Python Get Current Date | date.today() And More
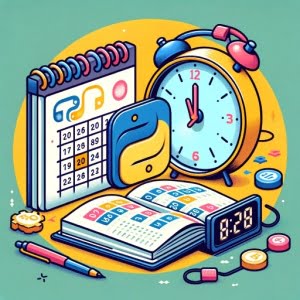
Ever found yourself needing to get the current date in Python but not sure how to go about it? You’re not alone. Many developers find themselves in this situation, but Python’s datetime module is here to help.
Think of Python’s datetime module as a digital calendar – it’s a tool that can provide you with the current date at your fingertips.
This guide will walk you through the process of getting the current date in Python, from the basics to more advanced techniques. We’ll cover everything from using the date.today()
function, formatting the date to your preference, to discussing alternative approaches and troubleshooting common issues.
So, let’s dive in and start mastering how to get the current date in Python!
TL;DR: How Do I Get the Current Date in Python?
To get the current date in Python, you can use the
date.today()
function from thedatetime
module. This function will return the current date in the ‘YYYY-MM-DD’ format.
Here’s a simple example:
from datetime import date
today = date.today()
print(today)
# Output:
# 2022-10-11
In this example, we first import the date
class from the datetime
module. Then, we use the today()
function of the date
class to get the current date. Finally, we print the date, which is displayed in the ‘YYYY-MM-DD’ format.
This is just a basic way to get the current date in Python. There’s much more to learn about handling dates and times in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding Python’s date.today() Function
- Formatting the Date with strftime
- Exploring Alternative Methods: time Module and Third-Party Libraries
- Troubleshooting Common Issues with Python’s Date
- Unpacking Python’s Datetime Module
- Expanding Your Knowledge: Beyond Current Dates
- Wrapping Up: Mastering Python’s Date Handling
Understanding Python’s date.today()
Function
At the heart of getting the current date in Python is the date.today()
function. This function is part of Python’s datetime
module, which provides a range of functionalities to deal with dates, times, and intervals.
How Does date.today()
Work?
The date.today()
function returns the current date in the ‘YYYY-MM-DD’ format. It doesn’t require any arguments, making it straightforward to use. Let’s see it in action:
from datetime import date
today = date.today()
print(today)
# Output:
# 2022-10-11
In this example, we first import the date
class from the datetime
module. Then, we use the today()
method of the date
class, which returns the current date. Finally, we print the date.
Advantages of date.today()
The date.today()
function is simple and easy to use. It doesn’t require any additional parameters or complex setup. This makes it a great choice for beginners or for simple tasks where you just need the current date.
Potential Pitfalls
While the date.today()
function is easy to use, it’s important to remember that it returns the date in the ‘YYYY-MM-DD’ format. If you need the date in a different format, you’ll need to use additional functions to format it. We’ll cover this in the ‘Advanced Use’ section.
Another potential pitfall is that date.today()
returns the current date according to the system’s local timezone. If you need the date in a different timezone, you’ll need to handle this separately. We’ll discuss this more in the ‘Troubleshooting and Considerations’ section.
Formatting the Date with strftime
Once you’ve mastered the basics of getting the current date in Python, you might want to present the date in a different format. Python’s strftime
function allows you to format the date and time in many different ways.
Using strftime
to Format Dates
The strftime
function stands for ‘string format time’. It converts a date to a string and allows you to specify the format. Here’s how you can use it:
from datetime import date
today = date.today()
formatted_date = today.strftime('%B %d, %Y')
print(formatted_date)
# Output:
# October 11, 2022
In this example, we use the strftime
function with the format specifier ‘%B %d, %Y’, which translates to ‘Month Day, Year’. As a result, the date is printed in a more readable format.
Understanding Format Codes
The strftime
function uses format codes to specify the format of the date. Here are some common format codes:
%Y
: Year with century, e.g. ‘2022’%m
: Month as a zero-padded decimal number, e.g. ’01’ for January%B
: Month as locale’s full name, e.g. ‘January’%d
: Day of the month as a zero-padded decimal, e.g. ’01’
You can find the full list of format codes in Python’s official documentation.
Best Practices for Using strftime
When using strftime
, it’s important to use the correct format codes for your needs. Also, keep in mind that strftime
returns a string, so if you need to perform date calculations, you should do them before converting the date to a string.
Exploring Alternative Methods: time
Module and Third-Party Libraries
While the datetime
module is the standard way to get the current date in Python, there are alternative methods you can use. These include using the time
module or third-party libraries. Let’s explore these alternatives.
Using the time
Module
Python’s time
module provides a function called time()
, which returns the current system time in seconds since the epoch (the point where the time starts). Here’s how you can use it to get the current date:
import time
timestamp = time.time()
current_date = time.ctime(timestamp)
print(current_date)
# Output:
# Tue Oct 11 22:14:48 2022
In this example, we first get the current system time in seconds using time()
. Then, we convert this timestamp into a string representing local time using ctime()
. Finally, we print the date and time.
Using Third-Party Libraries
There are also third-party libraries like arrow
that provide additional functionalities for handling dates and times. Here’s an example of how to get the current date using arrow
:
import arrow
current_date = arrow.now().format('YYYY-MM-DD')
print(current_date)
# Output:
# 2022-10-11
In this example, we use arrow
‘s now()
function to get the current date and time, and then format it to ‘YYYY-MM-DD’ using the format()
function.
Comparing the Methods
Method | Advantage | Disadvantage |
---|---|---|
datetime | Standard Python module, easy to use | Limited formatting options |
time | Provides system time in seconds, can be useful in some scenarios | More steps to get the current date |
arrow (third-party) | Provides additional functionalities, flexible formatting options | Requires installing an additional library |
In conclusion, while the datetime
module is the go-to choice for most scenarios, the time
module and third-party libraries like arrow
provide useful alternatives for getting the current date in Python. The best method to use depends on your specific needs and circumstances.
Troubleshooting Common Issues with Python’s Date
As you work with Python to get the current date, you may encounter some common issues. These could range from timezone discrepancies to handling leap years. Let’s explore these problems and their solutions.
Dealing with Timezone Issues
By default, Python’s date.today()
function returns the current date according to the system’s local timezone. If you need the date in a different timezone, you’ll need to handle this separately. Here’s how you can get the current date in a specific timezone using the pytz
library:
from datetime import datetime
import pytz
new_york_tz = pytz.timezone('America/New_York')
new_york_date = datetime.now(new_york_tz)
print(new_york_date.date())
# Output:
# 2022-10-11
In this example, we first import the datetime
and pytz
modules. We then specify the timezone for New York, and get the current date in that timezone.
Handling Leap Years
Python’s date
object handles leap years automatically when you get the current date. However, if you’re manipulating dates, especially when adding or subtracting years, you need to be careful. For instance, adding a year to February 29 will result in an error because the resulting date (February 29 of a non-leap year) does not exist.
To handle this, you can use Python’s date
object’s replace
method with a try-except block. If adding a year results in an error, you can add the year to March 1st instead:
from datetime import date
def add_year(d):
try:
return d.replace(year = d.year + 1)
except ValueError:
return d.replace(month = 3, day = 1, year = d.year + 1)
leap_day = date(2020, 2, 29)
print(add_year(leap_day))
# Output:
# 2021-03-01
In this example, we define a function add_year
that tries to add a year to the given date. If it encounters a ValueError
(which happens when adding a year to February 29 of a leap year), it adds the year to March 1st of the next year instead.
In conclusion, while Python makes it easy to get the current date, you need to be aware of potential issues and how to handle them. With the right knowledge and tools, you can effectively work with dates in Python.
Unpacking Python’s Datetime Module
To truly harness the power of getting the current date in Python, it’s essential to understand the underlying concepts. This includes the datetime module and the date object, as well as concepts like timezones and leap years.
The Datetime Module
In Python, the datetime module is a built-in module that provides classes for manipulating dates and times. The most commonly used classes are:
date
: Represents a date (year, month, day).time
: Represents a time of day.datetime
: Represents a date and time.
The datetime module also provides functions to parse strings representing dates and times and to format dates and times as strings.
The Date Object
The date object represents a date (year, month, and day) in an idealized calendar. The date object is produced by the date.today()
function, which we’ve been discussing. Here’s an example of creating a date object:
from datetime import date
d = date(2022, 10, 11)
print(d)
# Output:
# 2022-10-11
In this example, we create a date object representing October 11, 2022, and then print it.
Understanding Timezones
A timezone is a region of the globe that observes a uniform standard time for legal, commercial, and social purposes. Python’s datetime module uses the system’s local timezone when you get the current date. However, you can specify a different timezone if needed, as we discussed in the ‘Troubleshooting and Considerations’ section.
Dealing with Leap Years
A leap year is a year, occurring once every four years, which has 366 days including 29 February as an intercalary day. Python’s date object handles leap years automatically when you get the current date. However, if you’re manipulating dates, you need to handle leap years separately, as we discussed in the ‘Troubleshooting and Considerations’ section.
In conclusion, understanding these fundamentals will empower you to work effectively with dates in Python. With this knowledge, you’ll be prepared to handle a variety of scenarios and tackle any issues that come your way.
Expanding Your Knowledge: Beyond Current Dates
Mastering the retrieval of the current date in Python is just the beginning. The ability to manipulate and understand dates and times is an essential skill in many areas of programming, including logging, data analysis, and more.
The Role of Dates in Logging
In logging, timestamps are crucial for understanding when events occur. By using Python’s datetime module, you can add timestamps to your logs, making them easier to analyze and troubleshoot.
Data Analysis and Dates
In data analysis, dates and times are often key variables. Whether you’re analyzing sales over time, trends in user behavior, or seasonal patterns, being able to manipulate and analyze dates is crucial.
Exploring Datetime Arithmetic
Python’s datetime module also allows for datetime arithmetic. This means you can add and subtract dates and times, which can be useful for scheduling applications, reminders, and more. Here’s a simple example:
from datetime import date, timedelta
today = date.today()
week_later = today + timedelta(days=7)
print(week_later)
# Output:
# 2022-10-18
In this example, we use the timedelta
class to represent a duration. We then add this duration to the current date to get the date a week later.
Handling Timestamps
A timestamp is a sequence of characters denoting the date and/or time at which a certain event occurred. Python’s datetime module allows you to convert dates and times to timestamps, which can be useful for comparing events, storing dates and times in databases, and more.
Further Resources for Mastering Python Dates and Times
To further deepen your knowledge, here are some additional resources:
- Beginner’s Guide to Python Time Handling – Learn how Python’s time module simplifies tasks like measuring code execution time.
Simplifying Code Profiling with Python Timer – Master Python’s timer module for precise timing of program execution.
Understanding Python’s Datetime Module – Explore Python’s datetime module for versatile date and time operations.
Python’s Official Datetime Documentation – A comprehensive resource with detailed explanations and examples.
Python Date and Time Guide – A practical guide with real-world examples.
Python Timer Functions Explained – Learn how to use timer functions effectively in Python with this guide.
By exploring these resources and practicing your skills, you’ll become a master at handling dates and times in Python.
Wrapping Up: Mastering Python’s Date Handling
In this comprehensive guide, we’ve delved into the world of Python, focusing on how to get the current date. This seemingly simple task opens the door to a deeper understanding of Python’s datetime module and its capabilities.
We began with the basics, learning how to use the date.today()
function to get the current date. We then explored how to format the date to our preference using the strftime
function.
We also tackled common issues you might face, such as timezone discrepancies and handling leap years, providing you with solutions for each scenario.
We didn’t stop there. We ventured into alternative methods to get the current date in Python, such as using the time
module or third-party libraries like arrow
. This gave us a broader perspective on handling dates in Python.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
datetime | Standard Python module, easy to use | Limited formatting options, uses system’s local timezone |
time | Provides system time in seconds, can be useful in some scenarios | More steps to get the current date |
arrow (third-party) | Provides additional functionalities, flexible formatting options | Requires installing an additional library |
Whether you’re just starting out with Python or you’re looking to level up your date handling skills, we hope this guide has given you a deeper understanding of how to get the current date in Python.
Understanding how to work with dates is a fundamental skill in programming, applicable in a variety of scenarios from logging to data analysis. Now, you’re well equipped to handle dates in Python. Happy coding!