Learn Pytest: Python Testing Framework Guide
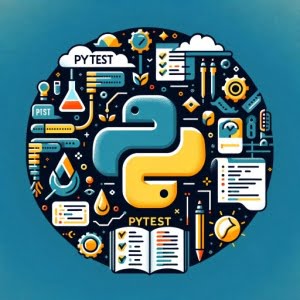
Are you grappling with testing your Python code? Consider pytest, your vigilant night watchman, ensuring your code runs as expected.
This guide will walk you through pytest, a powerful testing framework in Python, from basic usage to advanced features.
Whether you’re a beginner just starting out or an experienced developer looking to streamline your testing process, pytest offers a versatile solution. With its simple syntax and extensive functionality, pytest can help you write better, more reliable code.
So, let’s dive into the world of Python testing with pytest!
TL;DR: What is pytest in Python?
Pytest is a robust testing framework that enables you to write test codes using Python. You can write code to test anything, from simple functions to complex databases. Here’s a simple example:
def test_addition():
assert 1 + 1 == 2
# To run the test, you would use the command `pytest` in your terminal.
# Output:
# ============================= test session starts =============================
# collected 1 item
#
# test_sample.py . [100%]
#
# ============================== 1 passed in 0.12s ==============================
In this example, we’ve defined a simple test function test_addition
that asserts whether the sum of 1 and 1 equals 2. When we run pytest
in the terminal, it automatically finds the test, runs it, and provides a detailed report. The dot (.) in the output indicates that the test passed.
For more advanced usage and best practices, continue reading this comprehensive guide.
Table of Contents
Getting Started with Pytest
Installing Pytest
First things first, we need to install pytest. If you have Python installed, it’s as simple as running the following command in your terminal:
pip install pytest
# Output:
# Collecting pytest
# Downloading pytest-6.2.5-py3-none-any.whl (280 kB)
# ...
# Successfully installed pytest-6.2.5
This command uses pip, Python’s package installer, to download and install pytest.
Writing Your First Test
Now that pytest is installed, let’s write a simple test. In pytest, a test is a function that begins with the word ‘test’. Inside this function, we use assert statements to verify that our code behaves as expected. Here’s an example:
def test_addition():
assert 1 + 1 == 2
In this test, we’re asserting that 1 + 1 equals 2. If this statement is true, the test passes. If it’s false, the test fails.
Running Your Tests
To run your tests, simply navigate to the directory containing your test file and run the pytest
command:
pytest
# Output:
# ============================= test session starts =============================
# collected 1 item
#
# test_sample.py . [100%]
#
# ============================== 1 passed in 0.12s ==============================
In the output, the dot (.) indicates a passing test. If a test fails, it’s marked with an F.
Why Use Pytest?
Pytest offers several advantages for testing:
- Simplicity: Pytest’s assert statements are easy to write and read. There’s no need to remember special assertion methods like in other frameworks.
Detailed reports: Pytest provides detailed reports that can help you quickly identify and fix issues.
Powerful features: Pytest includes several powerful features for more advanced testing needs, which we’ll cover later in this guide.
However, pytest is not without its drawbacks. It can be overkill for very simple projects, and its extensive functionality can be overwhelming for beginners. But with practice and this guide, you can master pytest and make your Python testing efficient and effective.
Advanced Features of Pytest
Pytest Fixtures
Pytest fixtures allow you to create setup code for tests. This is useful when multiple tests need to use the same objects or conditions. Here’s an example:
import pytest
@pytest.fixture
def setup_data():
return [1, 2, 3]
def test_sum(setup_data):
assert sum(setup_data) == 6
In this example, setup_data
is a fixture that returns a list of numbers. This fixture is then used as an argument in test_sum
, providing the data for the test.
Parameterized Tests
Parameterized tests allow you to run a test with different sets of data. Here’s how you can do it:
import pytest
@pytest.mark.parametrize('num1, num2, result',
[(1, 2, 3),
(4, 5, 9),
(6, 7, 13)])
def test_add(num1, num2, result):
assert num1 + num2 == result
In this example, test_add
is run three times with different sets of parameters. This allows you to easily test a function with different inputs.
Pytest Plugins
Pytest supports a wide range of plugins for additional functionality. For example, the pytest-cov
plugin can be used to measure code coverage. Here’s how you can install and use it:
pip install pytest-cov
# Output:
# Collecting pytest-cov
# Downloading pytest_cov-3.0.0-py3-none-any.whl (20 kB)
# ...
# Successfully installed pytest-cov-3.0.0
pytest --cov=myproject
# Output:
# ============================= test session starts =============================
# collected 3 items
#
# test_sample.py ... [100%]
#
# ---------- coverage: platform win32, python 3.9.1-final-0 -----------
# Name Stmts Miss Cover
# ----------------------------------------
# myproject\__init__.py 2 0 100%
# myproject\myproject.py 20 4 80%
# ----------------------------------------
# TOTAL 22 4 82%
# ============================== 3 passed in 0.15s ==============================
In this example, pytest --cov=myproject
runs the tests and measures the code coverage of the myproject
package.
These advanced features make pytest a powerful tool for Python testing. However, they can also make pytest seem complex and intimidating. But with practice and understanding, these features can greatly enhance your testing capabilities.
Alternative Python Testing Frameworks
While pytest is a powerful and versatile testing framework, Python also offers other testing frameworks like unittest and doctest. Let’s compare these alternatives and understand their benefits and drawbacks.
Unittest
Unittest, also known as Python’s built-in testing framework, follows the xUnit architecture. Here’s an example of how to write a test in unittest:
import unittest
class TestAddition(unittest.TestCase):
def test_add(self):
self.assertEqual(1 + 1, 2)
if __name__ == '__main__':
unittest.main()
# Output:
# .
# ----------------------------------------------------------------------
# Ran 1 test in 0.000s
#
# OK
In unittest, tests are written as methods in a test class that inherits from unittest.TestCase
. The assertEqual
method is used to verify the test result.
Benefits of Unittest:
- Built into Python, so no need to install anything.
- Supports setup and teardown methods for test preparation and cleanup.
Drawbacks of Unittest:
- More verbose compared to pytest.
- Does not support fixtures or advanced parameterization out of the box.
Doctest
Doctest allows you to write tests as part of the documentation of a function or module. Here’s an example:
def add(a, b):
'''
This function adds two numbers.
>>> add(1, 1)
2
'''
return a + b
import doctest
doctest.testmod()
# Output:
# TestResults(failed=0, attempted=1)
In doctest, the tests are written as interactive examples in the docstring. The doctest.testmod()
function runs the tests.
Benefits of Doctest:
- Tests can serve as documentation.
- Built into Python.
Drawbacks of Doctest:
- Limited functionality compared to pytest and unittest.
- Not suitable for complex testing scenarios.
In conclusion, while unittest and doctest can be suitable for certain scenarios, pytest’s simplicity, powerful features, and extensive plugin support make it a great choice for most Python testing needs.
Troubleshooting Errors with Pytest
Even with the best of tools, you might encounter issues. Here are a few common errors or obstacles you might face when using pytest, along with their solutions and some best practices for optimization.
Test Not Found
One common issue is pytest not finding your tests. This could be due to several reasons:
- Your test file doesn’t start or end with
test
. - Your test function doesn’t start with
test
. - You’re not in the correct directory.
Ensure your test files and functions are correctly named, and you’re running pytest in the correct directory.
Assertion Errors
Assertion errors occur when a test fails. For example:
def test_addition():
assert 1 + 1 == 3
# Output:
# F
# =================================== FAILURES ===================================
# ________________________________ test_addition _________________________________
#
# def test_addition():
# > assert 1 + 1 == 3
# E assert (1 + 1) == 3
#
# test_sample.py:2: AssertionError
# =============================== short test summary info ===============================
# FAILED test_sample.py::test_addition - assert (1 + 1) == 3
# ============================== 1 failed in 0.12s ==============================
In this example, the test fails because 1 + 1 does not equal 3. The error message provides details on the failed assertion.
Best Practices and Optimization
- Keep tests small and simple: Each test should check a single condition. This makes it easier to identify and fix issues.
Use descriptive test names: The name of your test should describe what it checks. This makes your tests easier to understand.
Clean up after your tests: If your tests create files or modify the database, make sure they clean up after themselves. This prevents tests from interfering with each other.
Use fixtures for setup and teardown: Fixtures are a powerful feature in pytest that can handle setup and teardown for your tests.
Remember, the key to effective testing is not just writing tests, but writing good tests. With these tips and practices, you can make your pytest experience smoother and more efficient.
Role of Testing: Software Development
In the realm of software development, testing holds an undeniable importance. It’s the safety net that catches bugs, errors and unexpected behavior in our code before it reaches the end user. But what does testing entail? Let’s break it down.
The Different Types of Testing
There are different types of testing, each serving a distinct purpose:
- Unit Testing: This involves testing individual components of the software to ensure they work as expected. It’s like checking each brick in a wall.
Integration Testing: This tests the interaction between different software components. It’s akin to checking that the bricks in the wall fit together properly.
Functional Testing: This tests the software against the functional requirements/specifications. It’s like checking that the wall is in the right place in the building.
Regression Testing: This tests the software after modifications to ensure that existing functionality still works. It’s like checking that fixing a crack in the wall didn’t cause any other damage.
Pytest: A Tool for All Your Testing Needs
This is where pytest comes in. Pytest is a testing framework that allows you to write test codes using python, making it suitable for all types of testing.
For instance, let’s consider unit testing. Suppose we have a function add
in our code that we want to test:
def add(a, b):
return a + b
def test_add():
assert add(1, 1) == 2
In this example, test_add
is a unit test for the add
function. It checks whether the function correctly adds two numbers.
Running the test with pytest would look like this:
pytest
# Output:
# ============================= test session starts =============================
# collected 1 item
#
# test_sample.py . [100%]
#
# ============================== 1 passed in 0.12s ==============================
The output shows that the test passed, indicating that our add
function works as expected. In this way, pytest can be used to perform unit testing, and similarly for other types of testing.
In conclusion, testing is a crucial aspect of software development, and pytest is a powerful tool that can handle all your testing needs. By understanding the different types of testing and how to apply pytest for each, you can ensure that your code is robust and reliable.
Software Development Uses of Pytest
Pytest and Continuous Integration/Continuous Deployment (CI/CD)
In the realm of software development, pytest isn’t just a standalone tool. It fits snugly into the larger picture of development practices, especially in Continuous Integration/Continuous Deployment (CI/CD) pipelines.
CI/CD practices involve integrating changes and deploying them to production in a seamless, automated manner. Testing is a crucial part of this process, ensuring that the integrated code is bug-free and ready for deployment.
With pytest, you can automate your testing process and integrate it into your CI/CD pipeline. This way, every time a change is introduced, pytest runs the tests automatically. If any test fails, the pipeline is halted, and the error is reported, allowing for quick fixes.
Pytest and Test-Driven Development (TDD)
Pytest also plays a significant role in Test-Driven Development (TDD), a development practice where tests are written before the code. The code is then written and refactored until it passes the tests.
With its simple syntax and powerful features, pytest is an excellent tool for TDD. It allows you to write your tests easily and run them frequently, driving the development of your code.
Exploring Related Topics
Beyond just testing, pytest opens doors to explore related topics like mocking and test coverage. Mocking is a technique where a function’s dependencies are replaced with ‘mock’ objects, allowing for more controlled testing. Test coverage, on the other hand, measures the amount of code that is covered by your tests.
These topics delve deeper into the realm of testing, enhancing your understanding and capabilities in writing robust, reliable code.
Further Resources for Mastering Pytest
To continue your journey in mastering pytest and Python testing, here are some resources that provide a wealth of information:
- A Guide to Unit Testing in Python – Explore Python’s built-in unittest framework for writing and running unit tests.
Understanding ValueError in Python – Learn about common causes and solutions to Python’s ValueError exception and how to handle it in your code.
Pytest Documentation – The official pytest documentation is a comprehensive resource that covers every aspect of pytest.
Real Python – Real Python offers a variety of tutorials and articles on Python testing, including pytest.
Python Testing with pytest by Brian Okken – This book provides a deep dive into pytest, covering basic to advanced topics in a clear, understandable manner.
With these resources and this guide, you’re well on your way to becoming a pytest expert and elevating your Python testing skills.
Recap: Pytest Usage Guide in Python
In this comprehensive guide, we’ve explored the importance and usage of pytest
in Python.
We’ve dived into the basic usage, making our way from installation and writing simple tests, to running them and understanding the output. We’ve also delved into advanced features such as fixtures, parameterized tests, and plugins, which take your testing capabilities to the next level.
We’ve discussed common issues you might encounter when using pytest, like tests not being found or assertion errors, and how to troubleshoot them. We’ve also shared some best practices for writing good tests and optimizing your pytest experience.
In addition, we’ve seen how pytest fits into the larger picture of software development, playing a crucial role in practices like Continuous Integration/Continuous Deployment (CI/CD) and Test-Driven Development (TDD).
Lastly, we compared pytest with other Python testing frameworks like unittest
and doctest
, highlighting the benefits and drawbacks of each.
Here’s a brief comparison table for quick reference:
Testing Framework | Built-In | Syntax | Advanced Features |
---|---|---|---|
Pytest | No | Simple | Yes |
Unittest | Yes | More verbose | Limited |
Doctest | Yes | Different (In docstrings) | Limited |
In conclusion, while unittest
and doctest
can be suitable for certain scenarios, pytest’s simplicity, powerful features, and extensive plugin support make it a great choice for most Python testing needs.
Whether you’re a beginner just starting out or an experienced developer looking to streamline your testing process, pytest offers a versatile solution. With this guide and continuous practice, you can master pytest and make your Python testing efficient and effective.