Bash ‘case’ Statement | Scripting Control Flow Guide
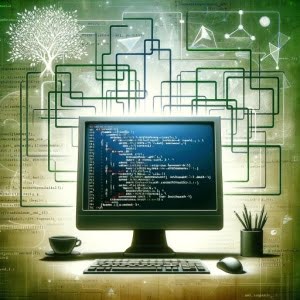
Are you struggling to make your bash scripts more efficient and readable? You’re not alone. Many developers find it challenging to control the flow of their scripts effectively.
Think of the bash case statement as a traffic cop at a busy intersection. It directs the flow of your script based on the value of a variable or expression, making your scripts more efficient and readable.
This guide will walk you through the basics to advanced usage of the bash case statement. We’ll cover everything from simple use cases to more complex scenarios, and even discuss alternative approaches and common issues.
So, let’s dive in and start mastering the bash case statement!
TL;DR: How Do I Use a Case Statement in Bash?
The bash
case
statement allows you to perform different actions based on the value of a variable or expression. To use it requires you to use thecase $[variable] in [action1];; [action2];; etc...
syntax. It’s a powerful tool that can make your scripts more efficient and easier to read.
Here’s a simple example:
variable="pattern1"
case $variable in
pattern1) echo "Matched pattern1" ;;
pattern2) echo "Matched pattern2" ;;
esac
# Output:
# 'Matched pattern1'
In this example, we have a variable named ‘variable’ with the value ‘pattern1’. The bash case statement checks the value of this variable against the patterns specified. Since the value matches ‘pattern1’, the command ‘echo “Matched pattern1″‘ is executed, and ‘Matched pattern1’ is printed to the console.
This is a basic way to use the bash case statement, but there’s much more to learn about it. Continue reading for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Bash Case Statement: The Basics
- Advanced Uses of the Bash Case Statement
- Exploring Alternative Approaches
- Troubleshooting Common Issues with Bash Case Statements
- Understanding Bash Control Flow Statements
- The Relevance of Bash Case Statement in Larger Scripts
- Wrapping Up: Mastering the Bash Case Statement
Bash Case Statement: The Basics
The bash case statement is an essential tool in shell scripting, especially when you need to make decisions based on the value of a variable or expression.
Here’s a simple example of how the bash case statement works:
animal="Dog"
case $animal in
Cat) echo "Furry and adorable" ;;
Dog) echo "Playful and loyal" ;;
Fish) echo "Silent and calming" ;;
*) echo "Unknown animal" ;;
esac
# Output:
# 'Playful and loyal'
In this code block, we have a variable named ‘animal’ with the value ‘Dog’. The bash case statement checks the value of this variable against the patterns specified (in this case, ‘Cat’, ‘Dog’, and ‘Fish’). Since the value matches ‘Dog’, the command ‘echo “Playful and loyal”‘ is executed, and ‘Playful and loyal’ is printed to the console.
The last pattern ‘*)’ is a catch-all pattern. If the value of the variable does not match any of the specified patterns, the command associated with this pattern will be executed.
The bash case statement is a powerful tool that can simplify your scripts and make them more readable. However, it’s important to use it correctly to avoid potential pitfalls, such as forgetting to include the ‘;;’ at the end of each pattern-action pair or not including a catch-all pattern when necessary.
Advanced Uses of the Bash Case Statement
As you gain more experience with bash scripting, you’ll find that the bash case statement is more versatile than it initially appears. One of its advanced uses is handling wildcards and combining it with other control flow statements.
Wildcards in Bash Case Statement
Wildcards, denoted by ‘*’, can be used in the bash case statement to match multiple patterns. Here’s an example:
filename="document.txt"
case $filename in
*.txt) echo "This is a text file." ;;
*.doc) echo "This is a Word document." ;;
*.pdf) echo "This is a PDF document." ;;
*) echo "Unknown file type." ;;
esac
# Output:
# 'This is a text file.'
In this code block, the variable ‘filename’ has the value ‘document.txt’. The bash case statement checks this value against the patterns specified, which include wildcards. Since the value ends with ‘.txt’, the command ‘echo “This is a text file.”‘ is executed, and ‘This is a text file.’ is printed to the console.
Combining Bash Case with Other Control Flow Statements
The bash case statement can also be combined with other control flow statements, like if-else or for loop, to create more complex scripts. Here’s an example:
for animal in Dog Cat Fish
do
case $animal in
Dog) echo "Dog: Man's best friend" ;;
Cat) echo "Cat: Independent and curious" ;;
Fish) echo "Fish: Silent swimmer" ;;
esac
done
# Output:
# 'Dog: Man's best friend'
# 'Cat: Independent and curious'
# 'Fish: Silent swimmer'
In this code block, we have a for loop that iterates over a list of animals. For each animal, the bash case statement checks the value against the specified patterns and executes the corresponding command. This combination of the bash case statement with a for loop allows us to handle multiple values efficiently.
Exploring Alternative Approaches
While the bash case statement is a powerful tool for conditional execution, it’s not the only option available in bash scripting. Other methods, such as if-else statements and the select statement, can also be used depending on your needs.
Using If-Else Statements
If-else statements are another common method for conditional execution in bash. They can be used in situations where you need to perform different actions based on whether a certain condition is true or false.
Here’s an example of how you can use an if-else statement to achieve a similar result as a bash case statement:
animal="Dog"
if [[ $animal == "Dog" ]]; then
echo "Playful and loyal"
elif [[ $animal == "Cat" ]]; then
echo "Furry and adorable"
else
echo "Unknown animal"
fi
# Output:
# 'Playful and loyal'
In this code block, we’re checking if the value of the variable ‘animal’ is ‘Dog’ or ‘Cat’. If it’s ‘Dog’, we print ‘Playful and loyal’. If it’s ‘Cat’, we print ‘Furry and adorable’. If it’s neither, we print ‘Unknown animal’.
Using the Select Statement
The select statement is a less common but still useful method for conditional execution in bash. It’s particularly useful when you need to create a simple menu system.
Here’s an example:
select animal in Dog Cat Fish
do
case $animal in
Dog) echo "Playful and loyal" ;;
Cat) echo "Furry and adorable" ;;
Fish) echo "Silent and calming" ;;
esac
done
# Output (after selecting '1'):
# 'Playful and loyal'
In this code block, we’re creating a menu with the select statement that allows the user to choose an animal. The bash case statement then checks the selected animal and prints a corresponding message.
Each of these methods has its advantages and disadvantages. The bash case statement is versatile and easy to read, but it can be more complex to write than an if-else statement. The if-else statement is simple and straightforward, but it can become unwieldy if you have many conditions to check. The select statement is useful for creating menus, but it’s not as versatile as the other two methods.
Troubleshooting Common Issues with Bash Case Statements
Even with the best planning, you may encounter issues when using the bash case statement. Let’s discuss some common problems and how to solve them.
Issue 1: Forgetting the Double Semicolon
One common issue is forgetting to end each pattern-action pair with a double semicolon (;;). This will result in a syntax error. Here’s an example:
animal="Dog"
case $animal in
Dog) echo "Playful and loyal"
Cat) echo "Furry and adorable"
esac
# Output:
# Syntax error near unexpected token `Cat'
To fix this issue, simply add a double semicolon at the end of each pattern-action pair:
animal="Dog"
case $animal in
Dog) echo "Playful and loyal" ;;
Cat) echo "Furry and adorable" ;;
esac
# Output:
# 'Playful and loyal'
Issue 2: No Catch-All Pattern
Another common issue is not including a catch-all pattern. If the value of the variable doesn’t match any of the specified patterns, and there’s no catch-all pattern, the bash case statement will do nothing. Here’s an example:
animal="Bird"
case $animal in
Dog) echo "Playful and loyal" ;;
Cat) echo "Furry and adorable" ;;
esac
# Output:
# No output
To fix this issue, add a catch-all pattern that will catch any value not matched by the other patterns:
animal="Bird"
case $animal in
Dog) echo "Playful and loyal" ;;
Cat) echo "Furry and adorable" ;;
*) echo "Unknown animal" ;;
esac
# Output:
# 'Unknown animal'
By being aware of these common issues and knowing how to solve them, you can avoid many pitfalls when using the bash case statement.
Understanding Bash Control Flow Statements
Before we delve deeper into the bash case statement, let’s take a moment to understand control flow statements in bash scripting.
Control flow statements are the building blocks of bash scripts. They allow you to control the flow of execution of your script based on conditions, loops, and patterns. The most common control flow statements are if-else, for loop, while loop, and of course, the case statement.
How Bash Interprets Patterns in a Case Statement
One of the key features of the bash case statement is its ability to interpret patterns. This is what allows it to execute different actions based on the value of a variable or expression.
Patterns in a bash case statement are not regular expressions. Instead, they are more similar to glob patterns used for filename expansion. Here’s a basic example:
number=5
case $number in
[1-3]) echo "Low" ;;
[4-6]) echo "Medium" ;;
[7-9]) echo "High" ;;
esac
# Output:
# 'Medium'
In this code block, the variable ‘number’ holds the value 5. The bash case statement checks this value against the patterns specified. The patterns here are ranges of numbers enclosed in square brackets. Since the value 5 falls within the range [4-6], the command ‘echo “Medium”‘ is executed, and ‘Medium’ is printed to the console.
Understanding how bash interprets patterns in a case statement is crucial for using it effectively. It allows you to create more complex and versatile scripts.
The Relevance of Bash Case Statement in Larger Scripts
The bash case statement is not just for simple scripts. Its power and versatility make it an essential tool in larger scripts and projects as well. By effectively directing the flow of your script based on the value of a variable or expression, the bash case statement can significantly improve the efficiency and readability of your code.
Exploring Related Concepts
To become even more proficient in bash scripting, consider exploring related concepts like bash loops and functions. Bash loops, for example, allow you to repeat certain actions a specific number of times or until a condition is met. Functions, on the other hand, allow you to group commands together and call them as a single command, making your scripts more modular and easier to maintain.
Here’s an example of how you can use a bash case statement in a function:
function animal_description() {
case $1 in
Dog) echo "Playful and loyal" ;;
Cat) echo "Furry and adorable" ;;
Fish) echo "Silent and calming" ;;
esac
}
animal_description Dog
animal_description Cat
animal_description Fish
# Output:
# 'Playful and loyal'
# 'Furry and adorable'
# 'Silent and calming'
In this code block, we’ve defined a function named ‘animal_description’ that takes one argument. Inside the function, we use a bash case statement to print a different description based on the argument passed to the function. When we call the function with ‘Dog’, ‘Cat’, and ‘Fish’ as arguments, we get the corresponding descriptions printed to the console.
Further Resources for Bash Scripting Mastery
To further deepen your understanding of bash scripting and the bash case statement, here are some resources that you might find useful:
- Advanced Bash-Scripting Guide: This is a comprehensive guide to bash scripting that covers everything from the basics to advanced topics.
Bash Guide for Beginners: This guide is perfect for beginners and covers all the basics of bash scripting.
Linuxize – Bash Case Statement: This article explains the syntax, usage, and provides examples to the Bash case statement.
Wrapping Up: Mastering the Bash Case Statement
In this comprehensive guide, we’ve journeyed through the world of bash scripting, focusing on the bash case statement. We’ve explored its usage, from basic scenarios to more complex ones, and provided solutions to common issues that might arise.
We began with the basics, learning how to use the bash case statement to make our scripts more efficient and readable. We then ventured into more advanced territory, exploring the use of wildcards and the combination of the bash case statement with other control flow statements.
Along the way, we tackled common challenges you might face when using the bash case statement, such as forgetting the double semicolon or not including a catch-all pattern, providing you with solutions for each issue.
We also looked at alternative approaches to conditional execution in bash, comparing the bash case statement with if-else statements and the select statement. Here’s a quick comparison of these methods:
Method | Versatility | Complexity | Ease of Use |
---|---|---|---|
Bash Case Statement | High | Moderate | High |
If-Else Statement | Moderate | Low | High |
Select Statement | Low | High | Moderate |
Whether you’re just starting out with bash scripting or you’re looking to level up your skills, we hope this guide has given you a deeper understanding of the bash case statement and its capabilities.
With its balance of versatility, complexity, and ease of use, the bash case statement is a powerful tool for conditional execution in bash. Happy scripting!