JavaScript Arrays Explained: Your Ultimate Guide
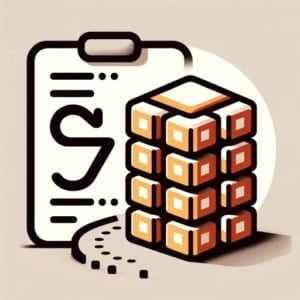
Are you finding JavaScript arrays a bit puzzling? You’re not alone. Many developers, especially beginners, find arrays in JavaScript a bit challenging to grasp. Think of JavaScript arrays as a versatile toolbox – a way to store multiple values in a single variable, providing a powerful tool for various tasks.
In this guide, we’ll walk you through the process of working with JavaScript arrays, from their creation, manipulation, and usage. We’ll cover everything from the basics of arrays to more advanced techniques, as well as alternative approaches.
So, let’s dive in and start mastering JavaScript arrays!
TL;DR: What is a JavaScript Array and How Do I Use It?
A JavaScript array is a type of variable that can hold multiple values. It’s like a list that can store multiple elements, and these elements can be of any data type, including numbers, strings, and objects.
Here’s a simple example of creating and using a JavaScript array:
let fruits = ['apple', 'banana', 'cherry'];
console.log(fruits[0]);
// Output:
// 'apple'
In this example, we’ve created an array named fruits
that holds three string values. We then use console.log(fruits[0]);
to print the first element of the array, which is ‘apple’.
This is just a basic way to use JavaScript arrays, but there’s much more to learn about creating, manipulating, and using arrays in JavaScript. Continue reading for a more detailed understanding and advanced usage scenarios.
Table of Contents
- Creating and Using JavaScript Arrays: A Beginner’s Guide
- Mastering Array Methods in JavaScript
- Exploring Alternative Data Structures in JavaScript
- Troubleshooting Common JavaScript Array Issues
- The Essence of Arrays in Programming
- Applying JavaScript Arrays in Larger Projects
- Further Resources for Mastering JavaScript Arrays
- Wrapping Up: JavaScript Arrays
Creating and Using JavaScript Arrays: A Beginner’s Guide
In JavaScript, creating an array is relatively straightforward. You can declare an array using square brackets []
, and you can populate it with values separated by commas. These values can be of any type – numbers, strings, objects, even other arrays.
Here’s a basic example of creating an array in JavaScript:
let numbers = [1, 2, 3, 4, 5];
console.log(numbers);
// Output:
// [1, 2, 3, 4, 5]
In the example above, we’ve created an array named numbers
that holds five numeric values. We then log the entire array to the console, and as expected, it outputs [1, 2, 3, 4, 5]
.
But how do we access individual elements in an array? JavaScript arrays are zero-indexed, meaning the first element is at index 0, the second element is at index 1, and so on. Here’s how you can access array elements:
let numbers = [1, 2, 3, 4, 5];
console.log(numbers[0]);
console.log(numbers[3]);
// Output:
// 1
// 4
In this example, numbers[0]
returns the first element of the array (1), and numbers[3]
returns the fourth element (4).
Arrays in JavaScript are dynamic, meaning you can change their size and the type of data they hold. This flexibility makes arrays a powerful tool for storing and organizing data in your code. However, this dynamism can also lead to potential pitfalls, such as accessing an index that doesn’t exist, which will return undefined
, or trying to perform operations on incompatible data types stored within the same array.
Mastering Array Methods in JavaScript
As you become more comfortable with JavaScript arrays, it’s time to explore some of the more advanced methods that can make your life easier. These methods allow you to manipulate arrays in more complex ways, such as adding or removing elements, or iterating over each element in the array.
The Push and Pop Methods
The push()
method allows you to add one or more elements to the end of an array. On the other hand, the pop()
method removes the last element from an array.
let fruits = ['apple', 'banana', 'cherry'];
fruits.push('orange');
console.log(fruits);
// Output:
// ['apple', 'banana', 'cherry', 'orange']
fruits.pop();
console.log(fruits);
// Output:
// ['apple', 'banana', 'cherry']
In the example above, we added ‘orange’ to the end of the fruits
array using push()
, and then removed it using pop()
.
The Shift and Unshift Methods
The shift()
method removes the first element from an array, while the unshift()
method adds one or more elements to the beginning of an array.
let fruits = ['apple', 'banana', 'cherry'];
fruits.unshift('orange');
console.log(fruits);
// Output:
// ['orange', 'apple', 'banana', 'cherry']
fruits.shift();
console.log(fruits);
// Output:
// ['apple', 'banana', 'cherry']
In this example, we added ‘orange’ to the start of the fruits
array using unshift()
, and then removed it using shift()
.
These methods provide a powerful way to manipulate JavaScript arrays, allowing you to add or remove elements from either end of the array. However, it’s important to remember that these methods mutate the original array, so use them with caution if you need to preserve the original array.
Exploring Alternative Data Structures in JavaScript
While arrays are an essential part of JavaScript, they are not the only way to store and manipulate data. As you advance in your JavaScript journey, you’ll encounter alternative data structures like objects and sets, which offer unique advantages and considerations.
Objects: Key-Value Pairs
An object in JavaScript is a collection of key-value pairs. Objects are useful when you want to store data in a structured way, where each value can be accessed via a unique key.
let student = {
name: 'John',
age: 20,
grade: 'A'
};
console.log(student.name);
// Output:
// 'John'
In this example, we created an object student
with three properties: name
, age
, and grade
. We can access each property using dot notation, as shown with student.name
.
Sets: Unique Values
A Set is a special type of object in JavaScript that only allows unique values. If you try to add a duplicate value to a Set, it will be ignored.
let uniqueNumbers = new Set([1, 2, 3, 1, 2]);
console.log(uniqueNumbers);
// Output:
// Set(3) { 1, 2, 3 }
In the example above, we created a Set uniqueNumbers
and tried to add duplicate numbers 1 and 2. The Set ignored the duplicates and only stored the unique numbers.
While these alternative data structures offer some benefits over arrays, they also have their drawbacks. Objects require you to know the key to access a value, which can be less intuitive than accessing an array by index. Sets only store unique values, which can be limiting if you need to store duplicates. As with most things in programming, the best tool depends on the specific requirements of your task.
Troubleshooting Common JavaScript Array Issues
While JavaScript arrays are incredibly useful, they can also lead to some common pitfalls if not handled correctly. In this section, we’ll discuss some of these issues and how to address them.
Accessing Non-Existent Indexes
One common mistake is trying to access an index that doesn’t exist in the array. This will return undefined
, which can lead to unexpected results in your code.
let fruits = ['apple', 'banana', 'cherry'];
console.log(fruits[3]);
// Output:
// undefined
In the example above, we tried to access the element at index 3 in the fruits
array. However, since the array only has three elements (with indexes 0, 1, and 2), there is no element at index 3, and JavaScript returns undefined
.
Modifying Arrays While Iterating Over Them
Another common pitfall is modifying an array while iterating over it. This can lead to unexpected results, as the array’s length and element indexes may change during the iteration.
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
numbers.splice(i, 1);
}
}
console.log(numbers);
// Output:
// [1, 3, 5]
In the example above, we tried to remove all even numbers from the numbers
array by iterating over it and using splice()
to remove elements. However, since splice()
changes the array’s length and the indexes of the remaining elements, the iteration skips some elements, resulting in an incorrect final array.
Understanding these common pitfalls can help you avoid them in your code and use JavaScript arrays more effectively and efficiently.
The Essence of Arrays in Programming
Arrays are fundamental to almost all programming languages, not just JavaScript. But what makes them so essential?
What is an Array?
An array is a data structure that can store a fixed-size sequence of elements of the same type. An array is used to store a collection of data, but it’s more useful to think of an array as a collection of variables of the same type.
let numbers = [1, 2, 3, 4, 5];
console.log(numbers);
// Output:
// [1, 2, 3, 4, 5]
In the example above, numbers
is an array that stores five integers. Instead of declaring five separate variables, we can store all these numbers in one array.
Why are Arrays Important?
Arrays are important because they allow us to store multiple values in a single variable, which can greatly simplify our code. They also provide us with a range of built-in methods to manipulate the data, such as sorting the elements, iterating over each element, or finding a specific element.
How are Arrays Implemented in JavaScript?
In JavaScript, arrays are dynamic, meaning they can change size as we add or remove elements. They can also hold elements of different types, unlike arrays in many other languages. This flexibility makes JavaScript arrays a powerful tool for many tasks, from storing a list of items to representing a grid in a game.
Applying JavaScript Arrays in Larger Projects
JavaScript arrays are not just for small scripts or simple tasks. They play a crucial role in larger projects, where organizing and manipulating data effectively becomes paramount. You’ll find arrays used in everything from managing user data, to controlling game logic, to manipulating the DOM in web development.
Arrays in Web Development
In web development, JavaScript arrays are often used to store and manipulate data from user inputs, API responses, or DOM elements. For instance, you might use an array to store a list of blog posts fetched from an API, then use array methods like map()
to generate the HTML for each post.
Arrays in Game Development
In game development, arrays can represent game boards, store game states, or manage in-game entities. For example, a 2D array can represent a grid in a game, where each cell in the array corresponds to a cell on the game board.
Arrays in Data Processing
In data processing scripts, arrays can store large amounts of data for analysis or transformation. You might use an array to store a series of temperature readings, then use array methods like reduce()
to calculate the average temperature.
Further Resources for Mastering JavaScript Arrays
To continue your journey in mastering JavaScript arrays, consider exploring these additional resources:
- MDN Web Docs: Array – A comprehensive guide to JavaScript arrays from the Mozilla Developer Network.
- JavaScript.info: Arrays – A detailed tutorial on JavaScript arrays, including methods and properties.
- Eloquent JavaScript: Data Structures: Objects and Arrays – A chapter from the book Eloquent JavaScript that covers objects and arrays.
Wrapping Up: JavaScript Arrays
In this comprehensive guide, we’ve delved into the world of JavaScript arrays, covering everything from basic creation and usage to advanced methods and alternative data structures.
We started with the basics, explaining how to create a JavaScript array and access its elements. We then moved on to more advanced topics, such as using methods like push()
, pop()
, shift()
, and unshift()
to manipulate arrays. We also discussed alternative ways to store and manipulate data in JavaScript, such as using objects and sets.
Along the way, we addressed common issues you might encounter when working with JavaScript arrays, such as accessing non-existent indexes or modifying arrays while iterating over them, and provided solutions to these problems.
Here’s a quick comparison of the methods and data structures we’ve discussed:
Method/Data Structure | Pros | Cons |
---|---|---|
JavaScript Array | Versatile, dynamic, supports multiple data types | Potential pitfalls like accessing non-existent indexes |
Array Methods (push, pop, etc.) | Powerful for manipulating arrays | Can mutate the original array |
Objects | Structured data storage, access values via unique keys | Less intuitive than accessing an array by index |
Sets | Only allows unique values | Cannot store duplicates |
Whether you’re a beginner just starting out with JavaScript arrays, or an experienced developer looking to brush up on your skills, we hope this guide has given you a deeper understanding of JavaScript arrays and their capabilities.
With their versatility and the powerful methods available for manipulating them, JavaScript arrays are an essential tool in any JavaScript developer’s toolbox. Happy coding!