Python Math Module: A Detailed Walkthrough
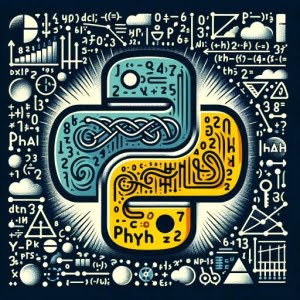
Are you wrestling with mathematical operations in Python? You’re not alone. Many developers find Python’s math module a bit challenging to grasp, but we’re here to help.
Think of Python’s math module as a handy calculator – a tool that can solve complex mathematical problems with ease. It’s a powerful way to extend the functionality of your Python scripts, making the math module extremely popular for handling mathematical operations.
In this guide, we’ll walk you through the process of using Python’s math module, from the basics to more advanced techniques. We’ll cover everything from importing the module, using its functions, to troubleshooting common issues.
Let’s dive in and start mastering Python’s math module!
TL;DR: How Do I Use Python’s Math Module?
To use Python’s math module, you first need to import it using
import math
. Then, you can use its functions to perform mathematical operations, such asmath.sqrt(16)
.
For example, to calculate the square root of a number:
import math
print(math.sqrt(16))
# Output:
# 4
In this example, we import the math module and use its sqrt
function to calculate the square root of 16, which outputs 4.
This is a basic way to use Python’s math module, but there’s much more to learn about performing mathematical operations in Python. Continue reading for a more detailed guide on how to use Python’s math module, including advanced techniques and alternative approaches.
Table of Contents
- Python Math Module: A Beginner’s Guide
- Python Math Module: Intermediate Techniques
- Exploring Alternative Math Libraries
- Overcoming Challenges with Python’s Math Module
- The Fundamentals of Python’s Math Module
- The Impact of Python’s Math Module in Data Analysis and Machine Learning
- Wrapping Up: Mastering Python’s Math Module
Python Math Module: A Beginner’s Guide
Let’s start with the basics: how to import and use Python’s math module. This module is like a toolbox filled with functions that help us perform mathematical operations in Python.
First, you need to import the module. In Python, we use the import
keyword to do this. Here’s how you can import the math module:
import math
Once the module is imported, you can start using its functions. Let’s try a simple operation: calculating the square root of a number.
import math
print(math.sqrt(16))
# Output:
# 4
In the example above, we used the sqrt
function from the math module to calculate the square root of 16, which outputs 4.
The math module is packed with a variety of functions that can help you perform complex mathematical operations. Some of the most commonly used functions include math.pow()
, math.pi
, math.log()
, and many more.
However, it’s important to note that while the math module is powerful, it also has its limitations. For instance, it doesn’t support complex numbers, and certain functions may not work with very large or very small numbers. But don’t worry, we’ll cover how to handle these issues in the ‘Troubleshooting and Considerations’ section of this guide.
Common Math Module Functions
Here’s a look at some of the more frequently used functions or methods that the math module offers in Python.
Function | Category | Description |
---|---|---|
math.sqrt(x) | Arithmetic | Returns the square root of x |
math.pow(x, y) | Arithmetic | Returns x raised to the power y |
math.pi | Constants | The mathematical constant π (pi), as a float |
math.e | Constants | The mathematical constant e , as a float |
math.log(x[, base]) | Logarithmic | Returns the logarithm of x to the specified base . If the base is not specified, it returns the natural logarithm |
math.exp(x) | Exponential | Returns e raised to the power x |
math.sin(x) | Trigonometry | Returns the sine of x radians |
math.cos(x) | Trigonometry | Returns the cosine of x radians |
math.radians(x) | Angle conversion | Converts x from degrees to radians |
math.degrees(x) | Angle conversion | Converts x from radians to degrees |
math.factorial(x) | Combinatorics | Returns the factorial of x (an exact integer >= 0) |
math.ceil(x) | Rounding | Returns the smallest integer greater than or equal to x |
math.floor(x) | Rounding | Returns the largest integer less than or equal to x |
math.tan(x) | Trigonometry | Returns the tangent of x radians |
math.sinh(x) | Hyperbolic | Returns the hyperbolic sine of x |
math.cosh(x) | Hyperbolic | Returns the hyperbolic cosine of x |
math.tanh(x) | Hyperbolic | Returns the hyperbolic tangent of x |
math.asin(x) | Inverse Trigonometry | Returns the arcsine of x in radians |
math.acos(x) | Inverse Trigonometry | Returns the arccosine of x in radians |
math.atan(x) | Inverse Trigonometry | Returns the arctangent of x in radians |
math.gamma(x) | Special Functions | Returns the Gamma function at x |
math.lgamma(x) | Special Functions | Returns the natural logarithm of the absolute value of the Gamma function at x |
math.hypot(x, y) | Euclidean Distance | Returns the Euclidean norm, sqrt(x*x + y*y) |
math.gcd(a, b) | Number Theory | Returns the greatest common divisor of the integers a and b |
math.isfinite(x) | Numeric Utilities | Returns True if x is neither an infinity nor a NaN |
math.isinf(x) | Numeric Utilities | Returns True if x is a positive or negative infinity, and False otherwise |
math.isnan(x) | Numeric Utilities | Returns True if x is a NaN, and False otherwise |
math.fsum(iterable) | Numeric Utilities | Returns an accurate floating point sum of values in the iterable |
math.copysign(x, y) | Numerical Operations | Returns x with the sign of y |
math.fabs(x) | Numeric Utilities | Returns the absolute value of x |
math.modf(x) | Numerical Operations | Returns the fractional and integer parts of x |
math.frexp(x) | Numerical Operations | Returns the mantissa and exponent of x as the pair (m, e) |
math.ldexp(x, i) | Numerical Operations | Returns x * (2**i) , computing this to machine precision |
math.asinh(x) | Inverse Hyperbolic | Returns the inverse hyperbolic sine of x |
math.acosh(x) | Inverse Hyperbolic | Returns the inverse hyperbolic cosine of x |
math.atanh(x) | Inverse Hyperbolic | Returns the inverse hyperbolic tangent of x |
math.fmod(x, y) | Numeric Utilities | Returns the remainder when x is divided by y |
math.remainder(x, y) | Numeric Operations | Returns the IEEE 754-style remainder of x with respect to y |
math.trunc(x) | Numeric Utilities | Returns x truncated to an Integral |
math.perm(n, k) | Combinatorics | Returns the number of ways to choose k items from n items without repetition and without order |
math.comb(n, k) | Combinatorics | Returns the number of ways to choose k items from n items without repetition and with order |
math.nan | Constants | A floating-point NaN (Not a Number) value |
math.inf | Constants | A floating-point positive infinity |
math.tau | Constants | The circumference-to-diameter ratio of a circle, exactly 2 * math.pi |
math.ulp(x) | Numeric Utilities | Returns the value of the least significant bit of the float x |
math.nextafter(x, y) | Numeric Utilities | Returns the next representable floating-point value after x towards y |
math.expm1(x) | Exponential | Returns exp(x) - 1 |
math.log1p(x) | Logarithmic | Returns log(1 + x) |
math.log2(x) | Logarithmic | Returns the base-2 logarithm of x |
math.dist(p, q) | Euclidean Distance | Returns the Euclidean distance between two points in either 2 or more dimensions |
math.atan2(y, x) | Trigonometry | Returns atan(y / x) in radians |
math.isqrt(n) | Numeric Utilities | Return the integer square root of the nonnegative integer n |
math.prod(iterable) | Numeric Utilities | Return the product of all items in the iterable |
After going through the full list, we now have outlined almost all the functions provided by the math
module in Python. It should be noted that for more complex calculations, we should understand how these functions work and when we should use each one. This makes Python a very powerful tool for mathematical calculations.
Python Math Module: Intermediate Techniques
Now that we’ve covered the basics and given you a list of the functions available, let’s delve into more complex functions in Python’s math module. These functions can help you solve more intricate mathematical problems, such as trigonometric or logarithmic calculations.
Trigonometric Functions
Python’s math module provides functions to perform trigonometric operations. Here’s how you can calculate the sine, cosine, and tangent of an angle (in radians):
import math
angle = math.pi / 4 # 45 degrees
print(math.sin(angle))
print(math.cos(angle))
print(math.tan(angle))
# Output:
# 0.7071067811865475
# 0.7071067811865476
# 0.9999999999999999
In the example above, we calculate the sine, cosine, and tangent of a 45-degree angle. The results are approximately 0.707, 0.707, and 1, respectively.
Logarithmic Functions
The math module also provides functions to perform logarithmic calculations. Here’s an example of how to calculate the natural logarithm and the logarithm base 10 of a number:
import math
print(math.log(10)) # natural logarithm
print(math.log10(10)) # base 10 logarithm
# Output:
# 2.302585092994046
# 1.0
In this example, we calculate the natural logarithm and the logarithm base 10 of 10. The results are approximately 2.30 and 1, respectively.
Rounding and Absolute Value Functions
Python’s math
module provides several functions for rounding numbers and getting their absolute values. Knowing when to use each one can help you manipulate numeric data more effectively.
The math.floor()
function returns the largest integer less than or equal to a given number:
import math
print(math.floor(3.5)) # Output: 3
Meanwhile, the math.ceil()
function returns the smallest integer greater than or equal to a given number:
import math
print(math.ceil(3.5)) # Output: 4
The math.fabs()
function computes the absolute value of a number:
import math
print(math.fabs(-3.5)) # Output: 3.5
Exponential and Power Functions
The math module also includes various functions for working with exponential and power operations. Below are examples of how to use these key functions.
The math.pow()
function returns a number raised to the power of a second number:
import math
print(math.pow(2, 3)) # Output: 8.0
On the other hand, math.exp()
returns e
(the base of natural logarithms) raised to the power of a given number, which is a common operation in scientific calculations:
import math
print(math.exp(1)) # Output: 2.718281828459045
These examples highlight the versatility of Python’s math
module and the wide range of mathematical operations you can perform, making it a valuable tool for anyone dealing with numeric data in Python.
Exploring Alternative Math Libraries
While Python’s math module is a powerful tool for mathematical operations, it’s not the only option available. Libraries like numpy and scipy offer additional functionalities and can sometimes be more efficient, especially when working with large datasets or complex mathematical computations.
Numpy: A Powerful Alternative
Numpy, short for ‘Numerical Python’, is a library that provides support for large, multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.
Here’s an example of how you can perform mathematical operations using numpy:
import numpy as np
# Creating an array
arr = np.array([1, 2, 3, 4, 5])
# Performing mathematical operations
print(np.sqrt(arr))
print(np.log(arr))
# Output:
# [1. 1.41421356 1.73205081 2. 2.23606798]
# [0. 0.69314718 1.09861229 1.38629436 1.60943791]
In the above example, we first create a numpy array. Then, we use numpy’s sqrt
and log
functions to calculate the square root and natural logarithm of each element in the array. The results are arrays of the calculated values.
Scipy: Advanced Mathematical Computations
Scipy, standing for ‘Scientific Python’, builds on numpy and provides more advanced functions for mathematical computations. It’s particularly useful for tasks like integration, differentiation, and optimization.
Here’s an example of how you can solve a mathematical optimization problem using scipy:
from scipy.optimize import minimize
# Objective function
def objective(x):
return x[0]**2 + x[1]**2
# Initial guess
x0 = [1, 1]
# Call the minimize function
res = minimize(objective, x0)
print(res.x)
# Output:
# [1.88846401e-08 1.88846401e-08]
In this example, we define an objective function that we want to minimize, provide an initial guess, and then call scipy’s minimize
function. The result is the values of x
that minimize our objective function.
Both numpy and scipy are powerful alternatives to Python’s math module. They provide a wide range of mathematical functions and are particularly useful for more complex tasks or when working with large datasets. However, for many common mathematical operations, Python’s math module is more than sufficient.
Overcoming Challenges with Python’s Math Module
Like any tool, Python’s math module has its quirks and limitations. In this section, we’ll discuss some common issues you might encounter when using the math module, along with solutions and workarounds.
Handling Complex Numbers
One common issue is that Python’s math module does not support complex numbers. If you try to perform an operation that results in a complex number, you’ll get a ValueError
. Here’s an example:
import math
try:
print(math.sqrt(-1))
except ValueError as e:
print(e)
# Output:
# math domain error
In the example above, we try to calculate the square root of -1, which should result in an imaginary number. However, the math module raises a ValueError
.
To handle complex numbers, you can use Python’s cmath
module, which supports complex numbers:
import cmath
print(cmath.sqrt(-1))
# Output:
# 1j
In this example, we use the cmath
module to calculate the square root of -1, which correctly outputs 1j
, the imaginary unit.
Dealing with Large Numbers
Another issue arises when dealing with very large numbers. Some functions in the math module, like math.factorial()
, can quickly result in numbers that are too large to handle.
import math
try:
print(math.factorial(10000))
except OverflowError as e:
print(e)
# Output:
# int too large to convert to float
In the example above, we try to calculate the factorial of 10,000, which results in an OverflowError
.
To handle such large numbers, you can use libraries like numpy
or scipy
, which are designed to handle large datasets efficiently.
These are just a few examples of the challenges you might face when using Python’s math module. By understanding these issues and knowing how to work around them, you can use the math module more effectively in your Python scripts.
The Fundamentals of Python’s Math Module
To fully appreciate the power of Python’s math module, it’s important to understand its background and the mathematical concepts it’s built upon.
Python’s math module is a standard library that provides mathematical functions and constants. It’s built on the C library functions for mathematical operations, ensuring efficiency and precision.
Here are some of the key features of Python’s math module:
- Mathematical Constants: The module provides mathematical constants, like
math.pi
for the mathematical constant pi andmath.e
for the mathematical constant e.
import math
print(math.pi)
print(math.e)
# Output:
# 3.141592653589793
# 2.718281828459045
In the example above, we print the constants pi and e, which output approximately 3.142 and 2.718, respectively.
- Arithmetic Functions: The math module includes standard arithmetic functions like
math.pow(x, y)
for raising x to the power y, andmath.sqrt(x)
for calculating the square root of x. Trigonometric Functions: The module provides functions for trigonometric calculations, like
math.sin(x)
,math.cos(x)
, andmath.tan(x)
.Hyperbolic Functions: The math module also includes functions for hyperbolic calculations, like
math.sinh(x)
,math.cosh(x)
, andmath.tanh(x)
.Logarithmic Functions: The module provides functions for logarithmic calculations, like
math.log(x)
for the natural logarithm andmath.log10(x)
for the base 10 logarithm.
These are just a few examples of the many functions available in Python’s math module. By mastering these functions, you can perform a wide range of mathematical operations in your Python scripts.
The Impact of Python’s Math Module in Data Analysis and Machine Learning
Python’s math module isn’t just for simple arithmetic or complex mathematical calculations. It also plays a crucial role in fields like data analysis and machine learning. Let’s explore how.
Data Analysis
In data analysis, mathematical operations are used to clean, analyze, and visualize data. Python’s math module provides the basic mathematical functions needed for these tasks. For instance, math.log()
can be used to transform skewed data, while math.sqrt()
can be used to calculate standard deviations.
Machine Learning
Machine learning involves a lot of mathematics, from simple arithmetic to complex calculus and linear algebra. Python’s math module is often used in the preprocessing and training stages of machine learning. For example, the math.exp()
function can be used in the activation function of a neural network.
Expanding Your Horizons: Numpy, Scipy, Pandas
While Python’s math module is powerful, you might need more advanced tools as you delve deeper into data analysis and machine learning. Libraries like numpy, scipy, and pandas provide more advanced mathematical functions and data structures designed for these fields.
For instance, numpy supports multi-dimensional arrays and matrices, making it ideal for linear algebra operations. Scipy builds on numpy and provides additional functions for tasks like integration, differentiation, and optimization. Pandas, on the other hand, provides data structures and functions designed to make data analysis fast and easy.
Further Resources for Mastering Python Math
If you’re interested in learning more about Python’s math module and other related libraries, here are some resources to help you on your journey:
- Simplifying Integer Division in Python – Explore Python’s floor division operator for obtaining integer results in division.
Python’s Official Documentation on Math Module provides a comprehensive list of the module’s functions and constants.
Numpy’s Official Documentation provides a detailed guide on how to use numpy’s functions and data structures.
In-depth Understanding of Python’s Math Module – Gain insights on how to use Python’s Math module to perform mathematical tasks.
Wrapping Up: Mastering Python’s Math Module
In this comprehensive guide, we’ve journeyed through the world of Python’s math module, a powerful tool for mathematical operations in Python.
We began with the basics, learning how to import and use the math module for simple arithmetic operations. We then ventured into more advanced territory, exploring complex functions for trigonometric and logarithmic calculations.
Along the way, we tackled common challenges you might face when using the math module, such as handling complex numbers and dealing with large numbers, providing you with solutions for each issue.
We also looked at alternative approaches to performing mathematical operations in Python, comparing Python’s math module with other libraries like numpy and scipy. Here’s a quick comparison of these libraries:
Library | Speed | Complexity | Use Case |
---|---|---|---|
Python Math Module | Fast | Low | Simple to moderate mathematical operations |
Numpy | Fast | Moderate | Large arrays, matrices, and complex calculations |
Scipy | Fast | High | Advanced mathematical computations |
Whether you’re just starting out with Python’s math module or you’re looking to level up your mathematical skills in Python, we hope this guide has given you a deeper understanding of the math module and its capabilities.
With its balance of simplicity and power, Python’s math module is a key tool for any Python programmer. Now, you’re well equipped to tackle any mathematical challenge in Python. Happy coding!