Python print() Function | Quick Reference Guide
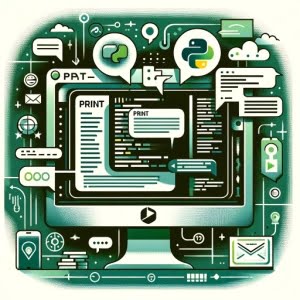
Ever felt like you’re wrestling with Python’s print function? Don’t fret! Consider the print function as your code’s loudspeaker, broadcasting data to your console.
This comprehensive guide will navigate you through Python’s print function, from the rudimentary usage to the more complex techniques. So, whether you’re a beginner or an intermediate aiming to up your Python game, you’re in the right place!
TL;DR: How Do I Use the Print Function in Python?
To print something in Python, you use the
print()
function. It’s as simple as calling the function and passing the message you want to print as an argument. Here’s a quick example:
print('Hello, World!')
# Output:
# Hello, World!
In this example, the print()
function takes the string ‘Hello, World!’ and prints it to the console. This is the most basic use of the ‘python print’ function, but there’s a lot more to it. Keep reading to explore the print function’s capabilities and learn how to leverage its full potential.
Table of Contents
Basic Use of Python’s Print Function
The print()
function in Python is one of the basic building blocks in the language. It’s used to output data to the standard output device (like your screen). The syntax is straightforward:
print(object(s), sep=separator, end=end, file=file, flush=flush)
Let’s break down this syntax:
object(s)
: This could be any object(s), and the print function will convert it into a string before writing it to the standard output. If you pass multiple objects, theprint()
function will print them all.sep=separator
: This is an optional parameter. You can specify how to separate the objects if you’re printing more than one. The default separator is a space.end=end
: This is another optional parameter. You can specify what theprint()
function should print at the end. The default is a newline ().
file=file
: This is yet another optional parameter. By default, theprint()
function outputs to your console. But you could specify a different output file or device here.flush=flush
: This is the final optional parameter. It’s used to specify whether the output is flushed (True) or buffered (False). The default is False.
Here’s a basic example that uses some of these parameters:
print('Hello', 'World', sep=', ', end='!
')
# Output:
# Hello, World!
In this example, we’re printing two strings: ‘Hello’ and ‘World’. We’re separating them with a comma and a space, and we’re ending the line with an exclamation mark. The ‘python print’ function is versatile and provides a lot of flexibility for displaying your data.
Diving Deeper into Python Print
As you become more familiar with Python, you’ll find that the print()
function offers more advanced features that can be incredibly useful. Let’s explore some of these advanced uses of ‘python print’.
Multiple Items and Formatting
The print()
function allows you to print multiple items at once, and it also supports formatted output. Here’s an example:
name = 'John'
age = 30
print(f'{name} is {age} years old.')
# Output:
# John is 30 years old.
In this example, we’re using an f-string (formatted string literal) to insert variables directly into the string. This is a powerful way to create dynamic messages.
Escape Sequences
Escape sequences let you insert special characters into your strings. For instance, you can use for a newline,
for a tab, or
\\
for a backslash. Here’s how you can use escape sequences with the print()
function:
print('Hello\nWorld!')
# Output:
# Hello
# World!
In this example, the escape sequence creates a newline, so ‘Hello’ and ‘World!’ are printed on separate lines. Escape sequences can make your output more readable and organized.
Exploring Alternatives to Python Print
While the print()
function is the go-to for outputting data in Python, there are other methods you can use depending on your specific needs. Let’s delve into some of these alternatives.
Writing with the write()
Function
The write()
function is a method of a file object. You can use it to write a string to a file. Here’s an example:
file = open('example.txt', 'w')
file.write('Hello, World!')
file.close()
# Check the content of the file:
# 'Hello, World!'
In this example, we’re opening a file named ‘example.txt’ in write mode ('w'
), writing a string to it, and then closing the file. The write()
function returns the number of characters written. It’s a powerful tool for writing data to files, but it doesn’t automatically add a newline at the end of the string, unlike print()
.
Logging Data with the logging
Module
For more complex applications, you might want to use the logging
module. This module provides a flexible framework for emitting log messages from Python programs. Here’s a simple example:
import logging
logging.basicConfig(level=logging.INFO)
logging.info('This is an info message')
# Output in the console:
# INFO:root:This is an info message
In this example, we’re setting the log level to INFO, which means the logger will handle all messages that are INFO level or higher. Then, we’re logging an info message. The logging
module is powerful and flexible, but it can be overkill for simple scripts or programs.
Choosing the right output method depends on your specific needs. If you’re just printing data for debugging or simple output, print()
is usually sufficient. If you’re writing data to a file, write()
can be a good choice. For more complex logging needs, the logging
module is a powerful tool.
Troubleshooting Python’s Print Function
Even with a function as straightforward as print()
, you might run into some common issues. Let’s go over some of these potential roadblocks and how to overcome them.
Printing Special Characters
You might run into problems when trying to print special characters. Remember, in Python strings, certain characters have special meanings. For example, is a newline, and
is a tab. If you want to print such characters literally, you need to escape them with a backslash or use a raw string. Here’s an example:
print('C:\\Users\\JohnDoe')
# Output:
# C:\Users\JohnDoe
In this example, we’re printing a Windows file path. Each backslash is escaped with another backslash.
Using print()
in a Function
If you’re using the print()
function inside another function, remember that print()
sends output to the console, not to the calling function. If you want to use the output in your function, you might need to use return
instead of print()
. Here’s an example to illustrate this:
def add_numbers(a, b):
return a + b
result = add_numbers(5, 3)
print(result)
# Output:
# 8
In this example, the add_numbers()
function returns the result, which is then printed. If we used print()
inside add_numbers()
, we wouldn’t be able to use the result in the rest of our code.
Best Practices and Optimization
When using the ‘python print’ function, it’s a good idea to follow some best practices. For example, it’s often better to use formatted string literals (f-strings) instead of concatenating strings with +
, as f-strings are more readable and efficient. Also, remember to close any files you open, or better yet, use a with
statement to handle files, which automatically closes the file when you’re done with it.
Python’s Output Functions and Formatting
To truly master ‘python print’, it’s crucial to understand the concept of standard output and how the print()
function interacts with it.
Standard Output in Python
In Python, standard output (often abbreviated as stdout) is the default destination for output generated by your program. When you use the print()
function without specifying a file or device, it sends the output to stdout, which is usually your console or terminal. Here’s an example:
print('Hello, World!')
# Output:
# Hello, World!
In this example, ‘Hello, World!’ is sent to stdout and displayed in the console.
How print()
Interacts with Standard Output
The print()
function in Python sends data to standard output by default, but you can also specify a different output destination using the file
parameter. For example, you can send output to a file or a different device. Here’s an example of how to do this:
with open('output.txt', 'w') as file:
print('Hello, World!', file=file)
# Check the content of 'output.txt':
# 'Hello, World!'
In this example, we’re opening a file named ‘output.txt’ in write mode, and then we’re using the print()
function to write ‘Hello, World!’ to the file. The print()
function interacts with standard output and other output destinations in a flexible way, making it a powerful tool for displaying and storing your data.
Python Print in Larger Scripts and Projects
The print()
function’s utility extends beyond simple scripts or programs. In larger projects, it can play a crucial role in debugging, logging, and data analysis. For instance, you might use print()
in a loop to track progress or inside a function to log intermediate results. Here’s an example:
for i in range(10):
print(f'Processing item {i+1}...')
# Output:
# Processing item 1...
# Processing item 2...
# ...
# Processing item 10...
In this example, we’re using the print()
function inside a loop to track the processing of items. This can be incredibly useful in larger scripts or projects where you want to monitor the progress of your code.
Accompanying Functions
In Python, print()
often goes hand in hand with other functions like input()
for user input, len()
for getting the length of an object, or str()
for converting an object to a string. These functions can work together to create interactive scripts or handle complex data.
For example, you might use print()
and input()
together to create an interactive script:
name = input('What is your name? ')
print(f'Hello, {name}!')
# Output:
# What is your name? John
# Hello, John!
In this example, we’re using input()
to get the user’s name and print()
to display a greeting. This combination of functions can create a more interactive and dynamic script.
Further Learning and Related Topics
To broaden your knowledge and proficiency in Python, we’ve curated a selection of additional resources that we hope you find useful:
- Printing Lists in Python – Explore Python’s print() function for outputting list elements to the console.
Mastering User Input in Python – A comprehensive guide to handling user input in Python from beginner to advanced concepts.
Python’s Official Tutorial on Input and Output – The official tutorial from Python on managing input and output interactions.
Python Console Output Techniques from Wikiversity – Learn different ways to output information to console in Python.
Remember, the path of learning is a never-ending journey, especially in the ever-evolving field of Python programming!
Wrapping Up Python’s Print Function
In this comprehensive guide, we’ve explored the powerful print()
function in Python. From basic to advanced usage, we’ve seen how this function serves as a versatile tool for broadcasting your code’s data to the console or other output destinations.
To recap, the print()
function allows you to output one or more objects, separated by a specified separator and ending with a specified character. We’ve seen how it can be used in simple scripts as well as larger projects, and how it interacts with standard output. We’ve also discussed how to handle common issues like printing special characters or using print()
in a function.
In addition to print()
, we’ve looked at alternative methods for outputting data, such as the write()
function for writing to files and the logging
module for more complex logging needs.
Here’s a quick comparison of these methods:
Method | Use Case | Example |
---|---|---|
print() | Simple output to console | print('Hello, World!') |
write() | Writing to files | file.write('Hello, World!') |
logging | Complex logging | logging.info('Info message') |
Remember, the right method depends on your specific needs. Whether you’re debugging, logging, or displaying data, Python offers a variety of tools to help you achieve your goals.
Thanks for joining us on this journey through ‘python print’! Keep exploring, keep learning, and keep coding!