How To Find a Square Root in Python
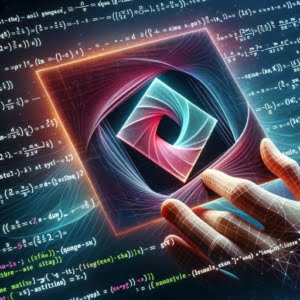
Ever wondered how to calculate square roots in Python? Python, with its powerful and versatile nature, simplifies this process significantly.
This article is a comprehensive guide to understanding and calculating square roots in Python. By the end of this guide, you’ll be proficient in computing square roots using various methods in Python. So, let’s embark on this journey of demystifying square roots in Python!
TL;DR: How can I calculate square roots in Python?
Python provides a built-in
math
module with asqrt()
function to calculate the square root of a given number. Here’s a simple example:
import math
# calculate the square root of 16
print(math.sqrt(16))
# Output: 4.0
This code will output 4.0
, which is the square root of 16. For more advanced methods, background, tips, and tricks, continue reading the rest of the article.
Table of Contents
Understanding Square Roots
Before we delve into Python’s methods for calculating square roots, it’s essential to understand what a square root is.
In mathematics, a square root of a number is a value that, when multiplied by itself, gives the original number. For instance, the square root of 9 is 3 because when 3 is multiplied by itself, it results in 9.
Example of square root concept:
# square root of 9
sqrt_9 = 9 ** 0.5
print(sqrt_9)
# multiplying square root by itself
result = sqrt_9 * sqrt_9
print(result)
This code will output 3.0
for the square root of 9 and 9.0
for the result of multiplying the square root by itself.
Calculating Square Roots in Python
Python simplifies the process of calculating square roots with its built-in math
module, which includes a sqrt()
method for this purpose.
import math
# calculate the square root of 16
print(math.sqrt(16))
# Output: 4.0
Executing this code returns 4.0
, the square root of 16.
The Importance of Precision
Precision is paramount in Python’s mathematical computations. The math
module guarantees a high level of precision, ensuring accurate results, especially when working with square roots that often result in irrational numbers.
Example of precision in Python’s mathematical computations:
import math
# calculate the square root of 10
print(math.sqrt(10))
This code will output 3.1622776601683795
, demonstrating Python’s precision in handling irrational numbers.
Alternative Square Root Calculation Methods in Python
In addition to the sqrt
function, Python also allows you to calculate the square root of a number using the exponentiation operator (**).
Any number to the power of 0.5 will give you the square root of that number. This can be taken advantage of with the exponentiation operator to calculate the square root.
# calculate the square root of 36
print(36 ** 0.5)
Running the above code will output 6.0
, the square root of 36.
Comparing the Two Methods
Both the sqrt
function and the exponentiation operator have their own advantages and disadvantages. The sqrt
function is straightforward and easy to use, but it requires you to import the math
module. The exponentiation operator, while not requiring any imports, may not be as intuitive to someone reading your code.
Troubleshooting: Common Errors and Their Solutions
While calculating square roots in Python, you might encounter some common errors. For instance, Python will throw a ValueError if you attempt to calculate the square root of a negative number using the math.sqrt
function.
This is because the square root of a negative number is undefined in the real number system. Python’s cmath
module, which can perform complex number operations, provides a solution to this.
import cmath
# calculate the square root of -1
print(cmath.sqrt(-1))
The above code will output 1j
, which is the imaginary unit.
Python: A Powerhouse for Mathematical Computations
While our focus has been on the calculation of square roots, it’s vital to note that Python’s capabilities for mathematical computations extend far beyond this.
Python is an adept tool for handling a wide array of mathematical operations, making it a suitable choice for tasks from simple arithmetic to complex mathematical computations.
Consider some Python libraries for math computations:
Library | Description |
---|---|
NumPy | Supports large, multi-dimensional arrays and matrices, along with a suite of mathematical functions to operate on these arrays. |
SciPy | Offers efficient and numerically accurate mathematical algorithms. |
Pandas | Provides high-performance, user-friendly data structures and data analysis tools. |
Matplotlib | Designed to create static, animated, and interactive visualizations in Python. |
Python boasts a multitude of libraries designed to simplify and optimize mathematical computations. Here are a few noteworthy ones:
- NumPy: This library supports large, multi-dimensional arrays and matrices, along with a suite of mathematical functions to operate on these arrays.
SciPy: Built on NumPy, SciPy is a library dedicated to scientific and technical computing. It offers efficient and numerically accurate mathematical algorithms.
Pandas: This library provides high-performance, user-friendly data structures and data analysis tools, making it ideal for mathematical computations on large datasets.
Matplotlib: This is a plotting library designed to create static, animated, and interactive visualizations in Python.
Python’s capabilities for mathematical computations are expansive and robust. Whether you’re performing basic calculations or developing complex mathematical models, Python provides the necessary tools and libraries to accomplish the task efficiently and accurately.
Its straightforward syntax and comprehensive support for mathematical operations make it a preferred choice for mathematicians, scientists, engineers, and anyone else who needs to perform mathematical computations.
Further Resources for Math in Python
To help you learn more about the Python Math Module, here are some helpful resources:
- Python Math: A Comprehensive Guide – Dive into Python’s math module to handle mathematical tasks with ease and precision.
Square Calculation in Python – Explore Python techniques for computing squares efficiently for mathematical operations.
Exploring Floor Division in Python – Master integer division techniques in Python for handling numerical computations.
Guide to Python Math Functions – Learn how to use various mathematical functions available in Python.
Python’s Math Module – Get a comprehensive understanding of the math module in Python.
Tutorial on Python Arithmetic Operators – Understand the use and application of different arithmetic operators in Python.
Using these resources to learn more about the Python Math Module will enhance your coding skills. This knowledge will help you solve complex math problems in your Python projects more easily.
Wrapping Up: Mastering Square Roots in Python
We’ve embarked on an enlightening journey through the realm of calculating square roots in Python, starting from the basic principles and advancing to more sophisticated techniques. Along the way, we’ve discovered how Python’s math
module and the sqrt
function simplify this fundamental mathematical operation. We’ve also highlighted how the exponentiation operator (**), while less intuitive, provides a swift and import-free alternative.
Our exploration didn’t end there. We ventured into the expansive landscape of Python’s mathematical capabilities, delving into its extensive array of libraries and tools for mathematical computations. From NumPy
and SciPy
to Pandas
and Matplotlib
, Python has proven to be a formidable force for tasks ranging from basic arithmetic to intricate scientific computations.
Whether you’re a Python novice or a seasoned programmer, we hope this guide has illuminated the process of calculating square roots in Python. So, keep exploring, keep coding, and above all, enjoy the journey!