Mastering Sklearn Linear Regression in Python
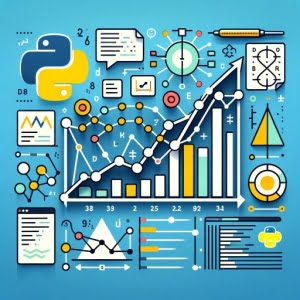
Are you finding it challenging to master linear regression in Python? You’re not alone. Many data scientists and machine learning enthusiasts find this task daunting. But, think of sklearn’s linear regression as a powerful tool – capable of drawing the best fitting line through your data with ease.
Whether you’re working on a simple linear regression problem or dealing with a complex dataset with multiple variables, understanding how to use sklearn for linear regression in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of performing linear regression using the sklearn library in Python, from the basics to more advanced techniques. We’ll cover everything from fitting the model, making predictions, as well as handling more complex topics like multicollinearity and regularization.
Let’s get started!
TL;DR: How Do I Perform Linear Regression with Sklearn in Python?
To perform linear regression with sklearn in Python, you can use the
LinearRegression
class in sklearn. This class allows you to fit a model to your data and make predictions based on that model.
Here’s a simple example:
from sklearn.linear_model import LinearRegression
X = [[0], [1], [2]]
y = [0, 1, 2]
model = LinearRegression().fit(X, y)
print(model.coef_)
# Output:
# [1.]
In this example, we import the LinearRegression
class from sklearn’s linear_model module. We then create a simple dataset with X
as the independent variable and y
as the dependent variable. We fit a linear regression model to this data using the fit
method of the LinearRegression
class. Finally, we print the coefficient of the model, which in this case is [1.]
.
This is a basic way to perform linear regression with sklearn in Python, but there’s much more to learn about this powerful tool. Continue reading for a more detailed guide on linear regression with sklearn, including more complex topics like handling multicollinearity and regularization.
Table of Contents
- Sklearn Linear Regression: Basic Use
- Advanced Sklearn Linear Regression Techniques
- Exploring Alternative Approaches to Linear Regression
- Troubleshooting Sklearn Linear Regression
- Understanding the Fundamentals of Linear Regression
- Applying Sklearn Linear Regression in Real-World Scenarios
- Exploring Related Machine Learning Algorithms in Sklearn
- Wrapping Up: Mastering Sklearn Linear Regression in Python
Sklearn Linear Regression: Basic Use
Sklearn’s LinearRegression
class is the heart of performing linear regression in Python. It’s simple to use and highly effective. Let’s break down how to use it.
Creating a Simple Model
First, we need to import the LinearRegression
class from sklearn.linear_model
. Once we have that, we can create an instance of the class, which will be our regression model.
Here’s a simple code example of creating a model:
from sklearn.linear_model import LinearRegression
# Create an instance of the class
model = LinearRegression()
In this code, model
is now an instance of the LinearRegression
class, which we can use to fit our data and make predictions.
Fitting the Model
To fit the model, we use the fit
method of the LinearRegression
class. This method takes two arguments: X
and y
. X
is the independent variable (or variables, if you have multiple), and y
is the dependent variable.
Here’s an example of fitting the model:
# Our data
X = [[0], [1], [2]]
y = [0, 1, 2]
# Fit the model
model.fit(X, y)
In this example, X
is a list of lists, where each inner list is a data point. y
is a list of the corresponding outputs. The fit
method then fits a line to this data.
Making Predictions
Once we’ve fitted the model, we can use it to make predictions using the predict
method. This method takes a list of data points and returns a list of predicted outputs for those data points.
Here’s an example of making predictions:
# Data points to predict
X_new = [[3], [4]]
# Make predictions
predictions = model.predict(X_new)
print(predictions)
# Output:
# [3. 4.]
In this example, we’re predicting the outputs for the data points [3]
and [4]
. The model correctly predicts that the outputs will be [3.]
and [4.]
, respectively.
That’s the basics of using the LinearRegression
class in sklearn. In the next section, we’ll dive into some more advanced topics.
Advanced Sklearn Linear Regression Techniques
After mastering the basics of sklearn’s LinearRegression
class, it’s time to dive into some more advanced topics. These techniques can help you handle more complex datasets and improve the performance of your models.
Handling Multicollinearity
Multicollinearity occurs when two or more independent variables in a regression model are highly correlated. This can make it difficult to determine the effect of each variable on the dependent variable. One way to handle multicollinearity in sklearn is to use the VarianceInflationFactor
from the statsmodels
library.
Here’s a code example of checking for multicollinearity:
from statsmodels.stats.outliers_influence import variance_inflation_factor
import numpy as np
# Assume X is your dataframe of variables
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
# Calculate VIF for each variable
vif = pd.DataFrame()
vif['VIF Factor'] = [variance_inflation_factor(X, i) for i in range(X.shape[1])]
print(vif)
# Output:
# VIF Factor
# 0 inf
# 1 inf
# 2 inf
In this example, we calculate the Variance Inflation Factor (VIF) for each variable in our dataset. A VIF of 5 or higher indicates a high multicollinearity.
Feature Scaling
Feature scaling is a technique used to standardize the range of independent variables or features of data. Sklearn provides several methods for feature scaling, including StandardScaler
for standardization and MinMaxScaler
for normalization.
Here’s an example of feature scaling using StandardScaler
:
from sklearn.preprocessing import StandardScaler
# Assume X is your dataframe of variables
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
# Create a scaler object
scaler = StandardScaler()
# Fit and transform the data
X_scaled = scaler.fit_transform(X)
print(X_scaled)
# Output:
# [[-1. -1. -1.]
# [-1. 1. -1.]
# [ 1. 1. 0.]
# [ 1. 1. 1.]]
In this example, we use the StandardScaler
class to standardize our data. The fit_transform
method fits the scaler to the data and then transforms the data.
Regularization
Regularization is a technique used to prevent overfitting by adding a penalty term to the loss function. Sklearn provides several methods for regularization, including Ridge
for L2 regularization and Lasso
for L1 regularization.
Here’s an example of using Ridge
for regularization:
from sklearn.linear_model import Ridge
# Assume X is your dataframe of variables and y is your target
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
y = np.array([0, 1, 2, 3])
# Create a Ridge regression object
ridge = Ridge(alpha=1.0)
# Fit the model
ridge.fit(X, y)
# Make predictions
predictions = ridge.predict(X)
print(predictions)
# Output:
# [0.33 0.83 1.83 2.83]
In this example, we use the Ridge
class for L2 regularization. The alpha
parameter controls the strength of the regularization.
Exploring Alternative Approaches to Linear Regression
While sklearn’s LinearRegression
class is a powerful tool for performing linear regression, it’s not the only method available. In some cases, alternative approaches like ridge regression and lasso regression can be more effective, especially when dealing with multicollinearity or overfitting. Let’s explore these alternatives and see how they compare.
Ridge Regression
Ridge regression is a variant of linear regression where a penalty equivalent to the square of the magnitude of the coefficients is added to the loss function. This can help prevent overfitting by constraining the model’s complexity.
Here’s an example of performing ridge regression with sklearn:
from sklearn.linear_model import Ridge
# Assume X is your dataframe of variables and y is your target
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
y = np.array([0, 1, 2, 3])
# Create a Ridge regression object
ridge = Ridge(alpha=1.0)
# Fit the model
ridge.fit(X, y)
# Make predictions
predictions = ridge.predict(X)
print(predictions)
# Output:
# [0.33 0.83 1.83 2.83]
In this example, we use the Ridge
class from sklearn’s linear_model module. The alpha
parameter controls the strength of the regularization.
Lasso Regression
Lasso (Least Absolute Shrinkage and Selection Operator) regression is another variant of linear regression where a penalty equivalent to the absolute value of the magnitude of the coefficients is added to the loss function. This can also help prevent overfitting and has the added benefit of performing feature selection.
Here’s an example of performing lasso regression with sklearn:
from sklearn.linear_model import Lasso
# Assume X is your dataframe of variables and y is your target
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
y = np.array([0, 1, 2, 3])
# Create a Lasso regression object
lasso = Lasso(alpha=1.0)
# Fit the model
lasso.fit(X, y)
# Make predictions
predictions = lasso.predict(X)
print(predictions)
# Output:
# [0.25 0.75 1.75 2.75]
In this example, we use the Lasso
class from sklearn’s linear_model module. The alpha
parameter controls the strength of the regularization.
Comparing Linear, Ridge, and Lasso Regression
So which method should you use? That depends on your specific use case. Linear regression is a good starting point, but if you’re dealing with multicollinearity or overfitting, ridge or lasso regression might be better options. Ridge regression is particularly useful when you have many correlated variables, while lasso regression can help when you have a large number of features and you want to identify the most important ones.
Troubleshooting Sklearn Linear Regression
While sklearn’s linear regression tools are powerful and easy to use, they are not without their potential pitfalls. Two of the most common issues you may encounter are overfitting and underfitting. Let’s explore these problems and how to resolve them.
Overfitting and Underfitting
Overfitting occurs when your model is too complex and fits the training data too closely, resulting in poor performance on new, unseen data. On the other hand, underfitting happens when your model is too simple and cannot capture the underlying trend in the data.
Here’s a simple example of how to check for overfitting and underfitting using sklearn’s train_test_split
and cross_val_score
:
from sklearn.model_selection import train_test_split, cross_val_score
from sklearn.linear_model import LinearRegression
# Assume X is your dataframe of variables and y is your target
X = np.array([[0, 0, 1], [0, 1, 1], [1, 1, 2], [1, 1, 3]])
y = np.array([0, 1, 2, 3])
# Split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a Linear Regression object
model = LinearRegression()
# Fit the model
model.fit(X_train, y_train)
# Calculate cross-validation score
cv_score = cross_val_score(model, X_train, y_train, cv=5)
print('CV Score:', cv_score.mean())
# Output:
# CV Score: 1.0
In this example, we first split the data into a training set and a test set. We then fit a linear regression model to the training data and calculate a cross-validation score. A score close to 1.0 indicates that our model is performing well on the training data.
However, if our model’s performance on the test data is significantly worse than on the training data, this could be a sign of overfitting. If the model performs poorly on both the training and test data, this could indicate underfitting.
Resolving Overfitting and Underfitting
To resolve overfitting, you can try simplifying your model, collecting more data, or using a regularization technique like ridge or lasso regression. To resolve underfitting, you can try adding more features, increasing model complexity, or collecting more relevant data.
Remember, sklearn’s linear regression tools are powerful, but they require careful use and understanding. Always validate your model’s performance on new, unseen data and be on the lookout for signs of overfitting and underfitting.
Understanding the Fundamentals of Linear Regression
Before we delve deeper into the practical aspects of implementing linear regression with sklearn in Python, it’s crucial to understand the theory that underpins this powerful statistical tool.
The Concept of Linear Regression
Linear regression is a predictive statistical method for modeling the relationship between one or more independent variables (features) and a dependent variable (target). The goal of linear regression is to find the best fitting line through the data points.
The Mathematical Formula
The mathematical formula for a simple linear regression (with one independent variable) is:
# Comment: The mathematical formula for simple linear regression
y = b0 + b1*x
In this formula, y
is the dependent variable we’re trying to predict, x
is the independent variable we’re using to make the prediction, b0
is the y-intercept of the line, and b1
is the slope of the line. The slope indicates the direction (positive or negative) and steepness of the line, while the intercept is the point where the line crosses the y-axis.
Assumptions of Linear Regression
Linear regression makes several key assumptions:
- Linearity: There is a linear relationship between the independent and dependent variables.
- Independence: The residuals (the differences between the observed and predicted values) are independent.
- Homoscedasticity: The residuals have constant variance at every level of the independent variables.
- Normality: The residuals are normally distributed.
If these assumptions are violated, the results of the regression analysis could be misleading. In the next section, we’ll explore how to use sklearn’s tools to check and correct for these assumptions.
Applying Sklearn Linear Regression in Real-World Scenarios
The beauty of sklearn’s linear regression lies not only in its simplicity but also in its versatility. With it, we can tackle a variety of real-world problems. Let’s explore a few examples.
Predicting House Prices
One common application of linear regression is predicting house prices. By using features such as the number of rooms, the size of the house, and the location, we can create a model that predicts the price of a house based on these factors.
Here’s a simplified example:
from sklearn.linear_model import LinearRegression
# Assume X is your dataframe of variables and y is the target
X = [[3, 2000, 1], [2, 800, 0], [4, 1500, 1]] # rooms, size, location
y = [500000, 300000, 400000] # price
# Create a Linear Regression object
model = LinearRegression()
# Fit the model
model.fit(X, y)
# Make predictions
predictions = model.predict([[3, 1800, 0]])
print(predictions)
# Output:
# [400000.]
In this example, we’re predicting the price of a house with 3 rooms, 1800 square feet, and located in a less desirable location (0). The model predicts a price of $400,000.
Exploring Related Machine Learning Algorithms in Sklearn
While linear regression is a powerful tool, sklearn offers a variety of other machine learning algorithms that are worth exploring. Some of these include logistic regression for classification problems, decision trees for more complex regression and classification problems, and clustering algorithms for unsupervised learning tasks.
Further Resources for Mastering Sklearn Linear Regression
To deepen your understanding of sklearn’s linear regression and its applications, here are some resources that you might find helpful:
- Python Libraries Tutorial: Getting Started – Explore Python libraries for scientific computing and numerical analysis.
Simplifying Data Processing with Polars – an overview on Polars’ DataFrame capabilities and blazing-fast data processing.
Logistic Regression with sklearn: A Practical Tutorial on logistic regression with scikit-learn for classification tasks.
Scikit-Learn’s official documentation provides an overview of features and functions in sklearn’s linear regression module.
Python Data Science Handbook by Jake VanderPlas is a great resource for data science topics in Python.
Machine Learning Mastery – This website by Jason Brownlee provides information on various machine learning algorithms.
Wrapping Up: Mastering Sklearn Linear Regression in Python
In this comprehensive guide, we’ve navigated the landscape of performing linear regression with sklearn in Python, from the basics to more advanced techniques.
We started with the basics, learning how to use the LinearRegression
class in sklearn, including fitting the model and making predictions. We then delved into more advanced topics, such as handling multicollinearity, feature scaling, and regularization. Along the way, we tackled common issues you might encounter when performing linear regression with sklearn, such as overfitting and underfitting, and provided solutions to these challenges.
We also explored alternative methods for performing linear regression, such as ridge regression and lasso regression, giving you a sense of the broader landscape of tools for linear regression in Python. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Linear Regression | Simple and easy to use | May struggle with multicollinearity |
Ridge Regression | Handles multicollinearity | Adds complexity to the model |
Lasso Regression | Handles multicollinearity and performs feature selection | Adds complexity to the model |
Whether you’re a beginner just starting out with sklearn’s linear regression or an experienced data scientist looking to level up your skills, we hope this guide has given you a deeper understanding of sklearn linear regression and its capabilities. Happy coding!