Java Constructors: A Deep Dive into Object Initialization
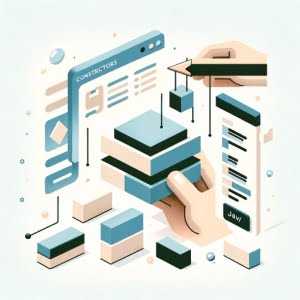
Are you finding it difficult to grasp the concept of constructors in Java? You’re not alone. Many developers find themselves puzzled when it comes to understanding and using constructors in Java, but we’re here to help.
Think of a constructor in Java as a blueprint for a building – it lays the foundation for creating objects, setting the stage for how your program will behave.
In this guide, we’ll walk you through the ins and outs of constructors in Java, from basic usage to advanced techniques. We’ll cover everything from the basics of constructors, their purpose, to more complex uses such as parameterized constructors and constructor overloading.
So, let’s dive in and start mastering constructors in Java!
TL;DR: What is a Constructor in Java and How Do I Use It?
A constructor in Java is a special method used to initialize objects after the class is defined, for example,
public MyClass() { x = 10;}
is the constructor inside ofpublic class MyClass
. The constructor also doesn’t have a return type. Here’s a simple example:
public class MyClass {
int x;
public MyClass() {
x = 10;
}
}
MyClass myObj = new MyClass();
System.out.println(myObj.x);
// Output:
// 10
In this example, we’ve defined a class MyClass
and a constructor within it. The constructor initializes the variable x
to 10. When we create an object myObj
of the class and print myObj.x
, it outputs 10, which shows that the constructor has been successfully used to initialize the object.
This is a basic way to use a constructor in Java, but there’s much more to learn about constructors, including parameterized constructors and constructor overloading. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Exploring the Basics of Constructors in Java
- Delving into Advanced Uses of Constructors in Java
- Exploring Alternative Approaches to Object Creation in Java
- Troubleshooting Common Issues with Java Constructors
- Understanding the Fundamentals of Java
- Beyond Constructors: Exploring Larger Projects and Design Patterns
- Further Resources for Mastering Java Constructors
- Wrapping Up: Java Constructors
Exploring the Basics of Constructors in Java
In Java, a constructor is a block of code that initializes the newly created object. A constructor resembles an instance method in Java but it’s not a method as it doesn’t have a return type. The name of the constructor must be the same as the name of the class. Like methods, constructors also contain the collection of statements(i.e. instructions) that are executed at the time of Object creation.
Constructors are used to provide different ways to create objects. This allows you to initialize an object’s properties at the time of their creation.
Here’s a simple example of a constructor:
public class Vehicle {
String color;
public Vehicle() {
color = "Red";
}
}
Vehicle myCar = new Vehicle();
System.out.println(myCar.color);
// Output:
// Red
In this example, we’ve defined a class Vehicle
, and within it, a constructor. This constructor initializes the color
property to “Red”. When we create a new Vehicle
object (myCar
), the color
property is automatically set to “Red”, as defined by our constructor.
Advantages of Using Constructors
- Constructors provide a way to set initial values for object attributes.
- They can make your code easier to read by clearly indicating how objects should be created.
- They help reduce bugs by ensuring that objects are always created with valid state.
Potential Pitfalls
- If not used carefully, constructors can lead to code duplication. If several constructors perform similar tasks, it might be better to use a method instead.
- Overusing constructors can make code harder to read. It’s important to only use constructors where necessary.
In the following sections, we’ll dive deeper into more complex uses of constructors, such as parameterized constructors and constructor overloading.
Delving into Advanced Uses of Constructors in Java
As you continue your journey with Java, you’ll find that constructors can do more than just initialize variables. They can also take parameters and be overloaded, providing more flexibility and control over object creation.
Parameterized Constructors
A parameterized constructor is a constructor that accepts arguments. This allows you to provide different values to the object at the time of its creation.
Let’s modify our previous Vehicle
class to include a parameterized constructor that accepts a color
parameter:
public class Vehicle {
String color;
public Vehicle(String color) {
this.color = color;
}
}
Vehicle myCar = new Vehicle("Blue");
System.out.println(myCar.color);
// Output:
// Blue
In this example, we’ve created a new Vehicle
object (myCar
) and passed “Blue” as an argument to the constructor. The constructor then sets the color
property to the passed argument, which is “Blue” in this case.
Constructor Overloading
Java allows constructors to be overloaded, meaning you can have multiple constructors in a class, each with a different parameter list. The correct constructor to use is determined at runtime based on the arguments you provide when creating the object.
Let’s add another constructor to our Vehicle
class that accepts both color
and type
parameters:
public class Vehicle {
String color;
String type;
public Vehicle(String color) {
this.color = color;
}
public Vehicle(String color, String type) {
this.color = color;
this.type = type;
}
}
Vehicle myCar = new Vehicle("Blue", "Sedan");
System.out.println(myCar.color + " " + myCar.type);
// Output:
// Blue Sedan
In this example, we’ve added a second constructor to our Vehicle
class that accepts both color
and type
parameters. When we create a new Vehicle
object (myCar
) and pass “Blue” and “Sedan” as arguments, the second constructor is used, setting both the color
and type
properties.
Best Practices
While constructors provide flexibility, they should be used judiciously to maintain clean and readable code. Here are some best practices to keep in mind:
- Keep it simple: Constructors should generally be used for setting initial state. Avoid complex logic in constructors.
- Use constructor chaining: If you have multiple constructors, you can use
this()
to call one constructor from another. This helps reduce code duplication. - Use meaningful names for parameters: Parameter names should clearly indicate their purpose. This makes your code easier to read and understand.
In the next section, we’ll explore alternative approaches to handle object creation and initialization.
Exploring Alternative Approaches to Object Creation in Java
While constructors are a common way to create and initialize objects in Java, they’re not the only way. Let’s delve into an alternative approach that you can use, particularly in more complex scenarios: factory methods.
Factory Methods: A Powerful Alternative
Factory methods are static methods that return an instance of the class. They’re typically used in situations where using a constructor isn’t clear or concise enough, or when you want to use a method name to better describe the created object.
Here’s an example of how you might use a factory method in our Vehicle
class:
public class Vehicle {
String color;
String type;
private Vehicle(String color, String type) {
this.color = color;
this.type = type;
}
public static Vehicle createBlueSedan() {
return new Vehicle("Blue", "Sedan");
}
}
Vehicle myCar = Vehicle.createBlueSedan();
System.out.println(myCar.color + " " + myCar.type);
// Output:
// Blue Sedan
In this example, instead of using a constructor to create a blue sedan, we’ve used a factory method: createBlueSedan()
. This method creates and returns a new Vehicle
object with the color set to “Blue” and the type set to “Sedan”. This approach can make your code more readable and expressive, particularly in complex scenarios.
Advantages and Disadvantages of Factory Methods
Like any approach, factory methods have their pros and cons. Here are some key points to consider:
Advantages:
- Increased readability: Factory methods have explicit names, which can make your code easier to read and understand.
- Flexibility: Factory methods can return any subtype of their return type, allowing more flexibility in your code.
Disadvantages:
- Code complexity: Factory methods can make your code more complex, as you’ll need to create a new method for each different way you want to create an object.
Code duplication: If not used carefully, you can end up with code duplication, as each factory method needs to manually create and initialize a new object.
Recommendations
While constructors are usually the go-to choice for object creation in Java, factory methods can be a powerful tool in your arsenal, particularly in more complex scenarios. As with any tool, the key is knowing when to use it. Use constructors for simple, straightforward object creation, and consider factory methods when you need more control or expressiveness.
Troubleshooting Common Issues with Java Constructors
As you work with constructors in Java, you may encounter certain issues or challenges. Let’s discuss some common problems and how to resolve them.
Dealing with Exceptions
One common issue is dealing with exceptions in constructors. If an exception is thrown during object creation, it can leave your object in an unstable state.
Consider this example:
public class Vehicle {
String color;
public Vehicle(String color) throws Exception {
if (color == null) {
throw new Exception("Color cannot be null");
}
this.color = color;
}
}
try {
Vehicle myCar = new Vehicle(null);
} catch (Exception e) {
e.printStackTrace();
}
// Output:
// java.lang.Exception: Color cannot be null
In this example, our constructor throws an Exception
if the color
parameter is null
. When we try to create a new Vehicle
with a null
color, the Exception
is thrown and caught, and our myCar
object is never created.
Solution: One way to handle this is to provide a default value that will be used if the constructor argument is null
. Another approach is to use the Optional class in Java 8 to avoid null values.
Overloading Pitfalls
Overloading constructors can provide flexibility, but it can also lead to confusion if not done carefully. If two constructors have the same number of parameters of different types, Java may not know which one to use.
Solution: To avoid this, make sure each overloaded constructor has a distinct parameter list. If this isn’t possible, consider using a factory method instead.
Remember, understanding the nuances of constructors in Java is key to utilizing them effectively in your code. The more you work with them, the more comfortable you’ll become.
Understanding the Fundamentals of Java
Before we delve deeper into the nuances of constructors in Java, it’s crucial to understand the fundamental principles of object-oriented programming (OOP) in Java. These principles include classes, objects, and methods, which form the backbone of Java programming.
Classes in Java
In Java, a class is a blueprint from which individual objects are created. A class can contain fields (variables) and methods to describe the behavior of an object.
Here’s a basic example of a class in Java:
public class Vehicle {
String color;
}
In this example, Vehicle
is a class that has one field: color
.
Objects in Java
An object is an instance of a class. It’s a basic unit of OOP and represents the real-life entities. A class creates a new data type that can be used to create objects of that type.
Here’s how you might create an object of the Vehicle
class:
Vehicle myCar = new Vehicle();
In this example, myCar
is an object of the Vehicle
class.
Methods in Java
Methods are where the real work is done. They are where the logic is written, data is manipulated, and all the actions are executed.
Here’s an example of a method in the Vehicle
class:
public class Vehicle {
String color;
void changeColor(String newColor) {
color = newColor;
}
}
Vehicle myCar = new Vehicle();
myCar.changeColor("Blue");
System.out.println(myCar.color);
// Output:
// Blue
In this example, changeColor
is a method that changes the color
of a Vehicle
object.
Understanding these fundamentals will help you grasp the concept of constructors in Java, as constructors are a special type of method used to initialize objects.
Beyond Constructors: Exploring Larger Projects and Design Patterns
While understanding constructors is vital, it’s equally crucial to recognize their role in larger projects and design patterns in Java. Constructors aren’t standalone elements but part of a bigger picture in object-oriented programming.
Constructors in Larger Projects
In larger projects, constructors play a critical role in ensuring object consistency and reducing bugs. By setting initial state with constructors, you can prevent invalid object states that could lead to runtime errors.
Constructors and Design Patterns
In design patterns, constructors are often used to enforce particular behaviors. For instance, in the Singleton pattern, a private constructor is used to ensure only one instance of the class exists.
Exploring Related Concepts
As you continue your journey in Java, consider exploring related concepts such as inheritance and encapsulation. These are fundamental principles of object-oriented programming that work hand-in-hand with constructors to create robust and flexible code.
Further Resources for Mastering Java Constructors
To continue your learning journey, check out these resources:
- Best Practices for Working with Objects in Java – Learn how to create and use objects effectively in Java programming.
Inner Class Concepts in Java – Learn how inner classes can enable tighter coupling and better abstraction.
Instance in Java Overview – Understand the concept of an instance in Java, a unique occurrence of a class in memory.
Oracle’s Java Tutorials are a comprehensive resource for Java, including in-depth articles on constructors.
GeeksforGeeks’ Java Programming Language provides a wealth of Java-related articles and tutorials.
Java Constructors by Baeldung offers a clear, in-depth overview of constructors in Java.
Remember, mastering Java, like any programming language, is a journey. Keep exploring, learning, and coding!
Wrapping Up: Java Constructors
In this comprehensive guide, we’ve delved deep into the world of constructors in Java. From understanding the basic use of constructors to exploring advanced techniques, we’ve covered the entire spectrum of using constructors in Java.
We began with the basics, learning how to create and initialize objects using constructors. We then ventured into more advanced territory, exploring parameterized constructors and constructor overloading. Along the way, we tackled common issues you might face when using constructors, such as dealing with exceptions and overloading pitfalls, providing you with solutions and workarounds for each issue.
We also looked at alternative approaches to object creation and initialization, focusing on the use of factory methods. This gave us a broader perspective on how to handle object creation in Java, beyond just using constructors.
Here’s a quick comparison of the methods we’ve discussed:
Method | Flexibility | Complexity | Use Case |
---|---|---|---|
Constructor | Moderate | Low | Simple object creation |
Parameterized Constructor | High | Moderate | Object creation with varying parameters |
Factory Method | High | High | Complex object creation |
Whether you’re just starting out with Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of constructors in Java and their role in object creation and initialization.
Mastering constructors in Java is a crucial step in becoming a proficient Java programmer. With this knowledge in your arsenal, you’re well equipped to write robust and efficient Java code. Happy coding!