automatically after the try block.
In this example, we create a new FileReader instance within the parentheses of the try statement. This FileReader instance is a resource that needs to be closed after use. By declaring the resource within the try block, Java automatically closes the FileReader after the try block is exited, whether by normal execution flow or by an exception being thrown.
The primary advantage of using the try-with-resources statement is that it ensures proper cleanup of resources, reducing the risk of resource leaks. It also makes your code cleaner and easier to read, as you don’t need explicit calls to the close() method in a finally block.
However, it’s important to note that the try-with-resources statement only works with instances of classes that implement the AutoCloseable or Closeable interfaces. If you’re working with a resource that doesn’t implement one of these interfaces, you’ll need to manage closing the resource manually.
Leveraging Try-With-Resources with Multiple Resources
Java’s try-with-resources statement isn’t limited to a single resource. It can handle multiple resources at once, which are closed in the reverse order from which they were created. This ensures that each resource is closed properly, even if an exception occurs.
Let’s look at an example where we read data from one file and write it to another:
try (FileReader fr = new FileReader("input.txt");
FileWriter fw = new FileWriter("output.txt")) {
int i;
while ((i = fr.read()) != -1) {
fw.write(i);
}
} catch (IOException e) {
e.printStackTrace();
}
// Output:
// The data from 'input.txt' is written into 'output.txt'. Both FileReader and FileWriter will be closed automatically after the try block.
In this example, we’re managing two resources: FileReader
and FileWriter
. Both are declared within the try statement and are automatically closed at the end of the statement. If an exception occurs within the try block, both resources are still closed properly.
Handling Exceptions in Try-With-Resources
One of the key benefits of using the try-with-resources statement is its robust exception handling. If an exception is thrown within the try block, it’s caught and handled by the catch block. If multiple exceptions are thrown — for example, when multiple resources fail to close — the first exception is caught and the others are suppressed.
Here’s an example:
try (FailingAutoCloseable fac = new FailingAutoCloseable()) {
throw new Exception("Exception from try block");
} catch (Exception e) {
System.out.println(e.getMessage());
for (Throwable t : e.getSuppressed()) {
System.out.println("Suppressed: " + t.getMessage());
}
}
// Output:
// Exception from try block
// Suppressed: Exception from close
In this example, FailingAutoCloseable
is a hypothetical class that throws an exception when closed. We also throw an exception directly within the try block. The catch block first catches and handles the exception from the try block, then it accesses and prints out the suppressed exception from the close()
method.
This feature of the try-with-resources statement ensures that you’re aware of all exceptions that occur while working with resources, not just the first one.
Exploring Alternative Methods for Resource Management
While the try-with-resources statement is a powerful tool in Java, it’s not the only way to manage resources. There are other methods you can use, such as the finally
block or the close()
method. Let’s dive into these alternatives and see how they compare.
Using the Finally Block
Before the introduction of the try-with-resources statement in Java 7, developers often used a finally
block to ensure that resources were closed properly. Here’s an example:
FileReader fr = null;
try {
fr = new FileReader("file.txt");
int i;
while ((i = fr.read()) != -1) {
System.out.print((char) i);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if (fr != null) {
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
// Output:
// Contents of the file.txt will be printed to the console. The FileReader will be closed in the finally block.
In this example, we manually close the FileReader
in the finally
block. This ensures that the FileReader
is closed whether an exception occurs or not.
However, this method is more verbose and error-prone than using a try-with-resources statement. You have to remember to close each resource and handle exceptions that might occur during closing.
Using the Close() Method
Another way to manage resources is by calling the close()
method on the resource. However, this method is manual and doesn’t offer the automatic resource management that try-with-resources provides.
FileReader fr = new FileReader("file.txt");
int i;
while ((i = fr.read()) != -1) {
System.out.print((char) i);
}
fr.close();
// Output:
// Contents of the file.txt will be printed to the console. The FileReader will be closed manually after use.
In this example, we manually close the FileReader
after use by calling fr.close()
. This method is straightforward, but it doesn’t handle exceptions automatically, and it requires you to remember to close each resource.
Overall, while these methods can be useful in some scenarios, the try-with-resources statement is generally the most effective and efficient way to manage resources in Java. It reduces the risk of resource leaks, handles exceptions automatically, and makes your code cleaner and easier to read.
Troubleshooting Java’s Try-With-Resources
While the try-with-resources statement is a powerful feature in Java, it’s not without its challenges. Let’s explore some common issues you might encounter and how to handle them.
Dealing with Non-Auto-Closeable Resources
The try-with-resources statement works with classes that implement the AutoCloseable
or Closeable
interfaces. If you’re working with a resource that doesn’t implement one of these interfaces, you’ll need to manage closing the resource manually.
For example, let’s say you have a hypothetical NonCloseableResource
class that doesn’t implement AutoCloseable
:
try {
NonCloseableResource ncr = new NonCloseableResource();
// use the resource
} finally {
ncr.close();
}
// Output:
// The NonCloseableResource will be closed manually in the finally block.
In this example, we use a finally
block to ensure that the NonCloseableResource
is closed properly. This is more manual and error-prone than using a try-with-resources statement, but it’s necessary when working with non-auto-closeable resources.
Handling Exceptions in Try-With-Resources
While the try-with-resources statement handles exceptions automatically, it’s important to understand how this works. If an exception occurs within the try block and another exception occurs while closing the resource, the first exception is caught and any subsequent exceptions are suppressed.
Here’s an example:
try (FailingAutoCloseable fac = new FailingAutoCloseable()) {
throw new Exception("Exception from try block");
} catch (Exception e) {
System.out.println(e.getMessage());
for (Throwable t : e.getSuppressed()) {
System.out.println("Suppressed: " + t.getMessage());
}
}
// Output:
// Exception from try block
// Suppressed: Exception from close
In this example, FailingAutoCloseable
is a hypothetical class that throws an exception when closed. We also throw an exception directly within the try block. The catch block first catches and handles the exception from the try block, then it accesses and prints out the suppressed exception from the close()
method.
This feature of the try-with-resources statement ensures that you’re aware of all exceptions that occur while working with resources, not just the first one.
Understanding Resource Management in Java
Resource management is a critical aspect of programming. In Java, resources are objects that must be closed manually after use. These include system resources like files, network connections, and database connections. Improper management of these resources can lead to resource leaks, which can, in turn, degrade system performance and cause your application to behave unpredictably.
Before Java 7, developers had to close these resources manually, typically in a finally
block to ensure that the resource was closed whether an exception occurred or not. However, this approach was verbose and error-prone.
Java 7 introduced a new feature to simplify resource management: the try-with-resources statement. This feature is based on the AutoCloseable
interface, which includes a single method, close()
, that closes the resource.
public interface AutoCloseable {
void close() throws Exception;
}
Any class that represents a resource which needs to be closed after use should implement AutoCloseable
and override its close()
method. This includes many classes in the Java standard library, like FileInputStream
, FileOutputStream
, PrintWriter
, Scanner
, and more.
With the try-with-resources statement, you can declare one or more resources within a try block, and Java will automatically close these resources at the end of the block. This feature ensures proper cleanup of resources, reduces the risk of resource leaks, and makes your code cleaner and easier to read.
Expanding Your Java Horizons: The Bigger Picture
The try-with-resources statement is not just a neat trick for managing a single file or network connection. It’s a powerful tool that can simplify resource management in larger projects and with various types of resources.
When you’re working with multiple resources, the try-with-resources statement can ensure that each resource is closed properly, even if an exception occurs. This can prevent resource leaks and other issues that can degrade system performance.
Moreover, the try-with-resources statement is closely related to other important concepts in Java, like exception handling and file I/O. By mastering the try-with-resources statement, you’re also getting a deeper understanding of these concepts.
Further Resources for Java’s Try-With-Resources
For more insights on Java exception handling and errors, Click Here for an in-depth guide.
If you’re interested in learning more about the try-with-resources statement and related concepts, here are some resources that you might find helpful:
- Understanding Exceptions in Java – Learn about checked and unchecked exceptions.
Basics of Java Try-Catch Blocks – Understand try-catch blocks in Java for exception handling.
Oracle’s Java Tutorials provide a comprehensive introduction to many features of the language.
Baeldung’s Guide to the Try-With-Resources Statement in Java provides a detailed look at the try-with-resources statement.
Try-with-Resources Feature in Java by GeekforGeeks provides a thorough breakdown of the try-with-resources feature in Java.
By exploring these resources and continuing to practice, you can become a master of resource management in Java and write cleaner, more efficient, and more reliable code.
Wrapping Up:
In this comprehensive guide, we’ve delved into the nitty-gritty of Java’s try-with-resources statement, a powerful feature that simplifies resource management and ensures your applications run efficiently and reliably.
We began with the basics, understanding how the try-with-resources statement works and how to use it in simple scenarios. We then delved into more advanced usage, exploring how to use the try-with-resources statement with multiple resources and handle exceptions. We also tackled common challenges you might encounter when using the try-with-resources statement, such as dealing with non-auto-closeable resources and handling multiple exceptions.
Along the way, we examined alternative methods for managing resources in Java, such as using a finally
block or the close()
method. We compared these methods with the try-with-resources statement, highlighting the advantages and drawbacks of each approach.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Try-With-Resources | Automatic resource management, robust exception handling | Only works with auto-closeable resources |
Finally Block | Works with all resources | More verbose, error-prone |
Close() Method | Straightforward, works with all resources | Manual, doesn’t handle exceptions automatically |
Whether you’re just starting out with Java or you’re looking to level up your resource management skills, we hope this guide has given you a deeper understanding of the try-with-resources statement and its capabilities.
With its automatic resource management and robust exception handling, the try-with-resources statement is a powerful tool for writing cleaner, more reliable Java code. Happy coding!