Python Modulo: % Operator Usage Guide
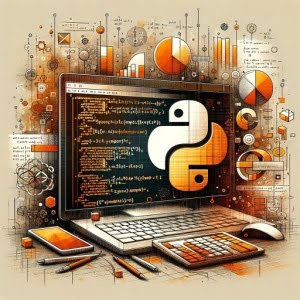
Do you find yourself grappling with the modulo operation in Python? Think of it as a clock that resets after hitting 12. The modulo operation, symbolized by ‘%’, gives you the remainder after division.
This comprehensive guide is designed to help you navigate the world of Python’s modulo operator, from its basic usage to advanced techniques. We’ll dive deep into the concept, explore various examples, and even troubleshoot common problems you might encounter.
So, let’s get started on this journey to mastering the modulo operation in Python.
TL;DR: How do I use the modulo operator in Python?
The modulo operation in Python is performed using the ‘%’ operator. It returns the remainder of a division operation. Here’s a simple example:
remainder = 10 % 3
print(remainder)
# Output:
# 1
In this example, we’re dividing 10 by 3. The division operation results in a quotient of 3 and a remainder of 1. The modulo operation, denoted by ‘%’, returns this remainder, which is 1.
For a more comprehensive understanding of the modulo operation, its applications, and how to use it in Python, keep reading!
Table of Contents
Understanding the Modulo Operation in Python
The modulo operation in Python is a mathematical operation that returns the remainder of a division. It’s denoted by the ‘%’ symbol. This operation is incredibly useful in various programming scenarios, from creating repeating patterns to checking if a number is even or odd.
Let’s break down a simple example:
remainder = 17 % 5
print(remainder)
# Output:
# 2
In this code, we’re dividing 17 by 5. While 5 goes into 17 three times (15), there’s a remainder of 2 left over. The modulo operation returns this remainder.
One of the main advantages of the modulo operation is its ability to wrap values around a range. This is especially useful when working with cyclic or repeating patterns. However, a common pitfall is misunderstanding the difference between the modulo operation and the division operation. While both are related, they serve different purposes: division returns the quotient, while modulo returns the remainder.
By mastering the modulo operation, you can solve a variety of programming challenges more efficiently and effectively.
Advanced Python Modulo Techniques
As you become more comfortable with Python’s modulo operation, you can start exploring more complex uses. Two common advanced uses of the modulo operation include determining whether a number is even or odd, and wrapping values within a range.
Determining Even or Odd Numbers
In Python, you can use the modulo operation to quickly check if a number is even or odd. An even number modulo 2 will always return 0, while an odd number modulo 2 will always return 1. Let’s look at an example:
num = 7
if num % 2 == 0:
print(f'{num} is even')
else:
print(f'{num} is odd')
# Output:
# 7 is odd
In this code, we’re using the modulo operation to check if the number 7 is even or odd. Since 7 modulo 2 equals 1, the code prints ‘7 is odd’.
Wrapping Values Within a Range
The modulo operation is also handy when you need to wrap values within a certain range. This is particularly useful in scenarios involving cyclic or repeating patterns. Here’s an example:
for i in range(10):
print(i % 3)
# Output:
# 0
# 1
# 2
# 0
# 1
# 2
# 0
# 1
# 2
# 0
In this example, we’re using the modulo operation to wrap values within the range of 0 to 2. The output repeats the sequence 0, 1, 2 as we iterate from 0 to 9.
Python’s divmod(): An Alternate Approach
Python offers another function, divmod()
, that can perform both division and modulo operations simultaneously. This function returns a tuple containing the quotient and the remainder.
Using divmod()
Here’s an example of how you can use the divmod()
function in Python:
division, remainder = divmod(17, 5)
print(f'Division: {division}, Remainder: {remainder}')
# Output:
# Division: 3, Remainder: 2
In this example, divmod(17, 5)
returns a tuple (3, 2)
, where 3 is the quotient of the division (17 divided by 5) and 2 is the remainder.
Benefits and Drawbacks of divmod()
The main benefit of using divmod()
is efficiency. It performs two operations (division and modulo) at once, which can be particularly useful when dealing with large numbers or complex calculations. However, the function also has its drawbacks. The main one being that it returns a tuple, which can be less intuitive to work with if you’re only interested in one of the values (either the quotient or the remainder).
When deciding whether to use the modulo operation or the divmod()
function, consider the needs of your specific task. If you need both the quotient and the remainder, divmod()
can be a more efficient choice. However, if you only need the remainder, using the modulo operation can make your code simpler and easier to read.
Troubleshooting Python’s Modulo Operation
While the modulo operation is a powerful tool in Python, it’s not without its pitfalls. Let’s discuss some common errors you might encounter and how to resolve them.
Division by Zero Error
One of the most common errors when using the modulo operation is the ZeroDivisionError
. This error occurs when you attempt to divide by zero, which is mathematically undefined. Here’s an example:
try:
print(10 % 0)
except ZeroDivisionError:
print('Error: Division by zero')
# Output:
# Error: Division by zero
In this example, we’re trying to divide 10 by 0 using the modulo operation. Python raises a ZeroDivisionError
, which we catch with a try/except
block and print a friendly error message.
Negative Numbers
Another potential pitfall is using the modulo operation with negative numbers. The result can be counter-intuitive if you’re not aware of how Python handles these cases. Here’s an example:
print(-10 % 3)
# Output:
# 1
Despite -10 being a negative number, the result is positive. This is because Python’s modulo operation always returns a number with the same sign as the divisor.
It’s important to keep these considerations in mind when using the modulo operation in Python. By understanding these potential pitfalls, you can write more robust and error-free code.
The Math Behind Python’s Modulo Operation
The modulo operation, represented by the ‘%’ symbol in Python, has its roots in mathematics. It’s a function that gives the remainder or signed remainder of a division, after one number is divided by another (called the divisor).
Let’s take a closer look at the mathematical concept behind the modulo operation:
print(10 % 3)
# Output:
# 1
In this example, we divide 10 by 3. The division results in a quotient of 3 and a remainder of 1. The modulo operation returns this remainder.
Modulo and Integer Division
The modulo operation is closely related to integer division in Python, represented by the ‘//’ operator. While the modulo operation returns the remainder of a division, integer division returns the largest whole number less than or equal to the division. Here’s an example:
print(10 // 3)
# Output:
# 3
In this example, 10 divided by 3 equals 3.3333. However, integer division returns the largest whole number less than or equal to the division, which is 3.
By understanding the mathematical principles behind the modulo operation, you can gain a deeper understanding of its function and uses in Python programming.
Modulo Operation in Real-World Programming
The modulo operation isn’t just a theoretical concept—it has practical applications in real-world programming tasks. Let’s explore some of these applications.
Cryptography and Modulo
In the field of cryptography, the modulo operation plays a crucial role in algorithms for encoding and decoding information. For instance, the RSA encryption algorithm uses modulo arithmetic to secure data transmission.
Generating Repeating Patterns
The modulo operation is also useful for generating repeating patterns in programming. For instance, you can use it to create cyclic animations or to distribute items evenly in a circular pattern.
for i in range(20):
print(i % 4)
# Output:
# 0
# 1
# 2
# 3
# 0
# 1
# 2
# 3
# ...
In this example, we’re using the modulo operation to create a repeating pattern of 0, 1, 2, 3.
Exploring Related Concepts
Once you have a solid understanding of the modulo operation, you might want to explore related concepts. Python offers a wealth of built-in operations and functions for you to discover. To get started, here are some online resourcecs:
- Python Math Logic: Simplified – Discover Python’s math module and its capabilities for handling numerical computations.
Symbol Usage in Python – Explore Python’s support for mathematical symbols like equals plus or minus.
Exploring Square Operations in Python – Discover Python’s built-in functions and operators for calculating squares easily.
Python Operators Explained – An elaborate guide to using and understanding various Python operators.
Python Operators and Expressions – In-depth resource covering Python operands, expressions and their effective use.
Python Operators Overview – Get a quick understanding of different Python operators from this beginner-friendly guide.
Wrapping Up Python’s Modulo Operation
In this guide, we’ve journeyed through the ins and outs of the modulo operation in Python. We’ve explored how it works, its basic usage, and even ventured into more advanced techniques.
We’ve seen how the modulo operation, represented by the ‘%’ symbol, can determine the remainder of a division operation. It’s a powerful tool in Python, used in various scenarios from checking if a number is even or odd to generating repeating patterns.
We’ve also delved into common issues, such as the ZeroDivisionError
and handling negative numbers. These are important considerations to keep in mind to ensure your code runs smoothly.
In addition to the modulo operation, we’ve discussed the divmod()
function, an alternative approach that performs both division and modulo at once. While it can be more efficient, especially with large numbers or complex calculations, it returns a tuple which might be less intuitive to work with.
Finally, we’ve touched on the real-world applications of the modulo operation, highlighting its role in cryptography and pattern generation. As a next step, consider exploring related concepts like integer division and bitwise operations to further expand your Python knowledge.
Remember, practice is key when it comes to mastering programming concepts. Keep experimenting with the modulo operation in your Python code, and soon it’ll become second nature.