Understanding Java Syntax: A Detailed Study Guide
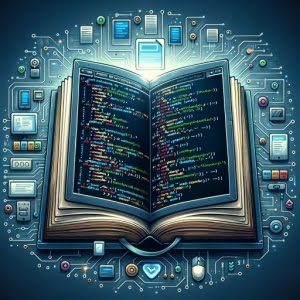
Are you finding Java syntax a bit challenging to grasp? You’re not alone. Many developers find themselves wrestling with the grammar rules of the Java programming language. Think of Java syntax as the building blocks of your Java programs, each one fitting together to create a robust and functional application.
Java syntax is the set of rules that dictate how your Java programs are written and interpreted. These rules are crucial for your Java programs to run correctly and efficiently.
This guide will walk you through the basics of Java syntax and help you become more proficient in Java programming. We’ll cover everything from declaring variables and defining methods to more complex concepts like inheritance, interfaces, and exception handling. We’ll also delve into advanced Java concepts like lambda expressions, generics, and annotations.
So, let’s dive in and start mastering Java syntax!
TL;DR: What is Java Syntax?
Java syntax is the set of rules that define how a Java program is written and interpreted. For instance, every statement in Java ends with a semicolon (;), ie.
System.out.println("Hello, World!");
and classes and methods are defined using specific syntax rules.
Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
# Output:
# Hello, World!
In this example, we’ve created a basic Java program. The public class HelloWorld
line defines a new class named HelloWorld. The public static void main(String[] args)
line defines the main method that is the entry point for any Java program. The System.out.println("Hello, World!");
line is a statement that prints the text “Hello, World!” to the console.
This is just a basic example of Java syntax, but there’s much more to learn about writing and understanding Java programs. Continue reading for a more detailed understanding and examples.
Table of Contents
- Java Syntax 101: Variables, Methods, and Classes
- Delving Deeper: Inheritance, Interfaces, and Exception Handling
- Advanced Java Syntax: Lambda Expressions, Generics, and Annotations
- Overcoming Java Syntax Challenges
- Delving into Java: History and Key Features
- Java Syntax in Real-World Applications
- Wrapping Up: Mastering Java Syntax
Java Syntax 101: Variables, Methods, and Classes
In the realm of Java programming, understanding the basic syntax is the first step towards mastering the language. Let’s start by discussing three fundamental elements of Java syntax: variables, methods, and classes.
Declaring Variables
In Java, a variable is a container that holds values that are used in a Java program. To declare a variable, you need to specify the type and name:
int myNumber;
String myText;
In this example, myNumber
is a variable of type int
(integer), and myText
is a variable of type String
. The variable names follow the type.
Defining Methods
A method in Java is a block of code that performs a specific task. The method is defined with the name of the method, followed by parentheses ()
. Java provides several types of methods, including instance methods, static methods, and abstract methods.
Here’s a simple example of a method in Java:
void sayHello() {
System.out.println("Hello, World!");
}
# Output:
# Hello, World!
In this example, sayHello
is a method that prints the text “Hello, World!” to the console when called.
Creating Classes
A class in Java is a blueprint for creating objects (a particular data structure), providing initial values for state (member variables or attributes), and implementations of behavior (member functions or methods).
Here’s a simple example of a class in Java:
public class MyClass {
int x = 5;
}
# To create an object of MyClass, we use the following code:
MyClass myObj = new MyClass();
System.out.println(myObj.x);
# Output:
# 5
In this example, MyClass
is a simple class with one variable x
. Then, we create an object myObj
of that class and access the variable x
.
Delving Deeper: Inheritance, Interfaces, and Exception Handling
As you become more comfortable with the basics of Java syntax, it’s time to explore some of the more complex concepts that allow for greater flexibility and efficiency in your code. Let’s take a closer look at inheritance, interfaces, and exception handling.
Java Inheritance
Inheritance is one of the four fundamental principles of Object-Oriented Programming (OOP). It allows a ‘child’ class to inherit the fields and methods of a ‘parent’ class.
public class Animal {
public void eat() {
System.out.println("The animal eats");
}
}
public class Dog extends Animal {
public void bark() {
System.out.println("The dog barks");
}
}
# Create a Dog object
Dog myDog = new Dog();
myDog.eat();
myDog.bark();
# Output:
# The animal eats
# The dog barks
In the example above, the Dog
class inherits the eat
method from the Animal
class, and also defines its own method bark
.
Java Interfaces
An interface in Java is a completely abstract class that contains only abstract methods. It is a way to achieve abstraction and multiple inheritance in Java.
interface Animal {
void eat();
}
class Dog implements Animal {
public void eat() {
System.out.println("The dog eats");
}
}
# Create a Dog object
Dog myDog = new Dog();
myDog.eat();
# Output:
# The dog eats
In the example above, Dog
implements the Animal
interface and provides an implementation for the eat
method.
Exception Handling in Java
Exception handling is a powerful mechanism that handles runtime errors so that normal flow of the application can be maintained.
try {
int divideByZero = 5 / 0;
} catch (ArithmeticException e) {
System.out.println("ArithmeticException => " + e.getMessage());
}
# Output:
# ArithmeticException => / by zero
In the example above, we use a try-catch block to handle the ArithmeticException
that occurs when we try to divide by zero.
Advanced Java Syntax: Lambda Expressions, Generics, and Annotations
As you further refine your Java programming skills, you’ll encounter advanced concepts that offer more efficient and flexible ways to code. These include lambda expressions, generics, and annotations.
Lambda Expressions in Java
Lambda expressions are a new and important feature of Java which were introduced in Java 8. They provide a clear and concise way to represent one method interface using an expression.
interface Drawable{
public void draw();
}
public class LambdaExpressionExample {
public static void main(String[] args) {
int width=10;
//with lambda
Drawable d2=()->{
System.out.println("Drawing "+width);
};
d2.draw();
}
}
# Output:
# Drawing 10
In the example above, we’ve defined a Drawable
interface with a single draw
method. In the main
method, we’ve created a lambda expression that implements the draw
method. The draw
method then prints out the value of width
.
Generics in Java
Generics were added to provide type-checking at compile time and to avoid class cast exception that was common while working with collection classes.
import java.util.*;
class TestGenerics{
public static void main(String args[]){
ArrayList<String> list=new ArrayList<String>();
list.add("hello");
String s=list.get(0);
System.out.println("word is: "+s);
}
}
# Output:
# word is: hello
In the example above, we’ve created a generic ArrayList
of String
type. This ensures that we can only add String
objects to the list, providing type safety.
Java Annotations
Annotations provide information about the code and they have no direct effect on the code they annotate. They are used for informational purposes, or for code analysis using annotation processing.
@Override
public String toString(){
return "Overriding toString() method";
}
# Output:
# Overriding toString() method
In the example above, the @Override
annotation is used to instruct the Java compiler that the toString
method is intended to override the toString
method in the superclass.
Overcoming Java Syntax Challenges
While learning Java syntax, you may encounter common errors or obstacles. Understanding these issues and their solutions can help you write more efficient and error-free code. Let’s explore some of these common problems and their solutions.
Missing Semicolons
One of the most common errors beginners in Java face is the missing semicolon at the end of a statement.
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!") // Missing semicolon here
}
}
# Output:
# Error: ';' expected
In the example above, the System.out.println
statement is missing a semicolon at the end, which results in a compile-time error. The solution is to ensure that every statement ends with a semicolon.
Mismatched Braces
Another common error is mismatched braces, either for class definitions, method definitions, or control structures like loops and conditionals.
public class Main {
public static void main(String[] args) {
int x = 10;
if (x > 5) {
System.out.println("x is greater than 5");
// Missing closing brace here
}
}
# Output:
# Error: '}' expected
In the example above, the if
statement is missing a closing brace, which results in a compile-time error. The solution is to ensure that all opening braces have corresponding closing braces.
Incorrect Class or Method Names
Java is case-sensitive, which means myVariable
, MyVariable
, and MYVARIABLE
are different identifiers. This can lead to errors if the case of a class or method name does not match when it is defined and when it is used.
public class Main {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Main main = new main(); // Incorrect case
# Output:
# Error: cannot find symbol
In the example above, the case of the class name Main
does not match when it is used to create an object, which results in a compile-time error. The solution is to ensure that the case of identifiers matches when they are defined and when they are used.
By understanding and avoiding these common errors, you can write cleaner, more efficient Java code. Remember that practice is key when it comes to mastering Java syntax.
Delving into Java: History and Key Features
Java, a class-based, object-oriented programming language, was developed by James Gosling at Sun Microsystems and released in 1995. It was designed to have as few implementation dependencies as possible, allowing developers to ‘write once, run anywhere’ (WORA). This means that compiled Java code can run on all platforms supporting Java without the need for recompilation.
Java is now one of the most popular programming languages in use, particularly for client-server web applications. Its syntax is similar to C and C++, but it has fewer low-level facilities than either of them.
Key Features of Java
Java offers several key features that make it a powerful programming language:
- Platform Independent: Unlike many other programming languages including C and C++, when Java is compiled, it is not compiled into platform-specific machine code, but into platform-independent byte code. This byte code is distributed over the web and interpreted by the Virtual Machine (JVM) on whichever platform it is being run.
Object-Oriented: In Java, everything is an Object. Java can be easily extended since it is based on the Object model.
Simple: Java is designed to be easy to learn. If you understand the basic concept of OOP Java, it would be easy to master.
Secure: With Java’s secure feature, it enables to develop virus-free, tamper-free systems. Authentication techniques are based on public-key encryption.
Robust: Java makes an effort to eliminate error-prone situations by emphasizing mainly on compile-time error checking and runtime checking.
Understanding the background and key features of Java provides a solid foundation for understanding Java syntax. The syntax rules of Java are the building blocks that allow you to leverage these features and write efficient, robust, and portable code.
Mastering Java syntax is crucial for effective programming in Java. It allows you to write code that is clean, easy to read, and easy to maintain. It also helps you avoid common programming errors and understand the logic behind the code you write. With a solid understanding of Java syntax, you’ll be well-equipped to tackle any Java programming challenge.
Java Syntax in Real-World Applications
Java syntax isn’t just a set of rules for writing code. It’s a tool that, when used effectively, can help you create robust, efficient, and maintainable applications. Whether you’re working on a small personal project or a large enterprise application, understanding and using Java syntax correctly is crucial.
Java Syntax in Larger Projects
In larger projects, the importance of correct Java syntax is magnified. Consistency in coding style, including adherence to Java syntax rules, makes the code easier to read and understand, which is particularly important when the project involves multiple developers. Furthermore, correct use of Java syntax can help prevent bugs that are difficult to detect and fix.
public class LargeProject {
// Use of correct Java syntax throughout the project
// can help prevent bugs and make the code easier to read
}
Real-World Applications of Java Syntax
In the real world, Java is used in a wide range of applications, from mobile apps to enterprise-level systems. For instance, Android apps are predominantly written in Java, and many large companies use Java for their back-end systems. In all of these applications, Java syntax plays a crucial role.
public class RealWorldApplication {
// Java syntax is used in a wide range of real-world applications
// from mobile apps to enterprise-level systems
}
Java Libraries, Frameworks, and Development Tools
Once you’ve mastered Java syntax, there’s still plenty more to learn. Java has a rich ecosystem of libraries, frameworks, and development tools that can help you write better code and build more complex applications. For example, libraries like JUnit for testing, Log4j for logging, and JDBC for database connectivity, all rely on a solid understanding of Java syntax. Similarly, frameworks like Spring and Hibernate, and development tools like Eclipse and IntelliJ IDEA, also require proficiency in Java syntax.
Further Resources for Mastering Java Syntax
If you’re looking to further enhance your understanding of Java syntax, here are a few resources that you might find helpful:
- The Many Applications of Java: What Is It Used For? – Learn about the basics of Java’s syntax and usage.
Exploring Collections in Java – Learn about interfaces such as List, Set, Queue, and Map, along with their implementations
Java Import Usage Explained – Master importing techniques for efficient code development in Java.
Oracle’s Java Tutorials cover everything from the basics to advanced topics.
Java Code Geeks offers a wealth of articles, tutorials, code examples, and other resources.
Baeldung offers in-depth tutorials on a wide range of Java topics, including syntax.
Wrapping Up: Mastering Java Syntax
In this comprehensive guide, we’ve journeyed through the world of Java syntax, exploring the rules and structures that govern the Java programming language.
We began with the basics, learning how to declare variables, define methods, and create classes. We then ventured into more advanced territory, exploring complex concepts such as inheritance, interfaces, and exception handling. Along the way, we tackled common challenges you might face when learning Java syntax, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to Java programming, comparing the standard way of doing things with advanced concepts like lambda expressions, generics, and annotations. Here’s a quick comparison of these concepts:
Concept | Complexity | Use Case |
---|---|---|
Standard Syntax | Low | Basic programming tasks |
Advanced Concepts | High | Complex programming tasks |
Whether you’re just starting out with Java or looking to level up your programming skills, we hope this guide has given you a deeper understanding of Java syntax and its importance in effective programming.
Mastering Java syntax is a crucial step in becoming a proficient Java developer. With its balance of simplicity and power, Java syntax allows you to write code that is efficient, readable, and robust. Happy coding!