Python Logging | Comprehensive Guide (With Examples)
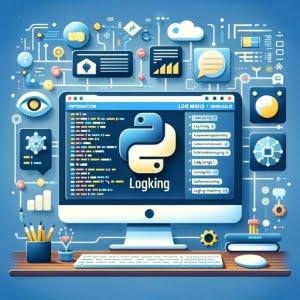
Ever feel like you’re playing detective when tracking down bugs in your Python code? Well, you’re not alone. But what if you had a secret weapon in your detective toolkit? That’s where logging in Python comes in.
Just as a detective relies on their notebook to keep track of clues and leads, a developer can rely on Python logging to keep track of what’s happening in their code.
In this comprehensive guide, we’ll walk you through everything you need to know about Python logging. From setting up basic logging to harnessing its more advanced features, we’ll provide you with the knowledge and tools to become a master of Python logging. So, put on your detective hat and let’s dive in!
TL;DR: How Do I Implement Logging in Python?
Python’s built-in logging module allows you to log messages with different severity levels. Here’s a simple example:
import logging
logging.basicConfig(level=logging.INFO)
logging.info('This is an info message')
# Output:
# INFO:root:This is an info message
In this example, we first import the logging module. We then set the basic configuration for the logging system, specifying that we want to log all messages with a severity level of INFO or higher. We then log an informational message using logging.info()
. The output shows that our message has been logged at the INFO level.
This is just the tip of the iceberg when it comes to Python logging. Read on for a more detailed guide on Python’s logging module, including how to set up more complex logging configurations, use advanced features, and troubleshoot common issues.
Table of Contents
Basics of Python Logging
Python’s built-in logging module is a versatile tool that allows you to log messages at different severity levels. The basic severity levels, in increasing order of severity, are DEBUG, INFO, WARNING, ERROR, and CRITICAL.
Here’s a simple example of how to log messages at these different levels:
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug('This is a debug message')
logging.info('This is an info message')
logging.warning('This is a warning message')
logging.error('This is an error message')
logging.critical('This is a critical message')
# Output:
# DEBUG:root:This is a debug message
# INFO:root:This is an info message
# WARNING:root:This is a warning message
# ERROR:root:This is an error message
# CRITICAL:root:This is a critical message
In the above example, we first import the logging module. We then set the basic configuration for the logging system, specifying that we want to log all messages with a severity level of DEBUG or higher. We then log messages at each of the five severity levels.
Customizing Output Format
Python logging also allows you to customize the format of your log messages. You can include a variety of information in your log messages, such as the time of the log message, the severity level of the message, and the message itself.
Here’s an example of how to customize the format of your log messages:
import logging
logging.basicConfig(format='%(asctime)s - %(levelname)s - %(message)s', level=logging.DEBUG)
logging.info('This is an info message')
# Output:
# 2022-03-20 14:58:15,123 - INFO - This is an info message
In this example, we’ve specified a format string in the basicConfig()
function. This format string contains placeholders for the time of the log message (%(asctime)s
), the severity level of the message (%(levelname)s
), and the message itself (%(message)s
). The output shows the log message with this customized format.
Advanced Features: Python Logging
Once you’ve mastered the basics of Python logging, it’s time to explore its more advanced features. This includes logging exceptions, configuring logging using a configuration file or a dictionary, and using handlers and filters.
Logging Exceptions
Python logging allows you to log exceptions, which can be incredibly useful for debugging. Here’s an example:
import logging
try:
x = 1 / 0
except Exception as e:
logging.error('An error occurred: %s', e, exc_info=True)
# Output:
# ERROR:root:An error occurred: division by zero
# Traceback (most recent call last):
# File "<stdin>", line 2, in <module>
# ZeroDivisionError: division by zero
In this example, we attempt to divide by zero, which raises a ZeroDivisionError
exception. We catch this exception and log it using logging.error()
. The exc_info=True
argument causes the exception’s traceback to be included in the log message.
Configuring Logging Using a Configuration File or a Dictionary
Python logging can also be configured using a configuration file or a dictionary. This allows you to set up complex logging configurations without modifying your application’s code.
Here’s an example of how to configure logging using a dictionary:
import logging
import logging.config
LOGGING_CONFIG = {
'version': 1,
'handlers': {
'console': {
'class': 'logging.StreamHandler',
'level': 'DEBUG',
},
},
'root': {
'handlers': ['console'],
'level': 'DEBUG',
},
}
logging.config.dictConfig(LOGGING_CONFIG)
logging.info('This is an info message')
# Output:
# This is an info message
In this example, we define a dictionary LOGGING_CONFIG
that specifies the logging configuration. We then pass this dictionary to logging.config.dictConfig()
to apply the configuration.
Using Handlers and Filters
Handlers and filters allow you to control where your log messages go and which messages get logged. For instance, you can use a StreamHandler
to send log messages to the console, and a FileHandler
to send log messages to a file.
Here’s an example of how to use handlers and filters:
import logging
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
handler = logging.StreamHandler()
handler.setLevel(logging.INFO)
filter = logging.Filter(name='__main__')
handler.addFilter(filter)
logger.addHandler(handler)
logger.info('This is an info message')
logger.debug('This is a debug message')
# Output:
# This is an info message
In this example, we first create a logger and set its level to DEBUG. We then create a StreamHandler
and set its level to INFO. We also create a Filter
that only allows messages from the __main__
module to be logged. We add this filter to the handler, and add the handler to the logger. As a result, only INFO and higher level messages from the __main__
module are logged to the console.
Exploring Alternatives Tools
While Python’s built-in logging module is powerful and versatile, there are alternative approaches to logging in Python. These include using third-party libraries like Loguru or structlog, and using Python’s warnings module for issuing warning messages.
Loguru: Simplified Logging
Loguru is a third-party library that simplifies logging in Python. It provides a more user-friendly interface and includes features like automatic log rotation and exception capturing.
Here’s an example of how to use Loguru:
from loguru import logger
logger.info('This is an info message')
# Output:
# 2022-03-20 14:58:15.123 | INFO | __main__:<module>:3 - This is an info message
In this example, we import the logger
object from the Loguru library and use it to log an informational message. The output shows the log message with a timestamp, the severity level, the location of the log call, and the message itself.
Structlog: Structured Logging
Structlog is another third-party library that provides structured logging. Structured logging is a logging approach where log messages are structured data, which makes them easier to process and analyze.
Here’s an example of how to use structlog:
import structlog
logger = structlog.get_logger()
logger.info('This is an info message')
# Output:
# 2022-03-20 14:58:15.123 [info ] This is an info message
In this example, we get a logger from the structlog library and use it to log an informational message. The output shows the log message with a timestamp, the severity level, and the message itself.
Python’s Warnings Module
Python’s warnings module is another tool you can use for logging. While it’s not a logging tool per se, it can be used to issue warning messages, which can be useful for logging.
Here’s an example of how to use the warnings module:
import warnings
warnings.warn('This is a warning message')
# Output:
# <stdin>:1: UserWarning: This is a warning message
In this example, we import the warnings module and use it to issue a warning message. The output shows the warning message along with its location.
Each of these alternative approaches has its pros and cons. Loguru provides a simplified interface and additional features, but it’s not as flexible as Python’s built-in logging module. Structlog provides structured logging, but it might be overkill for simple logging needs. The warnings module is part of Python’s standard library, but it’s not a full-fledged logging tool.
Troubleshooting Issues with Logging
As with any tool, you might encounter some issues when implementing logging in Python. These can range from problems with log message formatting to handling multi-threaded logging and managing log file rotation. Let’s address some of these common issues and discuss the solutions and best practices.
Formatting Log Messages
One common issue is formatting log messages. If your log messages aren’t formatted correctly, they can be difficult to read and understand. Here’s an example of how to format your log messages using Python’s logging module:
import logging
logging.basicConfig(format='%(asctime)s - %(levelname)s - %(message)s', level=logging.INFO)
logging.info('This is an info message')
# Output:
# 2022-03-20 14:58:15,123 - INFO - This is an info message
In this code block, we’ve specified a format string in the basicConfig()
function. This format string includes placeholders for the time of the log message (%(asctime)s
), the severity level of the message (%(levelname)s
), and the message itself (%(message)s
). The output shows the log message with this customized format.
Handling Multi-threaded Logging
Another common issue is handling logging in a multi-threaded environment. Python’s logging module is thread-safe, which means you can use it in a multi-threaded application without worrying about race conditions. However, you might encounter issues with log messages from different threads getting mixed up. To avoid this, you can use a logging.Thread
to ensure that each thread logs its messages separately.
Managing Log File Rotation
Managing log file rotation is another common issue. If your application generates a lot of log messages, your log file can quickly become very large. To manage this, you can use a logging.handlers.RotatingFileHandler
, which rotates the log file when it reaches a certain size.
import logging
from logging.handlers import RotatingFileHandler
handler = RotatingFileHandler('my_log.log', maxBytes=2000, backupCount=5)
logger = logging.getLogger(__name__)
logger.addHandler(handler)
for i in range(10000):
logger.info('Hello, world!')
In this example, we create a RotatingFileHandler
that rotates the log file (my_log.log
) when it reaches 2000 bytes. The backupCount
argument specifies that a maximum of five backup files are kept.
By understanding these common issues and how to address them, you can make the most of Python’s logging module and effectively troubleshoot any problems that arise.
The Importance of Logging
Logging is a fundamental aspect of software development that often goes unnoticed until a problem arises. But why is logging so important, and what makes good logging?
The Significance of Logging
Imagine navigating through a dense forest with no map or compass. That’s what debugging without logs can feel like. Logs serve as a compass, guiding developers through the intricate maze of code, providing valuable information about the application’s state and behavior.
In a nutshell, logging provides insights into what’s happening behind the scenes. It helps developers understand the flow of the code, track down bugs, and monitor the performance of the application. It’s also crucial for security audits, helping to detect and investigate suspicious activities.
Principles of Good Logging
But what constitutes good logging? Here are a few principles to consider:
- Relevance: Log messages should be relevant and meaningful. They should provide valuable information that helps understand the state of the application.
Clarity: Log messages should be clear and easy to understand. They should avoid technical jargon and be written in a language that’s easy for the intended audience to comprehend.
Consistency: Log messages should be consistent in their format and terminology. This makes them easier to read and analyze.
Appropriate Level: Log messages should be logged at an appropriate level. For instance, critical errors should be logged at the ERROR or CRITICAL level, while informational messages should be logged at the INFO level.
Minimalism: While it’s important to log enough information to understand what’s happening, excessive logging can lead to information overload. Striking a balance is key.
With a solid understanding of the importance of logging and the principles of good logging, you’re well-equipped to harness the power of Python’s logging module. Remember, good logging practices are not just about troubleshooting and debugging; they’re about creating a clear and insightful narrative of your application’s journey.
Debugging and Python Logging
Python logging is an essential tool in a developer’s toolkit, but it’s just one piece of the broader picture of application monitoring and debugging. It provides valuable insights into your code’s behavior, but to fully understand and optimize your application, you need to explore beyond logging.
From Logging to Monitoring and Debugging
While logging gives you a detailed account of your application, monitoring provides a higher-level view of the system’s health. It involves observing your application over time, tracking metrics like memory usage, CPU usage, and response times. Together, logging and monitoring provide a comprehensive view of your application, from detailed events to high-level trends.
Debugging, on the other hand, is the process of finding and fixing problems in your code. Logging aids debugging by providing clues about where and why a problem occurred. However, debugging often involves other techniques, such as using a debugger to step through your code and inspect its state.
Exploring Related Topics: Error and Exception Handling
Another crucial aspect of robust Python development is effective error and exception handling. Errors are inevitable in any application, but how you handle them can make a significant difference. Python provides tools for catching and handling exceptions, allowing you to control the program flow and provide useful error information.
Here’s a simple example of exception handling in Python:
try:
x = 1 / 0
except ZeroDivisionError as e:
logging.error('An error occurred: %s', e)
# Output:
# ERROR:root:An error occurred: division by zero
In this example, we attempt to divide by zero, which raises a ZeroDivisionError
exception. We catch this exception and log an error message, providing useful feedback about the error.
Further Learning Resources
To deepen your understanding of these topics, consider exploring the following resources:
- Pytest for Beginners – A Hands-On Tutorial on writing test cases with Pytest to validate your Python code.
Python’s try-except Exception Handling – Master the art of creating robust Python programs by effectively handling exceptions.
Assert Statements in Python – Learn about Python’s assert statement and its role in writing and running test cases.
Python’s Official Logging Documentation – This is Python’s official documentation for its built-in logging module, explaining how to leverage logging in Python applications.
Errors and Exceptions Overview: Python Documentation – The official Python tutorial on handling and understanding different types of errors and exceptions in Python.
Loguru Library for Python Logging – Loguru is a third-party library that simplifies logging in Python, and this is its official documentation.
Remember, mastering Python logging is a significant step, but it’s just the beginning. By exploring related topics and tools, you can become a more effective and confident Python developer.
Python Logging: Key Takeaways
Python logging is a powerful tool in any developer’s toolkit, providing valuable insights into your application’s behavior. Whether you’re a novice programmer or a seasoned developer, understanding Python logging is essential for effective debugging and monitoring.
We’ve covered the basics of Python logging, including how to log messages at different severity levels and how to customize the format of your log messages. Here’s a quick recap of a basic logging example:
import logging
logging.basicConfig(level=logging.DEBUG)
logging.debug('This is a debug message')
# Output:
# DEBUG:root:This is a debug message
We’ve also discussed common issues in Python logging, such as formatting log messages, handling multi-threaded logging, and managing log file rotation. Remember, understanding these issues and knowing how to address them can help you make the most of Python’s logging module.
Beyond Python’s built-in logging module, we’ve explored alternative approaches like using third-party libraries such as Loguru and structlog, and using Python’s warnings module. Each of these alternatives has its pros and cons, and the best choice depends on your specific needs.
Finally, we’ve discussed how Python logging fits into the larger picture of application monitoring and debugging. Remember, logging is just one piece of the puzzle. To fully understand and optimize your application, you need to explore beyond logging and delve into related topics like error and exception handling.
In conclusion, Python logging is a versatile and powerful tool that can greatly aid your debugging efforts. By understanding its basics, addressing common issues, and exploring alternative approaches, you can harness the power of Python logging to create robust and maintainable Python applications.