Understanding =+ (or -) Symbols in Python
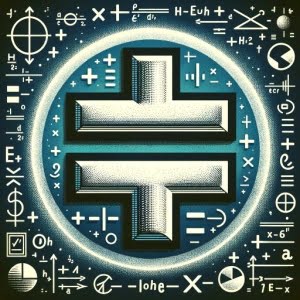
Are you finding it challenging to understand the += and -= operators in Python? You’re not alone. Many developers find these shorthand operators a bit puzzling, but they’re a powerful tool in Python’s arsenal.
Whether you’re incrementing a counter in a loop or adjusting values in data manipulation, understanding how to use the += and -= operators in Python can significantly streamline your coding process.
In this guide, we’ll walk you through the process of understanding and effectively using the += and -= operators in Python, from the basics to more advanced techniques. We’ll cover everything from their basic use, advanced use with different data types, alternative approaches, as well as common issues and their solutions.
Let’s get started!
TL;DR: What are the += and -= Operators in Python?
The += and -= operators in Python are shorthand operators that allow you to increment and decrement the value of a variable, respectively. They are efficient and can make your code cleaner and easier to read.
Here’s a simple example:
x = 5
x += 1
print(x) # Output: 6
y = 10
y -= 2
print(y) # Output: 8
In this example, we have two variables, x
and y
. We increment x
by 1 using the +=
operator, and decrement y
by 2 using the -=
operator. The output shows the new values of x
and y
after the operations.
These operators are just the tip of the iceberg when it comes to Python’s shorthand operators. Continue reading for a more in-depth look at these operators, their usage, and how they can streamline your Python programming.
Table of Contents
- Basic Use of += and -= Operators in Python
- += and -= Operators with Different Data Types
- Alternative Ways to Increment and Decrement Variables
- Troubleshooting Common Issues with += and -= Operators
- Python Operators: A Closer Look
- The Power of += and -= Operators in Python Programming
- Wrapping Up: Mastering += and -= Operators in Python
Basic Use of += and -= Operators in Python
The += and -= operators in Python are shorthand operators that offer a quick and efficient way to increment or decrement the value of a variable.
Let’s start with the +=
operator.
x = 10
x += 2
print(x) # Output: 12
In this example, we have a variable x
with an initial value of 10. The x += 2
operation increments the value of x
by 2. Therefore, the new value of x
is 12.
The +=
operator is a shorthand for x = x + 2
. It’s a more efficient way to write the same operation, and it makes your code cleaner and easier to read.
Now, let’s look at the -=
operator.
y = 10
y -= 2
print(y) # Output: 8
In this example, the y -= 2
operation decrements the value of y
by 2. Therefore, the new value of y
is 8.
The -=
operator is a shorthand for y = y - 2
. Just like the +=
operator, it provides a more efficient and cleaner way to write the decrement operation.
One thing to remember when using these shorthand operators is that the variable must be defined before you can use it with the +=
or -=
operator. If you try to use an undefined variable with these operators, you will get a NameError
.
z += 1 # Raises a NameError
This code will raise a NameError
because the variable z
has not been defined before we try to increment it with the +=
operator.
+= and -= Operators with Different Data Types
Python’s += and -= operators are not limited to numeric data types. They can be used with other data types like lists and strings, which can be incredibly useful in many scenarios.
Using += and -= Operators with Lists
Let’s take a look at how you can use the += operator with lists.
list1 = [1, 2, 3]
list1 += [4, 5]
print(list1) # Output: [1, 2, 3, 4, 5]
Here, we have a list list1
with initial elements 1, 2, and 3. The operation list1 += [4, 5]
appends the elements 4 and 5 to list1
. Therefore, the new value of list1
is [1, 2, 3, 4, 5].
However, the -= operator does not work with lists. If you try to use it, you will get a TypeError
.
list1 -= [4, 5] # Raises a TypeError
Using += Operator with Strings
The += operator can also be used with strings to concatenate them.
str1 = 'Hello'
str1 += ' World!'
print(str1) # Output: 'Hello World!'
In this example, the str1 += ' World!'
operation appends the string ‘ World!’ to str1
. Therefore, the new value of str1
is ‘Hello World!’.
Just like with lists, the -= operator does not work with strings. If you try to use it, you will get a TypeError
.
str1 -= ' World!' # Raises a TypeError
As you can see, the += and -= operators can be used with different data types in Python, but their behavior varies depending on the data type. Understanding how these operators work with different data types can help you write more efficient and cleaner code.
Alternative Ways to Increment and Decrement Variables
While the += and -= operators are commonly used in Python for incrementing and decrementing variables, there are other approaches you can use. However, it’s important to note that Python does not support the ++
and --
operators found in languages like C++ or Java. Trying to use these operators in Python will not result in a syntax error, but they won’t behave as you might expect.
The ++ and — Operators in Python
In Python, ++
and --
are not really operators. They are syntax from C-style languages and have different meanings in Python.
x = 5
x++ # Raises a SyntaxError
If you try to increment a variable using ++
in Python, you will get a SyntaxError
. The same applies to the --
operator.
y = 10
y-- # Raises a SyntaxError
Incrementing and Decrementing Without Shorthand Operators
You can increment or decrement variables in Python without using the += or -= operators. Here’s how you can do it:
x = 5
x = x + 1
print(x) # Output: 6
y = 10
y = y - 1
print(y) # Output: 9
In these examples, we increment x
by 1 and decrement y
by 1 by explicitly writing out the operations. While this method works, it’s more verbose and less efficient than using the += and -= operators.
Understanding these alternative approaches can give you a deeper understanding of how Python handles incrementing and decrementing operations. However, in practice, the += and -= operators are generally the preferred method due to their efficiency and readability.
Troubleshooting Common Issues with += and -= Operators
While the += and -= operators in Python are generally straightforward to use, there are a few common issues that you might encounter. Let’s walk through some of these potential pitfalls and how to handle them.
Undefined Variables
One common issue occurs when you try to use the += or -= operator on a variable that hasn’t been defined yet.
z += 1 # Raises a NameError
In this example, we’re trying to increment a variable z
that hasn’t been defined, which raises a NameError
. To avoid this, always ensure that a variable is defined before using it with these operators.
Incompatible Data Types
Another issue can arise when you try to use the += or -= operator with incompatible data types.
x = '5'
x += 2 # Raises a TypeError
Here, we’re trying to increment a string x
by an integer 2, which raises a TypeError
. The += operator cannot be used to add a string and an integer in Python. To avoid this, ensure that the data types are compatible when using these operators.
Using -= Operator with Lists and Strings
As we discussed in the previous section, the -= operator does not work with lists or strings in Python. Attempting to use it with these data types will raise a TypeError
.
list1 = [1, 2, 3]
list1 -= [2] # Raises a TypeError
str1 = 'Hello'
str1 -= 'o' # Raises a TypeError
In these examples, we’re trying to use the -= operator with a list and a string, which raises a TypeError
in both cases. To avoid this, remember that the -= operator can only be used with numeric data types in Python.
Understanding these common issues and how to avoid them can help you use the += and -= operators in Python more effectively and write cleaner, more efficient code.
Python Operators: A Closer Look
Before we delve deeper into the += and -= operators, it’s important to understand the basics of operators in Python. Operators are special symbols that carry out arithmetic or logical computation. The value or variable that the operator works on is called the operand.
Assignment Operators in Python
Assignment operators are used to assign values to variables. The most common assignment operator is the =
operator. For example:
x = 5
print(x) # Output: 5
In this example, the =
operator assigns the value 5 to the variable x
.
Shorthand Assignment Operators
Python also supports shorthand assignment operators, which perform an operation and assign the result to a variable in one step. The += and -= operators we’ve been discussing are examples of shorthand assignment operators.
x = 5
x += 1 # Equivalent to x = x + 1
print(x) # Output: 6
In this example, the +=
operator increments the value of x
by 1.
y = 10
y -= 2 # Equivalent to y = y - 2
print(y) # Output: 8
In this example, the -=
operator decrements the value of y
by 2.
Understanding these fundamentals of operators in Python is crucial for mastering the += and -= operators, and for writing efficient and readable code.
The Power of += and -= Operators in Python Programming
The += and -= operators in Python are not just simple tools for incrementing and decrementing variables. They have a much broader relevance in different areas of Python programming, such as in loops and data manipulation.
Using += and -= Operators in Loops
In loops, the += and -= operators can be used to increment or decrement a counter, which can control the flow of the loop.
# Using += in a for loop
counter = 0
for i in range(10):
counter += 1
print(counter) # Output: 10
In this example, we use the += operator to increment a counter in a for loop. The counter starts at 0 and is incremented by 1 in each iteration of the loop.
Using += and -= Operators in Data Manipulation
In data manipulation, the += operator can be used to append data to lists or concatenate strings, while the -= operator can adjust numeric data.
# Using += to append data to a list
list1 = [1, 2, 3]
list1 += [4, 5]
print(list1) # Output: [1, 2, 3, 4, 5]
In this example, we use the += operator to append the elements 4 and 5 to a list.
Understanding the relevance of the += and -= operators in these areas can help you leverage their power and write more efficient Python code.
Further Resources for Mastering += and -= Operators in Python
If you’re interested in learning more about the += and -= operators in Python, here are some resources that you might find helpful:
- Python Math Tutorial: Getting Started – Unlock the power of Python’s math module to solve complex mathematical problems.
Python Modulo: Using the Modulus Operator – Discover Python’s modulo operator for obtaining remainders in division.
Handling Infinite Values in Python – Discover Python infinity handling techniques for mathematical computations.
Python Operators – A comprehensive guide on Python operators, including the += and -= operators.
Python Assignment Operators Example– Tutorial provides examples of Python assignment operators in action.
Python Operators Tutorial – Covers all types of operators in Python, including arithmetic, logical, comparison, assignment, and bitwise operators.
Wrapping Up: Mastering += and -= Operators in Python
In this comprehensive guide, we’ve delved into the += and -= operators in Python, exploring their usage, benefits, and common issues.
We began with the basics, learning how to use the += and -= operators to increment and decrement variables. We then delved into more advanced usage, exploring how these operators work with different data types like lists and strings. Along the journey, we tackled common issues you might encounter when using these operators, such as undefined variables and incompatible data types, providing solutions for each issue.
We also looked at alternative approaches to incrementing and decrementing variables in Python, providing a broader perspective on the topic. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
+= and -= | Efficient, clean code | Requires variable to be defined |
x = x + 1 and y = y – 1 | Works without variable definition | More verbose |
Whether you’re a beginner just starting out with Python or an experienced developer looking to level up your skills, we hope this guide has given you a deeper understanding of the += and -= operators in Python. Happy coding!