Understanding ‘assert’ Keyword in Java: A Detailed Guide
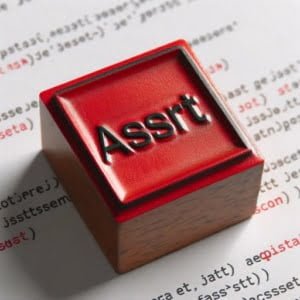
Are you finding assertions in Java puzzling? You’re not alone. Many developers find themselves in a maze when it comes to handling assertions in Java, but we’re here to help.
Think of Java’s assertions as a safety net in a trapeze act – they can catch unexpected behavior before it causes serious problems, providing a versatile and handy tool for various tasks.
This guide will walk you through the ins and outs of using assertions in Java, from the basics to advanced techniques. We’ll cover everything from the basic use of ‘assert’, its advantages and potential pitfalls, to more complex uses and even alternative approaches.
Let’s get started and master assertions in Java!
TL;DR: What is ‘assert’ in Java and how to use it?
assert
is a keyword in Java used for debugging. It enables you to test your assumptions about your program, used with the syntax:assert [variable with logic to test] : [output if test is failed]
. You can use it to verify if a certain condition holds true at a specific point in your code.
Here’s a simple example:
int x = 10;
assert x > 0 : 'x is negative';
# Output:
# (No output, as the assertion is true)
In this example, we’re using the ‘assert’ keyword to test the assumption that ‘x’ is greater than 0. If ‘x’ were not greater than 0, the program would throw an AssertionError with the message ‘x is negative’.
This is just a basic use of ‘assert’ in Java, but there’s much more to learn about using assertions for effective debugging. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
Basic Use of ‘assert’ in Java
The ‘assert’ keyword in Java is a powerful tool for debugging. It allows you to test your assumptions about your program, and can help you catch errors before they become problems.
Here’s a simple example of how you might use ‘assert’ in your code:
int x = 10;
assert x > 0 : 'x is negative';
# Output:
# (No output, as the assertion is true)
In this example, ‘assert’ tests the assumption that ‘x’ is greater than 0. If ‘x’ were not greater than 0, the program would throw an AssertionError with the message ‘x is negative’.
Understanding Assertions in Java
An assertion is a statement in Java that enables you to test your assumptions about your program. Each assertion contains a boolean expression that you believe will be true when the assertion executes. If it is not true, the system will throw an AssertionError.
Advantages of Using ‘assert’ in Java
- Detecting Bugs Early: Assertions can help you catch bugs early in the development cycle, making them easier to fix.
Simplifying Debugging: Assertions can make your code easier to debug, as they allow you to quickly isolate the conditions under which a bug occurs.
Improving Code Quality: Assertions can help improve the quality of your code by documenting your assumptions and making the code easier to understand.
Pitfalls of Using ‘assert’ in Java
While assertions can be extremely useful, there are a few potential pitfalls to be aware of:
- Assertions can be disabled: Assertions are disabled by default in Java. This means that if you’re relying on assertions to check the validity of your program, you may be in for a surprise when you run your code with assertions disabled.
Assertions should not change the state of a program: The assertion check should not have any side effects. It should not change the state of the program. If it does, it may lead to unexpected results when assertions are disabled.
Assertions should not be used for argument checking in public methods: Assertions are typically used for internal consistency checks. They are not intended to replace argument checking in public methods, as they can be disabled.
Advanced Usage of ‘assert’ in Java
As you become more comfortable with using ‘assert’ in Java, you can begin to explore more complex uses of this keyword. One such use is incorporating ‘assert’ with complex expressions.
Using ‘assert’ with Complex Expressions
Let’s consider an example where you want to ensure that a collection doesn’t contain null values. Here’s how you might use ‘assert’ in this scenario:
import java.util.Arrays;
import java.util.List;
List<String> names = Arrays.asList('Alice', 'Bob', null, 'Charlie');
for(String name : names) {
assert name != null : 'null value in names';
}
# Output:
# Exception in thread 'main' java.lang.AssertionError: null value in names
In this example, we’re iterating over a list of names and using ‘assert’ to check that each name is not null. If a null value is found, an AssertionError is thrown with the message ‘null value in names’.
Pros and Cons of Complex Assertions
While using ‘assert’ with complex expressions can be powerful, it’s important to understand the pros and cons.
Pros:
- Flexibility: Complex assertions provide you with the flexibility to validate more intricate conditions in your code.
Comprehensive Checks: They allow for more comprehensive checks, ensuring the robustness of your code.
Cons:
- Increased Complexity: The use of complex expressions can make assertions harder to read and understand, which might slow down the debugging process.
Performance Impact: Complex assertions could have a performance impact on your code, especially if the expressions are computationally expensive.
Remember, the key to effective use of assertions, whether simple or complex, is a balance. Use them where they make sense, and always keep readability and performance in mind.
Exploring Alternative Debugging Methods in Java
While ‘assert’ is a powerful tool for debugging in Java, it’s not the only one. There are other methods such as logging and exception handling that can also be effective in identifying and resolving bugs in your code. Let’s explore these alternatives.
Debugging with Logging
Logging is a simple yet effective way to track the execution of your code. It allows you to record information about what your program does, making it easier to identify where things might be going wrong.
Here’s an example of how you might use logging for debugging:
import java.util.logging.Logger;
public class DebugWithLogging {
private static final Logger LOGGER = Logger.getLogger(DebugWithLogging.class.getName());
public static void main(String[] args) {
LOGGER.info('Entering application.');
try {
int result = divide(10, 0);
} catch (ArithmeticException e) {
LOGGER.severe('An error occurred: ' + e.getMessage());
}
LOGGER.info('Exiting application.');
}
private static int divide(int a, int b) {
return a / b;
}
}
# Output:
# INFO: Entering application.
# SEVERE: An error occurred: / by zero
# INFO: Exiting application.
In this example, we’re using a logger to record when we enter and exit the application, as well as any severe errors that occur.
Debugging with Exception Handling
Exception handling in Java can also serve as a powerful debugging tool. It allows you to catch and handle errors in your code, preventing the program from crashing and allowing you to take corrective action.
Here’s an example of how you might use exception handling for debugging:
public class DebugWithExceptions {
public static void main(String[] args) {
try {
int result = divide(10, 0);
} catch (ArithmeticException e) {
e.printStackTrace();
}
}
private static int divide(int a, int b) {
return a / b;
}
}
# Output:
# java.lang.ArithmeticException: / by zero
# at DebugWithExceptions.divide(DebugWithExceptions.java:12)
# at DebugWithExceptions.main(DebugWithExceptions.java:6)
In this example, we’re using a try-catch block to handle an ArithmeticException that occurs when we try to divide by zero.
Pros and Cons of Alternative Debugging Methods
Pros:
- More Information: Both logging and exception handling can provide more information about the state of your program than assertions alone.
Better Control: Exception handling gives you the ability to catch and handle errors, preventing your program from crashing.
Cons:
- Increased Complexity: Both methods can increase the complexity of your code, making it harder to read and maintain.
Performance Impact: Logging can slow down your program, especially if it’s done excessively.
While ‘assert’ is a valuable tool for debugging, it’s important to be aware of these alternative methods. Depending on the situation, logging or exception handling may be a more effective choice.
Troubleshooting Common Issues with Assertions in Java
Like with any tool, using assertions in Java can sometimes lead to issues. Let’s discuss some common problems you might encounter, such as AssertionErrors, and how to handle them.
Understanding AssertionErrors
An AssertionError is thrown when an ‘assert’ statement fails. This typically happens when the boolean condition within the ‘assert’ statement evaluates to false.
For example:
int x = -10;
assert x > 0 : 'x is negative';
# Output:
# Exception in thread 'main' java.lang.AssertionError: x is negative
In this case, because ‘x’ is not greater than 0, an AssertionError is thrown with the message ‘x is negative’.
How to Handle AssertionErrors
When an AssertionError is thrown, it typically means there’s a bug in your code that needs to be fixed. The best way to handle an AssertionError is to understand why it was thrown and correct the issue.
Tips and Best Practices
- Use descriptive error messages: The more descriptive your error message, the easier it will be to understand why the assertion failed.
Don’t ignore AssertionErrors: If an AssertionError is thrown, it’s a sign that something is wrong with your code. Don’t simply catch the AssertionError and move on – investigate the issue and fix it.
Use assertions for internal invariant checks: Assertions are great for checking conditions that should always hold true within your code. They’re not meant to replace error handling for external errors, such as invalid user input or file I/O errors.
Remember, assertions are a tool to help you write better code. Used wisely, they can catch bugs early, simplify debugging, and improve code quality.
Understanding the Concept of Assertions
Assertions are a critical part of Java programming, particularly when it comes to debugging. But what exactly are they, and why are they so important?
What are Assertions?
In Java, an assertion is a statement that you believe to be true at the time the program executes. If it’s not true, the system will throw an AssertionError.
int x = 5;
assert x > 0 : 'x is negative';
# Output:
# (No output, as the assertion is true)
In this example, we’re asserting that ‘x’ is greater than 0. Since ‘x’ is indeed greater than 0, the program runs without throwing an AssertionError.
The Role of Assertions in Debugging
Assertions play a key role in debugging by helping you catch bugs early in the development cycle. By checking that certain conditions hold true throughout your code, you can identify and fix problems before they become more serious.
The Importance of Assertions in Java Programming
Assertions are not just useful for debugging – they can also improve the quality of your code. By documenting your assumptions about the program’s behavior, assertions make your code easier to understand and maintain.
Moreover, assertions can serve as a form of self-checking documentation. They can provide other developers with insights into your thought process and expectations about the code’s behavior, making collaboration easier.
In conclusion, assertions are a powerful tool in Java programming. They can improve the debugging process, enhance code quality, and facilitate collaboration among developers. While they should be used judiciously, they are undoubtedly a valuable addition to any Java programmer’s toolkit.
Assertions in Larger Projects and Test-Driven Development
Assertions are not just useful for small programs or scripts – they can also provide significant benefits in larger projects and in test-driven development (TDD).
The Role of Assertions in Larger Projects
In larger projects, assertions can help ensure that the various components of your program interact correctly. They can serve as a form of documentation, making it easier for other developers to understand your code. Furthermore, they can help catch bugs early, before they become more difficult to track down and fix.
Assertions and Test-Driven Development
In test-driven development, assertions are used to write tests before the actual code. These tests, which assert certain conditions, guide the development of the code. When the tests pass, you know your code is functioning as expected.
Exploring Related Concepts
If you’re interested in assertions, you might also want to explore related concepts like unit testing in Java. Unit tests, like assertions, help ensure that your code is working correctly. They can be particularly useful in test-driven development.
Further Resources for Mastering Assertions in Java
- Beginner’s Guide to Java Error Handling – Master Java error handling best practices.
Understanding java.lang.NoClassDefFoundError – Understand the Java error “java.lang.NoClassDefFoundError.”
Basics of Java Optional – Explore Java Optional for handling potentially null values.
Oracle’s Guide to Programming With Assertions explains how to use assertions in Java.
Baeldung’s Article on Assertions in Java covers everything from the basics to more advanced topics.
JUnit User Guide is a great place to start if you’re interested in exploring unit testing in Java.
These resources should provide you with a solid foundation for mastering assertions in Java and understanding their relevance in larger projects and test-driven development.
Wrapping Up: assert Keyword in Java
In this comprehensive guide, we’ve delved deep into the world of assertions in Java, exploring from the basics to more advanced techniques.
We began with the fundamentals, understanding what assertions are and their role in Java programming. We then moved onto the basic usage of ‘assert’, highlighting its advantages and potential pitfalls. From there, we ventured into more complex uses of ‘assert’, such as using it with complex expressions, and explored alternative debugging methods in Java, such as logging and exception handling.
Along our journey, we tackled common issues one might encounter when using assertions, offering solutions and workarounds for each issue. We also discussed the relevance of assertions in larger projects and test-driven development, and directed readers to more resources for deeper understanding.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Assertions | Detect bugs early, simplify debugging, improve code quality | Can be disabled, should not change the state of a program |
Logging | More information, better control | Increased complexity, performance impact |
Exception Handling | More information, better control | Increased complexity |
Whether you’re just starting out with ‘assert’ in Java or you’re looking to level up your debugging skills, we hope this guide has given you a deeper understanding of assertions and their capabilities.
With its balance of simplicity, flexibility, and power, ‘assert’ is a critical tool for debugging in Java. Now, you’re well equipped to make the most of assertions in your Java programming journey. Happy coding!