Clean Installs with NPM | Step-by-Step Guide
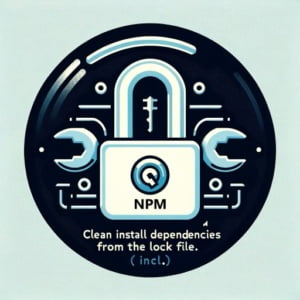
Performing a clean install with npm can be a lifesaver, especially when troubleshooting dependency issues. Here at IOFLOOD, we’ve faced this challenge multiple times during our development workflows. To aid others with this challenge, we have provided step-by-step instructions to help reset a project’s dependencies and ensure a clean installation. Ideally, this will allow you to resolve dependency conflicts.
This guide will navigate you through the npm clean install command, helping you understand its importance and how to use it effectively. Whether you’re dealing with inconsistent behavior across environments or simply want to ensure that your project dependencies are precisely as specified, this command is a critical tool in your arsenal.
Let’s simplify your development process and ensure smooth package management with npm!
TL;DR: How Do I Perform a Clean Install with npm?
To perform a clean installation of your project dependencies using npm, simply execute the command
npm ci
in your terminal.
Here’s a quick example:
npm ci
# Output:
# added 200 packages in 4.567s
This command effectively removes the existing node_modules directory and installs all the dependencies defined in your package-lock.json file, ensuring that your project’s dependencies are in a clean and consistent state. This process is crucial for avoiding discrepancies between development environments and can be particularly beneficial in continuous integration pipelines.
Intrigued? Keep reading for more detailed instructions and tips on mastering the npm clean install process.
Table of Contents
Basic Use: NPM Clean Install
Embarking on your journey with npm clean install
begins with understanding its basic use and fundamental differences from the more commonly used npm install
. This distinction is crucial for developers looking to ensure a stable and consistent environment for their Node.js projects.
The Basics: npm ci
While npm install
is often used to add new packages or update existing ones, npm ci
(clean install) serves a different purpose. It’s designed to provide a fresh start by removing the existing node_modules directory and installing all the dependencies exactly as specified in your package-lock.json file. Here’s how you can execute it:
npm ci
# Output:
# added 123 packages from 78 contributors and audited 321 packages in 9.87s
# found 0 vulnerabilities
The output indicates that npm ci
has successfully installed all the dependencies as defined, without any discrepancies. This process is faster and more reliable than a standard install, especially in continuous integration/continuous deployment (CI/CD) environments where consistency is key.
Why npm ci
Over npm install
?
The npm ci
command is preferred in scenarios where accuracy and consistency are crucial. Unlike npm install
, which can update packages and modify the package-lock.json file, npm ci
ensures that the installations are exactly as per the lock file, thus avoiding any unexpected changes. This is particularly important in team settings and production environments, where even minor differences in package versions can lead to significant issues.
In summary, npm ci
is your go-to command for resetting your project’s dependency tree to its original, pristine state. Whether you’re setting up a new development environment, dealing with bugs, or preparing for a production build, npm ci
offers a clean, reliable foundation.
Advanced npm ci Techniques
As you grow more comfortable with the npm ci
command, you’ll find its utility extends beyond just installing dependencies from your package-lock.json. It’s a powerful tool for managing complex scenarios involving private packages, different registries, and discrepancies in your package-lock.json file.
Handling Private Packages
Private packages often require authentication to download and install. When using npm ci
in a project that includes private packages, ensure your .npmrc
file is correctly configured with the necessary authentication tokens. Here’s an example setup:
# .npmrc configuration
//registry.npmjs.org/:_authToken=${NPM_TOKEN}
This configuration allows npm to authenticate against the registry using an environment variable NPM_TOKEN
. Ensure this token is securely stored and accessible in your CI/CD environment.
Utilizing Different Registries
Sometimes, projects may depend on packages from different registries. The npm ci
command respects the registry configurations specified in your .npmrc
file, allowing for seamless installations from multiple sources. For instance:
# .npmrc configuration
@myorg:registry=https://my-private-registry.example.com/
This line directs npm to fetch all packages scoped under @myorg
from a custom registry, ensuring that dependencies are correctly resolved from both public and private sources.
Managing Package-Lock.json Discrepancies
Discrepancies in the package-lock.json can lead to issues during the clean install process. The npm ci
command is designed to enforce the integrity of your package-lock.json, ensuring that all dependencies are installed as specified. If discrepancies are detected, npm will halt the installation and prompt for resolution. This strict adherence to the lock file maintains consistency across environments and reduces the likelihood of deployment issues.
Understanding these advanced use cases of npm ci
not only enhances your workflow but also ensures a higher level of reliability and consistency in your project’s dependency management. By mastering these techniques, you’ll be well-equipped to tackle complex scenarios with confidence.
Alternative Dependency Managers
While npm clean install
is a powerful command for managing Node.js project dependencies, the JavaScript ecosystem offers alternative tools that provide similar functionality with their unique advantages. Let’s dive into yarn and pnpm, two popular npm alternatives, and see how they stack up.
Yarn: Fast and Reliable
Yarn emerged as a Facebook project aiming to improve upon npm’s performance and consistency. One of its standout features is yarn install --frozen-lockfile
, which mirrors npm ci
by ensuring dependencies match exactly what’s in the yarn.lock file.
yarn install --frozen-lockfile
# Output:
# Success! Locked and loaded!
The command above ensures that all dependencies are installed according to the yarn.lock file, similar to npm’s package-lock.json. This feature is particularly useful for CI/CD pipelines, where consistency is crucial. Yarn’s cache mechanism and parallel installation process often result in faster builds compared to npm.
pnpm: Efficient and Disk Space Friendly
pnpm is another alternative that focuses on efficiency and saving disk space. It achieves this by hard linking packages from a single content-addressable storage. For clean installations, pnpm’s equivalent to npm ci
is pnpm install --frozen-lockfile
.
pnpm install --frozen-lockfile
# Output:
# Packages are hard linked from the content-addressable store to the virtual store
# Direct dependencies are linked directly to node_modules
This command ensures that your dependencies are installed exactly as specified in the pnpm-lock.yaml file, with the added benefit of saving disk space. pnpm’s strictness in package linking also helps in avoiding phantom dependencies, making your project’s dependency tree more reliable and easier to manage.
Making the Right Choice
Choosing between npm, yarn, and pnpm depends on your project’s specific needs and your development environment. While npm clean install
offers a straightforward approach to resetting your project’s dependencies, yarn and pnpm provide compelling alternatives with unique benefits, such as faster installations and more efficient storage usage. Each tool has its strengths, and understanding these can help you make an informed decision on the best tool for managing your project’s dependencies.
Troubleshooting npm ci Issues
When leveraging the power of npm ci
for a clean install, you might encounter a few roadblocks. These issues range from outdated package-lock.json files to missing scripts and network troubles. Understanding these common pitfalls and their solutions can enhance your npm experience.
Outdated package-lock.json
An outdated or mismatched package-lock.json file can prevent npm ci
from executing correctly, as this command relies on the integrity of the package-lock.json to install dependencies.
npm ci
# Output:
# npm ERR! Invalid: lock file's [email protected] does not satisfy abc@^1.0.1
The output indicates a version mismatch between the package-lock.json and the project’s dependencies. To resolve this issue, you may need to regenerate the package-lock.json by running npm install
and committing the updated file to your repository.
Missing Scripts and Dependencies
Sometimes, the clean install process might halt due to missing scripts or dependencies not defined in package-lock.json. This can occur if dependencies have been added manually to the node_modules directory without updating the package-lock.json.
npm ci
# Output:
# npm ERR! missing script: build
This error message highlights a missing script that npm ci
expected to find. Ensuring all scripts and dependencies are correctly defined in your package.json and package-lock.json files is crucial for a smooth installation process.
Network Problems
Network issues can also disrupt the npm ci
process, especially when installing packages from private registries or when the network is unstable.
npm ci
# Output:
# npm ERR! network timeout at ...
This output suggests a network timeout occurred during the installation. To mitigate network-related problems, consider verifying your network connection, using a reliable VPN if necessary, or configuring npm to use a proxy.
Ensuring a Smooth npm ci Process
To ensure a smooth npm clean install
process, regularly update your package-lock.json, accurately define all dependencies and scripts, and maintain a stable network connection. Addressing these considerations proactively can save time and prevent frustration, allowing you to focus on what you do best: building amazing software.
Understanding npm and Its Ecosystem
Before diving deeper into the npm clean install
command, it’s essential to grasp the fundamentals of npm, package-lock.json, and the node_modules directory. These components play a pivotal role in Node.js project management and understanding their functionality can significantly enhance your development workflow.
npm: The Node Package Manager
npm stands for Node Package Manager. It’s the default package manager for Node.js, allowing developers to share and consume packages from the npm registry. npm helps in managing project dependencies efficiently.
The Role of package-lock.json
The package-lock.json file is an auto-generated document created by npm. It precisely maps out the versions of each package and its dependencies installed in your project. This file ensures that the same versions of the packages are installed across different environments, providing consistency and reducing bugs.
{
"name": "your-project-name",
"version": "1.0.0",
"lockfileVersion": 1,
"requires": true,
"packages": {},
"dependencies": {
"your-dependency": {
"version": "1.2.3",
"integrity": "sha512-...",
"requires": {
"another-dependency": "^4.5.6"
}
}
}
}
The code block above is an example of what a section of the package-lock.json might look like. It highlights the version, integrity, and dependencies of a package. This detailed mapping is crucial for npm ci
to perform a clean install, ensuring that the exact versions specified are installed.
Understanding the node_modules Directory
The node_modules directory is where npm installs the project’s dependencies. It can become quite large as it contains not only the direct dependencies listed in your package.json but also their dependencies.
When you execute npm install
, npm checks the package-lock.json to install the exact versions of the packages listed. However, over time, discrepancies can occur due to manual changes or updates. This is where npm ci
comes into play, removing the existing node_modules directory and creating a fresh install based on the lock file, thus ensuring consistency across development, testing, and production environments.
Understanding these fundamentals is key to mastering npm and effectively managing your project’s dependencies. With a solid grasp of npm, package-lock.json, and the node_modules directory, you’re well-equipped to utilize npm clean install
for maintaining project health and consistency.
Continuous Integration and Beyond
The npm clean install
command, or npm ci
, plays a crucial role beyond local development, especially in continuous integration (CI) pipelines, automated deployments, and large-scale projects. Its ability to ensure reproducible builds and optimize build times makes it an indispensable tool in modern software development practices.
Ensuring Reproducible Builds with npm ci
In CI/CD environments, the consistency of builds across different stages and environments is paramount. npm ci
ensures that every build starts with the exact set of dependencies defined in package-lock.json, thus guaranteeing reproducibility.
npm ci
# Output:
# added 204 packages in 5.42s
The command above demonstrates how npm ci
quickly installs dependencies as specified, without updating or modifying the package-lock.json file. This behavior is crucial for avoiding discrepancies between development, testing, and production environments, ensuring that the software behaves as expected in every stage.
Optimizing Build Times in Large-Scale Projects
For large-scale projects, build times are a critical factor. npm ci
is optimized for speed, bypassing certain checks performed by npm install
and leveraging caching mechanisms more effectively.
npm ci --prefer-offline
# Output:
# added 204 packages in 3.67s
By using the --prefer-offline
flag, npm ci
can significantly reduce build times by prioritizing cached packages over fetching them from the network. This optimization is particularly beneficial in CI environments where build speed can directly impact the development workflow and productivity.
Further Resources for Mastering npm ci
To deepen your understanding of npm ci
and its applications in modern development practices, here are three external websites offering valuable insights and tutorials:
- npm Documentation: The official npm documentation provides a comprehensive overview of the
npm ci
command, including its options and expected behaviors. Node.js Project Best Practices: NodeSource offers best practices for Node.js projects, including tips on using
npm ci
for faster and more reliable builds.Continuous Integration with Node.js: A guide on implementing continuous integration in Node.js projects.
By exploring these resources, you can further enhance your skills and leverage npm ci
to its full potential, ensuring your projects are built efficiently and reliably.
Wrapping Up: npm Clean Installs
In this comprehensive guide, we’ve navigated the intricacies of the npm clean install
command, a vital tool for Node.js developers seeking to ensure a clean and consistent environment for project dependencies. This command, central to maintaining project health, resets your project’s foundation by aligning dependencies precisely as defined in your package-lock.json.
We began with the basics, exploring how to perform a clean installation using npm ci
, and the advantages it holds over the traditional npm install
. We delved into the differences, emphasizing npm ci
‘s role in avoiding discrepancies and ensuring a stable development, testing, and production environment.
Moving to more advanced usage, we examined handling private packages, utilizing different registries, and navigating package-lock.json discrepancies. These insights are crucial for developers working on complex projects or in team settings where consistency is paramount.
We also explored alternative tools like yarn and pnpm, comparing their approaches to clean installs. This comparison highlights the diverse ecosystem available for JavaScript developers, allowing for informed choices based on project needs and preferences.
Tool | Clean Install Command | Notable Feature |
---|---|---|
npm | npm ci | Aligns with package-lock.json |
yarn | yarn install --frozen-lockfile | Fast and reliable performance |
pnpm | pnpm install --frozen-lockfile | Efficient storage usage |
Whether you’re tackling a new Node.js project or managing an existing one, understanding the npm clean install
command and its alternatives equips you with the knowledge to ensure a smooth and efficient development process. The ability to reset your project’s dependency tree to its original state is an invaluable asset in today’s fast-paced development environments.
Happy coding! May your builds be quick and your dependencies consistent.