Square Numbers in Python: 5 Easy Methods
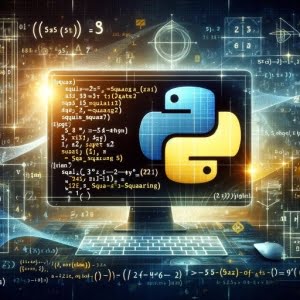
Are you grappling with how to square numbers in Python? You’re not alone. But there’s good news: Python, just like your good old calculator, is fully capable of squaring any number you throw at it. And it’s easier than you might think.
In this guide, we will walk you through the entire process of squaring numbers in Python. We’ll start with the basic exponentiation operator (**
) and then move on to more advanced methods.
So let’s dive right in and learn how to square numbers in Python!
TL;DR: How Do I Square a Number in Python?
Squaring a number in Python is straightforward. You can accomplish this using the exponentiation operator (
**
). You can get the square of 5 like this:5 ** 2
.
Here’s a simple example:
num = 5
square = num ** 2
print(square)
# Output:
# 25
In this example, we’ve defined a variable num
and assigned it the value 5. We then square num
using the exponentiation operator and print the result, which is 25.
If you’re interested in learning more about this, including some advanced methods of squaring numbers in Python, keep reading. We’ll cover everything you need to know!
Table of Contents
Squaring a Number: The Exponentiation Operator
Python provides several ways to square a number, but the simplest and most common method is using the exponentiation operator (**
). The syntax is straightforward: just take your number and follow it with **2
.
Here’s a basic example:
num = 7
square = num ** 2
print(square)
# Output:
# 49
In this example, we’ve assigned the value 7 to the variable num
. We then square num
using the **2
operation. The result, which is 49, is stored in the square
variable and then printed out.
Pros and Cons of the Exponentiation Operator
The exponentiation operator is simple and easy to use, making it perfect for beginners. It’s also highly readable, which is a big plus when you’re working on larger projects or collaborating with others.
However, it does have some limitations. For instance, it can only be used with numbers. If you try to use it with a list of numbers, you’ll get an error. But don’t worry, there are other methods for squaring a list of numbers, which we’ll cover in the ‘Alternative Approaches’ section.
Advanced Squaring in Python: pow and numpy.square
As you progress in your Python journey, you might come across situations where the exponentiation operator isn’t enough. Luckily, Python offers more advanced methods for squaring numbers, like the pow
function from the math library and the square
function from the numpy library.
Using the pow Function
The pow
function is part of Python’s built-in math
library. It takes two arguments: the base number and the exponent, and returns the base number raised to the power of the exponent.
Here’s how you can use it to square a number:
import math
num = 8
square = math.pow(num, 2)
print(square)
# Output:
# 64.0
In this example, we’ve imported the math
library and used the pow
function to square the number 8. The result is 64.0. Note that pow
returns a float, even when squaring integers.
Using numpy’s square Function
The square
function is part of the numpy library, a powerful tool for numerical computations in Python. This function can square individual numbers as well as entire arrays of numbers.
Here’s an example of how to use it:
import numpy as np
num = 9
square = np.square(num)
print(square)
# Output:
# 81
In this example, we’ve imported the numpy library (as np
for short) and used the square
function to square the number 9. The result is 81.
Pros and Cons of pow and numpy.square
Both the pow
function and numpy’s square
function offer more flexibility than the exponentiation operator. The pow
function can handle very large numbers and negative exponents, while numpy’s square
function can operate on entire arrays, making it ideal for data analysis tasks.
These functions do require you to import a library, which can slow down your code if you’re not using the other features of those libraries. Additionally, numpy’s
square
function might be overkill if you’re just squaring a single number.
List Comprehension and Lambda Functions
Python’s flexibility shines when it comes to squaring a list of numbers. Two powerful techniques you can use are list comprehension and lambda functions.
These methods provide concise and efficient ways to perform operations on a list, including squaring each number in the list.
Squaring a List with List Comprehension
List comprehension is a unique feature in Python that allows you to create a new list from an existing one by applying an operation to each element. Here’s how you can use it to square a list of numbers:
numbers = [1, 2, 3, 4, 5]
squares = [num ** 2 for num in numbers]
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, we’ve created a list of squares from our original list of numbers using list comprehension. The expression num ** 2 for num in numbers
generates a new list by squaring each number in the numbers
list.
Squaring a List with Lambda Functions
Lambda functions, also known as anonymous functions, allow you to define small, one-off functions. Combined with the map
function, you can use a lambda function to square a list of numbers:
numbers = [1, 2, 3, 4, 5]
squares = list(map(lambda num: num ** 2, numbers))
print(squares)
# Output:
# [1, 4, 9, 16, 25]
In this example, the lambda function lambda num: num ** 2
squares each number in the numbers
list. The map
function applies this lambda function to each element of the numbers
list, and the list
function converts the result back into a list.
Pros and Cons of List Comprehension and Lambda Functions
List comprehension and lambda functions are powerful tools for working with lists in Python. They’re concise, readable, and efficient, especially when dealing with large lists.
However, they can be harder to understand for beginners compared to the simpler methods we discussed earlier. If you’re new to Python, you might want to stick with the exponentiation operator or the pow
and square
functions until you’re more comfortable with the language.
Troubleshooting Common Errors
While squaring numbers in Python is generally straightforward, you may encounter some common errors. Let’s discuss a few of these potential pitfalls and how to avoid them.
Error: Unsupported Operand Types
One common error when attempting to square a number in Python is the TypeError: unsupported operand type(s) for ** or pow(): 'list' and 'int'
. This error occurs when you try to use the exponentiation operator or the pow
function on a list of numbers.
For example, the following code will produce an error:
numbers = [1, 2, 3, 4, 5]
squares = numbers ** 2
# Output:
# TypeError: unsupported operand type(s) for ** or pow(): 'list' and 'int'
In this case, Python is telling you that it doesn’t know how to use the **
operator with a list and an integer.
To fix this error, you can use list comprehension or the map
function with a lambda function, as we discussed in the ‘Alternative Approaches’ section.
Error: Using pow Without Importing Math
Another common error is forgetting to import the math
library before using the pow
function. If you try to use pow
without importing math
, you’ll get a NameError
.
Here’s an example that produces this error:
num = 6
square = math.pow(num, 2)
# Output:
# NameError: name 'math' is not defined
To fix this error, simply add import math
at the beginning of your code.
Best Practices
When squaring numbers in Python, keep the following best practices in mind:
- Use the exponentiation operator (
**
) for simple cases where you’re only squaring a single number. - Use the
pow
function or numpy’ssquare
function for more complex cases, such as when you need to handle very large numbers or perform operations on entire arrays. - When squaring a list of numbers, consider using list comprehension or a lambda function with
map
for a more Pythonic approach. - Always remember to import the necessary libraries before using their functions.
Python and Mathematical Operations
Python is a versatile language, widely used in a variety of fields, including data analysis, machine learning, web development, and more. One of the reasons for Python’s popularity is its robust support for mathematical operations.
Python can perform all the basic arithmetic operations you’d expect, such as addition (+
), subtraction (-
), multiplication (*
), and division (/
). But Python also supports more advanced operations, like modulus (%
), floor division (//
), and exponentiation (**
).
Expanding Your Python Math Skills
Squaring numbers is just the tip of the iceberg when it comes to mathematical operations in Python. Python supports a wide range of mathematical operations and functions, from basic arithmetic to complex functions in libraries like math
and numpy
.
If you’re interested in deepening your understanding of math in Python, consider exploring topics like:
- Other mathematical operations in Python, such as cube, square root, and logarithm
- The
math
andnumpy
libraries, which offer a wide range of mathematical functions and operations - How mathematical operations are used in data analysis and machine learning
Further Resources for Math in Python
For more insight on Python Math module, Click Here for a deep dive to unleash its full potential for numerical computations.
And for more online resources on related topics, check out these articles:
- Simplifying Modulus Calculation in Python – Master Python modulo operations for cyclic computations and indexing.
Square Root Operations in Python – Master Python techniques for computing square roots accurately and efficiently.
Python Mathematical Functions Tutorial – Engage with a video guide on utilizing mathematical functions in Python.
Applying Math Concepts with Python – Learn how to employ mathematical concepts in Python coding.
List of Python Math Modules and Functions – Access a comprehensive list of modules and functions in Python’s math library.
Wrapping Up:
In this comprehensive guide, we explored various methods to square numbers in Python, from the basic exponentiation operator to more advanced functions in the math
and numpy
libraries.
We also delved into alternative approaches like list comprehension and lambda functions for squaring lists of numbers.
Next we discussed common errors and best practices when squaring numbers in Python, and then we briefly touched on some other Python math operations.
Whether you’re a beginner or an experienced coder, understanding how to square numbers in Python is a valuable skill. As you continue to learn and grow in your Python journey, remember to experiment with different methods, learn from your mistakes, and never stop exploring. Happy coding!