Java Generics: Basics of Generic Programming
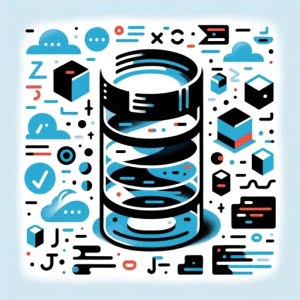
Are you finding it challenging to understand and use Java Generics? You’re not alone. Many developers find themselves grappling with the concept of generics in Java, but there’s a tool that can make this process more manageable.
Think of Java Generics as a Swiss Army knife – providing flexibility and type safety in your code. These features can help you avoid runtime errors, making your code more robust and maintainable.
This guide will walk you through the basics to the advanced usage of Java Generics. We’ll explore the core functionality of generics, delve into its advanced features, and even discuss common issues and their solutions.
So, let’s dive in and start mastering Java Generics!
TL;DR: What are Java Generics?
Java Generics are a feature in Java that allows type parameters when defining classes, interfaces, and methods. They are delimited by angle brackets (<>) before the class name, such as:
public class Box<T> {}
. They provide stronger type checks at compile time, enhancing code robustness and readability.
Here’s a simple example:
List<String> list = new ArrayList<String>();
list.add("Hello");
System.out.println(list.get(0));
# Output:
# 'Hello'
In this example, we’ve created a list that only accepts String objects. The List
notation is a generic type, indicating that this list will only hold strings. We then add a string to the list and print it out. This ensures type safety, as you can’t accidentally insert a non-string object into this list.
This is just a basic way to use Java Generics, but there’s much more to learn about them. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Java Generics: Basic Usage
Java Generics is a powerful feature that enhances your code’s flexibility and type safety. At the basic level, you can use generics in classes, interfaces, and methods. Let’s illustrate this with an example:
public class Box<T> {
private T t;
public void set(T t) {
this.t = t;
}
public T get() {
return t;
}
}
Box<Integer> integerBox = new Box<Integer>();
integerBox.set(new Integer(10));
Integer myInteger = integerBox.get();
System.out.println(myInteger);
# Output:
# 10
In this example, T
is a type parameter that will be replaced by a real type when an object of Box
class is created. We created a Box
of Integer
type and used it to store an Integer. When we retrieved the data, we didn’t need to cast it because the type was already known.
The benefits of using Java Generics include type safety and eliminating the need for typecasting. However, one potential pitfall is that you can’t use primitives like int
or char
as type parameters; you must use their wrapper classes like Integer
or Character
instead.
Java Generics: Advanced Usage
As you delve deeper into Java Generics, you’ll encounter more complex features such as bounded type parameters and wildcards. These features allow you to impose certain restrictions on the types that can be used as type parameters.
Bounded Type Parameters
Bounded type parameters are used when you want to limit the types that can be passed to a type parameter. For instance, you may want to ensure that the type parameter is a subclass of a certain class or implements a particular interface.
Let’s look at an example:
public class Box<T extends Number> {
private T t;
public void set(T t) {
this.t = t;
}
public T get() {
return t;
}
}
Box<Integer> integerBox = new Box<>();
integerBox.set(new Integer(10));
Integer myInteger = integerBox.get();
System.out.println(myInteger);
# Output:
# 10
In this example, T extends Number
means that the type parameter T
can replace any subclass of Number
class. Therefore, you can create a Box
of Integer
or Double
but not a Box
of String
because String
is not a subclass of Number
.
Wildcards
Wildcards are used to refer to an unknown type. The wildcard can be used as the type of a parameter, field, or local variable and sometimes as a return type. Let’s illustrate this with an example:
public void printList(List<?> list) {
for (Object item : list) {
System.out.println(item);
}
}
List<Integer> integerList = Arrays.asList(1, 2, 3);
List<String> stringList = Arrays.asList("one", "two", "three");
printList(integerList);
printList(stringList);
# Output:
# 1
# 2
# 3
# one
# two
# three
In this example, List
means a list of unknown type. Therefore, the printList
method can accept a list of any type.
The benefits of using bounded type parameters and wildcards include increased flexibility and type safety. However, they can also make your code more complex and harder to read, so use them judiciously.
Exploring Alternative Approaches: Raw Types
While Java Generics provide a robust way to ensure type safety, it’s crucial to know about alternatives. One such option is the use of raw types. A raw type is a generic class or interface name without any type arguments.
Let’s take a look at an example:
Box rawBox = new Box();
rawBox.set("Hello");
String myString = (String) rawBox.get();
System.out.println(myString);
# Output:
# 'Hello'
In this example, Box
is a raw type. You can store any type of object in rawBox
, but when you retrieve the data, you need to cast it to the correct type. This lack of type safety can lead to ClassCastException
at runtime if you make a mistake with the casting.
The benefit of using raw types is that they are compatible with older Java code that doesn’t use generics. However, the drawbacks include the lack of type safety and the need for type casting. Therefore, it’s recommended to use raw types only when necessary, such as when you’re dealing with legacy code that doesn’t use generics.
When deciding whether to use generics or raw types, consider the following factors:
- Type Safety: Generics provide compile-time type safety, while raw types do not.
- Code Readability: Generics make your code more readable by making the type of objects clear, while raw types do not.
- Legacy Code Compatibility: Raw types are compatible with legacy code that doesn’t use generics, while generics are not.
In conclusion, while raw types can be useful in certain situations, Java Generics are generally a more robust and safer choice for new code.
Troubleshooting Java Generics: Common Issues and Solutions
While Java Generics are powerful, developers often encounter certain issues. Two common problems are type erasure and unchecked warnings. Understanding these issues will help you write better and more robust code.
Type Erasure
Type erasure is a process by which the Java compiler removes all type parameters and replaces them with their bounds or with Object if the type parameters are unbounded. This can lead to unexpected behavior.
Let’s look at an example:
public class Box<T> {
private T t;
public void set(T t) {
this.t = t;
}
public T get() {
return t;
}
}
Box<Integer> integerBox = new Box<>();
integerBox.set(new Integer(10));
Box<String> stringBox = new Box<>();
stringBox.set("Hello");
System.out.println(integerBox.getClass().equals(stringBox.getClass()));
# Output:
# true
In this example, despite integerBox
and stringBox
being of different parameterized types, the output is true
. This is because of type erasure. The Java compiler removes the type parameters, so at runtime, both integerBox
and stringBox
are just instances of Box
.
Unchecked Warnings
Unchecked warnings are issued when the compiler encounters code that is not type-safe, but it cannot prove it due to type erasure.
Let’s illustrate this with an example:
List rawList = new ArrayList();
List<String> list = rawList; // unchecked warning
# Output:
# Note: Test.java uses unchecked or unsafe operations.
# Note: Recompile with -Xlint:unchecked for details.
In this example, we’re assigning a raw type List
to a parameterized type List
. This is not type-safe because rawList
can hold any type of objects, not just strings. However, the compiler can’t prove this is not type-safe due to type erasure, so it issues an unchecked warning.
To avoid these issues, follow these best practices:
- Always use parameterized types instead of raw types to ensure type safety.
- Be aware of type erasure when dealing with generic types at runtime.
- Pay attention to unchecked warnings and try to fix them to prevent potential runtime errors.
The Fundamentals of Java Generics
Java Generics are a fundamental feature of the language, crucial for writing robust and maintainable code. But why do we need them? And how do they improve type safety and maintainability?
The Need for Java Generics
Before the introduction of generics in Java 5, you could store any type of object in collections like List
and Map
. This lack of type restrictions could lead to bugs. For example, if you have a List
intended to store strings, but accidentally store an integer, the compiler won’t catch this mistake. The error will occur at runtime when you try to retrieve the integer as a string, resulting in a ClassCastException
.
Java Generics were introduced to provide compile-time type checking and eliminate the risk of ClassCastException
that was common while working with collection classes. This feature enables programmers to ensure that they are using the right type of objects.
Improving Type Safety and Maintainability
Java Generics improve type safety by enforcing compile-time type checks. This helps in identifying and fixing errors early in the development cycle, rather than at runtime.
Generics also enhance code maintainability. They provide a clear way of indicating the type of object stored in a collection, making the code easier to read and understand.
List<String> names = new ArrayList<String>();
names.add("John");
String name = names.get(0);
System.out.println(name);
# Output:
# 'John'
In this example, List
makes it clear that names
is a list of strings. This clarity improves code readability and maintainability.
Related Concepts: Polymorphism and Type Casting
Java Generics are closely related to the concepts of polymorphism and type casting. Polymorphism is a feature that allows one interface to be used for a general class of actions. This ties in with generics when you want to allow your generic class to accept any subclass of a particular superclass.
Type casting, on the other hand, is the process of converting a data type into another. Before generics, you had to manually cast the object retrieved from a collection. With generics, this casting is done automatically, reducing the risk of ClassCastException
.
By understanding these fundamental concepts, you can use Java Generics effectively to write robust and maintainable code.
Java Generics: Their Impact and Relevance
Java Generics are not just a standalone feature, but they play a significant role in the design of popular Java libraries and frameworks. This is due to their ability to provide type safety and code reusability.
Java Generics in Libraries and Frameworks
Many Java libraries and frameworks, such as Java Collections Framework (JCF), Java Streams API, and Spring Framework, heavily use generics to provide a flexible and type-safe API. For instance, JCF uses generics to ensure that you can only add the correct type of objects to a collection, eliminating the risk of ClassCastException
.
Exploring Related Topics
If you’re interested in delving deeper into Java Generics, you may want to explore related topics like the Java Collections Framework and the Java Streams API. The Java Collections Framework uses generics to provide type-safe collections, while the Java Streams API uses generics to provide a flexible and powerful functional programming API.
Further Resources for Mastering Java Generics
Generics are only a small part in Java Programming, and their use is improved with knowledge of other Java Fundamentals. We recommend looking into Java Data Structures in order to fully utilize generics. For a full guide on Java Data Structures, Click Here!
To further your understanding of Java Generics, consider checking out these resources:
- Exploring Java Dictionary – Master using Dictionary for associative array functionality in Java programs.
Deque in Java Overview – Discover Deque in Java, offering functionality for element insertion and removal.
Oracle’s Java Generics Tutorial provides an in-depth explanation of Java Generics, from the basics to advanced topics.
Java Generics and Collections covers all aspects of Java Generics and also delves into the Java Collections Framework.
Java Generics FAQs answers all your questions about Java Generics.
By understanding the relevance of Java Generics and exploring related topics, you can become a more proficient and versatile Java developer.
Wrapping Up: Java Generics
In this comprehensive guide, we’ve demystified the concept of Java Generics, a powerful feature in Java that provides flexibility and type safety in your code.
We began with the basics, understanding how to use Java Generics in classes, interfaces, and methods. We then delved into more advanced usage, such as bounded type parameters and wildcards. We also explored alternative approaches, discussing the use of raw types and their implications.
Along the journey, we tackled common issues one may encounter when using Java Generics, such as type erasure and unchecked warnings, and provided solutions to help you overcome these challenges.
Here’s a quick comparison of the methods we’ve discussed:
Method | Type Safety | Code Complexity | Compatibility |
---|---|---|---|
Java Generics | High | Moderate | High |
Raw Types | Low | Low | High |
Whether you’re a beginner just starting out with Java Generics or an experienced developer looking for a refresher, we hope this guide has provided you with a deeper understanding and practical knowledge of Java Generics.
The ability to use generics effectively is a powerful tool in a Java developer’s toolbox, enhancing code readability, maintainability, and robustness. Now, you’re well equipped to enjoy these benefits. Happy coding!