Python Add to Set | Guide (With Examples)
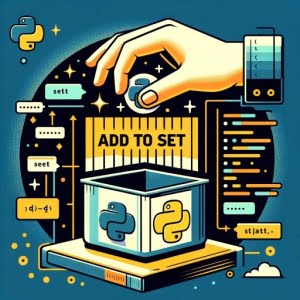
Are you finding it challenging to add elements to a set in Python? You’re not alone. Many developers find themselves puzzled when it comes to handling sets in Python, but we’re here to help.
Think of a set in Python as a basket, and each element you add is like a unique fruit. Adding elements to a set is a fundamental operation in Python that you’ll use frequently when dealing with collections of items.
This guide will walk you through the process of adding elements to a set in Python using the add function. We’ll cover everything from the basics to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering how to add to a set in Python!
TL;DR: How Do I Add an Element to a Set in Python?
To add an element to a set in Python, you can use the
add()
function likefruits.add('apple')
. This function allows you to add a single element to a set.
Here’s a simple example:
fruits = set()
fruits.add('apple')
print(fruits)
# Output:
# {'apple'}
In this example, we first create an empty set named fruits
. Then, we use the add()
function to add the string ‘apple’ to the fruits
set. When we print the fruits
set, we see that it now contains ‘apple’.
This is a basic way to add an element to a set in Python, but there’s much more to learn about managing sets efficiently. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Usage of Python’s add() Function
- Adding Multiple Elements to a Set in Python
- Exploring the update() Function in Python
- Handling Common Issues When Adding to a Set in Python
- Understanding Sets in Python
- The Importance of the add() Function
- Python Sets in Larger Contexts
- Wrapping Up: Mastering Python Sets with the add() Function
Basic Usage of Python’s add() Function
The add()
function in Python is a built-in method for sets that allows you to add a single element to a set. If the element already exists in the set, the function will not add a duplicate.
Here’s a simple example:
fruits = set()
fruits.add('apple')
fruits.add('banana')
fruits.add('apple')
print(fruits)
# Output:
# {'apple', 'banana'}
In the above code, we first create an empty set named fruits
. We then add ‘apple’ and ‘banana’ to the fruits
set using the add()
function. Notice that we attempted to add ‘apple’ twice, but when we print the fruits
set, ‘apple’ only appears once. This is because sets in Python only allow unique elements.
The add()
function is a simple, efficient way to ensure you’re working with unique elements in a set. However, it’s important to remember that sets are unordered collections. This means that the order in which you add elements to a set may not be the order in which they are stored or printed.
Advantages and Potential Pitfalls
The add()
function is straightforward and easy to use, making it a great tool for beginners learning Python. It’s also a powerful function for more advanced developers dealing with larger sets of data where ensuring uniqueness is crucial.
However, one potential pitfall to be aware of is that the add()
function only adds one element at a time. If you want to add multiple elements to a set at once, you’ll need to use a different method, such as the update()
function, which we will discuss later in this guide.
Adding Multiple Elements to a Set in Python
While the add()
function is great for adding individual elements to a set, what if you want to add multiple elements at once? One way is to use a loop in conjunction with the add()
function.
Consider the following example where we add multiple elements to a set using a for
loop:
fruits = set()
new_fruits = ['apple', 'banana', 'cherry', 'date']
for fruit in new_fruits:
fruits.add(fruit)
print(fruits)
# Output:
# {'apple', 'banana', 'cherry', 'date'}
In this example, we first create an empty set named fruits
and a list new_fruits
containing the elements we want to add to the fruits
set. We then use a for
loop to iterate over each element in new_fruits
, and add each one to the fruits
set using the add()
function.
This approach is effective when you have multiple elements to add to a set, especially when those elements are already stored in a list or other iterable. However, it’s worth noting that Python offers an even more efficient way to add multiple elements to a set at once, which we’ll explore in the next section.
Exploring the update() Function in Python
While using the add()
function in a loop is a valid way to add multiple elements to a set, Python provides a more efficient method: the update()
function. The update()
function allows you to add multiple elements to a set in a single operation.
Here’s an example:
fruits = set()
more_fruits = ['apple', 'banana', 'cherry', 'date']
fruits.update(more_fruits)
print(fruits)
# Output:
# {'apple', 'banana', 'cherry', 'date'}
In this example, we first create an empty set named fruits
and a list more_fruits
containing elements we want to add to the fruits
set. We then use the update()
function to add all elements from more_fruits
to the fruits
set in a single operation.
Comparing add() and update()
The add()
and update()
functions in Python both allow you to add elements to a set, but they are used in different scenarios.
- The
add()
function is used to add a single element to a set. It’s straightforward and easy to use, making it a great option for beginners or for adding individual elements. The
update()
function, on the other hand, allows you to add multiple elements to a set at once. This function is more efficient than using theadd()
function in a loop when you have multiple elements to add, especially when those elements are stored in a list or other iterable.
By understanding and using both of these functions, you can manage sets in Python more effectively and efficiently.
Handling Common Issues When Adding to a Set in Python
When working with sets in Python, you may encounter some common issues. Let’s discuss a few of these potential problems and how to solve them.
Adding a Mutable Element
One common issue arises when you try to add a mutable element, like a list, to a set. Since sets only contain immutable (unchangeable) elements, Python will throw an error. Here’s an example:
fruits = set()
fruits.add(['apple', 'banana'])
# Output:
# TypeError: unhashable type: 'list'
In this example, we try to add a list of fruits to the set. Python throws a TypeError because a list is mutable and cannot be added to a set.
One workaround for this issue is to convert the list to a tuple, which is an immutable data type, before adding it to the set:
fruits = set()
fruits.add(tuple(['apple', 'banana']))
print(fruits)
# Output:
# {('apple', 'banana')}
In this code, we first convert the list to a tuple using the tuple()
function, and then add it to the set. This time, Python does not throw an error, and the tuple is successfully added to the set.
By understanding the nature of sets and the types of elements they can contain, you can avoid common errors and find workarounds when necessary.
Understanding Sets in Python
Before we delve further into how to add elements to a set in Python, it’s crucial to understand what a set is and how it works.
A set in Python is an unordered collection of unique elements. It’s similar to a list or a tuple but does not allow duplicate elements. This feature makes sets highly useful when you want to eliminate duplicate entries in your data.
Here’s an example of creating a set in Python:
fruits = {'apple', 'banana', 'cherry'}
print(fruits)
# Output:
# {'apple', 'banana', 'cherry'}
In this example, we create a set named fruits
that contains three elements. When we print the fruits
set, we see that it contains each element only once, even if we tried to add a duplicate.
The Importance of the add() Function
The add()
function plays a crucial role in working with sets in Python. It allows you to add a single element to a set. If the element already exists in the set, the function won’t add a duplicate. This feature aligns with the principle of sets, which don’t allow duplicate elements.
Understanding the fundamentals of sets in Python and the role of the add()
function will help you manage sets more effectively and efficiently.
Python Sets in Larger Contexts
Understanding how to add elements to a set is a fundamental skill in Python, but it’s also important to consider how this knowledge applies to larger scripts or projects. Sets are particularly useful in data analysis, web development, and any scenario where you need to handle collections of unique items.
Exploring Set Operations in Python
Beyond simply adding to a set, Python offers a variety of operations that you can perform on sets, such as union, intersection, and difference. These operations allow you to compare sets and manipulate their contents in powerful ways.
For instance, the union operation combines the elements of two sets, while the intersection operation finds the common elements between two sets. The difference operation, on the other hand, finds the elements that are in one set but not the other.
Here’s a simple example of using these operations:
fruits = {'apple', 'banana', 'cherry'}
more_fruits = {'banana', 'date', 'fig'}
# Union
all_fruits = fruits.union(more_fruits)
print(all_fruits)
# Output:
# {'apple', 'banana', 'cherry', 'date', 'fig'}
# Intersection
common_fruits = fruits.intersection(more_fruits)
print(common_fruits)
# Output:
# {'banana'}
# Difference
unique_fruits = fruits.difference(more_fruits)
print(unique_fruits)
# Output:
# {'apple', 'cherry'}
In this example, we first create two sets, fruits
and more_fruits
. We then use the union()
, intersection()
, and difference()
functions to perform the respective operations on these sets.
Further Resources for Python Set Mastery
To continue your journey in mastering Python sets, check out the following resources:
- Dive into Python’s Data Types to enhance your mathematical capabilities in coding.
Python 2D Array: Working with Two-Dimensional Arrays – Explore 2D arrays in Python for efficient storage and tabular data manipulation.
Exploring Arrays in Python – Learn how to create and manipulate arrays in Python for efficient data handling.
Real Python’s Guide on Python Sets and Set Theory details Python’s sets and their relationship with set theory.
Python Set Operations – Learn set operations in Python with Programiz.
W3Schools’ Python Set Examples and Methods has a wide variety of examples and methods for Python sets.
Wrapping Up: Mastering Python Sets with the add() Function
In this comprehensive guide, we’ve delved deeply into the process of adding elements to a set in Python. We’ve explored the use of the add()
function, discussed its advantages and potential pitfalls, and even ventured into more advanced usage scenarios.
We began with the basics, learning how to add a single element to a set using the add()
function. We then explored how to add multiple elements to a set, first using a loop with the add()
function, and then introducing the update()
function for a more efficient approach.
Along the way, we tackled common issues you might encounter when adding elements to a set, such as trying to add a mutable element like a list. We provided solutions and workarounds for each issue, equipping you with the knowledge to handle these challenges in your own coding projects.
Here’s a quick comparison of the methods we’ve discussed:
Method | Use | Advantages | Potential Pitfalls |
---|---|---|---|
add() | Adding a single element to a set | Simple and easy to use | Only adds one element at a time |
add() in a loop | Adding multiple elements to a set | Can add multiple elements from an iterable | Less efficient for large datasets |
update() | Adding multiple elements to a set | Efficient for adding multiple elements at once | May be less intuitive for beginners |
Whether you’re just starting out with Python or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of how to add elements to a set in Python.
With its balance of simplicity and efficiency, the add()
function is a powerful tool for managing sets in Python. Now, you’re well equipped to handle sets in your own Python projects. Happy coding!