ArrayList in Java: Your Dynamic Arrays Usage Guide
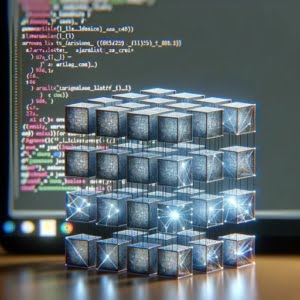
Are you grappling with ArrayLists in Java? You’re not alone. Many developers find themselves in a bind when it comes to handling dynamic arrays in Java, but we’re here to help.
Think of Java’s ArrayLists as a multi-storey building – allowing us to store data in multiple dimensions, providing a versatile and handy tool for various tasks.
In this guide, we’ll walk you through the process of working with ArrayLists in Java, from their creation, manipulation, and usage. We’ll cover everything from the basics of dynamic arrays to more advanced techniques, as well as alternative approaches.
Let’s get started!
TL;DR: What is an ArrayList in Java?
An ArrayList in Java is a part of the Java Collections Framework, housed in the java.util package. It is instantiated with the syntax:
ArrayList<String> newList = new ArrayList<String>();
. It offers us dynamic arrays in Java, which can grow and shrink as needed, unlike static arrays.
Here’s a simple example of how to create an ArrayList in Java:
ArrayList<String> emptyList = new ArrayList<String>();
# Output:
# An empty ArrayList named 'emptyList' of type String is created.
In this example, we’ve created an ArrayList named ’emptyList’ that can store String objects. At this point, ’emptyList’ is empty as we haven’t added any elements to it yet.
This is just a basic introduction to ArrayLists in Java. There’s a lot more to learn about ArrayLists, including how to add elements, retrieve elements, and perform more complex operations. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Getting Started with ArrayList in Java
- Advanced ArrayList Operations in Java
- Exploring Alternatives to ArrayList in Java
- Troubleshooting Common ArrayList Issues in Java
- Understanding Java Collections Framework and ArrayList
- Delving Deeper: ArrayList in Larger Java Applications
- Wrapping Up: ArrayList in Java
Getting Started with ArrayList in Java
In Java, creating an ArrayList is straightforward. Let’s dive into how to create an ArrayList, add elements to it, and retrieve elements from it.
Creating an ArrayList
Here’s how to create an ArrayList in Java:
ArrayList<String> list = new ArrayList<String>();
# Output:
# An empty ArrayList named 'list' of type String is created.
In this code, we’ve created an empty ArrayList named ‘list’ that can store String objects.
Adding Elements to an ArrayList
Now, let’s add some elements to our ArrayList:
list.add("Apple");
list.add("Banana");
list.add("Cherry");
# Output:
# The ArrayList 'list' now contains the elements: Apple, Banana, Cherry.
The add
method is used to add elements to the ArrayList. In this case, we’ve added ‘Apple’, ‘Banana’, and ‘Cherry’ to our list.
Retrieving Elements from an ArrayList
To retrieve elements from an ArrayList, we use the get
method:
String fruit = list.get(1);
# Output:
# The variable 'fruit' now contains the value: Banana.
The get
method retrieves the element at the specified index. Remember, ArrayList indices start at 0, so ‘list.get(1)’ retrieves the second element, which is ‘Banana’.
ArrayLists in Java are versatile and dynamic, which makes them an excellent choice for storing data that may change over time. However, it’s important to remember that ArrayLists are not synchronized, which means they’re not thread-safe. If you’re working with multiple threads, you’ll need to take additional steps to ensure thread safety.
Advanced ArrayList Operations in Java
Now that we’ve covered the basics, let’s dive into more complex operations with ArrayLists in Java. We’ll discuss removing elements, iterating over an ArrayList, and sorting.
Removing Elements from an ArrayList
Removing elements from an ArrayList is as simple as adding them. Let’s remove an element from our list:
list.remove("Banana");
# Output:
# The ArrayList 'list' now contains the elements: Apple, Cherry.
The remove
method removes the first occurrence of the specified element from the ArrayList. In this case, we’ve removed ‘Banana’ from our list.
Iterating Over an ArrayList
Often, we want to go through all the elements in an ArrayList. This process is called iteration. Here’s how to iterate over an ArrayList using a for-each loop:
for (String fruit : list) {
System.out.println(fruit);
}
# Output:
# Apple
# Cherry
In this code, we’ve used a for-each loop to print out each element in the ArrayList. The loop goes through each element in ‘list’, and for each element, it prints out the element.
Sorting an ArrayList
Sorting an ArrayList is easy with the Collections.sort()
method. Here’s how to sort an ArrayList in ascending order:
Collections.sort(list);
# Output:
# The ArrayList 'list' now contains the elements: Apple, Cherry.
The Collections.sort()
method sorts the elements of the ArrayList in ascending order. In this case, since our list only contains ‘Apple’ and ‘Cherry’, it remains the same after sorting.
These advanced operations can make your work with ArrayLists in Java much easier. Keep in mind that while ArrayLists are not synchronized or thread-safe by default, Java provides ways to make them safe for use in multi-threaded environments if necessary.
Exploring Alternatives to ArrayList in Java
While ArrayLists are a powerful tool, Java offers other data structures that can be used in place of ArrayLists, such as LinkedList and Vector. Let’s explore these alternatives, their usage, and effectiveness.
LinkedList: A Dynamic Data Structure
LinkedList is a part of the Java Collections Framework and provides a dynamic data structure where elements are linked using pointers. Here’s a simple example of using LinkedList in Java:
LinkedList<String> linkedList = new LinkedList<String>();
linkedList.add("Apple");
linkedList.add("Banana");
linkedList.add("Cherry");
# Output:
# A LinkedList named 'linkedList' of type String is created and contains the elements: Apple, Banana, Cherry.
In this example, we’ve created a LinkedList and added elements to it. LinkedLists are particularly useful when you need to frequently add and remove elements, but they can be slower than ArrayLists for certain operations, such as get and set operations.
Vector: A Synchronized Alternative
Vector, unlike ArrayList and LinkedList, is synchronized and therefore thread-safe. Here’s how to use a Vector in Java:
Vector<String> vector = new Vector<String>();
vector.add("Apple");
vector.add("Banana");
vector.add("Cherry");
# Output:
# A Vector named 'vector' of type String is created and contains the elements: Apple, Banana, Cherry.
In this code, we’ve created a Vector and added elements to it. Vectors can be useful in multi-threaded environments where thread safety is a concern, but they come with a performance cost.
Data Structure | Synchronized | Speed | Best Use Case |
---|---|---|---|
ArrayList | No | Fast | When frequent get/set operations are needed |
LinkedList | No | Varies | When frequent add/remove operations are needed |
Vector | Yes | Slow | When thread safety is a concern |
While ArrayLists are a go-to choice for many developers, it’s important to consider these alternatives based on the specific needs of your project.
Troubleshooting Common ArrayList Issues in Java
When working with ArrayLists in Java, you may encounter some common issues. Let’s discuss two of these: ConcurrentModificationException and IndexOutOfBoundsException, along with their solutions and workarounds.
Dealing with ConcurrentModificationException
If you try to modify an ArrayList while iterating over it, Java might throw a ConcurrentModificationException. Let’s see an example:
for (String fruit : list) {
if (fruit.equals("Banana")) {
list.remove(fruit);
}
}
# Output:
# Throws ConcurrentModificationException
To avoid this, you can use an Iterator to safely remove items during iteration:
Iterator<String> iterator = list.iterator();
while (iterator.hasNext()) {
String fruit = iterator.next();
if (fruit.equals("Banana")) {
iterator.remove();
}
}
# Output:
# The ArrayList 'list' now contains the elements: Apple, Cherry.
Handling IndexOutOfBoundsException
An IndexOutOfBoundsException is thrown if you try to access an index that is out of the range of the ArrayList. For example:
String fruit = list.get(3);
# Output:
# Throws IndexOutOfBoundsException
To avoid this exception, always ensure that the index you are trying to access is within the range of the ArrayList:
if (list.size() > 3) {
String fruit = list.get(3);
}
# Output:
# No exception is thrown
These are just a couple of the common issues you might encounter when working with ArrayLists in Java. Remember, understanding the error messages and knowing how to troubleshoot them will make you a more efficient and effective Java programmer.
Understanding Java Collections Framework and ArrayList
Before we delve deeper into ArrayList, it’s crucial to understand the Java Collections Framework and how ArrayList fits into it.
The Java Collections Framework
The Java Collections Framework is a set of interfaces and classes that provides a unified architecture for manipulating and representing collections. It allows for the manipulation of data structures, which can hold multiple items, such as lists, sets, and maps.
List<String> list = new ArrayList<>();
Set<String> set = new HashSet<>();
Map<String, String> map = new HashMap<>();
# Output:
# An ArrayList, HashSet, and HashMap are created.
In the above example, we’ve created an ArrayList, HashSet, and HashMap. Each of these is a part of the Java Collections Framework and serves a unique purpose in data handling.
The Role of ArrayList in Java Collections
ArrayList is a part of the List interface in the Java Collections Framework. It’s like a dynamic array, which can grow and shrink as needed. This makes ArrayLists a popular choice for storing data that may change over time.
Static vs. Dynamic Arrays
The key difference between static and dynamic arrays lies in their flexibility. Static arrays have a fixed length that is set at the time of creation. On the other hand, dynamic arrays, like ArrayLists, can change their size dynamically, allowing for more flexible data management.
int[] staticArray = new int[3];
ArrayList<Integer> dynamicArray = new ArrayList<>();
# Output:
# A static array of size 3 and an empty dynamic ArrayList are created.
In the above code, ‘staticArray’ has a fixed size of 3, while ‘dynamicArray’ can grow or shrink as needed.
Understanding these fundamentals of the Java Collections Framework and the nature of ArrayLists will help you utilize them more effectively in your Java applications.
Delving Deeper: ArrayList in Larger Java Applications
ArrayLists aren’t just for small-scale projects or standalone tasks. They play an integral role in larger Java applications, particularly in data processing and GUI development.
ArrayLists in Data Processing
In the realm of data processing, ArrayLists are invaluable. They allow for dynamic data manipulation, making it easy to add, remove, and retrieve data on the fly. This flexibility is essential in data processing, where the volume of data can change rapidly.
ArrayLists in GUI Development
When developing Graphical User Interfaces (GUIs) in Java, ArrayLists come into play in handling dynamic data. For example, if you’re creating a list that updates based on user interaction, an ArrayList can store the list items and update as needed.
Exploring Related Concepts: Multithreading and Serialization
As you continue your journey with ArrayLists and Java, two concepts you might want to explore further are multithreading and serialization. Multithreading can help you make your Java applications more efficient by performing multiple operations simultaneously. Serialization, on the other hand, is a mechanism of converting the state of an object into a byte stream, which is useful for saving state in applications.
Further Resources for Mastering ArrayList in Java
To deepen your understanding of ArrayLists and related concepts in Java, here are some resources you might find helpful:
- Introduction to Java Collections – Master the usage of Java Collections for efficient manipulation of data.
Understanding Array vs ArrayList – Learn about fixed-size arrays versus dynamically resizable ArrayLists.
Oracle’s Java Tutorials covers a wide range of topics, including collections and multithreading.
Baeldung’s Guide to Java ArrayList provides a deep dive into ArrayLists and its methods.
Java ArrayList Tutorial by W3Schools covers the basics of using ArrayList in Java.
Wrapping Up: ArrayList in Java
In this comprehensive guide, we’ve explored the ins and outs of ArrayList in Java. From the basics of creating and manipulating an ArrayList to advanced usage and alternative approaches, we’ve covered a wide range of topics to help you master this dynamic data structure.
We began with the basics, explaining how to create an ArrayList and add elements to it. We then moved on to more advanced topics, such as removing elements from an ArrayList, iterating over its elements, and sorting the ArrayList. We also discussed common issues you might encounter when working with ArrayLists, such as ConcurrentModificationException and IndexOutOfBoundsException, and provided solutions to these problems.
Next, we delved into alternative approaches to handling collections in Java, such as LinkedList and Vector. We compared these data structures with ArrayList, discussing their pros and cons to give you a broader perspective on data handling in Java.
Here’s a quick comparison of the methods we’ve discussed:
Data Structure | Synchronized | Speed | Best Use Case |
---|---|---|---|
ArrayList | No | Fast | When frequent get/set operations are needed |
LinkedList | No | Varies | When frequent add/remove operations are needed |
Vector | Yes | Slow | When thread safety is a concern |
Whether you’re just starting out with ArrayLists in Java or you’re looking to deepen your understanding, we hope this guide has been a valuable resource. The ability to handle dynamic data structures is a powerful tool in any Java developer’s arsenal. Now, you’re well equipped to handle collections in Java with confidence. Happy coding!