Python Data Types: A Comprehensive Guide
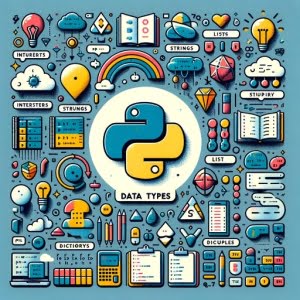
Are you finding it challenging to understand Python data types? You’re not alone. Many programmers, especially beginners, grapple with this concept. Think of Python data types as the building blocks of your Python program – understanding them is crucial for your programming journey.
Whether you’re just starting out with Python or looking to solidify your understanding of Python data types, this guide is for you.
This guide will walk you through the different data types in Python, from the basic to the more complex. We’ll cover everything from the basic data types like integers, floats, and strings to more complex ones like lists, tuples, sets, and dictionaries.
So, let’s dive in and start mastering Python data types!
TL;DR: What Are the Data Types in Python?
Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries. These data types are the foundation of Python programming and are used to store and manipulate data.
Here’s a simple example:
a = 5 # This is an integer
b = 5.0 # This is a float
c = 'hello' # This is a string
# Output:
# a is 5
# b is 5.0
# c is 'hello'
In this example, we’ve defined three variables: a
, b
, and c
. a
is an integer, b
is a float, and c
is a string. These are just three of the many data types available in Python.
But Python’s data types go far beyond integers, floats, and strings. Continue reading for a more detailed exploration of Python data types, including lists, tuples, sets, and dictionaries.
Table of Contents
Python Data Types: The Basics
Let’s start with the basics. Python has several simple data types, including integers, floats, and strings.
Integers
In Python, an integer is a whole number that can be positive, negative, or zero. Here’s an example of how to define an integer:
a = 5 # This is an integer
print(a)
# Output:
# 5
In this example, we’ve defined a variable a
and assigned it the integer value 5
. We then print the value of a
, which is 5
.
Floats
A float, or floating-point number, is a number with a decimal point. Here’s an example of a float:
b = 5.0 # This is a float
print(b)
# Output:
# 5.0
We’ve defined a variable b
and assigned it the float value 5.0
. When we print b
, the output is 5.0
.
Strings
A string is a sequence of characters. In Python, you can define a string using single, double, or triple quotes. Here’s an example of a string:
c = 'hello' # This is a string
print(c)
# Output:
# 'hello'
In this example, we’ve defined a variable c
and assigned it the string value 'hello'
. When we print c
, the output is 'hello'
.
Understanding these basic Python data types is the first step in becoming proficient in Python. In the next section, we’ll dive into more complex Python data types.
Advanced Python Data Types
Now that we’ve covered the basics, let’s dive into some of the more complex data types in Python: lists, tuples, sets, and dictionaries.
Lists
A list in Python is a collection of items that are ordered and changeable. Lists are written with square brackets. Here’s an example:
my_list = [1, 2, 3, 'apple', 'banana']
print(my_list)
# Output:
# [1, 2, 3, 'apple', 'banana']
In this example, my_list
is a list that contains three integers and two strings. Lists in Python can contain different types of data.
Tuples
A tuple is a collection of items that are ordered but unchangeable. Tuples are written with round brackets. Here’s an example:
tuple = (1, 2, 3, 'apple', 'banana')
print(tuple)
# Output:
# (1, 2, 3, 'apple', 'banana')
Here, tuple
is a tuple that contains three integers and two strings. Unlike lists, once a tuple is created, you can’t change its values.
Sets
A set is a collection of items that is unordered and unindexed. Sets are written with curly brackets. Here’s an example:
my_set = {1, 2, 3, 'apple', 'banana'}
print(my_set)
# Output:
# {1, 2, 3, 'apple', 'banana'}
In this example, my_set
is a set that contains three integers and two strings. Sets in Python are unordered, meaning the items will appear in a random order.
Dictionaries
A dictionary is a collection of items that are unordered, changeable, and indexed. Dictionaries are written with curly brackets, and they have keys and values. Here’s an example:
my_dict = {
'name': 'John',
'age': 30,
'city': 'New York'
}
print(my_dict)
# Output:
# {'name': 'John', 'age': 30, 'city': 'New York'}
In this example, my_dict
is a dictionary with three keys: ‘name’, ‘age’, and ‘city’. Each key has a value associated with it.
Understanding these advanced Python data types will allow you to handle more complex data structures in your Python programs.
Mutable and Immutable Python Data Types
In Python, data types are often categorized as mutable or immutable. This categorization is based on whether the value of an object can be changed after it has been created.
Mutable Data Types
Mutable data types are those that allow the contents of variables to be changed. Lists, sets, and dictionaries are examples of mutable data types. Here’s an example using a list:
my_list = [1, 2, 3]
print(my_list)
my_list[1] = 'two'
print(my_list)
# Output:
# [1, 2, 3]
# [1, 'two', 3]
In this example, we create a list my_list
with three integers. We then change the second item in the list from 2
to 'two'
. Because lists are mutable, we can change their contents after they’ve been created.
Immutable Data Types
Immutable data types, on the other hand, do not allow the contents of variables to be changed. Once a variable is assigned a value, that value can’t be changed. Integers, floats, strings, and tuples are examples of immutable data types. Here’s an example using a tuple:
tuple = (1, 2, 3)
print(tuple)
try:
tuple[1] = 'two'
except TypeError:
print('Cannot modify a tuple')
# Output:
# (1, 2, 3)
# Cannot modify a tuple
In this example, we create a tuple tuple
with three integers. We then try to change the second item in the tuple from 2
to 'two'
. However, because tuples are immutable, we can’t change their contents after they’ve been created, and Python raises a TypeError
.
Understanding the difference between mutable and immutable data types in Python will help you decide which type to use based on your specific needs.
Troubleshooting Python Data Types
As you work with Python data types, you may encounter some common issues. Understanding these issues and their solutions can help you write more efficient and error-free code.
Type Errors
One common issue is the TypeError
. This error occurs when an operation or function is applied to an object of inappropriate type. For example, trying to concatenate a string and an integer will result in a TypeError
:
try:
print('hello' + 5)
except TypeError:
print('Cannot concatenate string and integer')
# Output:
# Cannot concatenate string and integer
In this example, we try to concatenate the string 'hello'
and the integer 5
, but Python raises a TypeError
because these two data types can’t be concatenated.
The solution is to ensure that you’re using the correct data types for your operations. In this case, you could convert the integer to a string before concatenating:
print('hello' + str(5))
# Output:
# 'hello5'
Mutability Errors
Another common issue is trying to modify an immutable data type. As we discussed earlier, some Python data types are immutable, which means their values can’t be changed after they’re created. If you try to modify an immutable data type, Python will raise a TypeError
:
try:
tuple = (1, 2, 3)
tuple[1] = 'two'
except TypeError:
print('Cannot modify a tuple')
# Output:
# Cannot modify a tuple
In this example, we try to change the second item in the tuple from 2
to 'two'
, but Python raises a TypeError
because tuples are immutable.
The solution is to use a mutable data type if you need to modify the data. For example, you could use a list instead of a tuple if you need to change the items:
my_list = [1, 2, 3]
my_list[1] = 'two'
print(my_list)
# Output:
# [1, 'two', 3]
Understanding these common issues and their solutions will help you avoid errors and write more efficient Python code.
The Theory Behind Python Data Types
Understanding the theory behind Python data types is crucial for writing effective Python code. But what exactly are data types, and why are they so important in programming?
What Are Data Types?
In programming, a data type is a classification that specifies the type of value a variable can hold and what operations can be performed on it. Python has several built-in data types, including integers, floats, strings, lists, tuples, sets, and dictionaries.
Consider this simple example:
a = 5
print(type(a))
# Output:
# <class 'int'>
In this example, a
is a variable that holds the integer value 5
. When we use the type()
function to check the type of a
, Python tells us that a
is an integer (int
). This information is crucial because it determines what operations we can perform on a
.
Why Are Data Types Important?
Data types are important for several reasons. First, they help ensure data integrity by restricting the type of data that can be stored in a variable. For example, if a variable is intended to hold an integer, assigning a string to it could lead to unexpected results or errors.
Second, data types determine what operations can be performed on a variable. For example, you can perform arithmetic operations on integers and floats, but not on strings or lists.
Third, data types affect how a variable is stored in memory. Different data types require different amounts of memory.
Understanding the theory behind Python data types will help you write more efficient and error-free code. In the next section, we’ll discuss how to apply this knowledge in larger projects and real-world applications.
Applying Python Data Types in Real-World Applications
Understanding Python data types is more than just an academic exercise. It has practical implications in real-world programming and can significantly impact the efficiency and functionality of your Python projects.
For example, choosing the right data type can optimize memory usage and processing speed. If you’re working with numerical data, using integers instead of floats whenever possible can save memory and make your calculations faster. Similarly, using sets instead of lists for large collections of unique items can significantly speed up membership tests.
Understanding the difference between mutable and immutable data types is also crucial for writing robust code. If you’re passing a collection of items to a function and you don’t want the function to be able to change the collection, use a tuple instead of a list.
Moreover, understanding Python’s complex data types like lists, tuples, sets, and dictionaries can help you model complex real-world entities and relationships. For example, you could use a dictionary to model a graph, with the keys representing the nodes and the values representing the edges.
Further Resources for Mastering Python Data Types
If you want to deepen your understanding of Python data types, here are some resources you might find useful:
- Exploring Data Structures in Python – Learn about Python data structures for efficient data organization and manipulation.
Simplifying Set Operations in Python – Discover Python set operations and methods for set algebra and membership testing.
Official Documentation on Python Data Types – Get a comprehensive understanding of Python data structures.
Real Python’s Guide on Python Data Types – Dive into Python’s data types with Real Python’s comprehensive guide.
Python Data Types Tutorial by W3Schools provides an easy tutorial on Python data types for beginners.
These resources provide in-depth explanations and examples of Python data types, and they can help you master this important aspect of Python programming.
Wrapping Up: Mastering Python Data Types
In this comprehensive guide, we’ve delved into the world of Python data types, exploring from the basics to the more complex types.
We began with the basics, understanding simple Python data types like integers, floats, and strings. We then ventured into complex territory, exploring lists, tuples, sets, and dictionaries. We also discussed mutable and immutable data types and their implications in Python programming.
Along the way, we tackled common issues you might encounter when working with Python data types, such as TypeError
and mutability errors, providing solutions and workarounds for each issue.
We also explored the theory behind Python data types, understanding their importance in programming, and how they can impact the efficiency and functionality of your Python projects.
Here’s a quick comparison of Python data types for quick reference:
Data Type | Description | Mutable |
---|---|---|
Integer | Whole numbers | No |
Float | Decimal numbers | No |
String | Sequence of characters | No |
List | Ordered collection of items | Yes |
Tuple | Ordered, unchangeable collection of items | No |
Set | Unordered collection of unique items | Yes |
Dictionary | Unordered collection of key-value pairs | Yes |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your understanding of Python data types, we hope this guide has given you a deeper understanding of Python data types and their uses. Happy coding!