Singleton Class in Java: What It Is and How to Use It
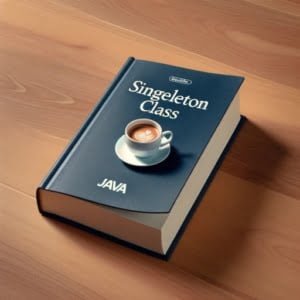
Are you puzzled by the Singleton class in Java? You’re not alone. Many developers find the Singleton class a bit of a mystery, but we’re here to clarify it for you.
Think of the Singleton class in Java as a private club with only one member allowed. It’s a unique concept in Java that allows only one instance of a class to be created, ensuring that a global point of access to it is always provided.
In this guide, we will demystify the Singleton class, its use cases, and how to implement it in Java. We’ll cover everything from the basics of Singleton to more advanced techniques, as well as alternative approaches. We’ll also discuss common issues encountered when implementing Singleton classes and their solutions.
So, let’s dive in and start mastering the Singleton class in Java!
TL;DR: What is a Singleton Class in Java?
A Singleton class in Java is a class that allows only one instance of itself to be created. This is achieved by making the constructor private with the syntax,
private Singleton() {}
and providing a static method to get the instance, such aspublic static Singleton getInstance(){}
.
Here’s a simple example:
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
In this example, we’ve created a Singleton class. The constructor of the class is private, which ensures that no other class can instantiate a new Singleton object. We provide a static method getInstance()
, which returns the single instance of the Singleton class. If the Singleton instance does not exist, it is created inside this method.
This is a basic way to implement a Singleton class in Java, but there’s much more to learn about Singleton classes, including advanced techniques and alternative approaches. Continue reading for a more detailed understanding and practical examples.
Table of Contents
Singleton Design Pattern: Basics and Implementation
The Singleton design pattern is part of the Gang of Four design patterns and falls under the creational design patterns category. The Singleton pattern restricts the instantiation of a class and ensures that only one instance of the class exists in the Java virtual machine.
The Singleton pattern includes a private constructor and a static method that returns the instance of the class. Here is a simple implementation of a Singleton class in Java:
public class Singleton {
// Private static variable of the same class that is the only instance of the class
private static Singleton single_instance = null;
// Private constructor to restrict instantiation of the class from other classes
private Singleton() {}
// Public static method to create instance of Singleton class
public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
In this code, we have a class Singleton
with a private static variable single_instance
of the same class. The variable is private to prevent direct access.
The constructor of the class is private, which ensures that no other class can instantiate a new Singleton object. We provide a static method getInstance()
, which returns the single instance of the Singleton class. If the Singleton instance does not exist, it is created inside this method.
Advantages and Potential Pitfalls of Singleton
The Singleton pattern has several advantages:
- It provides a single point of access to a particular instance, so it’s easy to maintain.
- It saves memory because only one instance of the class is created.
However, there are potential pitfalls:
- Singleton classes are difficult to test because they carry a state around for the lifetime of the application.
- They can be a source of bugs if not handled properly in a multithreaded environment.
Thread Safety in Singleton Classes
In a multithreaded environment, Singleton classes can cause issues if not handled properly. Multiple threads can potentially create multiple instances and violate the Singleton principle. So, how do we ensure thread safety in Singleton classes?
One way is to make the getInstance()
method synchronized. Here’s an example:
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
// Synchronized method to control simultaneous access
synchronized public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
In this example, we’ve made the getInstance()
method synchronized, which means only one thread can access this method at a time, preventing multiple instances.
Evaluating Singleton Implementations
While the synchronized method ensures thread safety, it can impact performance as multiple threads can’t access it simultaneously. There are other ways to implement Singleton with different pros and cons.
- Eager Initialization: This method creates the Singleton instance at the time of class loading. This is the simplest method, but it creates the instance even before it’s accessed, which could be a waste of resources if the instance isn’t used.
Lazy Initialization: This method creates the Singleton instance when it’s needed. This is what we’ve shown in our examples so far. While it saves resources, it can cause issues in a multithreaded environment if not handled properly.
Bill Pugh Singleton (Initialization-on-demand holder idiom): This method introduces a private static inner class that contains the instance of the Singleton class. When the singleton class is loaded, the inner class is not loaded and hence doesn’t create an instance. This is the most widely used approach as it doesn’t use synchronization.
Exploring Alternatives to Singleton Design Pattern
While the Singleton pattern is a useful tool in specific scenarios, it’s not always the best choice. There are other design patterns in Java that can be used as alternatives, such as the Factory and Prototype patterns.
Factory Design Pattern
The Factory pattern is a creational design pattern providing an interface for creating objects in a super class, but allows subclasses to alter the type of objects that will be created.
Here’s a basic example of the Factory pattern:
abstract class Animal {
abstract void makeSound();
}
class Dog extends Animal {
void makeSound() {
System.out.println("Woof");
}
}
class Cat extends Animal {
void makeSound() {
System.out.println("Meow");
}
}
class AnimalFactory {
static Animal create(String type) {
if ("Dog".equals(type)) {
return new Dog();
} else if ("Cat".equals(type)) {
return new Cat();
}
throw new IllegalArgumentException("Invalid type.");
}
}
class Main {
public static void main(String[] args) {
Animal dog = AnimalFactory.create("Dog");
dog.makeSound(); // Outputs: "Woof"
Animal cat = AnimalFactory.create("Cat");
cat.makeSound(); // Outputs: "Meow"
}
}
In this example, we have an Animal
abstract class with a makeSound
method. We have two subclasses, Dog
and Cat
, each implementing the makeSound
method. The AnimalFactory
class has a create
method that creates a new Dog
or Cat
based on the input.
The Factory pattern provides a way to encapsulate a group of individual factories that have a common theme without specifying their concrete classes.
Prototype Design Pattern
The Prototype pattern is also a creational design pattern. It involves creating objects by copying an existing object. This pattern is used when the type of objects to create is determined by a prototypical instance, which is cloned to produce new objects.
Here’s a basic example of the Prototype pattern:
abstract class Shape implements Cloneable {
abstract void draw();
public Object clone() {
Object clone = null;
try {
clone = super.clone();
} catch (CloneNotSupportedException e) {
e.printStackTrace();
}
return clone;
}
}
class Rectangle extends Shape {
void draw() {
System.out.println("Drawing Rectangle");
}
}
class Circle extends Shape {
void draw() {
System.out.println("Drawing Circle");
}
}
class Main {
public static void main(String[] args) {
Shape originalRectangle = new Rectangle();
Shape clonedRectangle = (Shape) originalRectangle.clone();
clonedRectangle.draw(); // Outputs: "Drawing Rectangle"
Shape originalCircle = new Circle();
Shape clonedCircle = (Shape) originalCircle.clone();
clonedCircle.draw(); // Outputs: "Drawing Circle"
}
}
In this example, we have an abstract Shape
class that implements Cloneable
and provides a clone
method. We have two subclasses, Rectangle
and Circle
, each implementing the draw
method. In the main
method, we create a new Rectangle
and Circle
, then clone them.
The Prototype pattern can be beneficial when object creation is time-consuming, and we need to produce a copy of an existing object.
Choosing the Right Design Pattern
Choosing the right design pattern depends on the problem you’re trying to solve. While Singleton is beneficial for controlling access to shared resources, Factory and Prototype can be better choices when you need to create objects dynamically or clone existing objects. Understanding the strengths and weaknesses of each pattern will help you make the right decision.
Troubleshooting Singleton Classes in Java
While implementing Singleton classes in Java, you might encounter some common issues. Let’s discuss these problems, their solutions, and some best practices for optimization.
Issue 1: Singleton Class Not Returning Unique Instance
A typical issue is the Singleton class not returning a unique instance. This usually happens when the Singleton class is not correctly implemented. If the getInstance()
method is not properly synchronized in a multithreaded environment, multiple threads can create multiple instances of the Singleton class.
Here’s an example of this issue:
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
class Main {
public static void main(String[] args) {
Thread thread1 = new Thread(() -> {
Singleton singleton1 = Singleton.getInstance();
System.out.println(singleton1.hashCode());
});
Thread thread2 = new Thread(() -> {
Singleton singleton2 = Singleton.getInstance();
System.out.println(singleton2.hashCode());
});
thread1.start();
thread2.start();
}
}
In this example, we have two threads trying to get an instance of the Singleton class. If the threads run simultaneously, they might end up creating two instances of the Singleton class, violating the Singleton principle.
Solution: Synchronized getInstance() Method
A solution to this issue is to synchronize the getInstance()
method. This ensures that only one thread can access the method at a time.
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
// Synchronized method to control simultaneous access
synchronized public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
In this example, we’ve made the getInstance()
method synchronized, which means only one thread can access this method at a time, preventing multiple instances.
Best Practices and Optimization
While the synchronized method ensures thread safety, it can impact performance as multiple threads can’t access it simultaneously. A better solution is to use the “double-checked locking” principle. With this technique, the synchronized block is used inside the if condition with an additional check to ensure that only one instance of the Singleton class is created.
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
public static Singleton getInstance()
{
if (single_instance == null) {
synchronized (Singleton.class) {
if (single_instance == null)
single_instance = new Singleton();
}
}
return single_instance;
}
}
In this example, the synchronized block is used inside the if condition. This reduces the scope of the synchronized block and improves performance.
Understanding Design Patterns in Java
Design patterns are reusable solutions to common problems in software design. They represent best practices and are templates that can be used in many different situations.
In Java, there are three types of design patterns:
- Creational Patterns: These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Singleton, Factory, and Prototype are examples of creational patterns.
Structural Patterns: These patterns deal with object composition and ensure that different parts of a system work together seamlessly. Adapter, Bridge, and Composite are examples of structural patterns.
Behavioral Patterns: These patterns are specifically concerned with communication between objects. Observer, Strategy, and Template Method are examples of behavioral patterns.
The Role of Singleton in Design Patterns
The Singleton pattern falls under the category of creational patterns. It’s used when we need to ensure that only one object of a particular class is created. All further references to objects of the Singleton class refer to the same underlying instance.
public class Singleton {
private static Singleton single_instance = null;
private Singleton() {}
public static Singleton getInstance()
{
if (single_instance == null)
single_instance = new Singleton();
return single_instance;
}
}
In this code, we have a Singleton class. The constructor of the class is private, which ensures that no other class can instantiate a new Singleton object. We provide a static method getInstance()
, which returns the single instance of the Singleton class. If the Singleton instance does not exist, it is created inside this method.
The Importance of Design Patterns in Software Development
Design patterns are important in software development for several reasons:
- Efficiency: Design patterns provide developers with a way to solve common problems efficiently.
Communication: Design patterns provide a standard terminology and are specific to the scenario, making the code easier to understand by other developers.
Best Practices: Using design patterns promotes reusability and leads to more robust and highly maintainable code.
Understanding and implementing design patterns, such as Singleton, can greatly enhance your skills as a Java developer.
Singleton Classes in Larger Projects
Singleton classes play a crucial role in larger projects, especially when dealing with resources that need to be shared across different parts of the application. For instance, database connections, configurations, and logging services often use Singleton classes to ensure a single point of access and to prevent unnecessary instantiations.
Multithreading and Singleton
Multithreading is a common scenario where Singleton classes are used. In a multithreaded environment, ensuring that only one instance of a class is created can be a challenge. Singleton classes provide a solution by restricting the instantiation of a class to a single object. This is particularly useful in scenarios where simultaneous access to a shared resource can lead to unexpected results.
Object-Oriented Programming and Singleton
In the context of object-oriented programming, the Singleton pattern ensures that a class has only one instance and provides a global point of access to it. This aligns with the principles of data encapsulation and abstraction, as the Singleton class encapsulates its sole instance and provides a way to access it globally.
Further Resources for Mastering Singleton Classes in Java
To delve deeper into the Singleton design pattern and its applications in Java, here are some additional resources:
- Understanding Objects in Java: A Comprehensive Guide – Explore the fundamental concept of objects in Java programming.
Exploring Default Constructor Usage – Learn how default constructors initialize object instances with default values.
Getter and Setter Implementation in Java – The best practices for getter and setter methods in Java classes.
Java Design Patterns explores various design patterns in Java, including Singleton.
Baeldung’s The Singleton Pattern in Java focuses on different ways to implement the Singleton pattern in Java.
GeeksforGeek’s Singleton Class in Java explains the Singleton design pattern in Java.
Wrapping Up: Singleton Class
In this comprehensive guide, we’ve unraveled the Singleton class in Java, a unique design pattern that ensures only one instance of a class exists in the Java virtual machine.
We began with the basics, shedding light on the Singleton class, its definition, and its implementation. We then explored the Singleton design pattern, its advantages, and potential pitfalls. We also provided a simple code example demonstrating how to implement a Singleton class in Java.
We then ventured into more advanced territory, discussing thread safety in Singleton classes and how to ensure it. We also explored different Singleton implementations and their pros and cons. We then shifted our focus to alternative approaches to Singleton, such as the Factory and Prototype patterns, and provided examples, benefits, drawbacks, and decision-making considerations for each.
Finally, we addressed common issues encountered when implementing Singleton classes and provided solutions, code examples, and best practices for optimization. We also underlined the importance of design patterns in software development and where Singleton fits in.
Here’s a quick comparison of the methods we’ve discussed:
Method | Pros | Cons |
---|---|---|
Singleton | Single point of access, Memory efficient | Difficult to test, Can cause bugs in multithreaded environment |
Factory | Flexible, More suited for complex objects | Can become complex |
Prototype | Fast object cloning, More suited when object creation is costly | Requires complex implementation |
Whether you’re just starting out with Singleton classes in Java or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of Singleton classes and their alternatives.
With its unique property of allowing only one instance of a class, Singleton is a powerful tool in your Java arsenal. Now, you’re well equipped to use the Singleton class in your Java projects. Happy coding!