Java Optional: Managing Nullable Object References
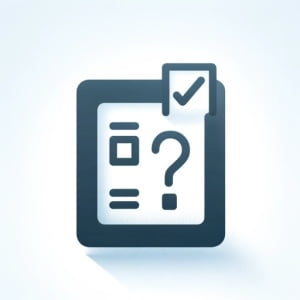
Ever found yourself grappling with null pointer exceptions in Java? You’re not alone. Many developers find themselves in this predicament, but there’s a tool that can help you avoid these pitfalls.
Think of Java Optional as a safety net – a safety net that can save you from the perils of null pointer exceptions. It’s a powerful feature in Java that allows you to handle null values more gracefully, reducing the likelihood of encountering runtime errors.
In this guide, we’ll walk you through the ins and outs of Java Optional, from basic usage to advanced techniques. We’ll cover everything from creating and using Optional objects, to more complex uses such as using Optional with streams and lambda expressions. We’ll also discuss alternatives to Optional, common issues you may encounter, and their solutions.
So, let’s dive in and start mastering Java Optional!
TL;DR: What is Java Optional?
The Java
Optional
class is a container object that may or may not contain a non-null value. Instantiated with the syntax,Optional<String> optional = Optional.of('String');
, It is used to represent nullable object references in Java, providing a more robust alternative to null references.
Here’s a simple example:
Optional<String> optional = Optional.of('Hello');
System.out.println(optional.get());
// Output:
// 'Hello'
In this example, we create an Optional object that contains the string ‘Hello’. The Optional.of()
method is used to create an Optional object that contains a non-null value. The get()
method is then used to retrieve the value contained in the Optional. If the Optional were empty, calling get()
would throw a NoSuchElementException
.
This is just a basic introduction to Java Optional. Continue reading for a more in-depth understanding, including advanced usage scenarios and best practices.
Table of Contents
Getting Started with Java Optional
Java Optional is a powerful tool for handling nullable object references. It’s essentially a container that can hold a non-null value, or no value at all. In this section, we’ll cover the basic usage of Java Optional, including how to create and use an Optional object.
Creating an Optional Object
To create an Optional object, you can use one of three methods: Optional.of()
, Optional.ofNullable()
, and Optional.empty()
.
Optional<String> optionalOf = Optional.of('Hello');
Optional<String> optionalOfNullable = Optional.ofNullable(null);
Optional<String> optionalEmpty = Optional.empty();
In this example, Optional.of('Hello')
creates an Optional object that contains the string ‘Hello’. If you were to pass a null value to Optional.of()
, it would throw a NullPointerException
.
Optional.ofNullable(null)
creates an Optional object that may contain a null value. If the passed value is null, the created Optional object is empty.
Optional.empty()
creates an empty Optional object.
Using an Optional Object
Once you’ve created an Optional object, you can use methods like isPresent()
, ifPresent()
, or orElse()
to interact with the value it may or may not contain.
optionalOf.ifPresent(System.out::println); // Prints 'Hello'
System.out.println(optionalOfNullable.orElse('World')); // Prints 'World'
System.out.println(optionalEmpty.isPresent()); // Prints 'false'
In this example, ifPresent()
is used with a method reference to print the value contained in the Optional object if it’s present. orElse()
is used to provide a default value if the Optional object is empty. isPresent()
returns a boolean indicating whether the Optional object contains a value.
Advantages and Pitfalls
Using Java Optional can help you avoid NullPointerExceptions
, as it forces you to think about the case where a value might be absent. However, it’s not a silver bullet. Overuse of Optional can lead to overly complex code, and it’s still possible to get a NoSuchElementException
if you use get()
on an empty Optional without first checking if a value is present. Therefore, it’s important to use Optional judiciously and always check whether a value is present before attempting to retrieve it.
Deep Dive into Advanced Java Optional
As you become more comfortable with Java Optional, you can start to explore its more advanced features. In this section, we’ll discuss how to use Optional with streams and lambda expressions, which can help you write cleaner and more efficient code.
Using Optional with Streams
Streams in Java are sequences of elements supporting sequential and parallel aggregate operations. You can use Optional with streams to perform complex data transformations and computations.
List<Optional<String>> listOfOptionals = Arrays.asList(Optional.empty(), Optional.of('Hello'), Optional.of('World'));
List<String> filteredValues = listOfOptionals.stream()
.flatMap(Optional::stream)
.collect(Collectors.toList());
System.out.println(filteredValues); // Prints '[Hello, World]'
In this example, we have a list of Optional objects. We want to extract the values from the Optional objects that are present and ignore the ones that are absent. We achieve this by using flatMap()
with Optional::stream
as the argument. This effectively transforms each present value into a single-element stream and each absent value into an empty stream. We then collect the results into a list.
Leveraging Lambda Expressions with Optional
Lambda expressions can be used with Optional to execute code only when a value is present, making your code more readable and reducing boilerplate.
Optional<String> optional = Optional.of('Hello');
optional.ifPresent(value -> System.out.println('Value is present: ' + value)); // Prints 'Value is present: Hello'
In this example, we use ifPresent()
with a lambda expression as the argument. The lambda expression is only executed if the Optional contains a value. This allows us to handle the case where a value is present without having to write explicit null checks.
Intermediate-Level Considerations
While using Optional with streams and lambda expressions can make your code more concise and expressive, it’s important to understand that these techniques come with their own trade-offs. They can make your code more difficult to understand for developers who aren’t familiar with these concepts, and misuse can lead to subtle bugs. As with all tools, it’s important to use them judiciously and understand their implications.
Exploring Alternatives to Java Optional
While Java Optional is a powerful tool for handling nullable object references, it’s not the only game in town. There are other techniques you can use to handle null values in Java, such as null checks and exceptions. In this section, we’ll discuss these alternatives, their benefits and drawbacks, and when you might choose to use them over Optional.
The Classic Null Check
Before Optional was introduced in Java 8, the most common way to handle null values was to use a simple if-else null check.
String value = null;
if (value != null) {
System.out.println(value);
} else {
System.out.println('Value is null');
}
// Output:
// 'Value is null'
In this example, we check if the value is not null before trying to print it. If the value is null, we print a default message instead.
The benefit of this approach is its simplicity. However, it’s easy to forget to perform a null check, which can lead to a NullPointerException
.
Exceptions as an Alternative
Another way to handle null values is to use exceptions. You can throw an exception when a null value is encountered, and then catch that exception and handle it appropriately.
String value = null;
try {
Objects.requireNonNull(value, 'Value cannot be null');
System.out.println(value);
} catch (NullPointerException e) {
System.out.println(e.getMessage());
}
// Output:
// 'Value cannot be null'
In this example, we use the Objects.requireNonNull()
method to throw a NullPointerException
if the value is null. We then catch that exception and print its message.
The benefit of this approach is that it makes it explicit when a null value is not allowed. However, exceptions are expensive to create and handle, so this approach should be used sparingly.
When to Use What?
Choosing between Optional, null checks, and exceptions depends on your specific use case. If you’re dealing with a value that may or may not be present, and you want to avoid NullPointerExceptions
, Optional can be a good choice. If you’re dealing with a value that should never be null, and a null value indicates a programming error, then throwing an exception can be appropriate. And sometimes, a simple null check is all you need.
Remember, the goal is to write clear and correct code. Use the tool that best fits your needs and makes your intentions clear.
Troubleshooting Common Issues with Java Optional
Like any tool, Java Optional comes with its own set of challenges and common issues. One of the most common issues you may encounter when using Optional is NoSuchElementException
. Let’s dive into this issue, explore possible solutions and workarounds, and provide some handy tips.
Dealing with NoSuchElementException
A NoSuchElementException
is thrown when you try to retrieve a value from an empty Optional object using the get()
method. This often happens when you assume the Optional contains a value when it doesn’t.
Optional<String> emptyOptional = Optional.empty();
try {
System.out.println(emptyOptional.get());
} catch (NoSuchElementException e) {
System.out.println('Caught an exception: ' + e.getMessage());
}
// Output:
// 'Caught an exception: No value present'
In this example, we create an empty Optional object and then try to retrieve a value from it using get()
. This results in a NoSuchElementException
.
Solutions and Workarounds
The best way to avoid a NoSuchElementException
is to always check whether a value is present before trying to retrieve it. You can do this using the isPresent()
method or the ifPresent()
method.
if (emptyOptional.isPresent()) {
System.out.println(emptyOptional.get());
} else {
System.out.println('Optional is empty');
}
// Output:
// 'Optional is empty'
In this example, we use isPresent()
to check if the Optional contains a value before trying to retrieve it. If the Optional is empty, we print a default message instead.
Pro Tips for Using Java Optional
- Always check if a value is present before trying to retrieve it.
- Use
orElse()
ororElseGet()
to provide a default value when the Optional is empty. - Avoid using
get()
unless you’re absolutely sure the Optional contains a value. - Use
ifPresent()
with a method reference or lambda expression to execute code only when a value is present.
Remember, Java Optional is a tool to help you write safer and more readable code. Use it wisely, and it can help you avoid many common pitfalls in Java programming.
Understanding Nullability in Java
Before we delve deeper into the advanced use-cases of Java Optional, it’s crucial to have a solid understanding of the concept of nullability in Java, and why Optional was introduced in the first place.
The Problem with Null References
Null references have been a source of headache for developers in many programming languages, including Java. A null reference is essentially a reference that doesn’t point to any object in memory, and trying to access an object through a null reference results in a NullPointerException
.
String str = null;
System.out.println(str.length());
// Output:
// Exception in thread 'main' java.lang.NullPointerException
In this code block, we’re trying to get the length of a string that is null, resulting in a NullPointerException
. Null references can be difficult to debug and handle, especially in large codebases.
The Birth of Java Optional
To address the issues with null references, Java introduced the Optional class in Java 8. Optional is a container object that may or may not contain a non-null value. Instead of using null references, you can use an Optional object to represent the absence of a value.
Optional<String> optional = Optional.ofNullable(null);
System.out.println(optional.isPresent() ? optional.get().length() : 'No value present');
// Output:
// 'No value present'
In this example, we’re using an Optional object to represent a potentially null string. We then use the isPresent()
method to check if a value is present before trying to retrieve it. This helps us avoid a NullPointerException
.
Why Use Java Optional?
Java Optional can help you write more robust code by forcing you to think about the case where a value might be absent. It provides several utility methods to handle the absence of a value in a more controlled and explicit way. While it’s not a silver bullet for all null-related issues, it’s a powerful tool that can help you write safer and more readable code.
Java Optional in Large-Scale Applications
Java Optional has a significant role to play in large-scale applications. It can help improve the readability and maintainability of your code by providing a clear and explicit way to handle the absence of a value. It also encourages developers to think about the possibility of absent values, leading to more robust code.
When using Optional in large-scale applications, here are a few best practices to keep in mind:
- Use
Optional.ofNullable()
when you have a value that might be null, andOptional.of()
when you have a non-null value. - Avoid using
Optional.get()
unless you’re absolutely sure the Optional contains a value. Instead, use methods likeorElse()
,orElseGet()
, ororElseThrow()
to provide a default value or throw an exception when the Optional is empty. - Use
Optional.ifPresent()
to execute code only when a value is present. - Use
Optional.map()
andOptional.flatMap()
to transform the value of an Optional without having to unwrap it.
Exploring Related Concepts
Java Optional is just one tool in the toolbox. To write effective Java code, it’s important to understand and master related concepts like Java Streams and Lambda Expressions.
Java Streams provide a way to process sequences of elements in a functional programming style, while Lambda Expressions provide a way to pass behavior into methods.
List<Optional<String>> listOfOptionals = Arrays.asList(Optional.empty(), Optional.of('Hello'), Optional.of('World'));
List<String> filteredValues = listOfOptionals.stream()
.flatMap(Optional::stream)
.collect(Collectors.toList());
System.out.println(filteredValues); // Prints '[Hello, World]'
In this example, we use a Java Stream to filter a list of Optional objects and extract the present values. We then use a Lambda Expression (Optional::stream
) to transform each Optional into a stream of its present values.
Further Resources for Mastering Java Optional
To continue your journey of mastering Java Optional and related concepts, here are a few resources that you might find helpful:
- Java Error Handling Tutorial: Getting Started – Discover common Java error scenarios.
Understanding Assertion in Java – Learn how to use assert for debugging and testing.
Java Exception Handling Basics – Understand exceptions in Java for error handling.
Oracle’s Official Java Tutorial covers all aspects of Java programming, including Optional, Streams, and Lambda Expressions.
Baeldung’s Guide to Java Optional provides a deep dive into Java Optional, with lots of examples and explanations.
Oracle’s Introduction to Java Streams provides an introduction to Java Streams, with a focus on how to use them with Optional.
Wrapping Up: Mastering Java Optional for Nullability Management
In this comprehensive guide, we’ve explored the ins and outs of Java Optional, a crucial feature in Java for handling nullable object references. We’ve delved into the fundamentals of nullability in Java, the problems with null references, and how Java Optional can help mitigate these issues.
We began with the basics, learning how to create and use Optional objects in Java. We then ventured into more advanced territory, exploring how to use Optional with streams and lambda expressions. Along the way, we tackled common challenges you might face when using Optional, such as NoSuchElementException, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to handling nullability in Java, comparing Java Optional with traditional null checks and exceptions. Here’s a quick comparison of these methods:
Method | Robustness | Ease of Use |
---|---|---|
Java Optional | High | Moderate |
Null Checks | Low | High |
Exceptions | Moderate | Low |
Whether you’re just starting out with Java Optional or you’re looking to level up your nullability management skills, we hope this guide has given you a deeper understanding of Java Optional and its capabilities.
With its balance of robustness and ease of use, Java Optional is a powerful tool for handling nullable object references in Java. Now, you’re well equipped to enjoy the benefits of Java Optional. Happy coding!