Bash Scripting Guide: How to Ensure Exit on Error
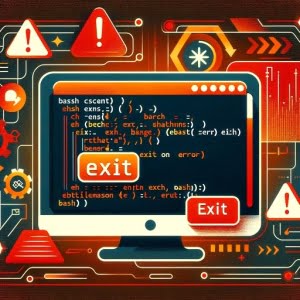
Are you finding it challenging to ensure your bash scripts exit when an error occurs? You’re not alone. Many developers find themselves puzzled when it comes to handling errors in bash scripts, but we’re here to help.
Think of bash scripts as a cautious driver – they can be programmed to stop at the first sign of trouble. This feature can be a lifesaver, preventing the execution of further commands that may lead to undesirable outcomes or even system damage.
In this guide, we’ll walk you through the process of making bash scripts exit on error, from basic use to advanced techniques. We’ll cover everything from the simple ‘set -e’ command to more complex methods involving ‘trap’ and specific exit codes.
Let’s get started and master the art of exiting bash scripts on error!
TL;DR: How Do I Make a Bash Script Exit on Error?
The simplest way to make a bash script exit when an error occurs is by using the
'set -e'
command at the beginning of your bash script. This command instructs the bash script to stop execution if any command it runs returns a non-zero exit status, which is the convention for indicating an error in bash.
Here’s a simple example:
#!/bin/bash
set -e
command1
command2
# Output:
# If 'command1' fails, the script will stop executing and will not run 'command2'.
In this script, the ‘set -e’ command is used right after the shebang line. This means that if ‘command1’ fails (i.e., returns a non-zero exit status), the script will immediately stop, and ‘command2’ will not be executed.
This is just the tip of the iceberg when it comes to handling errors in bash scripts. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- The Basics: Using ‘set -e’ in Bash Scripts
- Advanced Error Handling: The ‘trap’ Command
- Exploring Alternatives: ‘set -o pipefail’ and ‘set -u’
- Navigating Common Challenges in Bash Error Handling
- Understanding the Fundamentals: Bash Scripting, Error Handling, and Exit Statuses
- Going Beyond: The Relevance of Error Handling in Larger Projects
- Wrapping Up: Mastering Bash Exit on Error
The Basics: Using ‘set -e’ in Bash Scripts
The ‘set -e’ command is a fundamental part of error handling in bash scripts. It instructs the script to terminate as soon as any command it executes fails, i.e., returns a non-zero exit status. This is a straightforward and efficient way to prevent further execution when an error is encountered.
Let’s look at an example to better understand how it works:
#!/bin/bash
set -e
# This command will pass
echo "This will run"
# This command will fail
ls /nonexistentdirectory
# This command will not run
echo "This won't run"
# Output:
# 'This will run'
# ls: cannot access '/nonexistentdirectory': No such file or directory
In this script, the ‘echo’ command prints ‘This will run’, and then the ‘ls’ command tries to list the contents of a directory that doesn’t exist, which causes it to fail. Because we’ve used ‘set -e’, the script stops executing at this point, and the final ‘echo’ command does not get run.
While ‘set -e’ is a powerful command and great for simple scripts, it’s not without its potential pitfalls. For instance, some commands return a non-zero exit status not because they’ve failed, but because of their specific functionality. In such cases, ‘set -e’ might cause your script to exit prematurely. Therefore, it’s crucial to understand the commands you’re using in your script and how they behave.
In the coming sections, we will explore more advanced techniques for error handling in bash scripts.
Advanced Error Handling: The ‘trap’ Command
As we progress in our journey of understanding bash exit on error, let’s introduce a more advanced technique: the ‘trap’ command. ‘trap’ allows us to specify commands to be executed when certain signals are received by the script. This can be particularly useful for dealing with specific error codes.
Let’s look at an example to illustrate how ‘trap’ can be used:
#!/bin/bash
handle_error() {
echo "An error occurred on line $1"
exit 1
}
trap 'handle_error $LINENO' ERR
# This command will pass
echo "This will run"
# This command will fail
ls /nonexistentdirectory
# This command will not run
echo "This won't run"
# Output:
# 'This will run'
# ls: cannot access '/nonexistentdirectory': No such file or directory
# An error occurred on line 12
In this script, we’ve defined a function called handle_error
that takes one argument, the line number where the error occurred. This function is called whenever an ERR signal is received, which happens whenever a command fails. As a result, when the ‘ls’ command fails, our handle_error
function is called, and the script exits with a message indicating the line on which the error occurred.
Using ‘trap’ in this way provides more control over error handling compared to ‘set -e’. It allows for more detailed error messages and gives us the ability to handle errors in a more granular way. However, it’s also more complex and can make scripts more difficult to read and maintain, especially for beginners. Therefore, it’s often best used in larger scripts where advanced error handling is necessary.
Exploring Alternatives: ‘set -o pipefail’ and ‘set -u’
While ‘set -e’ and ‘trap’ are powerful tools for handling errors in bash scripts, they aren’t the only options available. Two other commands that can be particularly useful are ‘set -o pipefail’ and ‘set -u’.
Using ‘set -o pipefail’
The ‘set -o pipefail’ command ensures that your script exits if any command in a pipeline fails, not just the last one. This can be useful in scripts where you’re chaining together multiple commands using pipes.
Let’s look at an example:
#!/bin/bash
set -o pipefail
echo "This will run"
echo "This will fail" | ls /nonexistentdirectory
echo "This won't run"
# Output:
# 'This will run'
# ls: cannot access '/nonexistentdirectory': No such file or directory
In this script, the ‘echo “This will fail”‘ command is piped into ‘ls /nonexistentdirectory’. Because we’ve used ‘set -o pipefail’, the script stops executing when the ‘ls’ command fails, and ‘echo “This won’t run”‘ is not executed.
Using ‘set -u’
The ‘set -u’ command causes your script to exit if it tries to use an uninitialized variable. This can help catch typos and other bugs related to variables.
Here’s an example:
#!/bin/bash
set -u
echo "This will run"
echo $nonexistentvariable
echo "This won't run"
# Output:
# 'This will run'
# ./script.sh: line 5: nonexistentvariable: unbound variable
In this script, the ‘echo $nonexistentvariable’ line tries to echo a variable that hasn’t been initialized. Because we’ve used ‘set -u’, the script stops executing at this point, and ‘echo “This won’t run”‘ is not executed.
Both ‘set -o pipefail’ and ‘set -u’ provide additional ways to catch errors and prevent your script from executing further. They are especially useful in larger scripts where errors might be harder to track down. However, they should be used judiciously, as they can also make scripts more complex and harder to understand for beginners.
Handling errors in bash scripts isn’t always straightforward. There are potential issues that you might encounter, especially when using the ‘set -e’, ‘trap’, ‘set -o pipefail’, and ‘set -u’ commands. Let’s discuss some of these common challenges and their solutions.
Ignoring Errors in Certain Lines
Sometimes, you might want to ignore errors from specific commands. You can achieve this by appending ‘|| true’ to the end of the command. Here’s an example:
#!/bin/bash
set -e
echo "This will run"
ls /nonexistentdirectory || true
echo "This will run too"
# Output:
# 'This will run'
# ls: cannot access '/nonexistentdirectory': No such file or directory
# 'This will run too'
In this script, even though the ‘ls’ command fails, the script doesn’t exit because of the ‘|| true’ at the end of the line. The final ‘echo’ command is executed as expected.
Dealing with ‘set -e’ in Functions
Another common issue is that ‘set -e’ doesn’t work in certain contexts, such as within functions or subshells. In these cases, errors might not cause the script to exit as expected. Here’s an example to illustrate this:
#!/bin/bash
set -e
failing_function() {
ls /nonexistentdirectory
}
echo "This will run"
failing_function || true
echo "This will run too"
# Output:
# 'This will run'
# ls: cannot access '/nonexistentdirectory': No such file or directory
# 'This will run too'
In this script, the ‘ls’ command within the function fails, but the script doesn’t exit because the function call is followed by ‘|| true’.
These are just a couple of the potential issues you might encounter when trying to make bash scripts exit on error. Understanding these challenges and their solutions can help you write more robust and reliable scripts.
Understanding the Fundamentals: Bash Scripting, Error Handling, and Exit Statuses
To fully grasp the concept of ‘exit on error’ in bash scripts, it’s essential to understand some fundamental concepts: bash scripting, error handling, and exit statuses.
Bash Scripting Basics
A bash script is a plain text file containing a series of commands. These scripts are executed by the bash shell, and they allow us to automate tasks that would otherwise require manual input.
#!/bin/bash
# This is a simple bash script
echo "Hello, world!"
# Output:
# 'Hello, world!'
In this basic script, the ‘echo’ command prints the string ‘Hello, world!’ to the terminal.
Error Handling in Bash
Error handling is a critical aspect of programming. In bash, we handle errors by checking the exit status of commands. By convention, a command returns a zero exit status if it succeeds and a non-zero status if it fails. We can use this information to make our bash scripts more robust and reliable.
#!/bin/bash
# Attempt to create a directory
mkdir /tmp/mydirectory
# Check if the command succeeded
if [ $? -eq 0 ]; then
echo "Directory created successfully"
else
echo "Failed to create directory"
fi
# Output:
# 'Directory created successfully' or 'Failed to create directory'
In this script, we attempt to create a directory using the ‘mkdir’ command. We then check the exit status of this command using ‘$?’. If the exit status is zero, we print a success message. Otherwise, we print an error message.
Understanding Exit Statuses
An exit status is a number returned by a command to indicate its success or failure. By convention, a zero exit status indicates success, while a non-zero exit status indicates failure. Understanding exit statuses is crucial for error handling in bash scripts.
#!/bin/bash
# This command will succeed
echo "This will run"
echo $? # Prints the exit status of the previous command
# This command will fail
ls /nonexistentdirectory
echo $? # Prints the exit status of the previous command
# Output:
# 'This will run'
# 0
# ls: cannot access '/nonexistentdirectory': No such file or directory
# 2
In this script, we run two commands and print their exit statuses. The ‘echo’ command succeeds, so its exit status is zero. The ‘ls’ command fails, so its exit status is non-zero.
Understanding these fundamentals will give you a solid foundation for mastering ‘exit on error’ in bash scripts.
Going Beyond: The Relevance of Error Handling in Larger Projects
Error handling in bash scripts is not just relevant for simple scripts; it becomes even more crucial when you’re dealing with larger scripts or projects. Robust error handling can prevent your script from executing harmful commands when an error occurs, saving you from potential data loss or system damage.
Debugging in Bash
One related concept you might want to explore is debugging in bash. Debugging is the process of identifying and fixing errors in your script. Bash provides several tools for debugging, such as the ‘-x’ option that prints each command before it’s executed, which can help you trace the source of an error.
#!/bin/bash
set -x # Enable debugging
echo "This will run"
ls /nonexistentdirectory
# Output:
# + echo 'This will run'
# 'This will run'
# + ls /nonexistentdirectory
# ls: cannot access '/nonexistentdirectory': No such file or directory
In this script, the ‘set -x’ command enables debugging, causing each command to be printed before it’s executed. This can help you see exactly what your script is doing, making it easier to identify where an error occurs.
Writing Robust Bash Scripts
Another concept worth exploring is how to write robust bash scripts. A robust script is one that can handle errors gracefully and continue to function correctly even in unexpected situations. This involves not just error handling, but also good practices such as using clear and meaningful variable names, commenting your code, and organizing your script in a logical and understandable way.
Further Resources for Bash Scripting Mastery
If you’re interested in diving deeper into bash scripting and error handling, here are some resources that you might find helpful:
- GNU Bash Manual: The official manual for bash, providing comprehensive documentation on all its features.
- How to Exit When Errors Occur in Bash: This GeeksforGeeks article provides insights into handling errors in Bash scripts by discussing different techniques.
- Bash Scripting Tutorial: A detailed tutorial covering everything from the basics to more advanced topics in bash scripting.
Remember, mastering bash scripting and error handling is a journey. Take your time, practice regularly, and don’t be afraid to make mistakes. Happy scripting!
Wrapping Up: Mastering Bash Exit on Error
In this comprehensive guide, we’ve journeyed through the world of bash scripting, focusing on how to make bash scripts exit on error. We’ve explored various methods and commands, from the simple ‘set -e’ to more advanced techniques involving ‘trap’, ‘set -o pipefail’, and ‘set -u’.
We began with the basics, learning how to use the ‘set -e’ command to make a bash script exit as soon as any command it executes fails. We then delved into the ‘trap’ command, which provides more control over error handling by allowing us to specify commands to be executed when certain signals are received.
Next, we explored alternative approaches, such as ‘set -o pipefail’, which makes a script exit if any command in a pipeline fails, and ‘set -u’, which causes a script to exit if it tries to use an uninitialized variable. We also discussed common challenges you might encounter when trying to make bash scripts exit on error, and provided solutions to help you overcome these issues.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
set -e | Simple, effective for basic scripts | May cause premature exit for certain commands |
trap | Offers granular control, allows for detailed error messages | More complex, may make scripts harder to read for beginners |
set -o pipefail | Ensures script exits if any command in a pipeline fails | Can make scripts more complex |
set -u | Prevents usage of uninitialized variables | May not be necessary for all scripts |
Whether you’re just starting out with bash scripting or you’re looking to level up your error handling skills, we hope this guide has given you a deeper understanding of how to make bash scripts exit on error.
With these techniques in your toolkit, you’re well-equipped to write robust and reliable bash scripts. Happy scripting!