Python How-To | Import Libraries From Another Directory
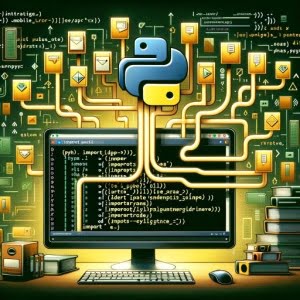
Ever felt like you’re wrestling with Python to import modules from a different directory? You’re not alone.
This comprehensive guide will walk you through the process of importing Python modules from different directories, covering everything from basic to advanced techniques.
Whether you’re a beginner just starting out or a seasoned pro looking to brush up on your skills, there’s something here for everyone. So let’s dive in and demystify Python imports!
TL;DR: How Do I Import a Python Module from Another Directory?
The answer is straightforward: You can use Python’s
sys.path.append()
function to add the directory to the system path. Here’s a simple example:
import sys
sys.path.append('/path/to/directory')
import your_module
In the above example, we first import the sys
module. Then we use the sys.path.append()
function to add the directory where our module resides. Finally, we import the module itself. It’s as simple as that!
If you’re interested in mastering Python imports, including more advanced techniques and potential pitfalls, keep reading. This guide will give you a comprehensive understanding of Python’s import system.
Table of Contents
Basic Use: Python’s sys.path.append()
Function
Python’s sys.path.append()
function is the most basic and commonly used method to import modules from different directories.
When you’re importing a module in Python, it searches for it in the directories listed in sys.path
. So, if you want to import a module from a directory that’s not already in sys.path
, you can simply add that directory using sys.path.append()
.
Here’s a simple example:
import sys
# print the original sys.path
print('Original sys.path:', sys.path)
# append a new directory to sys.path
sys.path.append('/path/to/directory')
# print the updated sys.path
print('Updated sys.path:', sys.path)
# now you can import your module
import your_module
In this example, we first print the original sys.path
to see what directories Python is currently looking in. Then we append a new directory to sys.path
and print it again to confirm that our new directory has been added. Finally, we import our module.
This method is simple and straightforward, but it does have a potential pitfall: it only affects
sys.path
for the current session. If you close Python and open it again,sys.path
will be reset to its original state.
If you’re working on a larger project or a script that will be run multiple times, you’ll need to append your directory to sys.path
each time the script is run.
As you progress in your Python journey, you may find yourself needing more control over your directories. This is where the os
module comes in handy, specifically os.path
and os.chdir()
.
The os.path
module contains functions for manipulating file paths, and os.chdir()
changes the current working directory. By changing the working directory, you can import Python modules as if they were in the same directory as your script.
Let’s see how this works with an example:
import os
# print the current working directory
print('Original working directory:', os.getcwd())
# change the working directory
os.chdir('/path/to/directory')
# print the updated working directory
print('Updated working directory:', os.getcwd())
# now you can import your module
import your_module
In this code block, we first print the original working directory using os.getcwd()
. Then we change the working directory to the directory where our module resides using os.chdir()
. We print the updated working directory to confirm the change, and finally, we import our module.
One key thing to remember is that using
os.chdir()
changes the working directory permanently for the current session, unlikesys.path.append()
. So any subsequent file operations will be performed in the new working directory unless you change it again.
While this method gives you more control, it also comes with more responsibility. You need to make sure to change the working directory back to its original state if other parts of your code depend on it. It’s also worth noting that changing the working directory can have side effects if your code is multithreaded, as the working directory is a process-wide setting.
Alternative Approaches: Beyond Basic Imports
While sys.path.append()
and os.chdir()
are great tools for importing modules from different directories, Python offers a variety of other methods. Let’s explore some of these alternatives: relative imports, the imp
module, and the importlib
module.
Relative Imports
Relative imports allow you to import modules based on their relative position to the current module. They are particularly useful when working with larger projects that have a complex directory structure.
Here’s an example:
# Suppose you have the following directory structure:
#
# my_project/
# ├── main.py
# └── my_module/
# └── sub_module.py
# You can use a relative import in main.py to import sub_module.py like this:
from .my_module import sub_module
In this example, the dot (.
) before my_module
indicates that my_module
is in the same directory as the current module (main.py
).
The imp
Module
The imp
module provides functions to help find and load Python modules. Here’s how you can use it:
import imp
# load a source module from a file
file, pathname, description = imp.find_module('my_module', ['/path/to/directory'])
my_module = imp.load_module('my_module', file, pathname, description)
In this code block, imp.find_module()
finds the module and returns a tuple containing the open file, the pathname of the file, and a description. imp.load_module()
then loads the module from the open file.
The importlib
Module
The importlib
module is a powerful tool for advanced imports. It allows you to import a module programmatically using its name as a string:
import importlib
# import a module using its name as a string
my_module = importlib.import_module('my_module')
In this example, importlib.import_module()
imports the module and returns it, allowing you to assign it to a variable.
Method | Advantages | Disadvantages |
---|---|---|
sys.path.append() | Simple and straightforward | Changes are session-specific |
os.chdir() | Changes are permanent for the session | Can have side effects in multithreaded code |
Relative imports | Useful for complex directory structures | Can be confusing if overused |
imp module | Provides low-level import functions | More complex than other methods |
importlib module | Allows programmatic imports | Requires the module name as a string |
Each of these methods has its advantages and disadvantages, and the best one to use depends on your specific needs. It’s important to understand all the tools at your disposal so you can choose the most effective one for your task.
Troubleshooting Common Import Issues
Even with the right tools and techniques, you might encounter some common issues while importing Python modules from different directories. Let’s discuss some of these problems and how to tackle them.
Tackling ‘ModuleNotFoundError’
The most common issue you might run into is the ‘ModuleNotFoundError’. This error occurs when Python can’t find the module you’re trying to import. Here’s an example:
# trying to import a non-existent module
import non_existent_module
Running this code would result in the following output:
# Output
ModuleNotFoundError: No module named 'non_existent_module'
This error typically means that Python can’t find your module in the directories listed in sys.path
. To fix this, you can add the directory containing your module to sys.path
using sys.path.append()
as we discussed earlier.
Dealing with Relative Import Issues
Relative imports can be very useful, but they can also be a source of confusion. If you’re not careful, you might get an error like this:
from .my_module import sub_module
Running this code might result in the following output:
# Output
ImportError: attempted relative import with no known parent package
This error means that Python doesn’t know what the parent package of your current module is. To fix this, make sure that your script is part of a package (i.e., it’s inside a directory that contains an __init__.py
file), and that you’re running your script using the -m
option.
For example, if your script is named main.py
, you should run it like this: python -m main
Other Considerations
Remember that changes to sys.path
or the working directory are only effective for the current session. If you need your changes to persist across sessions, you might need to consider other methods like modifying the PYTHONPATH environment variable.
Also, keep in mind that changing the working directory using os.chdir()
can have side effects, especially in multithreaded code. Always make sure to change the working directory back to its original state after you’re done importing your modules.
Python’s Import System and System Path
Before diving deeper into the mechanisms of importing modules from various directories in Python, it’s essential to grasp the fundamentals of Python’s import system and the concept of the system path.
When you import a module in Python, the interpreter searches for it in a list of directories known as the system path. This list is stored in sys.path
and includes several locations, such as the directory containing the input script (or the current directory if the interpreter is interactive), and the site-packages directory where third-party modules are installed.
Here’s how you can check your current system path:
import sys
# print the system path
print(sys.path)
Running this code will output something like this:
# Output
['', '/usr/lib/python3.8', '/usr/lib/python3.8/lib-dynload', '/home/user/.local/lib/python3.8/site-packages', '/usr/local/lib/python3.8/dist-packages']
Each string in this list is a directory on your system. When you try to import a module, Python checks each of these directories in order for a file that matches the name of the module.
The Role of Directories in Python
In Python, a directory is considered a package if it contains an __init__.py
file. Packages can contain other packages (directories) and modules (Python files). This structure allows you to organize your code in a hierarchical manner, making it easier to manage and understand.
Relative and Absolute Imports
When importing modules, Python allows you to use either relative or absolute imports. An absolute import specifies the full path (starting from the root package) to the module you’re importing, while a relative import specifies the path to the module relative to the current module.
Here’s an example of an absolute import:
# absolute import
import my_package.my_module
And here’s an example of a relative import:
# relative import
from . import my_module
In the relative import, the dot (.
) represents the current package. You can use multiple dots to represent parent packages: ..
is the parent package, ...
is the grandparent package, and so on.
Understanding these fundamentals will help you navigate Python’s import system and effectively manage your code across different directories.
Exploring Further
So far, we’ve covered the basics and some advanced techniques of importing Python modules from different directories. But as you venture into larger projects or start working with complex Python packages, you’ll need to understand more about Python’s import system and how it interacts with package management.
The Role of __init__.py
and __main__.py
In Python, a directory is considered a package if it contains an __init__.py
file. This file is executed when the package is imported, and it can be used to initialize package-level variables or perform other setup tasks. Here’s an example:
# __init__.py
print('Initializing package...')
If you import this package, you’ll see the following output:
# Output
Initializing package...
In contrast, __main__.py
is executed when you run the package as a script. This allows you to add code to your package that is only executed when the package is run directly, not when it is imported.
Package Management and Imports
When working with larger projects, you might find yourself dealing with multiple dependencies that need to be managed. Python’s package managers, such as pip and conda, can help you manage these dependencies and ensure that your imports work as expected.
Further Learning Resources
Importing modules from different directories is just one aspect of Python’s import system. To deepen your understanding, you might want to explore topics like module reloading, dynamic imports, and the internals of Python’s import system. Here are some resources to get you started:
- IOFlood’s Python Libraries Article dives deep into libraries for handling files, directories, and data formats.
Text Pattern Matching in Python: Regex Simplified – Learn fundamental regex syntax and pattern creation in Python.
Exploring Pygame: A Toolkit for Game Development – Explore the popular Pygame library for game development in Python.
Python’s Official Documentation on Modules explains Python modules with the official Python documentation.
Python’s Official Documentation on Import System describes Python’s import system and proper usage tips.
Python Module of the Week Series on Importlib – Dives into the Importlib module in Python for versatile code importation.
Remember, the key to mastering Python imports is practice and exploration. Don’t be afraid to experiment with different techniques and dig deeper into the topics that interest you.
Recap:
Throughout this guide, we’ve explored various ways to import Python modules from different directories.
We started with the basics, using sys.path.append()
to add the desired directory to Python’s system path. We then delved into more advanced methods, such as changing the working directory with os.chdir()
, using relative imports, and employing the imp
and importlib
modules for more complex scenarios.
Each method has its advantages and potential pitfalls. For instance, sys.path.append()
is simple and straightforward, but it only affects the current session. On the other hand, os.chdir()
changes the working directory permanently for the session, but it can have side effects in multithreaded code.
We also touched upon common issues you might encounter, like ‘ModuleNotFoundError’ and problems with relative imports, and provided some solutions and workarounds.
Here’s a quick comparison of the methods we discussed:
Method | Advantages | Disadvantages |
---|---|---|
sys.path.append() | Simple and straightforward | Changes are session-specific |
os.chdir() | Changes are permanent for the session | Can have side effects in multithreaded code |
Relative imports | Useful for complex directory structures | Can be confusing if overused |
imp module | Provides low-level import functions | More complex than other methods |
importlib module | Allows programmatic imports | Requires the module name as a string |
In the end, the best method to use depends on your specific needs and the complexity of your project. By understanding these different techniques, you’ll be well-equipped to handle any Python import task that comes your way.