Java Date Class: A Complete Usage Guide
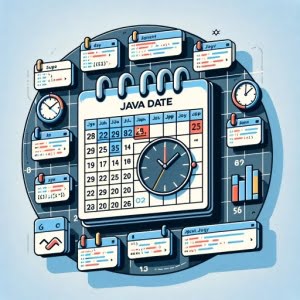
Ever felt like you’re wrestling with dates in Java? You’re not alone. Many developers find Java’s date handling a bit daunting. Think of Java’s date classes as a calendar – a calendar that allows you to manipulate and format dates in various ways.
Java provides powerful tools to work with dates, making it extremely popular for scheduling tasks, formatting dates for display in a user interface, or even for basic date manipulation.
In this guide, we’ll walk you through the process of using dates in Java, from the basics to more advanced techniques. We’ll cover everything from creating simple Date objects, comparing dates, adding or subtracting days, to converting between Date and other classes like LocalDate, and even troubleshooting common issues.
Let’s kick things off and learn to master dates in Java!
TL;DR: How Do I Use Dates in Java?
Java provides the Date class for handling dates. You can create a Date object using the following syntax:
Date date = new Date();
This creates a new Date object representing the current date and time.
Here’s a simple example:
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
System.out.println("Current time in milliseconds = " + date.getTime());
}
}
# Output:
# Current time in milliseconds = 1639809040512
In this example, we create a new instance of the Date
class which is initialized with the current date and time. The getTime()
method is then utilized to print the current time in milliseconds since January 1, 1970, 00:00:00 GMT.
This is just a basic way to use the Date class in Java, but there’s much more to learn about handling dates in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Harnessing the Power of Java Date Class
Java’s Date class, part of the java.util package, is one of the most fundamental classes you’ll encounter when dealing with dates. It encapsulates the current date and time.
Creating Date Objects
Creating a Date object in Java is straightforward. You can create a new Date object representing the current date and time using the default constructor, like so:
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
System.out.println(date);
}
}
# Output:
# Wed Dec 15 10:18:11 PST 2021
In this snippet, we first import the java.util.Date class. Then, within the main method, we create a new Date object named date
. The new Date()
call invokes the Date class’s default constructor, which initializes date
with the current date and time. The System.out.println(date);
line then prints this date and time.
Understanding the Output
The output of this code is the current date and time, formatted as: Day Mon DD HH:MM:SS Z YYYY
, where:
Day
is the day of the week (Sun, Mon, Tue, etc.)Mon
is the month (Jan, Feb, Mar, etc.)DD
is the day of the month (01 through 31)HH:MM:SS
is the time in 24-hour formatZ
is the time zone (PST, EST, etc.)YYYY
is the four-digit year
So, the output Wed Dec 15 10:18:11 PST 2021
represents Wednesday, December 15, 2021 at 10:18:11 in the PST time zone.
This is the most basic use of the Date class in Java. However, the Date class offers much more functionality for manipulating and formatting dates, which we’ll explore in the next sections.
Going Beyond Basics with Java Date
Once you’re comfortable with creating Date objects and displaying them, you can start exploring more complex operations. These include comparing dates, adding or subtracting days, and even converting between Date and other classes like LocalDate.
Comparing Dates in Java
Java provides several ways to compare Date objects. One of the most common methods is to use the compareTo()
function, which returns a negative integer, zero, or a positive integer depending on whether the Date object is earlier than, equal to, or later than the other Date.
Here’s an example:
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date1 = new Date();
Date date2 = new Date();
// Sleep for 1 second
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
date2.setTime(System.currentTimeMillis());
System.out.println("Date1: " + date1);
System.out.println("Date2: " + date2);
System.out.println("Comparison: " + date1.compareTo(date2));
}
}
# Output:
# Date1: Wed Dec 15 10:18:11 PST 2021
# Date2: Wed Dec 15 10:18:12 PST 2021
# Comparison: -1
In this code, we first create two Date objects, date1
and date2
, both representing the current time. We then sleep for 1 second and set the time of date2
to the current time again. This means that date2
is now 1 second later than date1
. When we compare date1
and date2
using compareTo()
, it returns -1, indicating that date1
is earlier than date2
.
Adding and Subtracting Days
To add or subtract days to a Date object, we need to convert it to a Calendar object first. Let’s add 5 days to the current date:
import java.util.Calendar;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
Calendar calendar = Calendar.getInstance();
calendar.setTime(date);
calendar.add(Calendar.DATE, 5); // Add 5 days
date = calendar.getTime();
System.out.println("New date: " + date);
}
}
# Output:
# New date: Mon Dec 20 10:18:11 PST 2021
In this code, we first create a Date object representing the current date and a Calendar object. We set the Calendar’s time to the current date using setTime()
, then add 5 days using add()
. Finally, we get the new date from the Calendar using getTime()
and print it.
Converting Between Date and LocalDate
Java 8 introduced the LocalDate class, which is often a better choice for handling dates without times. Here’s how to convert a Date to a LocalDate:
import java.time.LocalDate;
import java.time.ZoneId;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
LocalDate localDate = date.toInstant().atZone(ZoneId.systemDefault()).toLocalDate();
System.out.println("LocalDate: " + localDate);
}
}
# Output:
# LocalDate: 2021-12-15
In this code, we first create a Date object. We then convert it to a LocalDate by calling toInstant()
to get an Instant object, atZone()
to convert the Instant to a ZonedDateTime, and finally toLocalDate()
to get a LocalDate. The result is the current date in the format YYYY-MM-DD
.
These advanced techniques allow you to perform a wide range of operations with dates in Java, making the Date class a powerful tool in your Java arsenal.
Exploring Alternatives: Calendar and LocalDate Classes
While the Date class is a powerful tool for handling dates in Java, it’s not the only one. The Calendar and LocalDate classes offer alternative approaches, each with its own pros and cons.
The Power of the Calendar Class
The Calendar class, also part of the java.util package, provides methods for manipulating dates based on the calendar field, such as getting the date of the next week.
Here’s an example of using the Calendar class to get the date of the next week:
import java.util.Calendar;
public class Main {
public static void main(String[] args) {
Calendar calendar = Calendar.getInstance();
calendar.add(Calendar.WEEK_OF_YEAR, 1);
System.out.println("Next week: " + calendar.getTime());
}
}
# Output:
# Next week: Wed Dec 22 10:18:11 PST 2021
In this code, we create a Calendar object representing the current date and time. We then add 1 week to it using the add()
method and print the new date.
The Simplicity of the LocalDate Class
Java 8 introduced the LocalDate class, part of the java.time package. Unlike Date and Calendar, LocalDate represents a date without a time or time zone, which makes it a better choice for dates where the time isn’t important.
Here’s how to get today’s date and the date of the next week using LocalDate:
import java.time.LocalDate;
public class Main {
public static void main(String[] args) {
LocalDate today = LocalDate.now();
LocalDate nextWeek = today.plusWeeks(1);
System.out.println("Today: " + today);
System.out.println("Next week: " + nextWeek);
}
}
# Output:
# Today: 2021-12-15
# Next week: 2021-12-22
In this code, we first get today’s date using LocalDate.now()
. We then add 1 week to it using plusWeeks()
and print both dates.
Comparing Date, Calendar, and LocalDate
While all three classes can be used to handle dates in Java, they each have their strengths and weaknesses:
- The Date class is simple and straightforward, but lacks many features and is largely deprecated.
- The Calendar class is more powerful and flexible than Date, but its API is complex and not very intuitive.
- The LocalDate class has a simple and intuitive API and is a better choice for dates without times, but it’s only available in Java 8 and later.
Choosing between these classes depends on your specific needs and the version of Java you’re using.
Working with dates in Java can sometimes present challenges. Two of the most common issues developers face are timezone discrepancies and date formatting problems. Let’s discuss these issues and explore some best practices to solve them.
Timezone Troubles
Java’s Date class represents a specific instant in time, with millisecond precision. However, it does not contain any timezone information. This can lead to confusion when you’re dealing with dates across different timezones.
One solution to this problem is to use the java.util.TimeZone class or the java.time.ZonedDateTime class, available since Java 8.
Here’s an example of how to get the current date and time in a specific timezone using ZonedDateTime:
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class Main {
public static void main(String[] args) {
ZonedDateTime zonedDateTime = ZonedDateTime.now(ZoneId.of("America/Los_Angeles"));
System.out.println("Los Angeles time: " + zonedDateTime);
}
}
# Output:
# Los Angeles time: 2021-12-15T10:18:11.123456-08:00[America/Los_Angeles]
In this code, we first get the current date and time in the America/Los_Angeles timezone using ZonedDateTime.now()
. We then print this date and time. The output includes the date, time, offset from UTC, and timezone ID.
Date Formatting Problems
Another common issue is formatting dates in a specific format. Java provides the java.text.SimpleDateFormat class for formatting and parsing dates in a locale-sensitive manner.
Here’s how to format a Date object in the format YYYY-MM-DD
:
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = formatter.format(date);
System.out.println("Formatted date: " + formattedDate);
}
}
# Output:
# Formatted date: 2021-12-15
In this code, we first create a Date object representing the current date. We then create a SimpleDateFormat object with the desired format, and format the date using format()
. The result is the current date in the format YYYY-MM-DD
.
By understanding these common issues and their solutions, you can handle dates in Java more effectively and avoid potential pitfalls.
Understanding Java Date Fundamentals
To fully grasp how to work with dates in Java, we need to delve into the fundamentals of how dates are represented and the underlying concepts of the Date, Calendar, and LocalDate classes.
Date Representation in Java
In Java, a date is represented as a point in time since the ‘epoch’ – 00:00:00 Coordinated Universal Time (UTC), January 1, 1970. This is stored as a long integer representing the number of milliseconds since the epoch, not including leap seconds. This method of representation allows for precise date and time tracking, down to the millisecond.
Here’s an example of getting the current time in milliseconds since the epoch:
public class Main {
public static void main(String[] args) {
long millis = System.currentTimeMillis();
System.out.println("Milliseconds since epoch: " + millis);
}
}
# Output:
# Milliseconds since epoch: 1639594091000
This code uses the System.currentTimeMillis()
method to get the current time in milliseconds since the epoch and prints it.
Core Concepts: Date, Calendar, and LocalDate
Java provides several classes for handling dates:
- Date: Part of the java.util package, the Date class represents a specific instant in time, with millisecond precision. However, it’s largely deprecated in favor of Calendar and LocalDate due to various design issues.
Calendar: Also part of the java.util package, the Calendar class provides methods for manipulating dates based on the calendar field, such as getting the date of the next week. However, its API is complex and not very intuitive.
LocalDate: Introduced in Java 8, the LocalDate class is part of the java.time package. Unlike Date and Calendar, LocalDate represents a date without a time or time zone, which makes it a better choice for dates where the time isn’t important.
Understanding these fundamentals is key to effectively working with dates in Java. With this knowledge, you can choose the right class for your needs and understand how Java represents and manipulates dates.
Real-World Applications of Java Date Handling
Java’s date handling capabilities are not just for academic exercises. They are used extensively in real-world applications, from scheduling tasks to formatting dates for display in user interfaces.
Scheduling Tasks with Java Date
Scheduling tasks is a common requirement in many Java applications. For example, you might want to send a daily email to your users, or run a cleanup job every night. Java’s Timer and TimerTask classes, combined with the Date class, make it easy to schedule tasks.
Here’s an example of scheduling a task to run after a specific delay:
import java.util.Date;
import java.util.Timer;
import java.util.TimerTask;
public class Main {
public static void main(String[] args) {
Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
System.out.println("Task executed at: " + new Date());
}
};
timer.schedule(task, 5000); // Execute after 5 seconds
}
}
# Output:
# Task executed at: Wed Dec 15 10:23:16 PST 2021
In this code, we create a Timer object and a TimerTask that prints the current date and time. We then schedule the task to run after a delay of 5000 milliseconds (5 seconds) using the timer.schedule()
method.
Formatting Dates for Display
When displaying dates in a user interface, it’s often necessary to format them in a specific way. Java’s SimpleDateFormat class allows you to format Date objects into strings in any format you like.
Here’s an example of formatting a Date object in the format YYYY-MM-DD
:
import java.text.SimpleDateFormat;
import java.util.Date;
public class Main {
public static void main(String[] args) {
Date date = new Date();
SimpleDateFormat formatter = new SimpleDateFormat("yyyy-MM-dd");
String formattedDate = formatter.format(date);
System.out.println("Formatted date: " + formattedDate);
}
}
# Output:
# Formatted date: 2021-12-15
In this code, we first create a Date object representing the current date. We then create a SimpleDateFormat object with the desired format, and format the date using format()
. The result is the current date in the format YYYY-MM-DD
.
Further Resources for Mastering Java Date Handling
To deepen your understanding of date handling in Java, consider exploring the following resources:
- Exploring Java Class Basics – Learn how to define, create, and utilize classes in Java for modular and organized code.
Java LocalDate Overview – Discover LocalDate in Java, a class for representing date without time zone information.
Java Date and Time API Guide provides a comprehensive overview of the new date and time API introduced in Java 8
Working with Dates in Java Tutorial by vogella provides a detailed explanation of working with dates and times in Java.
Java Date and Calendar Examples Page by Mkyong provides examples of how to get the current date, convert a string to a date, and compare dates in Java.
Wrapping Up: Mastering Java Date Handling
In this comprehensive guide, we’ve delved into the depths of handling dates in Java, exploring everything from basic usage to advanced techniques.
We began with the basics, learning how to create and use Date objects in Java. We then progressed to more advanced topics, such as comparing dates, adding or subtracting days, and converting between Date and other classes like LocalDate. Along the way, we explored alternative approaches to handling dates, introducing the Calendar and LocalDate classes as powerful alternatives to the Date class.
Throughout our journey, we tackled common challenges that developers often encounter when working with dates in Java, such as timezone issues and date formatting problems. We provided solutions and best practices for each issue, equipping you with the knowledge to handle dates in Java effectively.
We also compared the Date, Calendar, and LocalDate classes, highlighting the strengths and weaknesses of each approach:
Class | Pros | Cons |
---|---|---|
Date | Simple and straightforward | Lacks many features, largely deprecated |
Calendar | Powerful and flexible | Complex API, not very intuitive |
LocalDate | Simple and intuitive API, better choice for dates without times | Only available in Java 8 and later |
Whether you’re just starting out with Java date handling or you’re looking to level up your skills, we hope this guide has provided you with a deeper understanding of how to manipulate and format dates in Java.
With its robust date handling capabilities, Java is a powerful tool for any developer’s arsenal. Now, you’re well equipped to handle any date-related challenges that come your way. Happy coding!