Mastering Java Inner Classes: From Basics to Advanced
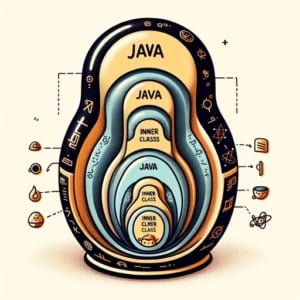
Ever felt like you’re wrestling with encapsulating code within a class in Java? You’re not alone. Many developers find Java inner classes a bit challenging. Think of Java inner classes as secret compartments within a suitcase – keeping related items together and providing a neat and organized way to structure your code.
Java inner classes are a powerful way to logically group classes and to access the outer class’s private members, making them an extremely useful tool in the Java developer’s toolkit.
In this guide, we’ll walk you through the process of using Java inner classes, from the basics to more advanced techniques. We’ll cover everything from defining and using a simple inner class, to dealing with more complex uses such as static inner classes, local inner classes, and anonymous inner classes. We’ll also discuss alternative approaches to achieve similar functionality without using inner classes, and even troubleshoot common issues that may arise when using Java inner classes.
Let’s dive in and start mastering Java inner classes!
TL;DR: What is a Java Inner Class and How Do I Use It?
A Java inner class is a class that is defined within another class, used for logically grouping classes and accessing the outer class’s private members. Here’s a simple example:
class OuterClass {
class InnerClass {
// Inner class code here
}
}
In this example, InnerClass
is defined within OuterClass
. This is a basic inner class in Java. The code for InnerClass
goes inside the inner class block.
This is just a basic way to use Java inner classes, but there’s much more to learn about them. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Use of Java Inner Classes
- Delving Deeper: Advanced Uses of Java Inner Classes
- Exploring Alternatives: Beyond Java Inner Classes
- Troubleshooting Java Inner Classes: Common Issues and Solutions
- Understanding Java Classes: The Foundation of Inner Classes
- Java Inner Classes in the Bigger Picture
- Wrapping Up: Mastering Java Inner Classes
Basic Use of Java Inner Classes
Java inner classes, also known as nested classes, offer a unique way to encapsulate code that belongs together. They can be used to increase the readability and maintainability of your code. Let’s start with a basic example of how to define and use a Java inner class.
public class OuterClass {
private String message = "Hello, World!";
class InnerClass {
void printMessage() {
System.out.println(message);
}
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
OuterClass.InnerClass inner = outer.new InnerClass();
inner.printMessage();
}
}
// Output:
// Hello, World!
In this example, we’ve defined an OuterClass
with a private string message
. Inside OuterClass
, we’ve defined an InnerClass
with a method printMessage()
that prints the outer class’s message
. In the main
method, we first create an instance of OuterClass
, then create an instance of InnerClass
using the outer class instance, and finally call printMessage()
on the inner class instance.
The major advantage of using inner classes is that they can access the private members of the outer class, like the message
variable in our example. This allows for a high level of encapsulation.
However, it’s important to note that inner classes can lead to more complex and hard-to-read code if not used properly. Always ensure your use of inner classes is justified and adds to the clarity of your code.
Delving Deeper: Advanced Uses of Java Inner Classes
As we dive deeper into Java inner classes, we’ll explore static inner classes, local inner classes, and anonymous inner classes. Each of these types has its specific use cases and benefits.
Static Inner Classes
A static inner class is a static member of the outer class and can be used without an instance of the outer class. Here’s an example:
public class OuterClass {
static class StaticInnerClass {
void printMessage() {
System.out.println("Hello from StaticInnerClass!");
}
}
public static void main(String[] args) {
OuterClass.StaticInnerClass inner = new OuterClass.StaticInnerClass();
inner.printMessage();
}
}
// Output:
// Hello from StaticInnerClass!
In this example, StaticInnerClass
is a static member of OuterClass
, so you can create an instance of StaticInnerClass
without first creating an instance of OuterClass
. This can be useful when you want to group related classes but don’t need to access non-static members of the outer class.
Local Inner Classes
A local inner class is defined within a method of the outer class. Here’s an example:
public class OuterClass {
void outerMethod() {
class LocalInnerClass {
void printMessage() {
System.out.println("Hello from LocalInnerClass!");
}
}
LocalInnerClass inner = new LocalInnerClass();
inner.printMessage();
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
outer.outerMethod();
}
}
// Output:
// Hello from LocalInnerClass!
In this example, LocalInnerClass
is defined within the outerMethod
of OuterClass
. This can be useful when you need to encapsulate logic that is only relevant within a specific method.
Anonymous Inner Classes
An anonymous inner class is a class without a name, defined and instantiated in a single statement. Here’s an example:
interface MessagePrinter {
void printMessage();
}
public class Main {
public static void main(String[] args) {
MessagePrinter printer = new MessagePrinter() {
public void printMessage() {
System.out.println("Hello from AnonymousInnerClass!");
}
};
printer.printMessage();
}
}
// Output:
// Hello from AnonymousInnerClass!
In this example, we’ve defined an anonymous inner class that implements the MessagePrinter
interface. This can be useful when you need a one-time use class to implement an interface or extend a class.
Exploring Alternatives: Beyond Java Inner Classes
While Java inner classes offer powerful tools for encapsulation and logical grouping, there are alternative approaches to achieve similar functionality. Let’s explore some of these alternatives and their advantages and disadvantages.
Using Separate Classes
One alternative to using inner classes is to simply use separate classes. For example, instead of defining an inner class within an outer class, you can define each class separately.
public class OuterClass {
private String message = "Hello, World!";
}
public class SeparateClass {
OuterClass outer = new OuterClass();
void printMessage() {
System.out.println(outer.message);
}
}
// Output:
// Error: The field OuterClass.message is not visible
In this example, we’ve tried to access a private member of OuterClass
from SeparateClass
, but it results in an error because private members are not visible outside of their class. This illustrates a key limitation of using separate classes: they cannot access each other’s private members.
Using Interfaces
Another alternative is to use interfaces. An interface in Java is a blueprint of a class that contains static constants and abstract methods. Here’s an example:
interface MessagePrinter {
void printMessage();
}
public class MyClass implements MessagePrinter {
public void printMessage() {
System.out.println("Hello, World!");
}
public static void main(String[] args) {
MyClass myClass = new MyClass();
myClass.printMessage();
}
}
// Output:
// Hello, World!
In this example, MyClass
implements the MessagePrinter
interface and provides an implementation for the printMessage()
method. This can be a powerful way to ensure that a class adheres to a certain contract, but it doesn’t provide the encapsulation benefits of inner classes.
In conclusion, while Java inner classes can be a powerful tool for encapsulation and logical grouping, there are alternative approaches that can be used depending on your specific needs and constraints.
Troubleshooting Java Inner Classes: Common Issues and Solutions
While Java inner classes can be a powerful tool in your Java toolkit, they can sometimes lead to unexpected issues. Let’s discuss some common problems and how to resolve them.
Scope Issues
One common issue is scope-related. Because an inner class has access to the members of the outer class, it can sometimes be unclear which variables are being referred to. Consider this example:
public class OuterClass {
private String message = "Hello, Outer World!";
class InnerClass {
private String message = "Hello, Inner World!";
void printMessage() {
System.out.println(message);
}
}
public static void main(String[] args) {
OuterClass outer = new OuterClass();
OuterClass.InnerClass inner = outer.new InnerClass();
inner.printMessage();
}
}
// Output:
// Hello, Inner World!
In this example, both OuterClass
and InnerClass
have a private string message
. When printMessage()
is called on an instance of InnerClass
, it prints the message
from InnerClass
, not OuterClass
. This is because the inner class’s message
variable shadows the outer class’s message
variable.
To access the outer class’s message
variable from the inner class, you can use the OuterClass.this
syntax:
void printMessage() {
System.out.println(OuterClass.this.message);
}
This will print Hello, Outer World!
, the message
from OuterClass
.
Best Practices
To avoid common issues when using Java inner classes, follow these best practices:
- Keep inner classes small and simple. If an inner class is becoming too complex, it might be better to make it a separate class.
- Avoid shadowing variables. If an inner class needs to access a member of the outer class, give it a unique name to avoid confusion.
- Use static inner classes when possible. Static inner classes do not have a reference to an outer class instance, which can save memory and avoid potential memory leaks.
Understanding Java Classes: The Foundation of Inner Classes
Before we delve deeper into Java inner classes, it’s important to understand the fundamental concept of classes in Java. A class is the blueprint from which individual objects are created. In the real world, you’ll often find many individual objects all of the same kind. For example, your bicycle is an instance (object) of the class of objects known as bicycles.
In the software world, you also define data types. A data type defines the values that a variable can take and the operations that can be performed on it. An object is an instance of a data type. A class is a user-defined data type.
Here’s a simple example of a class in Java:
public class Bicycle {
private int gear;
private int speed;
public Bicycle(int gear, int speed) {
this.gear = gear;
this.speed = speed;
}
public void applyBrake(int decrement) {
speed -= decrement;
}
public void speedUp(int increment) {
speed += increment;
}
}
In this example, Bicycle
is a class that has two private variables: gear
and speed
. It has a constructor to initialize these variables when a new object of the class is created, and it has two methods: applyBrake
and speedUp
.
Encapsulation and Information Hiding
One of the core principles of object-oriented programming is encapsulation. Encapsulation is the mechanism of hiding data (variables) and code (methods) together as a single unit. In Java, the basis of encapsulation is the class.
Encapsulation provides the following benefits:
- Control of data: The data is not accessible to the outside world, and it’s only accessible to members of the same class.
- Increased security: Data and methods are hidden from the outside world, and access is granted only through the methods of the class.
Java inner classes take encapsulation a step further. By defining a class within another class, you can group related classes together and control their visibility in a more granular way.
Java Inner Classes in the Bigger Picture
Java inner classes are not just theoretical constructs; they have practical applications in larger projects and design patterns. They can be a powerful tool for code organization and encapsulation, particularly in complex Java applications.
Inner Classes in Design Patterns
Design patterns are proven solutions to common problems in software design. They represent best practices and can speed up the development process by providing tested, optimized solutions. Java inner classes can play a role in several design patterns, such as the Builder pattern, the Factory pattern, and the Observer pattern.
For example, in the Builder pattern, a static inner class is often used to construct an object of the outer class. The inner class can access the outer class’s private members, which allows it to initialize them in a controlled manner.
Exploring Related Topics
If you’re interested in diving deeper into Java, there are several related topics that you might find interesting. Inheritance, polymorphism, and interfaces in Java are all fundamental concepts in object-oriented programming that tie in closely with inner classes.
- Inheritance is a mechanism that allows one class to acquire the properties (fields) and behaviors (methods) of another class. It’s a key way to promote code reuse and establish relationships between classes.
Polymorphism is the ability of an object to take on many forms. It’s a powerful concept that can make your code more flexible and extensible.
Interfaces in Java are a way to establish a contract for what a class should do, without specifying how it should do it. They’re a key way to achieve abstraction in Java.
Further Resources for Mastering Java Inner Classes
To continue your journey towards mastering Java inner classes and these related topics, check out these resources:
- IOFlood’s Guide to Java Objects – Explore inheritance, polymorphism, and encapsulation through objects in Java.
Default Constructor in Java – Understand the role of the default constructor in Java.
Constructor in Java Overview – Learn about special constructors methods in Java, for initializing object instances.
Oracle’s Java Tutorials offer comprehensive and official documentation on all aspects of Java.
Baeldung’s Java articles provide a wealth of tutorials and guides on a wide range of Java topics.
GeeksforGeeks’ Java Programming offers a variety of tutorials on Java inner classes and related topics.
Wrapping Up: Mastering Java Inner Classes
In this comprehensive guide, we’ve explored the concept of Java inner classes, their usage, benefits, and potential pitfalls. We’ve journeyed from understanding the basics of inner classes to tackling more complex uses and even delving into the alternatives.
We began with the basics, learning how to define and use a simple Java inner class. We then ventured into more advanced territory, exploring static inner classes, local inner classes, and anonymous inner classes. We also tackled common issues you might face when using Java inner classes, such as scope issues, and provided solutions and best practices.
Alongside this, we looked at alternative approaches to achieve similar functionality without using inner classes, such as using separate classes or interfaces. This gave us a broader perspective on the different ways to handle similar situations in Java.
Here’s a quick comparison of the methods we’ve discussed:
Inner Class Type | Pros | Cons |
---|---|---|
Regular Inner Class | Access to outer class’s private members | Can lead to more complex code |
Static Inner Class | Can be used without outer class instance | Cannot access non-static outer class members |
Local Inner Class | Encapsulates logic within a method | Limited scope |
Anonymous Inner Class | Useful for one-time use classes | Can lead to less readable code |
Whether you’re just starting out with Java inner classes or you’re looking to level up your Java skills, we hope this guide has given you a deeper understanding of Java inner classes and their capabilities.
With their ability to encapsulate code and provide logical grouping, Java inner classes are a powerful tool in any Java developer’s toolkit. Happy coding!