Creating Files in Python: Step-by-Step Tutorial
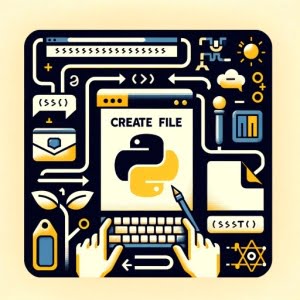
Are you finding it challenging to create a file using Python? You’re not alone. Many developers grapple with this task, but Python, like a skilled craftsman, can easily create and manipulate files.
Python’s file handling capabilities are like a Swiss army knife – versatile and ready for any challenge. Whether you’re dealing with text files, CSV files, or any other file types, Python has got you covered.
This guide will walk you through the process of creating files in Python, from the basic syntax to more advanced techniques. We’ll explore Python’s core file handling functionality, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering file creation in Python!
TL;DR: How Do I Create a File in Python?
To create a file in Python, you can use the
open()
function with the ‘a’ mode such asfile = open('myfile.txt', 'a')
. This function opens a file for writing, creating the file if it does not exist.
Here’s a simple example using the ‘w’ method instead:
file = open('myfile.txt', 'w')
file.close()
# Output:
# Creates a new file named 'myfile.txt' in the current directory
In this example, we’re using the open()
function to create a file named ‘myfile.txt’. The ‘w’ mode tells Python to open the file for writing. If ‘myfile.txt’ does not exist, Python will create it. Note: If the file does exist, Python will overwrite it. Therefore, a safer method is to use the ‘a’ mode (append). After we’re done with the file, we use file.close()
to close it and free up any system resources.
This is a basic way to create a file in Python, but there’s much more to learn about file handling in Python. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Python File Creation: The Basics
Creating a file in Python is as simple as calling the built-in open()
function. The open()
function is a versatile tool in Python’s file handling toolbox, and it’s the first function you’ll likely encounter when dealing with files.
Understanding the open()
Function
The open()
function has a straightforward syntax:
open(file, mode)
The function requires two parameters:
file
: The name (and path, if necessary) of the file you want to open.mode
: A string that indicates what mode you want the file to be opened in. To append to a file, we use ‘a’ (append mode).
Here’s a basic example of appending to a file named ‘example.txt’:
file = open('example.txt', 'a')
file.close()
# Output:
# Opens 'example.txt' in append mode, in the current directory. If the file does not exist, it will be created.
In this modified example, ‘example.txt’ is the file which we want to append to, and ‘a’ is the mode we’re opening the file in. If ‘example.txt’ does not exist, Python will create it. If it does exist, Python will open it and prepare to append data to the end of the file. When we’re finished with the file, we use file.close()
to close it. This helps to release system resources.
Advantages and Pitfalls of the open()
Function
The open()
function is easy to use and understand, making it great for beginners. It’s also incredibly versatile, capable of handling different types of files and modes.
However, one potential pitfall of the open()
function is that it can overwrite existing files without warning when used in ‘w’ mode. Always ensure that the file you’re writing to doesn’t already contain important data, or use ‘a’ mode to append to the file instead of overwriting it.
Advanced File Creation in Python
As you become more comfortable with Python, you’ll discover that its file handling capabilities extend beyond simple text files. Python can create and manipulate a variety of file types, including CSV files, binary files, and more.
Creating Different Types of Files
Let’s look at how to create a CSV file and write some data into it:
import csv
with open('data.csv', 'w', newline='') as file:
writer = csv.writer(file)
writer.writerow(['Name', 'Age'])
writer.writerow(['John Doe', '30'])
writer.writerow(['Jane Doe', '28'])
# Output:
# Creates a new CSV file named 'data.csv' and writes some data into it
In this example, we’re using Python’s csv
module to create a CSV file named ‘data.csv’. We’re then writing some data into it using the writerow()
function. The newline=''
parameter in the open()
function ensures that the data is written correctly on all platforms.
Handling Errors When Creating Files
When dealing with files, it’s important to handle potential errors. For example, if you try to open a file in a directory that doesn’t exist, Python will raise a FileNotFoundError
:
try:
file = open('/nonexistent_directory/myfile.txt', 'w')
except FileNotFoundError as e:
print(f'An error occurred: {e}')
# Output:
# An error occurred: [Errno 2] No such file or directory: '/nonexistent_directory/myfile.txt'
In this example, we’re trying to open a file in a directory that doesn’t exist. Python raises a FileNotFoundError
, which we catch and handle by printing an error message.
Python’s ability to create and manipulate different types of files, along with its robust error handling, makes it a powerful tool for file handling.
Exploring Alternative Approaches in Python
Python provides multiple ways to create files, each with its unique advantages and trade-offs. One such alternative to the open()
function is the os
module.
Using the os
Module to Create Files
The os
module in Python provides a way of using operating system dependent functionality, including creating files. Here’s how you can create a file using the os
module:
import os
try:
os.mknod('newfile.txt')
except FileExistsError as e:
print(f'An error occurred: {e}')
# Output:
# Creates a new file named 'newfile.txt' in the current directory
In this example, we’re using the os.mknod()
function to create a new file. If the file already exists, Python will raise a FileExistsError
, which we catch and handle by printing an error message. This method can be useful if you want to ensure that you don’t accidentally overwrite an existing file.
Weighing the Pros and Cons
The os
module provides more direct access to OS-level operations. It can be more efficient and offer more control than the open()
function. However, it also comes with its drawbacks. The os
module’s functions are lower-level, which means they can be more complex and harder to use correctly. Also, because they interact directly with the OS, they can sometimes behave differently on different systems.
While the open()
function is simpler and more straightforward, the os
module can provide more control and efficiency. Your choice between them will depend on your specific needs and the trade-offs you’re willing to make.
Remember, Python’s strength lies in its versatility. Don’t hesitate to explore different approaches and find the one that suits your needs best.
Troubleshooting Common Issues in Python File Creation
Like any programming task, creating files in Python can sometimes lead to unexpected issues. By understanding these common issues and their solutions, you can save yourself a lot of time and frustration.
Handling ‘FileNotFoundError’
One common issue when creating files in Python is the ‘FileNotFoundError’. This error occurs when Python can’t find the file or directory you’re trying to access. For example, if you’re trying to create a file in a directory that doesn’t exist, Python will raise a ‘FileNotFoundError’:
try:
file = open('/nonexistent_directory/myfile.txt', 'w')
except FileNotFoundError as e:
print(f'An error occurred: {e}')
# Output:
# An error occurred: [Errno 2] No such file or directory: '/nonexistent_directory/myfile.txt'
In this example, we’re trying to open a file in a directory that doesn’t exist. Python raises a FileNotFoundError
, which we catch and handle by printing an error message.
Tips for Avoiding Common Issues
Here are some tips to help you avoid these common issues:
- Always check that the directory you’re trying to create a file in actually exists.
- Be careful when using ‘w’ mode with the
open()
function, as it can overwrite existing files without warning. - Use exception handling to catch and handle any errors that occur when creating files.
By understanding these common issues and how to handle them, you can create files in Python more effectively and efficiently.
Python’s File Handling Capabilities: A Deep Dive
Python’s file handling capabilities go beyond just creating files. It provides a robust framework for reading from files, appending to files, and even deleting files.
Reading from Files in Python
Reading from a file in Python is as simple as opening the file in ‘r’ mode and calling the read()
function:
try:
file = open('myfile.txt', 'r')
content = file.read()
print(content)
file.close()
except FileNotFoundError as e:
print(f'An error occurred: {e}')
# Output:
# Prints the content of 'myfile.txt'
In this example, we’re opening ‘myfile.txt’ in ‘r’ (read) mode and reading its content with the read()
function. If ‘myfile.txt’ doesn’t exist, Python raises a FileNotFoundError
, which we catch and handle.
Appending to Files in Python
Appending to a file in Python involves opening the file in ‘a’ mode and writing to it. This adds the new content to the end of the file, preserving any existing content:
file = open('myfile.txt', 'a')
file.write('
New line of text')
file.close()
# Output:
# Appends a new line of text to 'myfile.txt'
Here, we’re opening ‘myfile.txt’ in ‘a’ (append) mode and writing a new line of text to it. The new text is added to the end of the file, and all existing content is preserved.
Deleting Files in Python
Python can also delete files. This is done using the os.remove()
function:
import os
try:
os.remove('myfile.txt')
except FileNotFoundError as e:
print(f'An error occurred: {e}')
# Output:
# Deletes 'myfile.txt'
In this example, we’re using the os.remove()
function to delete ‘myfile.txt’. If ‘myfile.txt’ doesn’t exist, Python raises a FileNotFoundError
, which we catch and handle.
Python’s file handling capabilities are comprehensive and versatile, making it a powerful tool for any task involving file manipulation.
Expanding Your Python File Handling Skills
Creating files is a fundamental part of Python programming, but it’s just the tip of the iceberg. As you delve deeper into Python, you’ll find that file handling plays a crucial role in larger scripts and projects.
Working with File Paths
Understanding file paths and how to work with them is essential when creating files. Python provides the os
module which has several functions to work with file paths effectively.
import os
# Get the current working directory
print(os.getcwd())
# Output:
# '/home/username'
In this example, the os.getcwd()
function returns the current working directory. This is useful when you need to create files in the same directory as your script.
Checking If a File Exists
Before creating a file, you might want to check if it already exists. Python makes this easy with the os.path
module.
import os
if os.path.isfile('myfile.txt'):
print('File exists')
else:
print('File does not exist')
# Output:
# 'File exists' or 'File does not exist', depending on whether 'myfile.txt' exists
In this example, the os.path.isfile()
function checks if ‘myfile.txt’ exists and returns True
or False
accordingly.
Getting the Size of a File
Python can also get the size of a file, which can be useful in many situations.
import os
size = os.path.getsize('myfile.txt')
print(f'The size of myfile.txt is {size} bytes')
# Output:
# 'The size of myfile.txt is X bytes', where X is the size of 'myfile.txt'
In this example, the os.path.getsize()
function returns the size of ‘myfile.txt’ in bytes.
Further Resources for Python File Handling Mastery
To further your understanding of Python’s file handling capabilities, check out the following resources:
- Python OS Module – A quick overview on interacting with the operating system in Python and the “os” module.
Resource Management with Python’s “with” – Explore the world of context managers and automatic resource cleanup.
File Path Handling Simplified: Python’s os.path – Discover the usage of “os.path” for handling paths and directories.
Python’s official file handling tutorial provides guidelines on reading and writing files in Python.
Real Python’s guide to reading and writing files in Python offers practical examples and tips for file I/O operations.
Python For Beginners’ tutorial on file handling gives an easy-to-understand introduction to working with files in Python.
These resources provide in-depth tutorials and examples that can help you master Python’s file handling capabilities.
Wrapping Up: Mastering File Creation in Python
In this comprehensive guide, we’ve delved into the world of Python, exploring how to create files using this versatile programming language. From the basic syntax to advanced techniques, we’ve covered every aspect of file creation in Python, providing you with the knowledge and tools to handle any file-related task.
We began with the basics, learning how to create a file using the open()
function. We then ventured into more advanced territory, exploring how to create different types of files, handle errors, and use alternative methods like the os
module.
Along the way, we tackled common issues you might encounter when creating files in Python, such as ‘FileNotFoundError’, and provided solutions to help you overcome these challenges.
We also compared the open()
function with the os
module, giving you a sense of the broader landscape of file creation methods in Python. Here’s a quick comparison of these methods:
Method | Simplicity | Versatility | Error Handling |
---|---|---|---|
open() function | High | Moderate | Requires explicit handling |
os module | Moderate | High | Raises specific errors |
Whether you’re just starting out with Python or you’re looking to level up your file handling skills, we hope this guide has given you a deeper understanding of file creation in Python.
With its balance of simplicity, versatility, and robust error handling, Python is a powerful tool for file creation. Happy coding!