Python Classes Usage Guide (With Examples)
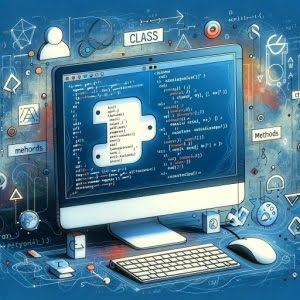
Ever found yourself grappling with the concept of Python classes? Don’t worry, you’re not alone. Many find this fundamental object-oriented programming concept a bit challenging at first. But think of a class as a blueprint for creating objects – a template if you will. It’s a crucial element in Python, and mastering it can open up a whole new world of coding efficiency and elegance.
In this comprehensive guide, we aim to demystify Python classes. We’ll start with the basics, helping you understand how to define and use classes in Python. Then, we’ll gradually delve into more advanced concepts, ensuring you have a solid grasp of Python classes. So, whether you’re a beginner just starting out or an intermediate looking to level up your Python skills, this guide has got you covered. Let’s dive in and unravel the mystery of Python classes together.
TL;DR: How Do I Create a Python Class?
Creating a class in Python is simple and straightforward. You can do so using the
class
keyword.
Here’s a quick example:
class MyClass:
x = 5
p1 = MyClass()
print(p1.x)
# Output:
# 5
In this code snippet, we define a class MyClass
with a property x
set to 5. Then, we create an object p1
of this class and print the value of x
using p1.x
, which outputs 5
.
This is just the tip of the iceberg when it comes to Python classes. Keep reading for a more detailed explanation and advanced usage examples. You’ll soon be a pro at handling Python classes!
Table of Contents
Defining a Class in Python
In Python, we define a class using the class
keyword. Let’s take a look at a simple example:
class MyClass:
x = 10
In the code above, we’ve defined a class named MyClass
with a property x
set to 10.
Creating an Object
Once we have a class defined, we can create objects or instances of this class. Here’s how:
p1 = MyClass()
In this line of code, p1
is an object of MyClass
.
Accessing Class Properties
After creating an object, we can access the properties of the class using the object. Here’s how to access the x
property using the p1
object:
print(p1.x)
# Output:
# 10
In the code snippet above, p1.x
accesses the x
property of the MyClass
through the p1
object, and print(p1.x)
prints the value of x
, which is 10
.
Understanding these basic concepts is the first step towards mastering Python classes. As you get more comfortable, you can start exploring more advanced topics like methods, inheritance, and more.
Exploring Python Class Methods
Once you’re comfortable with creating a class and accessing its properties, it’s time to dive into methods. In Python, methods are functions that belong to a class. Let’s add a method to our MyClass
:
class MyClass:
x = 10
def greet(self):
print("Hello, welcome to MyClass!")
p1 = MyClass()
p1.greet()
# Output:
# Hello, welcome to MyClass!
In this example, greet
is a method that prints a greeting. We call this method using the object p1
with p1.greet()
.
The __init__
Function
The __init__
function is a special method in Python classes. It serves as an initializer or constructor that Python automatically calls when creating a new instance of the class. Let’s see it in action:
class MyClass:
def __init__(self, name):
self.name = name
def greet(self):
print("Hello, " + self.name)
p1 = MyClass("Alice")
p1.greet()
# Output:
# Hello, Alice
In the __init__
method, we define a name
parameter and assign it to the name
property of the class. When creating the p1
object, we pass “Alice” as an argument, which is used as the name
for p1
. p1.greet()
then prints a personalized greeting.
Understanding Inheritance in Python
Inheritance allows us to define a class that inherits all the properties and methods from another class. The class being inherited from is called the parent class, and the class that inherits is the child class. Let’s create a child class that inherits from MyClass
:
class ChildClass(MyClass):
pass
p2 = ChildClass("Bob")
p2.greet()
# Output:
# Hello, Bob
Here, ChildClass
inherits from MyClass
, so it has access to the greet
method. p2 = ChildClass("Bob")
creates an instance of ChildClass
with the name “Bob”, and p2.greet()
prints a greeting to Bob.
These advanced concepts take you a step closer to mastering Python classes, enabling you to write more efficient and organized code.
Exploring Metaclasses in Python
Python offers even more advanced ways to work with classes, such as metaclasses. In Python, a metaclass is the class of a class, meaning it’s a class that creates and controls other classes, much like how classes create and control objects.
Here’s an example of a simple metaclass:
class Meta(type):
def __init__(cls, name, bases, attrs):
attrs['info'] = 'Added by Meta'
super().__init__(name, bases, attrs)
class MyClass(metaclass=Meta):
pass
p1 = MyClass()
print(p1.info)
# Output:
# Added by Meta
In this example, Meta
is a metaclass that adds an info
attribute to any class it creates. MyClass
is created with Meta
as its metaclass, so when we create an instance p1
of MyClass
and print p1.info
, it outputs Added by Meta
.
Metaclasses can be powerful, but they come with increased complexity and can lead to more difficult-to-find bugs.
Using Decorators with Python Classes
Decorators provide another alternative approach to work with classes in Python. A decorator is a function that takes another function and extends the behavior of the latter function without explicitly modifying it.
Here’s an example of a simple decorator applied to a class method:
def my_decorator(func):
def wrapper(self):
print('Before call')
result = func(self)
print('After call')
return result
return wrapper
class MyClass:
@my_decorator
def greet(self):
print("Hello, world!")
p1 = MyClass()
p1.greet()
# Output:
# Before call
# Hello, world!
# After call
In this example, my_decorator
is a decorator function that prints messages before and after the call to the function it decorates. By using @my_decorator
above the greet
method in MyClass
, we apply the decorator to that method. So, when we call p1.greet()
, it prints ‘Before call’, ‘Hello, world!’, and ‘After call’.
Decorators can make your code more readable and reusable, but they can also make debugging more challenging due to their implicit nature.
Whether you choose to use these advanced features like metaclasses and decorators depends on your specific needs, the complexity you’re willing to manage, and the trade-offs you’re willing to make.
Common Pitfalls and Solutions with Python Classes
While working with Python classes, you might encounter some common issues. Here, we’ll take a look at these potential obstacles and how to resolve them.
Undefined Class Error
One common error is trying to create an instance of a class that hasn’t been defined yet. Consider the following code:
p1 = MyClass()
# Output:
# NameError: name 'MyClass' is not defined
In this case, Python raises a NameError
because MyClass
hasn’t been defined yet. To fix this, you need to define MyClass
before creating an instance of it.
Misusing the self
Parameter
Another common mistake is forgetting the self
parameter in class methods. This parameter is a reference to the current instance of the class and is used to access class properties. Here’s an erroneous example:
class MyClass:
def __init__(self, name):
self.name = name
def greet():
print("Hello, " + self.name)
p1 = MyClass("Alice")
p1.greet()
# Output:
# TypeError: greet() takes 0 positional arguments but 1 was given
In this example, Python raises a TypeError
because the greet
method doesn’t have the self
parameter. To fix this, you need to include self
as the first parameter of the greet
method.
Best Practices and Optimization Tips
When working with Python classes, remember to follow these best practices for clean and efficient code:
- Use clear and descriptive names for your classes, methods, and properties.
- Keep your classes small and focused on a single responsibility.
- Use inheritance wisely to avoid unnecessary complexity.
- Document your classes and methods using docstrings.
By understanding these common pitfalls and best practices, you’ll be better equipped to use Python classes effectively and write more robust code.
Understanding Object-Oriented Programming
Object-oriented programming (OOP) is a programming paradigm that uses ‘objects’ to design applications and software. These objects are instances of classes, which can include both data in the form of properties (also known as attributes), and code, in the form of methods (functions associated with an object).
Here’s an example of a class, which is a blueprint for creating objects in Python:
class MyClass:
x = 5
p1 = MyClass()
print(p1.x)
# Output:
# 5
In this example, MyClass
is a class with a property x
set to 5. p1
is an object of MyClass
, and p1.x
prints the value of x
.
Objects and Methods in Python
In Python, everything is an object. This includes integers, strings, lists, and even functions and classes themselves. Objects are instances of a class, which define a set of attributes and methods.
Methods are functions defined within a class that perform a specific action or computation. They can access and modify the data within an object:
class MyClass:
def greet(self):
print("Hello, world!")
p1 = MyClass()
p1.greet()
# Output:
# Hello, world!
In this example, greet
is a method of MyClass
, and p1.greet()
calls this method, printing ‘Hello, world!’.
The Power of Inheritance
Inheritance is a powerful feature of OOP that promotes code reuse. A class can inherit properties and methods from another class, allowing you to create a general class first and then later define subclasses that implement more specific behavior:
class Parent:
def greet(self):
print("Hello, world!")
class Child(Parent):
pass
p1 = Child()
p1.greet()
# Output:
# Hello, world!
Here, Child
is a subclass of Parent
and inherits its greet
method. So, calling p1.greet()
prints ‘Hello, world!’.
By understanding these fundamental concepts of OOP in Python, you’ll have a solid foundation to effectively use and understand Python classes.
Python Classes in Larger Projects
Python classes are not just theoretical constructs, but practical tools that play a pivotal role in larger projects. They provide structure, facilitate code reuse, and can make your code more readable and maintainable. For example, in a web application, you might have classes representing users, blog posts, or database connections.
class User:
def __init__(self, username, email):
self.username = username
self.email = email
user1 = User('Alice', '[email protected]')
print(user1.username)
# Output:
# Alice
In this code snippet, we define a User
class with username
and email
properties. We then create a user1
instance of the User
class. This is a simple example, but in a real-world application, the User
class might have additional properties and methods for handling user authentication, permissions, and other features.
Expanding Your Knowledge: Modules and Packages
While mastering Python classes is a significant milestone, it’s just the beginning of your Python journey. To further enhance your Python skills, consider exploring related topics like modules and packages.
- Modules: In Python, a module is a file containing Python definitions and statements. It’s a way of organizing related code into a single file, which can then be imported and used in other programs.
Packages: A package is a way of organizing related modules into a directory hierarchy. It’s essentially a directory that contains multiple module files and a special
__init__.py
file to indicate that it’s a package.
These concepts often go hand-in-hand with classes, especially in larger projects. They allow you to organize your classes and other code in a more structured manner, making it easier to manage and maintain your codebase.
Further Resources
For more in-depth information about the aforementioned topics, consider checking out the following guides on Python.
- Exploring Object-Oriented Programming in Python – Master the concepts of classes, objects, and inheritance in Python to design robust and scalable applications.
Exploring Abstract Classes in Python – Master Python abstract class usage for implementing polymorphism and code organization.
Python Counter: Quick Reference Guide – Explore Python Counter for efficient counting and frequency analysis of elements.
Python Classes and Objects – Deep dive into Python classes and objects with GeeksforGeeks.
Python’s Official Documentation on Classes – Comprehensive guide to Python’s classes from official sources.
Python Classes Tutorial – Learn about Python classes in an easy manner with W3Schools.
These resources offer detailed explanations, practical examples, and useful tips to help you get the most out of powerful Python features.
Python Classes: Key Takeaways
To wrap things up, let’s summarize the key points about Python classes we’ve covered in this guide:
- Definition: A Python class is a blueprint for creating objects. It encapsulates attributes and methods that define the properties and behaviors of the objects created from it.
Basic Usage: You can define a class using the
class
keyword, create an object from it, and access its properties.
class MyClass:
x = 5
p1 = MyClass()
print(p1.x)
# Output:
# 5
Advanced Usage: Python classes support more complex concepts like methods, the
__init__
function, and inheritance, allowing you to write more efficient and organized code.Alternative Approaches: Python offers advanced features like metaclasses and decorators to work with classes, but they come with increased complexity.
Troubleshooting: Common issues when working with Python classes include undefined classes and misuse of the
self
parameter. Understanding these pitfalls can help you write more robust code.Beyond: Python classes play a pivotal role in larger projects, providing structure and facilitating code reuse. Related topics like modules and packages often go hand-in-hand with classes.
By mastering Python classes, you’re taking a significant step in your Python journey. Whether you’re a beginner just starting out or an intermediate looking to level up your Python skills, understanding Python classes is a valuable asset that will serve you well in your coding projects.