Understanding Java Exceptions: Types and How to Handle
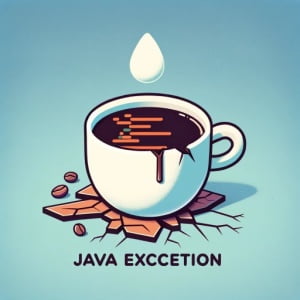
Are you finding it challenging to deal with Java exceptions? You’re not alone. Many developers grapple with this task, but there’s a mechanism that can make this process a breeze.
Think of Java’s exception handling as a vigilant security guard – it can prevent your program from crashing due to unexpected events. These unexpected events can occur on any system, even those with the most robust code.
This guide will walk you through the process of understanding and handling exceptions in Java, from the basics to more advanced topics. We’ll cover everything from using try-catch blocks, creating custom exceptions, to dealing with common issues and their solutions.
So, let’s dive in and start mastering Java exception handling!
TL;DR: What is a Java Exception and How Do I Handle It?
An exception in Java is an event that disrupts the normal flow of the program. The two main types of exceptions are
checked
andunchecked
. They can be handled with various methods such as, a try-catch block:try{} catch(){}
A It is handled using try-catch blocks. Here’s a simple example:
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero");
}
# Output:
# 'Cannot divide by zero'
In this example, we’re trying to divide a number by zero, which is not allowed in mathematics. This operation throws an ArithmeticException
. The try-catch
block catches this exception and prints a custom error message ‘Cannot divide by zero’ instead of crashing the program.
This is a basic way to handle exceptions in Java, but there’s much more to learn about exception handling, including creating custom exceptions, using finally blocks, and more. Continue reading for a more in-depth understanding and advanced usage scenarios.
Table of Contents
- Basic Use of Try-Catch Blocks in Java
- Advanced Exception Handling: Custom Exceptions, Finally Blocks, and Multi-Catch
- Alternative Approaches to Exception Handling in Java
- Troubleshooting Common Issues with Java Exceptions
- Understanding Java Exceptions: The What, Why, and Types
- The Relevance of Exception Handling in Larger Projects and Related Topics
- Wrapping Up: Mastering Java Exception Handling
Basic Use of Try-Catch Blocks in Java
In Java, the primary mechanism to handle exceptions is using try-catch
blocks. The try
block contains the code that might throw an exception, and the catch
block contains the code to handle the exception if one occurs.
Let’s look at a basic example:
try {
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("Array index is out of bounds");
}
# Output:
# 'Array index is out of bounds'
In this example, we’re trying to access the 11th element of an array that only has three elements. This operation throws an ArrayIndexOutOfBoundsException
. The try-catch
block catches this exception and prints a custom error message ‘Array index is out of bounds’ instead of crashing the program.
The advantages of using try-catch
blocks are numerous. They allow you to control the flow of your program and prevent it from crashing due to unexpected exceptions. They also allow you to provide more user-friendly error messages.
However, there are potential pitfalls to be aware of. Overusing try-catch
blocks can make your code harder to read and understand. Also, catching exceptions that you do not know how to handle can lead to unexpected behavior. It’s best practice to only catch exceptions that you know how to handle and let the rest propagate up to a higher level where they can be handled appropriately.
Advanced Exception Handling: Custom Exceptions, Finally Blocks, and Multi-Catch
As you progress in your Java journey, you’ll encounter scenarios that require more advanced exception handling techniques. Let’s explore some of these.
Creating Custom Exceptions
Java allows you to create your own custom exceptions. These are useful when you want to throw exceptions specific to your application’s logic. Here’s how you can create a custom exception:
class CustomException extends Exception {
public CustomException(String errorMessage) {
super(errorMessage);
}
}
try {
throw new CustomException("This is a custom exception");
} catch (CustomException e) {
System.out.println(e.getMessage());
}
# Output:
# 'This is a custom exception'
In this example, we’ve created a CustomException
class that extends the Exception
class. We then throw an instance of CustomException
in our try
block and catch it in our catch
block.
Using Finally Blocks
A finally
block is a block that gets executed no matter whether an exception is thrown or not. Here’s an example:
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("Cannot divide by zero");
} finally {
System.out.println("This is the finally block");
}
# Output:
# 'Cannot divide by zero'
# 'This is the finally block'
In this example, even though an ArithmeticException
is thrown and caught, the finally
block is still executed.
Multi-Catch Blocks
Java 7 introduced the ability to catch multiple exceptions in a single catch block. This can make your code cleaner and easier to read. Here’s an example:
try {
int[] myNumbers = {1, 2, 3};
System.out.println(myNumbers[10]);
} catch (ArrayIndexOutOfBoundsException | NullPointerException e) {
System.out.println("An exception occurred");
}
# Output:
# 'An exception occurred'
In this example, we’re catching both ArrayIndexOutOfBoundsException
and NullPointerException
in the same catch block. If either exception is thrown, the same error message will be printed.
Alternative Approaches to Exception Handling in Java
While try-catch
blocks are the most common way to handle exceptions, Java provides other mechanisms that you might find useful in certain scenarios. One such approach is the use of the throws
keyword.
Using the Throws Keyword
The throws
keyword is used in the method signature to indicate that the method might throw the specified exceptions. It’s a way of signaling to the caller of the method that they should be prepared to handle these exceptions.
Here’s an example of how to use the throws
keyword:
public class Main {
public static void main(String[] args) {
try {
methodThatThrowsException();
} catch (Exception e) {
System.out.println("Exception handled in main method");
}
}
static void methodThatThrowsException() throws Exception {
throw new Exception("Exception thrown in method");
}
}
# Output:
# 'Exception handled in main method'
In this example, the methodThatThrowsException
method is declared with the throws Exception
keyword, indicating that it might throw an Exception
. Inside the main
method, we call methodThatThrowsException
inside a try
block and handle the potential Exception
in a catch
block.
Using the throws
keyword can make your code cleaner by removing the need for try-catch
blocks in your methods. However, it pushes the responsibility of exception handling to the caller of the method. This could be a drawback if the caller is not equipped to handle the exceptions properly.
In the end, the choice between using try-catch
blocks and the throws
keyword depends on your specific use case and how you want to structure your code.
Troubleshooting Common Issues with Java Exceptions
Working with exceptions in Java can sometimes lead to unexpected issues. Let’s discuss some common problems and their solutions.
Catching the General Exception Class
A common mistake is to catch the general Exception
class, which catches all exceptions, including those that you might not be prepared to handle. This can lead to unexpected behavior and make debugging more difficult.
try {
int divideByZero = 5 / 0;
} catch (Exception e) {
System.out.println("An exception occurred");
}
# Output:
# 'An exception occurred'
In this example, we’re catching all exceptions, not just ArithmeticException
. This might not be what we want. It’s generally better to catch specific exceptions that you know how to handle.
Ignoring Exceptions
Another common issue is ignoring exceptions, i.e., catching an exception but not doing anything in the catch block. This can make it difficult to understand what’s going wrong in your program.
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
// do nothing
}
In this example, an ArithmeticException
is thrown, but the catch block is empty. This means that the exception is effectively ignored, and it might be difficult to debug why the division operation is not working as expected.
Not Closing Resources in Finally Block
If you’re using resources like files or database connections in your try
block, you should close them in a finally
block. Failing to do this can lead to resource leaks.
FileReader reader = null;
try {
reader = new FileReader("myfile.txt");
int character = reader.read();
// do something with character
} catch (IOException e) {
System.out.println("An error occurred");
} finally {
if (reader != null) {
try {
reader.close();
} catch (IOException e) {
System.out.println("Failed to close reader");
}
}
}
In this example, we’re using a FileReader
to read a file. We close the FileReader
in a finally
block to ensure that it gets closed even if an exception is thrown.
Understanding Java Exceptions: The What, Why, and Types
Before we delve deeper into handling exceptions in Java, let’s take a step back and understand what exceptions are, why they are used, and the different types of exceptions that exist in Java.
What are Exceptions?
In Java, an exception is an event that disrupts the normal flow of the program. It’s an object that represents an error or a condition that prevents the program from continuing to execute normally. For instance, dividing a number by zero, accessing an out-of-bounds array element, or trying to read a file that doesn’t exist are actions that can lead to exceptions.
Why are Exceptions Used?
Exceptions are used to signal that an error has occurred while executing the program. They provide a way to transfer control from one part of a program to another. When an error occurs within a method, the method creates an exception object and hands it off to the runtime system. This object contains information about the error, including its type and the state of the program when the error occurred.
Types of Exceptions in Java
Java exceptions are classified into two main types: checked exceptions and unchecked exceptions.
- Checked Exceptions: These are exceptions that a method is expected to catch. They extend the
Exception
class and are used for recoverable errors. An example isIOException
.
try {
FileReader reader = new FileReader("nonexistentfile.txt");
} catch (IOException e) {
System.out.println("File not found");
}
# Output:
# 'File not found'
In this example, FileReader
throws an IOException
if the file does not exist. The try-catch
block catches this exception and prints a custom error message.
- Unchecked Exceptions: These are exceptions that a method is not required to catch. They extend the
RuntimeException
class and are used for programming errors. An example isNullPointerException
.
try {
String str = null;
System.out.println(str.length());
} catch (NullPointerException e) {
System.out.println("Null value encountered");
}
# Output:
# 'Null value encountered'
In this example, we’re trying to get the length of a null string, which throws a NullPointerException
. The try-catch
block catches this exception and prints a custom error message.
Understanding these fundamentals about exceptions in Java will help you better grasp the subsequent sections on handling exceptions.
The Relevance of Exception Handling in Larger Projects and Related Topics
Exception handling is not just a tool for small projects or simple programs. It plays an integral role in larger, more complex projects too. The ability to handle exceptions properly can be the difference between a smoothly running application and one riddled with problems.
Exception Handling in Large-Scale Projects
In large-scale projects, exception handling is essential for maintaining the stability and reliability of the software. It allows developers to anticipate and handle errors that can occur during the execution of the program, ensuring that the software can recover gracefully from unexpected events. Without proper exception handling, an unanticipated error could cause the entire system to crash, leading to a poor user experience and potential data loss.
Error Handling and Debugging
Exception handling is closely related to error handling and debugging. While exception handling deals with unexpected events during the execution of the program, error handling is a broader concept that involves dealing with any kind of error, whether it’s a syntax error, logic error, or runtime error.
Debugging, on the other hand, is the process of finding and fixing errors in your code. Proper exception handling can make the debugging process easier by providing meaningful error messages and preserving the program’s state at the time of the error, making it easier to track down the source of the problem.
Further Resources for Mastering Java Exception Handling
For those who want to delve deeper into Java exception handling, here are some valuable resources:
- IOFlood’s Java Errors Explained Article can help you understand Java error messages and debugging.
Understanding Java Optional Class – Learn how to use Optional to avoid NullPointerExceptions.
Java Try-With-Resources Basics – Explore the try-with-resources statement in Java for resource management.
Oracle’s Java Tutorials on Exceptions provide an overview try-catch blocks, the finally block, and custom exceptions in Java.
Baeldung’s Guide on Java Exception Handling goes into more detail about the different types of exceptions and how to handle them.
GeeksforGeeks’ Java Exception Handling Articles cover topics like exception propagation and chaining, and using the throws keyword in Java.
Wrapping Up: Mastering Java Exception Handling
In this comprehensive guide, we’ve delved into the world of Java exceptions, providing you with the knowledge and tools to handle them effectively in your programs.
We began with understanding what Java exceptions are and why they are used. We then explored the basics of handling exceptions using try-catch
blocks, providing you with simple, practical examples to get you started. We gradually moved into more advanced topics, such as creating custom exceptions, using finally
blocks, and catching multiple exceptions in a single catch
block.
Along the way, we tackled common issues you might encounter when dealing with exceptions, such as catching the general Exception
class, ignoring exceptions, and not closing resources in a finally
block. We provided solutions and best practices to help you avoid these pitfalls and write robust, error-resistant code.
We also discussed alternative approaches to handling exceptions, such as using the throws
keyword. This method can make your code cleaner by removing the need for try-catch
blocks in your methods, but it also pushes the responsibility of exception handling to the caller of the method.
Approach | Pros | Cons |
---|---|---|
Try-Catch Blocks | Control over exception handling, user-friendly error messages | Can make code harder to read if overused |
Throws Keyword | Cleaner code, less need for try-catch blocks | Pushes responsibility of exception handling to the caller |
Whether you’re just starting out with Java or you’re an experienced developer looking to brush up on your exception handling skills, we hope this guide has been a valuable resource. Understanding and effectively handling exceptions is a crucial skill in Java programming, and with the knowledge you’ve gained from this guide, you’re well-equipped to handle any exception that comes your way. Happy coding!