Java Bean Explained: Object Encapsulation Guide
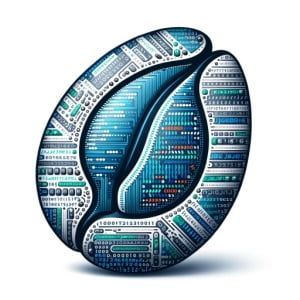
Ever felt overwhelmed when trying to understand Java Beans? You’re not alone. Many developers find the concept of Java Beans a bit complex. But, think of a Java Bean as a Lego block in a larger construction – it’s a reusable software component in Java that can be used to build bigger and more complex applications.
Java Beans, like Lego blocks, can be assembled in various ways to create a wide range of structures (or in this case, applications). They provide a standard way to encapsulate many objects into a single object, the Bean, making your code cleaner and easier to manage.
In this guide, we’ll demystify Java Beans, helping you understand what they are, how to use them, and why they are important in Java programming. We’ll start from the basics and gradually delve into more advanced usage scenarios, discussing their advantages, potential pitfalls, and how to troubleshoot common issues.
So, let’s dive in and start mastering Java Beans!
TL;DR: What is a Java Bean?
A Java Bean is a reusable software component in Java
. It is a class that encapsulates many objects into a single object (the bean), and can be instantiated the same way as a normal Java class,public class MyBean { //getters, setters, and methods for the class}
. It’s a way to create reusable components for your Java applications.
Here’s a simple example of a Java Bean:
public class MyBean {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
}
In this example, MyBean
is a Java Bean. It has a private field name
and two public methods: getName()
and setName(String name)
. These methods are known as getter and setter methods, and they are used to retrieve and update the value of name
, respectively.
This is a basic introduction to Java Beans, but there’s much more to learn about creating and using them effectively. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Creating a Java Bean: The Basics
- Java Beans in JSPs and Servlets
- Exploring Alternatives: POJOs and EJBs
- Troubleshooting Java Beans: Common Errors and Solutions
- The Concept of Encapsulation in Java
- Java Beans in Larger Projects
- Complementary Concepts to Java Beans
- Java Beans and Microservices
- Wrapping Up: Effective Java Programming with Java Beans
Creating a Java Bean: The Basics
To create a Java Bean, you need to follow some rules. A Java Bean is a class that:
- Is serializable (implements the
Serializable
interface). - Has a public no-argument constructor.
- Provides methods to set and get the values of properties (known as setter and getter methods).
Let’s create a simple Java Bean to understand how it works.
import java.io.Serializable;
public class StudentBean implements Serializable {
private String name;
private int age;
public StudentBean() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
In this example, StudentBean
is a Java Bean. It implements the Serializable
interface, has a public no-argument constructor, and provides getter and setter methods for its properties name
and age
.
The advantages of using Java Beans include code reusability, encapsulation of logic, and easy interaction with other components. However, it’s worth noting that Java Beans can sometimes lead to ‘over-encapsulation’, where simple operations are wrapped into unnecessary methods, leading to bloated code.
Java Beans in JSPs and Servlets
Java Beans can be used effectively in JSPs (JavaServer Pages) and Servlets, two key technologies in the world of Java web applications. They can be used to encapsulate data that can be reused across multiple JSPs or Servlets.
Consider the following example where a Java Bean is used in a JSP:
<%@ page import="com.example.StudentBean" %>
<jsp:useBean id="student" class="com.example.StudentBean" />
<jsp:setProperty name="student" property="name" value="John Doe" />
<jsp:setProperty name="student" property="age" value="22" />
Student Name: <jsp:getProperty name="student" property="name" />
Student Age: <jsp:getProperty name="student" property="age" />
In this example, we’re using the StudentBean
we defined earlier. The jsp:useBean
tag is used to instantiate the Bean, and the jsp:setProperty
tags are used to set the values of the Bean’s properties. Finally, the jsp:getProperty
tags are used to retrieve and display these values.
Using Java Beans in JSPs and Servlets can greatly simplify your code, making it cleaner and easier to maintain. However, as your applications get more complex, you might find that Java Beans are not flexible enough to meet your needs. In such cases, you might want to consider using more powerful alternatives such as POJOs (Plain Old Java Objects) or EJBs (Enterprise JavaBeans).
Exploring Alternatives: POJOs and EJBs
Java offers other concepts that can be used as alternatives to Java Beans, such as POJOs (Plain Old Java Objects) and EJBs (Enterprise JavaBeans). These concepts offer more flexibility and functionality, making them suitable for more complex applications.
Plain Old Java Objects (POJOs)
A POJO is a simple Java object that doesn’t extend or implement some specialized classes or interfaces in the Java framework. Here’s an example of a POJO:
public class StudentPOJO {
private String name;
private int age;
// Constructor, getters, and setters omitted for brevity
}
In this example, StudentPOJO
is a POJO. It’s similar to our Java Bean example, but it doesn’t implement the Serializable
interface. POJOs offer the advantage of simplicity and flexibility, but they lack some of the features provided by Java Beans, such as event handling and persistence.
Enterprise JavaBeans (EJBs)
EJBs are a part of the Java EE (Enterprise Edition) framework and provide a robust architecture for building large-scale, distributed applications. Here’s an example of a session EJB:
import javax.ejb.Stateless;
@Stateless
public class StudentEJB {
public String getStudentDetails() {
return "John Doe, 22";
}
}
In this example, StudentEJB
is a stateless session EJB. It provides a method getStudentDetails()
that returns a string. EJBs offer advanced features such as transaction management and security, but they are more complex and heavier than Java Beans and POJOs.
When deciding which concept to use, consider the complexity of your application, the features you need, and the resources you have. Java Beans are great for simple, small-scale applications, while POJOs offer more flexibility and EJBs are suitable for large-scale, enterprise applications.
Troubleshooting Java Beans: Common Errors and Solutions
While working with Java Beans, you might encounter some common issues. Here are a few and how to solve them:
Error: Bean Not Serializable
If your Java Bean doesn’t implement the Serializable
interface, you might encounter a java.io.NotSerializableException
when you try to serialize it. Here’s an example:
public class NonSerializableBean {
private String name;
// Constructor, getters, and setters omitted for brevity
}
If you try to serialize an instance of NonSerializableBean
, you’ll get an exception:
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
public class Main {
public static void main(String[] args) {
try (ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream("bean.ser"))) {
NonSerializableBean bean = new NonSerializableBean();
oos.writeObject(bean);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, the main
method tries to serialize an instance of NonSerializableBean
. It results in a java.io.NotSerializableException
.
To fix this, make sure your Java Bean implements the Serializable
interface:
import java.io.Serializable;
public class SerializableBean implements Serializable {
private String name;
// Constructor, getters, and setters omitted for brevity
}
Error: No Public No-Argument Constructor
If your Java Bean doesn’t have a public no-argument constructor, some frameworks (like JSP) might not be able to instantiate it. Make sure your Java Bean has a public no-argument constructor, even if it’s empty.
Best Practices and Optimization Tips
- Keep your Java Beans simple. Remember, they are meant to encapsulate data, not complex business logic.
- Use meaningful names for your Java Beans and their properties. This makes your code easier to read and maintain.
- Consider making your Java Bean immutable if its state doesn’t need to change after it’s created. This can make your code safer and easier to reason about.
The Concept of Encapsulation in Java
One of the core principles of Object-Oriented Programming (OOP) is encapsulation. Encapsulation is the mechanism of hiding data and behavior within a single unit, a class in Java. It helps to safeguard the data from being accessed directly, maintaining the integrity of the objects.
Encapsulation and Java Beans
Java Beans are a prime example of encapsulation. They encapsulate many objects into a single object (the bean), providing methods to access and modify these objects. This is done using getter and setter methods. Here’s a simple Java Bean that demonstrates encapsulation:
public class EncapsulatedBean {
private String secretData;
public String getSecretData() {
return secretData;
}
public void setSecretData(String secretData) {
this.secretData = secretData;
}
}
In this example, the EncapsulatedBean
class encapsulates a secretData
field. The getSecretData()
and setSecretData(String secretData)
methods are the only ways to access and modify secretData
. This ensures that secretData
can’t be changed arbitrarily, protecting its integrity.
Other Related Concepts
While Java Beans are a great tool for encapsulation, Java offers other concepts that provide similar functionality. For instance, POJOs (Plain Old Java Objects) also encapsulate data and behavior, but they don’t have to follow the strict rules of Java Beans. Similarly, EJBs (Enterprise JavaBeans) provide a more robust mechanism for encapsulation, suitable for large-scale, enterprise applications.
Understanding encapsulation and how it relates to Java Beans is crucial for effective Java programming. It allows you to write cleaner, more secure code, and is a fundamental concept in OOP.
Java Beans in Larger Projects
Java Beans play a crucial role in larger projects and architectures. Their reusability makes them ideal for creating complex applications where certain components need to be used repeatedly. They can be used to build modular applications, where each module is a Java Bean that can be plugged into the application as needed.
Complementary Concepts to Java Beans
When working with Java Beans, you might also encounter other related concepts. For instance, in web applications, Java Beans often work hand in hand with Servlets and JSPs. In enterprise applications, you might find Java Beans being used alongside EJBs (Enterprise JavaBeans) and JPA (Java Persistence API).
Java Beans and Microservices
In the world of microservices, Java Beans can be used to encapsulate the data that is sent between services. This can simplify the communication between services and make your microservices architecture easier to manage.
Further Resources for Java Beans
To further your understanding of Java Beans and related concepts, here are some resources you might find useful:
- Java Packages: A Quick Overview – Learn how to package and distribute Java applications for deployment.
Apache POI Overview – Discover Apache POI, a Java library for reading and writing Microsoft Office documents.
Understanding Java Swing – Learn how to build desktop graphical user interfaces (GUIs) with Swing.
Oracle’s JavaBeans Tutorial covers the basics of Java Beans and how to use them in your applications.
JavaBeans Tutorial by University of Washington provides a comprehensive guide to JavaBeans.
JSP JavaBeans by Tutorialspoint explains how to use JavaBeans in JSP (JavaServer Pages) applications.
Wrapping Up: Effective Java Programming with Java Beans
In this comprehensive guide, we’ve delved into the world of Java Beans, a cornerstone of Java programming that enables the creation of reusable software components.
We began with the basics, exploring what a Java Bean is and how to create one. We then advanced to using Java Beans in JSPs and Servlets, showcasing their role in the development of Java web applications. To ensure you’re well-equipped to tackle any challenges, we discussed common issues you might encounter when working with Java Beans and provided solutions for these problems.
Beyond the basics, we explored alternative approaches to Java Beans, such as POJOs and EJBs. These alternatives offer more flexibility and functionality, making them suitable for more complex applications. Here’s a quick comparison of these methods:
Method | Flexibility | Functionality | Complexity |
---|---|---|---|
Java Beans | Moderate | Moderate | Low |
POJOs | High | Low | Low |
EJBs | High | High | High |
Whether you’re a beginner just starting out with Java Beans, or an experienced developer looking to refine your skills, we hope this guide has given you a deeper understanding of Java Beans and their role in Java programming.
With their balance of reusability, encapsulation, and ease of use, Java Beans are a powerful tool in your Java programming toolkit. Happy coding!