Using Train Test Split in Sklearn: A Complete Tutorial
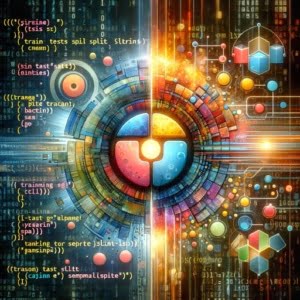
Are you looking to split your dataset for machine learning? Just like a skilled chef preparing ingredients for a gourmet meal, Scikit-learn can help you efficiently divide your data into training and testing sets.
This comprehensive guide will walk you through the process, from the basics to more advanced techniques. Whether you’re a beginner just starting out or an experienced data scientist looking to refine your skills, this tutorial will provide you with the knowledge you need to master the train_test_split
function in Scikit-learn.
So, let’s dive in and start slicing our data!
TL;DR: How Do I Split My Dataset Using Scikit-Learn?
You can use the
train_test_split
function from thesklearn.model_selection
module. Here’s a simple example:
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2)
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
This code splits your dataset (X, y) into a training set (80%) and a test set (20%). The train_test_split
function is a quick and efficient way to prepare your data for machine learning models.
But there’s more to it than just this basic usage. Read on for a more detailed explanation and advanced usage scenarios.
Table of Contents
- Getting Started with train_test_split in Scikit-Learn
- Advanced Techniques with train_test_split
- Exploring Alternative Methods for Data Splitting
- Troubleshooting train_test_split
- Understanding the Need for Data Splitting
- Using train_test_split in Larger Projects
- Wrapping Up: Mastering train_test_split in Scikit-Learn
Getting Started with train_test_split
in Scikit-Learn
The train_test_split
function is a powerful tool in Scikit-learn’s arsenal, primarily used to divide datasets into training and testing subsets. This function is part of the sklearn.model_selection
module, which contains utilities for splitting data. But how does it work? Let’s dive in.
from sklearn.model_selection import train_test_split
# Assume X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
In the above code, X
and y
are your features and labels, respectively. The train_test_split
function shuffles the dataset and then splits it. The test_size
parameter determines the proportion of the original dataset to include in the test split. In this case, we’ve set it to 0.2, meaning 20% of the data will be used for the test set, and the remaining 80% for the training set.
The random_state
parameter is used for initializing the internal random number generator, which will decide the splitting of data into train and test indices. This is to ensure reproducibility. If you don’t specify or pass an integer to the random_state
parameter, you might get a different output each time you split the data because of the shuffling.
While train_test_split
is a handy function, it’s important to be aware of potential pitfalls. For instance, if your dataset is imbalanced (i.e., one class has significantly more samples than another), the function could create a training set that doesn’t accurately represent the overall distribution of classes. But don’t worry, we’ll cover how to handle such issues in the ‘Advanced Use’ section.
Advanced Techniques with train_test_split
Once you’ve mastered the basics of train_test_split
, it’s time to explore some of its more complex uses. Two such techniques are stratified sampling and setting a random seed.
Stratified Sampling with train_test_split
Stratified sampling is a method of sampling that involves dividing a population into homogeneous subgroups known as strata, and then sampling from each stratum. In the context of train_test_split
, stratified sampling can be useful when dealing with imbalanced datasets to ensure that the training and test datasets have the same proportion of class labels as the input dataset.
Here’s how you can use stratified sampling with train_test_split
:
from sklearn.model_selection import train_test_split
# Assume X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42, stratify=y)
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
In the above code, we’ve added the stratify
parameter and set it to y
, which is our label or target variable. This ensures that the distribution of labels will be the same in the training and test sets as they are in the original dataset.
Setting a Random Seed with train_test_split
As you may have noticed, we’ve been setting the random_state
parameter in our examples. This parameter is the seed used by the random number generator. Setting a seed ensures that the splits you generate are reproducible. If you don’t set a seed, you might get different splits every time you run the code, which can make your results hard to replicate.
from sklearn.model_selection import train_test_split
# Assume X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
In the above code, we’ve set random_state
to 42. This means that every time we run this code, we’ll get the same split, which is important for reproducibility in machine learning experiments.
Exploring Alternative Methods for Data Splitting
While train_test_split
from Scikit-Learn is a popular choice for dividing datasets, there are other libraries like Pandas and NumPy that offer alternative methods. Let’s explore these options.
Splitting Data with Pandas
Pandas is a powerful data manipulation library in Python. It has a function called sample
which can be used to randomly sample rows from a DataFrame. Here’s an example:
import pandas as pd
data = pd.DataFrame(X, columns=['Features'])
data['Target'] = y
# Randomly sample 80% of your dataframe
train = data.sample(frac=0.8, random_state=42)
# Drop the training data to create a test set
test = data.drop(train.index)
# Output:
# 'train' and 'test' are now your split datasets
In this example, we first convert our data into a Pandas DataFrame and then use the sample
function to create a training set. The frac
parameter is used to specify the fraction of rows to return. We then use the drop
function to create a test set by removing the training data from the original DataFrame.
Using NumPy for Data Splitting
NumPy is a library for the Python programming language, adding support for large, multi-dimensional arrays and matrices, along with a large collection of high-level mathematical functions to operate on these arrays.
import numpy as np
# Assume indices is an array of indices
indices = np.arange(X.shape[0])
np.random.shuffle(indices)
train_idx, test_idx = indices[:int(0.8*X.shape[0])], indices[int(0.8*X.shape[0]):]
X_train, X_test = X[train_idx], X[test_idx]
y_train, y_test = y[train_idx], y[test_idx]
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
In the above code, we first create an array of indices and then shuffle it. We then split this array into training and test indices, and use these to create our training and test sets.
While these alternative methods can be useful, they also have their drawbacks. The Pandas method, for example, requires your data to be in a DataFrame, which might not always be the case. The NumPy method, on the other hand, requires manual shuffling and splitting, which can be prone to errors. In contrast, train_test_split
from Scikit-Learn is specifically designed for splitting datasets and provides additional features like stratified sampling.
Troubleshooting train_test_split
While train_test_split
is a powerful tool, it’s not without its challenges. Let’s discuss some common issues you may encounter when using this function and how to troubleshoot them.
Dealing with Imbalanced Data
One common issue is dealing with imbalanced data. If one class in your dataset has significantly more samples than another, train_test_split
might create a training set that doesn’t accurately represent the overall distribution of classes. Here’s how you can handle this issue:
from sklearn.model_selection import train_test_split
# Assume X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42, stratify=y)
# Output:
# X_train, X_test, y_train, y_test are now your split datasets
In the above code, we’ve added the stratify
parameter and set it to y
, which is our label or target variable. This ensures that the distribution of labels will be the same in the training and test sets as they are in the original dataset.
Handling Small Datasets
Another issue is handling small datasets. If your dataset is too small, the test set might end up being too small to be representative of the data. In this case, you might want to consider using cross-validation instead of a simple train/test split.
from sklearn.model_selection import cross_val_score
from sklearn.linear_model import LogisticRegression
# Assume X and y are your features and labels
clf = LogisticRegression(random_state=42)
scores = cross_val_score(clf, X, y, cv=5)
# Output:
# 'scores' contains the cross-validation scores
In the above code, we’ve used the cross_val_score
function to perform 5-fold cross-validation. This function splits the data into 5 subsets and then trains and evaluates the model 5 times, each time with a different subset as the test set.
Remember, train_test_split
is a versatile function, but it’s not a one-size-fits-all solution. Depending on your data and the problem you’re trying to solve, you might need to consider alternative approaches or additional preprocessing steps.
Understanding the Need for Data Splitting
Before we delve deeper into the technical aspects of train_test_split
, it’s crucial to understand why we split our dataset into training and testing sets in machine learning and what these concepts of overfitting and underfitting are.
The Rationale Behind Data Splitting
In machine learning, our goal is to build models that generalize well to new, unseen data. To achieve this, we need a way to measure how well our model is likely to perform on such data. That’s where the concept of splitting our data into a training set and a test set comes in.
The training set is used to train our model, while the test set is used to evaluate its performance on unseen data. This setup helps us estimate how well the model has learned the underlying patterns in the data and how it will likely perform in the real world.
Overfitting and Underfitting
When training a machine learning model, we strive to find a balance between learning the data too well and not learning it well enough. These two extremes are known as overfitting and underfitting.
Overfitting occurs when a model learns the training data too well. It captures not only the underlying patterns but also the noise and outliers in the data. As a result, it performs well on the training data but poorly on new, unseen data.
# Example of a model that may be overfitting
from sklearn.tree import DecisionTreeClassifier
# Assume X_train and y_train are your training features and labels
clf = DecisionTreeClassifier(max_depth=None)
clf.fit(X_train, y_train)
# Output:
# 'clf' is a decision tree classifier that may be overfitting
In the above code, we’ve trained a decision tree classifier with no maximum depth, which means it can grow deep enough to perfectly classify every sample in the training set, potentially capturing noise and outliers.
Underfitting, on the other hand, occurs when a model fails to capture the underlying patterns in the data. It performs poorly on both the training data and new, unseen data.
# Example of a model that may be underfitting
from sklearn.tree import DecisionTreeClassifier
# Assume X_train and y_train are your training features and labels
clf = DecisionTreeClassifier(max_depth=1)
clf.fit(X_train, y_train)
# Output:
# 'clf' is a decision tree classifier that may be underfitting
In the above code, we’ve trained a decision tree classifier with a maximum depth of 1, which means it can only make one decision, potentially failing to capture more complex patterns in the data.
By splitting our data into a training set and a test set and evaluating our model’s performance on the test set, we can get an estimate of how well our model is doing in terms of balancing between overfitting and underfitting.
Using train_test_split
in Larger Projects
train_test_split
is not just for basic data splitting. It’s a versatile function that can be used in larger machine learning projects, such as building a machine learning pipeline. In a pipeline, data preprocessing, model training, and model evaluation steps are combined into a single scikit-learn estimator.
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from sklearn.pipeline import make_pipeline
# Assume X and y are your features and labels
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Create a pipeline
pipeline = make_pipeline(
StandardScaler(),
LogisticRegression(random_state=42)
)
# Fit the pipeline on the training data
pipeline.fit(X_train, y_train)
# Output:
# 'pipeline' is a machine learning pipeline fitted on the training data
In the above code, we first split the data using train_test_split
. We then create a pipeline that first standardizes the data using StandardScaler
and then fits a LogisticRegression
model. The pipeline is finally fitted on the training data.
Exploring Related Concepts: Cross-Validation
While train_test_split
is a great tool for creating a simple train/test split, it’s worth exploring related concepts like cross-validation. Cross-validation is a more robust method of evaluating model performance, where the dataset is split into ‘k’ folds and the model is trained and evaluated ‘k’ times, each time with a different fold as the test set.
Scikit-learn provides several functions for performing cross-validation, such as cross_val_score
and cross_validate
. These functions are worth exploring if you want to get a more accurate estimate of your model’s performance.
Further Resources for Mastering train_test_split
To deepen your understanding of train_test_split
and related concepts, here are some resources you might find useful:
- Simplifying Python Library Selection – Learn how Python libraries simplify complex tasks and save development time.
Getting Started with Pygame: Creating Games in Python – Learn how to use Pygame and make games and simulation.
Python Data Analysis with the Polars Library – Learn how to work with large datasets effortlessly using Polars.
Scikit-Learn Documentation – The official documentation explains
train_test_split
and its parameters.Machine Learning Mastery – This tutorial provides info on using
train_test_split
for machine learning algorithms.DataCamp – This tutorial covers machine learning workflows in Python, including data splitting using
train_test_split
.
Wrapping Up: Mastering train_test_split
in Scikit-Learn
Throughout this guide, we’ve explored the ins and outs of train_test_split
in Scikit-Learn, a crucial function for splitting datasets in machine learning.
We learned how to use it at a basic level and then dove into more advanced techniques, including stratified sampling and setting a random seed. We also discussed common issues, such as dealing with imbalanced data and handling small datasets, and how to troubleshoot them.
In addition to train_test_split
, we explored alternative methods for splitting data using other libraries like Pandas and NumPy. Each method has its own advantages and drawbacks, and the best one to use depends on your specific needs and the nature of your data.
To summarize, here’s a comparison of the different methods we discussed:
Method | Advantages | Disadvantages |
---|---|---|
train_test_split | Easy to use, Supports stratified sampling | May not be suitable for small or imbalanced datasets |
Pandas sample | Works well with DataFrames | Requires data to be in a DataFrame |
NumPy splitting | Gives full control over the splitting process | Requires manual shuffling and splitting |
Remember, the key to effective machine learning is understanding your tools and knowing how to use them to suit your needs. Whether you’re just starting out in data science or looking to refine your skills, mastering data splitting techniques like train_test_split
is a crucial step in your journey. Keep practicing and exploring, and you’ll be a data splitting pro in no time!