2D Array in Java: Configuring Two-Dimensional Arrays
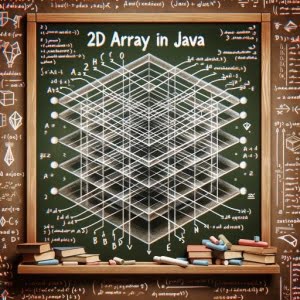
Are you finding it challenging to work with 2D arrays in Java? You’re not alone. Many developers find themselves puzzled when it comes to handling 2D arrays in Java, but we’re here to help.
Think of Java’s 2D arrays as a grid of cells – allowing us to store multiple values in a structured format, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with 2D arrays in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics of multi-dimensional arrays to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: How Do I Create a 2D Array in Java?
To create a 2D array in Java, you use the following syntax:
int[][] array = new int[rows][columns];
. This creates a two-dimensional array with a specified number of rows and columns.
Here’s a simple example:
int[][] array = new int[2][2];
// Output:
// array: [[0, 0], [0, 0]]
In this example, we’ve created a 2D array named array
with 2 rows and 2 columns. By default, all elements of this array are initialized to zero.
This is just a basic way to create a 2D array in Java. There’s much more to learn about working with 2D arrays, including accessing and modifying elements, iterating over the array, and more. Continue reading for a comprehensive guide on mastering 2D arrays in Java.
Table of Contents
- Creating, Accessing, and Modifying Elements in a 2D Array
- More Complex Operations with 2D Arrays
- Exploring Alternative Approaches to 2D Arrays
- Troubleshooting Common Issues with 2D Arrays
- Understanding Arrays and Their Multidimensionality
- The Relevance of 2D Arrays in Real-World Applications
- Wrapping Up: Two-Dimensional Arrays
Creating, Accessing, and Modifying Elements in a 2D Array
Creating a 2D Array
To create a 2D array in Java, we use the following syntax: type[][] arrayName = new type[rows][columns];
.
Here’s a simple example of creating a 2D array in Java:
int[][] array = new int[3][3];
// Output:
// array: [[0, 0, 0], [0, 0, 0], [0, 0, 0]]
This code creates a 2D array named array
with 3 rows and 3 columns. By default, all elements of the array are initialized to zero.
Accessing Elements in a 2D Array
To access an element in a 2D array, you specify the indices of the element in the format arrayName[row][column]
.
Here’s how you can access an element in the array
we created above:
int element = array[1][1];
// Output:
// element: 0
This code retrieves the element at the second row and second column of array
(remember, array indices start at 0).
Modifying Elements in a 2D Array
Modifying an element in a 2D array is similar to accessing an element. You specify the indices of the element you want to modify and assign a new value to it.
Here’s how you can modify an element in array
:
array[1][1] = 5;
// Output:
// array: [[0, 0, 0], [0, 5, 0], [0, 0, 0]]
This code assigns the value 5 to the element at the second row and second column of array
.
In this section, we’ve learned the basics of working with 2D arrays in Java. We’ve seen how to create a 2D array, and how to access and modify its elements. This knowledge forms the foundation for more advanced operations with 2D arrays, which we’ll cover in the next section.
More Complex Operations with 2D Arrays
Iterating Over a 2D Array
You can iterate over a 2D array using nested for-each loops. The outer loop iterates over the rows and the inner loop iterates over the columns.
Here’s an example:
int[][] array = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
for (int[] row : array) {
for (int element : row) {
System.out.print(element + " ");
}
System.out.println();
}
// Output:
// 1 2 3
// 4 5 6
// 7 8 9
This code prints each element in array
, row by row.
Finding the Length of a 2D Array
You can find the total number of rows in a 2D array using array.length
and the number of columns in a row using array[row].length
.
Here’s how you can find the number of rows and columns in array
:
int rows = array.length;
int columns = array[0].length;
// Output:
// rows: 3
// columns: 3
This code finds the number of rows and columns in array
.
Sorting a 2D Array
Java doesn’t provide a built-in method to sort a 2D array, but you can sort each row of the array individually using Arrays.sort()
.
Here’s an example:
import java.util.Arrays;
int[][] array = {{3, 2, 1}, {6, 5, 4}, {9, 8, 7}};
for (int[] row : array) {
Arrays.sort(row);
}
// Output:
// array: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This code sorts each row in array
in ascending order.
In this section, we’ve explored more advanced operations with 2D arrays in Java, including iterating over a 2D array, finding its length, and sorting it. These operations are crucial for manipulating 2D arrays and solving more complex problems.
Exploring Alternative Approaches to 2D Arrays
Using ArrayLists
One alternative to using 2D arrays is to use an ArrayList of ArrayLists. This provides more flexibility as the size of the ArrayList can change dynamically.
Here’s an example of creating a 2D ArrayList and adding elements to it:
import java.util.ArrayList;
ArrayList<ArrayList<Integer>> arrayList = new ArrayList<>();
ArrayList<Integer> row1 = new ArrayList<>();
row1.add(1);
row1.add(2);
row1.add(3);
ArrayList<Integer> row2 = new ArrayList<>();
row2.add(4);
row2.add(5);
row2.add(6);
arrayList.add(row1);
arrayList.add(row2);
// Output:
// arrayList: [[1, 2, 3], [4, 5, 6]]
This code creates a 2D ArrayList named arrayList
and adds two rows to it. The advantage of this approach is that you can add or remove rows and columns dynamically, which is not possible with a 2D array.
However, the downside is that ArrayLists have more overhead than arrays, as they are objects and have additional methods and attributes.
Using the Arrays Class
The Arrays class in Java provides static methods for manipulating arrays. For example, you can use the Arrays.deepToString()
method to print a 2D array, which is easier than using nested loops.
Here’s an example:
import java.util.Arrays;
int[][] array = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
System.out.println(Arrays.deepToString(array));
// Output:
// [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This code prints array
using Arrays.deepToString()
. The advantage of this approach is that it provides a quick and easy way to print a 2D array.
However, the downside is that the Arrays class does not provide methods for more complex operations, such as sorting a 2D array or finding the maximum element.
In this section, we’ve explored alternative approaches to working with 2D arrays in Java, including using ArrayLists and the Arrays class. These methods provide more flexibility and convenience, but also have their downsides. The best approach depends on the specific requirements of your task.
Troubleshooting Common Issues with 2D Arrays
Dealing with Out-of-Bounds Errors
One of the most common issues you might encounter when working with 2D arrays in Java is the ArrayIndexOutOfBoundsException
. This error occurs when you try to access or modify an element at an index that is outside the valid range of indices for the array.
Here’s an example that throws this exception:
int[][] array = new int[3][3];
int element = array[3][3]; // throws ArrayIndexOutOfBoundsException
// Output:
// Exception in thread "main" java.lang.ArrayIndexOutOfBoundsException: 3
This code tries to access the element at the fourth row and fourth column of array
, which does not exist because array
only has three rows and three columns. The valid indices for array
are 0 to 2.
To avoid this error, always ensure that you are accessing or modifying elements within the valid range of indices for the array. You can use the length
property of the array to find the number of rows and the number of columns in a row.
Here’s how you can do it:
int rows = array.length;
int columns = array[0].length;
if (rows > 3 || columns > 3) {
System.out.println("Index out of bounds");
} else {
int element = array[3][3];
}
// Output:
// Index out of bounds
This code checks if the indices are within the valid range before accessing the element at the fourth row and fourth column of array
.
In this section, we’ve discussed how to troubleshoot a common issue when working with 2D arrays in Java. By understanding the causes of these issues and how to avoid them, you can work with 2D arrays more effectively and efficiently.
Understanding Arrays and Their Multidimensionality
What Are Arrays in Java?
An array in Java is a data structure that allows you to store multiple values of the same type in a single variable. This can be extremely useful when you need to store many values, but you don’t want to create a separate variable for each one.
Here’s a simple example of an array in Java:
int[] array = {1, 2, 3, 4, 5};
// Output:
// array: [1, 2, 3, 4, 5]
This code creates an array named array
that can store five integers. The values of the array are initialized to 1, 2, 3, 4, and 5.
Why Use Arrays?
Arrays are used when we need to store and manipulate a large amount of data efficiently. They are particularly useful when the data is structured, as in a list, a grid, or a matrix. Arrays also form the basis for many algorithms and data structures.
How Do Arrays Work in Java?
In Java, an array is an object. This means that it has attributes (such as length
) and methods (such as clone
). The elements of an array are stored in contiguous memory locations, which allows for efficient access and modification.
The Concept of Multidimensionality in Arrays
A multidimensional array is an array of arrays. The most common type of multidimensional array is the two-dimensional array, or 2D array, which is essentially a grid of values.
Here’s an example of a 2D array in Java:
int[][] array = {{1, 2, 3}, {4, 5, 6}, {7, 8, 9}};
// Output:
// array: [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
This code creates a 2D array named array
with three rows and three columns. The values of the array are initialized to the numbers 1 to 9.
In this section, we’ve explored the fundamentals of arrays in Java, including what they are, why they are used, and how they work. We’ve also introduced the concept of multidimensionality in arrays. With this foundation, we can delve deeper into the specifics of 2D arrays and how to work with them.
The Relevance of 2D Arrays in Real-World Applications
2D Arrays in Game Development
2D arrays are widely used in game development, especially in the development of grid-based games such as chess, tic-tac-toe, or minesweeper. In these games, the game board can be represented as a 2D array, where each cell corresponds to a square on the board.
Here’s a simple example of how you might use a 2D array in a tic-tac-toe game:
char[][] board = {{'X', 'O', 'X'}, {'O', 'X', 'O'}, {'X', 'O', 'X'}};
// Output:
// board: [['X', 'O', 'X'], ['O', 'X', 'O'], ['X', 'O', 'X']]
This code creates a 2D array named board
that represents a tic-tac-toe board. The ‘X’ and ‘O’ characters represent the moves made by the two players.
2D Arrays in Image Processing
2D arrays also play a crucial role in image processing. An image can be represented as a 2D array of pixels, where each pixel corresponds to a cell in the array. This allows for efficient manipulation of the image, such as applying filters, resizing, or cropping.
Exploring 3D Arrays and Other Data Structures
If you’ve mastered 2D arrays and are looking for a new challenge, you might want to explore 3D arrays and other data structures in Java. A 3D array is essentially an array of 2D arrays, and it can be used in applications such as 3D graphics or multi-layered game boards.
Further Resources for Mastering Java Arrays
To find out more on how to manipulate arrays in Java, including adding, removing, and modifying elements, Click Here!
We have also gathered various free resources on the Java Array class below:
- Java Array Length – Learn how to retrieve the length of an array in Java.
Understanding Array Methods in Java – Learn about array initialization, modification, and traversal techniques.
Oracle’s Java Tutorials on Arrays provides a comprehensive guide to 2D and 3D arrays in Java.
GeeksforGeeks’ Java Array Articles covers a wide range of topics related to arrays in Java.
Multidimensional Arrays in Java by Programiz provides a detailed understanding of multidimensional arrays in Java.
Wrapping Up: Two-Dimensional Arrays
In this comprehensive guide, we’ve delved into the world of 2D arrays in Java, exploring from the basics to more advanced techniques.
We started with the fundamentals, learning how to create, access, and modify elements in a 2D array. We then ventured into more complex operations, such as iterating over a 2D array, finding its length, and sorting it. We also explored alternative approaches to working with 2D arrays, including using ArrayLists and the Arrays class. Along the way, we tackled common issues that you might encounter when working with 2D arrays, such as out-of-bounds errors, providing you with solutions and tips to overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
2D Arrays | Efficient, easy to use | Fixed size |
ArrayLists | Dynamic size | More overhead |
Arrays Class | Convenience methods | Limited functionality |
Whether you’re a beginner just starting out with 2D arrays in Java, or an experienced developer looking for a refresher, we hope this guide has helped you deepen your understanding and enhance your skills.
The ability to efficiently store and manipulate data in a structured, grid-like format is a powerful tool in any developer’s toolkit. With your newfound mastery of 2D arrays in Java, you’re well equipped to tackle even more complex data structures and algorithms. Happy coding!