Python Integer Division: How To Use the // Floor Operator
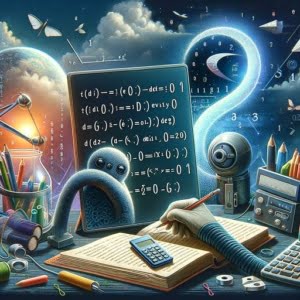
Python, a powerful and flexible language, is a mainstay in many fields, from web development to data processing. To truly tap into its potential, it’s crucial to understand its basic mathematical operations, including division.
In this blog post, we’re setting out on a deep dive into Python’s division operations. We’ll explore the different ways to perform division, including using built-in functions, handling user input, and leveraging powerful Python libraries.
So, if you’ve ever found yourself scratching your head over Python integer division, or just want to polish your Python skills, buckle up! This is going to be a journey worth embarking on.
TL;DR: How do I perform Integer division in Python?
Python provides two types of division – float and integer. The / operator is used for float division, while the // operator is used for integer division. For example, 10 / 3 results in 3.3333333333333335 (float division), while 10 // 3 results in 3 (integer division). Read on for more advanced methods, background, tips and tricks.
# Float division
print(10 / 3) # Output: 3.3333333333333335
# Integer division
print(10 // 3) # Output: 3
Table of Contents
Performing Division in Python
Let’s kick things off with the basics. In Python, there are two primary types of division at your disposal – float and integer. You might wonder, what’s the difference?
When you use float division, you end up with floating-point numbers as a result. On the other hand, integer division, our primary focus in this post, returns the quotient as a whole number.
Python simplifies this by using different operators for each. The / operator is used for float division, while the // operator is used for integer division. For instance, if you were to divide 10 by 3 using the / operator, you’d get 3.3333333333333335. But if you use the // operator for the same operation, you’d get 3. That’s Python integer division for you!
There’s a fascinating quirk about Python’s integer division – when you’re dealing with negative results, Python rounds towards negative infinity. So, if you were to divide -10 by 3, you’d get -4, not -3 as you might expect.
Divison Operators
In addition to understanding these types of division, it’s also important to know how to use the operators that make them possible. Python offers three basic division operators – /, //, and %.
The / operator performs float division. So, if you’re looking for a result that includes decimal points, this is the operator to use. For example, 10 / 3 would give you 3.3333333333333335.
Next up, we have the // operator. This is the one that performs integer division. It’s perfect when you want your result to be a whole number. For example, 10 // 3 would give you 3, not 3.33. It’s a quick and efficient way to perform integer division without creating unnecessary floating-point numbers.
Lastly, we have the % operator. This one returns the remainder of a division. So, if you were to divide 10 by 3, the remainder would be 1, and that’s exactly what 10 % 3 would give you.
Operator | Usage | Output |
---|---|---|
/ | Float division (10 / 3) | 3.3333333333333335 |
// | Integer division (10 // 3) | 3 |
% | Remainder of a division (10 % 3) | 1 |
Here’s a quick Python code snippet to illustrate these operators in action:
# Float division
print(10 / 3) # Output: 3.3333333333333335
# Integer division
print(10 // 3) # Output: 3
# Remainder of a division
print(10 % 3) # Output: 1
Custom Division Functions in Python
Python’s flexibility and customization extend to its division operations too. You can create your own custom division functions to handle different types of numbers and situations.
Creating a custom division function is as simple as defining a function using the / operator for floating-point division or the // operator for integer division.
For instance, if you wanted a function that performs integer division, you could define it like this:
def integer_division(dividend, divisor):
return dividend // divisor
Then, you can call this function anytime you need to perform integer division. It’s a great way to keep your code clean and organized.
Divmod to integer divide and get remainder
But Python takes it a step further with the divmod function. This handy function simultaneously provides the quotient and the remainder of a division, enhancing code readability and efficiency. Here’s how you can use it:
quotient, remainder = divmod(10, 3)
print('Quotient:', quotient)
print('Remainder:', remainder)
This will output:
Quotient: 3
Remainder: 1
Differences between Python 2.x and Python 3.x in Division Operations
Python has evolved significantly since its inception, with each version introducing new features and enhancements. One aspect that has seen a change over the years is how Python handles division.
In Python 2.x, if you used the / operator with two integers, it would perform integer division. So, 10 / 3 would yield 3, not 3.33. However, Python 3.x brought about a change. In Python 3.x, the / operator always performs float division, regardless of the operands. So, 10 / 3 in Python 3.x would give you 3.33.
This might seem like a minor modification, but it can significantly impact your Python code. If you’re accustomed to Python 2.x’s way of handling division and are unaware of the change in Python 3.x, you might encounter unexpected results in your calculations.
Here’s a Python code snippet to illustrate the difference:
# Python 2.x
print 10 / 3 # Output: 3
# Python 3.x
print(10 / 3) # Output: 3.3333333333333335
Using NumPy for Division in Python
Python’s robust ecosystem of libraries is one of its standout features, simplifying and optimizing a variety of tasks. In the realm of numerical operations, libraries such as NumPy and SciPy are game-changers. These libraries are capable of handling large datasets and performing complex calculations with ease, making them invaluable tools for Python programmers.
One feature of NumPy that deserves special mention is the numpy.divide() function. This function can perform element-wise division on Python lists or arrays. This means that instead of writing a loop to divide each element in a list by a number, you can use numpy.divide() to accomplish the task in a single line. Here’s a quick example to illustrate this:
import numpy as np
# Create a numpy array
arr = np.array([10, 20, 30, 40])
# Divide each element by 10
result = np.divide(arr, 10)
print(result) # Output: array([1., 2., 3., 4.])
As you can see, numpy.divide() simplifies the process of performing division on a list of numbers. This can be incredibly beneficial when dealing with large-scale data or when you need to execute your code quickly.
Further Resources for Python Math
To deepen your comprehension and application of Python Math, here are some resources that could prove instrumental:
- Exploring Python’s Math Module – Explore Python’s math module for efficient mathematical operations and functions.
Square Root in Python: Finding Square Roots – Dive into various methods for finding the square root of a number in Python.
Simplifying Incrementing by 1 in Python – Learn Python incrementation techniques for increasing values by one in code.
Comprehensive Guide on NumPy Arrays – Get a thorough understanding of working with arrays in NumPy.
Exploring Array Functions in Python’s NumPy Module – Learn about array functionalities in detail within the NumPy library.
Offical Reference Documentation for NumPy – Access the complete set of official documentation for the NumPy library.
By spending time with these, you will get better at Python Math, which can help you solve tough math problems in your coding projects.
Conclusion
And there you have it, a comprehensive guide to mastering division operations in Python. We’ve navigated through the various types of division – float and integer – and the operators that enable them. We’ve ventured into the realm of custom functions, empowering you to create your own solutions for diverse division scenarios. Lastly, we’ve explored how libraries like NumPy can streamline your division tasks, particularly when dealing with large datasets.
Refine your coding skills using our comprehensive Python syntax reference.
So, when you’re faced with a division task in Python next time, remember the lessons from this guide. Whether you’re using the / operator for float division, the // operator for Python integer division, or crafting your own custom division function, you’ll be well-prepared to tackle it. Here’s to many more coding adventures!