Python Abstract Classes | Usage Guide (With Examples)
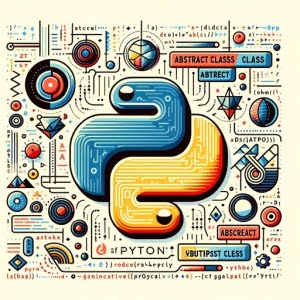
Are you finding it challenging to understand abstract classes in Python? You’re not alone. Many developers grapple with this concept, but once mastered, it can significantly streamline your coding process.
Think of abstract classes as blueprints for other classes, guiding the structure of derived classes. They are a powerful tool in the Python programmer’s arsenal, enabling you to write more efficient, readable, and maintainable code.
In this guide, we’ll walk you through the ins and outs of abstract classes in Python, from basic usage to advanced techniques. We’ll cover everything from creating an abstract class using the abc
module, to more complex uses of abstract classes, and even alternative approaches.
Let’s get started!
TL;DR: How Do I Create an Abstract Class in Python?
In Python, you can create an abstract class using the
abc
module. This module provides the infrastructure for defining abstract base classes (ABCs).
Here’s a simple example:
from abc import ABC, abstractmethod
class AbstractClassExample(ABC):
@abstractmethod
def do_something(self):
pass
In this example, we import the ABC
and abstractmethod
from the abc
module. We then define an abstract class AbstractClassExample
using the ABC
as the base class. The abstractmethod
decorator is used to declare the method do_something
as an abstract method. This means that any class that inherits from AbstractClassExample
must provide an implementation of the do_something
method.
This is a basic way to create an abstract class in Python, but there’s much more to learn about using and understanding abstract classes. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Creating an Abstract Class in Python: The Basics
- Advanced Abstract Classes: Beyond the Basics
- Exploring Alternatives: Metaclasses and Third-Party Libraries
- Troubleshooting Abstract Classes: Common Errors and Solutions
- Best Practices and Optimization
- Python Abstract Classes: An OOP Perspective
- Abstract Classes in the Wild: Real-World Applications
- Wrapping Up: Mastering Python Abstract Classes
Creating an Abstract Class in Python: The Basics
In Python, abstract classes are created using the abc
module, which stands for Abstract Base Class. This module provides the necessary infrastructure for defining abstract base classes (ABCs) in Python. The key components of this module that we’ll use are the ABC
class and the abstractmethod
decorator.
Understanding the abc
Module
The abc
module provides the ABC
class that we can use as a base class for creating abstract classes. Here’s a simple example:
from abc import ABC
class MyAbstractClass(ABC):
pass
In this example, we’ve created a class MyAbstractClass
that inherits from ABC
. However, at this point, MyAbstractClass
is not yet an abstract class because it doesn’t contain any abstract methods.
Using the abstractmethod
Decorator
To create an abstract method, we use the abstractmethod
decorator. An abstract method is a method declared in an abstract class but doesn’t contain any implementation. Subclasses of the abstract class are generally expected to provide an implementation for these methods. Here’s an example:
from abc import ABC, abstractmethod
class MyAbstractClass(ABC):
@abstractmethod
def my_abstract_method(self):
pass
In this example, my_abstract_method
is an abstract method. If we create a subclass of MyAbstractClass
and try to create an instance without providing an implementation for my_abstract_method
, Python will raise a TypeError
.
class MyConcreteClass(MyAbstractClass):
pass
# This will raise a TypeError
instance = MyConcreteClass()
# Output:
# TypeError: Can't instantiate abstract class MyConcreteClass with abstract method my_abstract_method
Advantages and Potential Pitfalls
Using abstract classes provides a clear structure for your code and makes it easier to work in a team setting. It ensures that certain methods must be implemented in any subclass, which can help prevent errors.
However, it’s important to remember that Python’s abc
module is not enforced at the language level but rather at the runtime level. This means that errors related to not implementing abstract methods in a subclass will only be caught at runtime, not during syntax checking or linting. Therefore, thorough testing is crucial when working with abstract classes in Python.
Advanced Abstract Classes: Beyond the Basics
After mastering the basics of abstract classes in Python, you may find yourself needing more advanced techniques. Abstract classes can be used in more complex scenarios like multiple inheritance and interface implementation. Let’s dive into these concepts.
Multiple Inheritance with Abstract Classes
Python supports multiple inheritance, a feature where a class can inherit from more than one base class. This includes abstract classes. Here’s an example:
from abc import ABC, abstractmethod
class Base1(ABC):
@abstractmethod
def method_from_base1(self):
pass
class Base2(ABC):
@abstractmethod
def method_from_base2(self):
pass
class Derived(Base1, Base2):
def method_from_base1(self):
return 'method_from_base1 implementation'
def method_from_base2(self):
return 'method_from_base2 implementation'
# Now we can create an instance of Derived
instance = Derived()
print(instance.method_from_base1())
print(instance.method_from_base2())
# Output:
# 'method_from_base1 implementation'
# 'method_from_base2 implementation'
In this example, Derived
is a subclass of both Base1
and Base2
and provides an implementation for the abstract methods from both base classes.
Implementing Interfaces with Abstract Classes
In Python, abstract classes can also be used to implement interfaces. An interface is a blueprint for a class, much like an abstract class, but it usually only contains method signatures and no implementation.
Here’s an example of using an abstract class to define an interface:
from abc import ABC, abstractmethod
class MyInterface(ABC):
@abstractmethod
def method1(self):
pass
@abstractmethod
def method2(self):
pass
class MyClass(MyInterface):
def method1(self):
return 'method1 implementation'
def method2(self):
return 'method2 implementation'
# Now we can create an instance of MyClass
instance = MyClass()
print(instance.method1())
print(instance.method2())
# Output:
# 'method1 implementation'
# 'method2 implementation'
In this example, MyInterface
is an abstract class that serves as an interface. MyClass
is a concrete class that provides an implementation for the methods defined in MyInterface
.
Using abstract classes for more complex scenarios like these can greatly enhance the structure and readability of your code. However, as with any powerful tool, it’s important to use these techniques judiciously and in the right context.
Exploring Alternatives: Metaclasses and Third-Party Libraries
While the abc
module is a powerful tool for creating abstract classes in Python, there are other approaches you can use, such as metaclasses and third-party libraries like zope.interface
. Let’s dive into these alternative methods.
Using Metaclasses to Create Abstract Classes
A metaclass in Python is a class of a class, or rather, a class that defines the behavior of other classes. You can use metaclasses to create abstract classes in Python. Here’s an example:
class AbstractClassMeta(type):
def __init__(cls, name, bases, attrs):
if not cls.__abstractmethods__:
raise TypeError("Can't instantiate abstract class")
class AbstractClass(metaclass=AbstractClassMeta):
@property
@abstractmethod
def my_abstract_method(self):
pass
In this example, AbstractClassMeta
is a metaclass that raises a TypeError
if the class it’s being applied to doesn’t have any abstract methods. AbstractClass
uses AbstractClassMeta
as its metaclass and defines an abstract method my_abstract_method
.
Leveraging Third-Party Libraries: zope.interface
The zope.interface
library is a third-party library that provides a way to define interfaces and abstract classes in Python. Here’s an example:
from zope.interface import Interface, implementer
class IMyInterface(Interface):
def my_abstract_method():
"""This is an abstract method"""
@implementer(IMyInterface)
class MyClass:
def my_abstract_method(self):
return 'my_abstract_method implementation'
# Now we can create an instance of MyClass
instance = MyClass()
print(instance.my_abstract_method())
# Output:
# 'my_abstract_method implementation'
In this example, IMyInterface
is an interface that defines an abstract method my_abstract_method
. MyClass
provides an implementation for my_abstract_method
and is declared to implement IMyInterface
using the @implementer
decorator.
These alternative approaches to creating abstract classes in Python can provide additional flexibility and capabilities beyond what the abc
module offers. However, they also come with their own trade-offs. Metaclasses can be complex and difficult to understand, while third-party libraries add external dependencies to your project. As always, it’s important to consider the specific needs and constraints of your project when deciding which approach to use.
Troubleshooting Abstract Classes: Common Errors and Solutions
Working with abstract classes in Python can sometimes lead to errors or obstacles. Let’s explore some common issues and their solutions.
Instantiating an Abstract Class
One common mistake when working with abstract classes is attempting to create an instance of an abstract class. Since abstract classes are meant to be a blueprint for other classes, they cannot be instantiated directly. Trying to do so will lead to a TypeError
.
from abc import ABC, abstractmethod
class MyAbstractClass(ABC):
@abstractmethod
def my_abstract_method(self):
pass
# Trying to instantiate an abstract class
instance = MyAbstractClass()
# Output:
# TypeError: Can't instantiate abstract class MyAbstractClass with abstract method my_abstract_method
The solution is to create a concrete subclass that implements all the abstract methods of the abstract class, and then instantiate that subclass.
Not Implementing All Abstract Methods
Another common error is not implementing all the abstract methods in a subclass of an abstract class. If a subclass doesn’t provide implementations for all abstract methods of the superclass, Python will raise a TypeError
when you try to create an instance of the subclass.
from abc import ABC, abstractmethod
class MyAbstractClass(ABC):
@abstractmethod
def my_abstract_method(self):
pass
class MyConcreteClass(MyAbstractClass):
pass
# Trying to instantiate a subclass that doesn't implement all abstract methods
instance = MyConcreteClass()
# Output:
# TypeError: Can't instantiate abstract class MyConcreteClass with abstract method my_abstract_method
The solution is to ensure that all subclasses implement all abstract methods of the superclass.
Best Practices and Optimization
When working with abstract classes in Python, it’s important to follow best practices to ensure your code is efficient and maintainable. Here are a few tips:
- Use abstract classes when you want to provide a common interface for different classes.
- Don’t overuse abstract classes. If a class doesn’t need to provide an interface and doesn’t have any abstract methods, it probably doesn’t need to be an abstract class.
- Keep abstract classes small and focused. They should define a specific interface, not try to do too many things at once.
- Remember that Python’s
abc
module is a runtime feature. Errors related to abstract classes won’t be caught until your code is actually running, so make sure to thoroughly test any code that uses abstract classes.
Python Abstract Classes: An OOP Perspective
To fully understand the concept of abstract classes in Python, it’s crucial to have a grasp of some fundamental principles of Object-Oriented Programming (OOP). These principles include inheritance, encapsulation, and polymorphism. Let’s examine these concepts and the role of abstract classes in each.
Inheritance: The Parent-Child Relationship
Inheritance is an OOP principle that allows one class to inherit the properties and methods of another class. In the context of abstract classes, the abstract class serves as the parent class, and the subclasses that implement the abstract methods are the child classes.
from abc import ABC, abstractmethod
class Parent(ABC):
@abstractmethod
def common_method(self):
pass
class Child(Parent):
def common_method(self):
return 'Implemented in Child class'
child = Child()
print(child.common_method())
# Output:
# 'Implemented in Child class'
In this example, Parent
is an abstract class with an abstract method common_method
. Child
is a subclass of Parent
that provides an implementation for common_method
.
Encapsulation: Hiding the Complexity
Encapsulation is the principle of hiding the internal workings of an object and exposing only what is necessary. Abstract classes play a role in encapsulation by defining a clear interface that other classes can implement, hiding the complexity of the underlying implementation.
Polymorphism: One Interface, Many Implementations
Polymorphism is the ability of an object to take on many forms. With abstract classes, polymorphism is achieved through the implementation of the abstract methods in the subclasses. Each subclass can provide a different implementation, allowing for a variety of behaviors.
from abc import ABC, abstractmethod
class AbstractAnimal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(AbstractAnimal):
def make_sound(self):
return 'Woof!'
class Cat(AbstractAnimal):
def make_sound(self):
return 'Meow!'
dog = Dog()
cat = Cat()
print(dog.make_sound())
print(cat.make_sound())
# Output:
# 'Woof!'
# 'Meow!'
In this example, Dog
and Cat
are subclasses of the abstract class AbstractAnimal
and provide different implementations for the make_sound
method, demonstrating polymorphism.
In conclusion, abstract classes in Python play a crucial role in implementing the principles of OOP. They provide a blueprint for creating subclasses, encapsulate complexity, and enable polymorphism, making your code more structured, maintainable, and flexible.
Abstract Classes in the Wild: Real-World Applications
Abstract classes are not just theoretical constructs, but they have practical applications in larger projects and real-world scenarios. They are often used in software design patterns, such as the Template Method pattern, where an abstract class defines a ‘skeleton’ of an algorithm in an operation and defers some steps to subclasses.
from abc import ABC, abstractmethod
class AbstractClass(ABC):
def template_method(self):
self.primitive_operation1()
self.primitive_operation2()
@abstractmethod
def primitive_operation1(self):
pass
@abstractmethod
def primitive_operation2(self):
pass
class ConcreteClass(AbstractClass):
def primitive_operation1(self):
print('Primitive operation 1 implementation')
def primitive_operation2(self):
print('Primitive operation 2 implementation')
concrete = ConcreteClass()
concrete.template_method()
# Output:
# 'Primitive operation 1 implementation'
# 'Primitive operation 2 implementation'
In this example, AbstractClass
defines the template_method
which outlines the algorithm’s structure and calls primitive_operation1
and primitive_operation2
, which are abstract methods. The ConcreteClass
provides the specific implementations for these operations.
Related Topics: Exploring Further
As you continue to explore abstract classes, you’ll likely encounter related topics such as mixins, interfaces, and software design patterns. These concepts often accompany abstract classes in typical use cases and provide additional tools for structuring and organizing your code.
Further Resources for Mastering Python Abstract Classes
To deepen your understanding of abstract classes and related topics, here are some resources that offer more in-depth information:
- Python OOP Best Practices – Dive into inheritance in Python to build relationships between classes and reuse code.
Exploring the Object Class in Python – Learn about Python objects as instances of classes, representing real-world entities.
Understanding Classes in Python – Learn how to define and instantiate classes in Python for object-oriented programming.
Python’s Official Documentation on the ABC Module – Learn about Python’s abstract base class module from official sources.
Real Python’s Guide on Abstract Base Classes – Detailed explanation of abstract base classes in Python.
Python-Course’s Tutorial on Abstract Classes – Step-by-step guide to understanding abstract classes in Python.
These resources provide a wealth of information and examples that can help you master the use of abstract classes in Python and apply them effectively in your own projects.
Wrapping Up: Mastering Python Abstract Classes
In this comprehensive guide, we’ve navigated through the concept of abstract classes in Python. From understanding the basic use to exploring advanced techniques, we’ve covered the essential aspects of this powerful programming construct.
We started with the basics, learning how to create an abstract class using the abc
module. We then ventured into more advanced territory, discussing complex uses such as multiple inheritance and interface implementation.
Along the way, we tackled common challenges you might face when using abstract classes and provided solutions to help you overcome these hurdles.
We also looked at alternative approaches to creating abstract classes, such as using metaclasses and third-party libraries like zope.interface
. This gave us a broader understanding of the various ways to implement abstract classes in Python.
Here’s a quick comparison of these methods:
Method | Flexibility | Complexity | External Dependencies |
---|---|---|---|
abc module | Moderate | Low | None |
Metaclasses | High | High | None |
zope.interface | Moderate | Moderate | Yes |
Whether you’re a beginner just starting out with abstract classes or an experienced Python developer looking to level up your skills, we hope this guide has given you a deeper understanding of abstract classes in Python and how to use them effectively in your code. Happy coding!