Python Regex: Guide to the ‘re’ Library and Functions
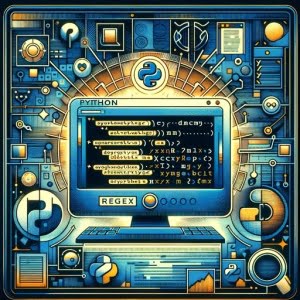
Are you wrestling with regular expressions in Python? Fear not, you’re not alone. Regular expressions, or regex, can feel like an impenetrable fortress of complexity. But just like a seasoned detective, Python’s regex module can help you uncover any pattern you’re searching for in a string.
This comprehensive guide will take you from a novice pattern hunter to a proficient regex user. We will explore the basics of Python regex, take a dive into more advanced techniques, and even delve into some alternative approaches. So buckle up, and let’s start our journey into the world of Python regex.
TL;DR: How Do I Use Regular Expressions in Python?
Python’s
re
module provides functions to work with Regular Expressions. Let’s illustrate this with a simple example:
import re
pattern = r'\w+'
string = 'Hello, World!'
matches = re.findall(pattern, string)
print(matches)
# Output:
# ['Hello', 'World']
In the code snippet above, we import the re
module, define a pattern we’re looking for (in this case, any word character), and then use the findall
function to search our string (‘Hello, World!’) for any matches to our pattern. The result is a list of all matches found (['Hello', 'World']
).
Interested in unraveling more about Python regular expressions? Continue reading for a comprehensive guide that covers everything from the basics to advanced techniques.
Table of Contents
- Python Regex: Getting Started with the Basics
- Python Regex: Unlocking Intermediate Techniques
- Exploring Alternative Python Regex Libraries
- Dealing with Regex Pitfalls in Python
- The Theory Behind Python Regex: Finite Automata and Regex Syntax
- Python Regex in the Wild: Data Cleaning and Web Scraping
- Additional Resources for Python Libraries
- Python Regex: Summing Up the Journey
Python Regex: Getting Started with the Basics
Python’s re
module is the gatekeeper to using regular expressions. Let’s start by importing it:
import re
With the module imported, we can now start defining patterns and searching for them in strings. The most basic use of regex is to check if a pattern exists within a string. Let’s see this in action:
pattern = 'Python'
string = 'I love Python!'
match = re.search(pattern, string)
print(match)
# Output:
# <re.Match object; span=(7, 13), match='Python'>
In the above example, we define a pattern (‘Python’) and a string (‘I love Python!’). We then use the search
function from the re
module to look for our pattern within the string. If a match is found, the search
function returns a match object. The match object’s output tells us that ‘Python’ was found in the string, starting at index 7 and ending at index 13.
The re
module also provides other functions like match
(checks if the pattern matches at the beginning of the string), findall
(returns all non-overlapping matches as a list), split
(splits the string by the occurrences of a pattern), and sub
(replaces one or many matches with a string).
Stay tuned as we dive deeper into Python regex and uncover more advanced techniques in the following sections.
Python Regex: Unlocking Intermediate Techniques
As we delve deeper into Python regex, we encounter more complex patterns and techniques. These include groups, lookaheads, and lookbehinds. Let’s explore these techniques one by one.
Grouping in Python Regex
Grouping allows us to combine several patterns into one. It’s like putting a part of a regex inside parentheses ( )
. Let’s see an example:
pattern = '(Python|Java)'
string = 'I love Python and Java!'
matches = re.findall(pattern, string)
print(matches)
# Output:
# ['Python', 'Java']
In the code above, we define a pattern that matches either ‘Python’ or ‘Java’. The findall
function returns all matches found in the string, which are ‘Python’ and ‘Java’.
Lookaheads in Python Regex
Lookaheads allow us to match a pattern only if it’s followed by another pattern. A positive lookahead is denoted by (?=...)
and a negative lookahead by (?!...)
. Here’s a positive lookahead example:
pattern = 'Python(?= programming)'
string = 'I love Python programming!'
match = re.search(pattern, string)
print(match)
# Output:
# <re.Match object; span=(7, 13), match='Python'>
The pattern ‘Python(?= programming)’ matches ‘Python’ only if it’s followed by ‘ programming’. The search
function finds a match since ‘Python’ in our string is indeed followed by ‘ programming’.
Lookbehinds in Python Regex
Lookbehinds are the opposite of lookaheads. They match a pattern only if it’s preceded by another pattern. A positive lookbehind is denoted by (?<=...)
and a negative lookbehind by (?<!...)
. Let’s see a positive lookbehind in action:
pattern = '(?<=love )Python'
string = 'I love Python!'
match = re.search(pattern, string)
print(match)
# Output:
# <re.Match object; span=(7, 13), match='Python'>
The pattern ‘(?<=love )Python’ matches ‘Python’ only if it’s preceded by ‘love ‘. The search
function finds a match since ‘Python’ in our string is indeed preceded by ‘love ‘.
These advanced techniques can unlock new possibilities and make your Python regex more powerful. Stay with us as we explore alternative approaches and delve even deeper into Python regex.
Exploring Alternative Python Regex Libraries
While Python’s built-in re
module is powerful, there are third-party libraries that offer additional features and capabilities. One such library is regex
, a module that’s fully compatible with re
but includes extra functionality.
The Power of the regex
Module
Let’s start by installing the regex
module. You can do this using pip:
pip install regex
Now let’s see the regex
module in action:
import regex
pattern = '\p{L}+'
string = 'Hello, World!'
matches = regex.findall(pattern, string)
print(matches)
# Output:
# ['Hello', 'World']
The pattern ‘\p{L}+’ matches any sequence of letters. This is a Unicode property escape, which is not supported by the re
module but is supported by regex
. As you can see, the regex
module can handle more complex patterns than re
.
Weighing the Pros and Cons
The regex
module is more powerful than re
, but it also has some downsides. It’s slower than re
and not included in Python’s standard library, which means it needs to be installed separately. However, if you need the extra functionality, regex
can be a great tool in your Python regex toolkit.
Whether you choose to use re
or regex
depends on your specific needs. As you continue to explore Python regex, you’ll develop a better understanding of which tool is best for each job.
Dealing with Regex Pitfalls in Python
Regular expressions are a powerful tool, but they can also be tricky. Here, we’ll discuss some common issues you might encounter when working with Python regex, along with solutions and best practices.
Special Characters and Python Regex
Special characters in regex, also known as metacharacters, can cause unexpected results if not properly handled. These characters include . ^ $ * + ? { } [ ] \ | ( )
. To match these characters literally, you need to escape them using a backslash \
.
pattern = 'Python\+'
string = 'I love Python+'
match = re.search(pattern, string)
print(match)
# Output:
# <re.Match object; span=(7, 14), match='Python+'>
In the example above, we’re searching for ‘Python+’ in our string. The plus sign is a special character in regex, so we escape it with a backslash. The search
function finds a match.
Unicode and Python Regex
Working with Unicode characters can be another challenge in Python regex. However, Python’s re
module supports Unicode characters. You can use the special sequence \w
to match any Unicode word character.
pattern = '\w+'
string = '你好, World!'
matches = re.findall(pattern, string)
print(matches)
# Output:
# ['你好', 'World']
In this example, our pattern matches any sequence of word characters, including Unicode characters. The findall
function returns all matches found in the string, which includes the Chinese characters ‘你好’ and the English word ‘World’.
Regular expressions can be complex, but understanding these common issues and how to handle them can make your journey with Python regex smoother and more productive.
The Theory Behind Python Regex: Finite Automata and Regex Syntax
To truly master Python regex, it’s important to understand the theory behind regular expressions. This includes the concept of finite automata and the syntax of regex.
Finite Automata: The Engine Behind Regex
Finite automata are theoretical machines used to recognize patterns. They are the engine that powers regular expressions. In the context of regex, a finite automaton reads a string character by character. If the string matches the defined pattern, the automaton accepts it; otherwise, it rejects it.
While finite automata are a complex subject, understanding their basic function can help you appreciate the power and efficiency of regular expressions.
Python Regex Syntax: The Building Blocks of Patterns
Python regex syntax consists of special characters and sequences that define search patterns. Here are some of the most common ones:
.
: Matches any character except newline\w
: Matches any word character (equivalent to[a-zA-Z0-9_]
)\d
: Matches any digit (equivalent to[0-9]
)*
: Matches zero or more repetitions of the preceding regex+
: Matches one or more repetitions of the preceding regex?
: Matches zero or one repetition of the preceding regex{m,n}
: Matches at leastm
and at mostn
repetitions of the preceding regex
Here’s an example that uses some of these syntax elements:
pattern = '\w+@\w+\.com'
string = 'My email is [email protected].'
match = re.search(pattern, string)
print(match)
# Output:
# <re.Match object; span=(13, 29), match='[email protected]'>
In the code above, the pattern ‘\w+@\w+.com’ matches any email address that consists of word characters, followed by ‘@’, followed by more word characters, followed by ‘.com’. The search
function finds a match in our string.
Understanding Python regex syntax and the theory behind regular expressions can help you write more efficient and effective regex. As we continue to explore Python regex, we’ll see how these concepts apply to real-world scenarios.
Python Regex in the Wild: Data Cleaning and Web Scraping
Regular expressions are not just a theoretical concept; they have practical applications in many areas of programming. Two of the most common applications are data cleaning and web scraping.
Data Cleaning with Python Regex
Data cleaning is a crucial step in any data analysis project. Python regex can help us to clean and preprocess data efficiently. For instance, we can use regex to remove unwanted characters, extract useful information, or standardize data formats.
Here’s a simple example of data cleaning using Python regex:
import re
# A list of dirty data
data = ['123-45-6789', '987 65 4321', '100.200.300.400', 'hello, world!']
# Define a pattern for Social Security numbers
pattern = '\d{3}-\d{2}-\d{4}'
# Clean the data
clean_data = [item for item in data if re.match(pattern, item)]
print(clean_data)
# Output:
# ['123-45-6789']
In this example, we have a list of dirty data. We define a pattern for Social Security numbers and use a list comprehension with re.match
to filter out any items that don’t match this pattern. The result is a list of clean data.
Web Scraping with Python Regex
Web scraping is another area where Python regex shines. While libraries like Beautiful Soup or Scrapy are often used for web scraping, regex can be useful for extracting information from web pages.
However, it’s important to note that regex should not be used to parse HTML in most cases, as HTML is not a regular language and can’t be accurately parsed with regular expressions. Instead, regex can be used to extract specific patterns of text within the HTML content.
For a more in-depth look at Python’s string methods and the Beautiful Soup library for web scraping, stay tuned for our upcoming articles. These topics will further expand your Python skills and open new possibilities for your projects.
Additional Resources for Python Libraries
To expand your knowledge about the vast array of Python Libraries and enhance your proficiency, we would like to introduce a curated collection of resources:
- Python Libraries for Efficient Coding – Explore libraries for building web applications and content management systems.
Data Clustering with K-Means in Python – Discover how to group data points into clusters based on similarity with K-means.
Simplifying Imports from External Directories in Python – Dive into the world of module organization and path manipulation.
Python Package Index (PyPI) – Explore and download various Python packages with the official Python Package Index.
Best Python Libraries – Discover the best Python libraries as compiled by BairesDev.
Python Libraries – Read about Python libraries extensively with LearnPython.
Diving deep into these resources will open up a myriad of possibilities for your Python projects.
Python Regex: Summing Up the Journey
We’ve traversed the landscape of Python regular expressions, starting from the basics, to advanced techniques, and even exploring alternative approaches. Let’s recap the key points:
The Basics of Python Regex
We learned how to use Python’s re
module to define patterns and search for them in strings. We saw that functions like search
, match
, findall
, split
, and sub
are the workhorses of Python regex.
import re
pattern = 'Python'
string = 'I love Python!'
match = re.search(pattern, string)
print(match)
# Output: <re.Match object; span=(7, 13), match='Python'>
Advanced Techniques: Grouping, Lookaheads, and Lookbehinds
We delved into more complex regex techniques, including grouping, lookaheads, and lookbehinds. These techniques allow us to create more sophisticated patterns and make our Python regex more powerful.
pattern = '(Python|Java)'
string = 'I love Python and Java!'
matches = re.findall(pattern, string)
print(matches)
# Output: ['Python', 'Java']
Alternative Approaches: The regex
Module
We explored the regex
module, a third-party library that offers additional features beyond the re
module. While regex
is slower and needs to be installed separately, it can handle more complex patterns and offers extra functionality.
import regex
pattern = '\p{L}+'
string = 'Hello, World!'
matches = regex.findall(pattern, string)
print(matches)
# Output: ['Hello', 'World']
Troubleshooting: Special Characters and Unicode
Finally, we discussed common issues when working with Python regex, such as dealing with special characters and Unicode. We saw that understanding these issues and how to handle them can make our Python regex journey smoother and more productive.
pattern = 'Python\+'
string = 'I love Python+'
match = re.search(pattern, string)
print(match)
# Output: <re.Match object; span=(7, 14), match='Python+'>
Whether you’re a beginner or an experienced developer, mastering Python regex can boost your productivity and open up new possibilities in your projects. We hope this guide has been a helpful companion on your Python regex journey.