How to Append to a Bash Array | ‘+=’ Operator Guide
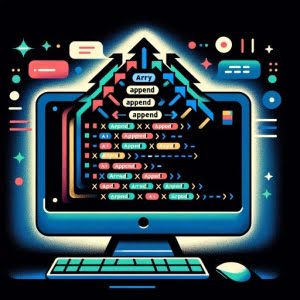
Are you finding it challenging to append elements to an array in Bash? You’re not alone. Many developers find themselves puzzled when it comes to appending to arrays in Bash, but we’re here to help.
Think of an array in Bash as a train, and you’re the conductor adding more carriages. Each carriage represents an element, and the train as a whole represents the array. Appending to an array is like adding more carriages to your train, extending its length and capacity.
In this guide, we’ll walk you through the process of appending to arrays in Bash, from the basics to more advanced techniques. We’ll cover everything from using the ‘+=’ operator to append a single element, appending multiple elements at once, to even appending arrays to arrays.
Let’s get started on this journey to mastering Bash arrays!
TL;DR: How Do I Append to an Array in Bash?
In Bash, you can append to an array using the
'+='
operator, with the syntax,array+=('elementToAdd')
. This operator allows you to add an element to the end of an array.
Here’s a simple example:
arr=('Hello' 'World')
arr+=('!')
echo ${arr[*]}
# Output:
# 'Hello World !'
In this example, we start with an array arr
containing two elements, ‘Hello’ and ‘World’. We then use the ‘+=’ operator to append the exclamation mark ‘!’ to the array. Finally, we print the entire array, which now includes the appended element, resulting in ‘Hello World !’.
This is just a basic way to append to an array in Bash. There’s much more to learn about managing arrays in Bash, including appending multiple elements at once and appending arrays to arrays. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Understanding the Basics: Appending to Bash Arrays
- Append Multiple Elements and Arrays in Bash
- Alternative Methods to Append to Arrays in Bash
- Common Pitfalls and Solutions When Appending to Bash Arrays
- Bash Arrays and the ‘+=’ Operator: A Deep Dive
- Bash Arrays: Exploring Further and Beyond
- Wrapping Up: Mastering Bash Arrays and Appending Operations
Understanding the Basics: Appending to Bash Arrays
Appending to an array in Bash is a fundamental operation that every programmer should understand. The most straightforward way to append to an array in Bash is by using the ‘+=’ operator. This operator allows you to add an element to the end of an array.
Consider the following example:
fruits=('Apple' 'Banana' 'Cherry')
fruits+=('Date')
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Date'
In this example, we start with an array fruits
containing three elements: ‘Apple’, ‘Banana’, and ‘Cherry’. We then use the ‘+=’ operator to append the ‘Date’ to the array. When we print the entire array using echo ${fruits[*]}
, it now includes the appended element, resulting in ‘Apple Banana Cherry Date’.
The ‘+=’ operator in Bash is quite powerful and easy to use. It allows you to append elements to an array quickly, making it an essential tool in your Bash scripting toolkit.
However, it’s important to note that while the ‘+=’ operator is straightforward, it can have some potential pitfalls. For example, if you’re not careful, you might accidentally overwrite an existing array instead of appending to it. Always ensure that you’re using the ‘+=’ operator correctly to avoid such issues.
Append Multiple Elements and Arrays in Bash
As you become more comfortable with Bash arrays, you might find yourself needing to append multiple elements or even entire arrays at once. Fortunately, Bash has you covered.
Appending Multiple Elements
You can append multiple elements to an array in Bash by providing more than one element in the parentheses. Let’s see this in action:
fruits=('Apple' 'Banana' 'Cherry')
fruits+=('Date' 'Elderberry' 'Fig')
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Date Elderberry Fig'
In this example, we’re appending ‘Date’, ‘Elderberry’, and ‘Fig’ to the fruits
array. When we print the array, we can see all six fruits.
Appending Arrays to Arrays
In addition to appending single elements, Bash also allows you to append entire arrays to an existing array. This can be incredibly useful when you need to combine arrays. Here’s how you can do it:
fruits=('Apple' 'Banana' 'Cherry')
moreFruits=('Date' 'Elderberry' 'Fig')
fruits+=(${moreFruits[@]})
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Date Elderberry Fig'
In this example, we have two arrays, fruits
and moreFruits
. We append moreFruits
to fruits
using the ‘+=’ operator and the array expansion syntax ${moreFruits[@]}
. The result is a single fruits
array that contains all six fruit names.
Appending multiple elements or entire arrays at once can save you time and make your scripts more efficient. However, it’s important to understand how these operations work to avoid unexpected results. As always, practice and experimentation are key to mastering these advanced Bash array techniques.
Alternative Methods to Append to Arrays in Bash
While the ‘+=’ operator is the standard way to append to arrays in Bash, there are other methods you can use. One such alternative is array concatenation.
Array Concatenation
Array concatenation is a process where you combine two arrays to form a single array. This can be achieved using the syntax array1=(${array1[@]} ${array2[@]})
. Let’s see this in action:
fruits=('Apple' 'Banana' 'Cherry')
moreFruits=('Date' 'Elderberry' 'Fig')
fruits=(${fruits[@]} ${moreFruits[@]})
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Date Elderberry Fig'
In this example, we’re concatenating moreFruits
to fruits
. The result is a single fruits
array that contains all six fruit names.
The advantage of array concatenation is that it provides an alternative way to append arrays, which can be useful in certain scenarios. However, it can also be more verbose and less intuitive than using the ‘+=’ operator, especially for beginners.
As with any programming technique, the best method to use depends on your specific needs and the context of your script. It’s always a good idea to understand and experiment with different methods so you can choose the most effective approach for your situation.
Common Pitfalls and Solutions When Appending to Bash Arrays
While appending to arrays in Bash is generally straightforward, there are a few common issues that you might encounter. Let’s discuss these potential pitfalls and how to resolve them.
Dealing with Spaces in Array Elements
One common issue arises when you’re dealing with array elements that contain spaces. Let’s look at an example:
fruits=('Apple' 'Banana' 'Cherry Pie')
fruits+=('Date' 'Elderberry')
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Pie Date Elderberry'
In this example, we have an array element ‘Cherry Pie’ that contains a space. When we print the array, it treats ‘Cherry Pie’ as a single element, which is what we want.
However, if we don’t use quotes around ‘Cherry Pie’, Bash will treat ‘Cherry’ and ‘Pie’ as separate elements. This is because Bash uses spaces to separate elements in an array. Here’s what happens when we don’t use quotes:
fruits=(Apple Banana Cherry Pie)
fruits+=(Date Elderberry)
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Pie Date Elderberry'
In this case, ‘Cherry’ and ‘Pie’ are treated as separate elements, which is not what we intended.
The solution is simple: always use quotes around array elements that contain spaces. This ensures that Bash treats them as a single element.
Avoid Overwriting Existing Arrays
Another common pitfall is accidentally overwriting an existing array when you intend to append to it. This usually happens when you use the ‘=’ operator instead of the ‘+=’ operator.
fruits=('Apple' 'Banana' 'Cherry')
fruits=('Date')
echo ${fruits[*]}
# Output:
# 'Date'
In this example, we intended to append ‘Date’ to the fruits
array. However, we used the ‘=’ operator, which replaced the entire fruits
array with a new array containing only ‘Date’.
To avoid this issue, always use the ‘+=’ operator when you want to append to an array. This ensures that you’re adding to the existing array instead of replacing it.
Understanding these common issues and how to resolve them can help you avoid potential headaches when working with arrays in Bash. Remember to always test your scripts to ensure they’re working as expected.
Bash Arrays and the ‘+=’ Operator: A Deep Dive
Before we can fully understand how to append to arrays in Bash, we need to understand what Bash arrays are and how they work. Additionally, we’ll explore the ‘+=’ operator and its role in array manipulation.
Bash Arrays: A Brief Overview
In Bash, an array is a variable that can hold multiple values. Each value (also called an element) in an array is assigned a unique index, starting from zero. You can access each element in the array using its index.
Here’s an example of a Bash array:
fruits=('Apple' 'Banana' 'Cherry')
echo ${fruits[1]}
# Output:
# 'Banana'
In this example, we have an array fruits
with three elements. We access the second element (index 1) using echo ${fruits[1]}
, which prints ‘Banana’.
The ‘+=’ Operator in Bash
The ‘+=’ operator in Bash is used to append to variables, including arrays. When used with an array, it adds a new element to the end of the array.
Let’s see this in action:
fruits=('Apple' 'Banana' 'Cherry')
fruits+=('Date')
echo ${fruits[*]}
# Output:
# 'Apple Banana Cherry Date'
In this example, we use the ‘+=’ operator to append ‘Date’ to the fruits
array. When we print the array, we see that ‘Date’ has been added to the end.
The ‘+=’ operator is a powerful tool in Bash. Understanding how it works with arrays is key to mastering array manipulation in Bash.
Bash Arrays: Exploring Further and Beyond
Appending to arrays in Bash is more than just a basic operation – it’s a skill that can greatly enhance your shell scripting abilities. It allows you to create dynamic scripts that can handle varying amounts of data, making your scripts more flexible and powerful.
Array Slicing in Bash
One related concept you might want to explore is array slicing. This is a technique where you extract a subset of an array. Here’s an example:
fruits=('Apple' 'Banana' 'Cherry' 'Date' 'Elderberry' 'Fig')
slicedFruits=(${fruits[@]:1:3})
echo ${slicedFruits[*]}
# Output:
# 'Banana Cherry Date'
In this example, we’re slicing the fruits
array to get a new array slicedFruits
that contains only ‘Banana’, ‘Cherry’, and ‘Date’.
Iterating Over Arrays in Bash
Another important concept is iterating over arrays. This is where you go through each element in an array and perform some operation. Here’s an example:
fruits=('Apple' 'Banana' 'Cherry')
for fruit in ${fruits[@]}; do
echo $fruit
# Output:
# 'Apple'
# 'Banana'
# 'Cherry'
In this example, we’re using a for
loop to iterate over the fruits
array and print each fruit.
Further Resources for Mastering Bash Arrays
For those who wish to delve deeper into Bash arrays, here are some valuable resources:
- Bash Guide for Beginners: This guide covers the basics of Bash, including arrays, and is a great starting point for beginners.
Advanced Bash-Scripting Guide: This guide goes beyond the basics and covers more advanced topics, including array operations.
Bash Array Tutorial: This tutorial focuses specifically on Bash arrays and provides a variety of examples and use cases.
Remember, mastering Bash arrays requires practice and experimentation. Don’t be afraid to try out different techniques and push your boundaries. Happy scripting!
Wrapping Up: Mastering Bash Arrays and Appending Operations
In this comprehensive guide, we’ve delved deep into the world of Bash arrays, specifically focusing on how to append to arrays in Bash. We’ve explored the basic ‘+=’ operator, highlighted its usage, and discussed common issues and their solutions.
We began with the basics, learning how to use the ‘+=’ operator to append single and multiple elements to a Bash array. We then ventured into more advanced territory, exploring how to append entire arrays to an existing array. Along the way, we tackled common challenges you might face, such as dealing with spaces in array elements and avoiding overwriting existing arrays, providing you with solutions for each issue.
We also looked at alternative approaches to appending to arrays in Bash, such as array concatenation. We compared these methods and discussed their advantages and disadvantages, giving you a sense of the broader landscape of array manipulation techniques in Bash.
Method | Pros | Cons |
---|---|---|
‘+=’ Operator | Simple, intuitive, and versatile | Can be misused to overwrite arrays |
Array Concatenation | Useful for combining arrays | More verbose and less intuitive |
Whether you’re just starting out with Bash arrays or you’re looking to level up your shell scripting skills, we hope this guide has given you a deeper understanding of how to append to arrays in Bash.
The ability to manipulate arrays is a powerful tool in Bash scripting. With the knowledge you’ve gained from this guide, you’re now well equipped to handle arrays in Bash confidently. Happy scripting!