Log4j in Action: Your Guide to Java Logging
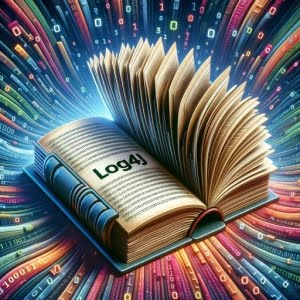
Ever felt overwhelmed with logging in Java? You’re not alone. Many developers find themselves in a maze when it comes to handling logs in Java. But, there’s a tool that can make this process a breeze.
Like a diligent secretary, Log4j keeps track of your application’s activities, providing a detailed account of its operations. It’s a popular logging utility in Java, known for its speed, flexibility, and reliability.
This guide will walk you through the process of using Log4j, from the basics to more advanced techniques. We’ll cover everything from setting up Log4j, using its logging methods, to exploring its advanced features and even troubleshooting common issues.
So, let’s dive in and start mastering Log4j!
TL;DR: What is Log4j and How Do I Use It?
Log4j is a reliable, fast, and flexible logging framework (APIs) written in Java, developed by Apache. To use Log4j, you need to configure it, and then use its logging methods in your code. For instance, to log a simple message, you could use
Logger logger = LogManager.getLogger(); logger.info('Hello, Log4j!');
.
Here’s a simple example:
import org.apache.log4j.Logger;
public class MyApp {
private static final Logger logger = Logger.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Hello, Log4j!");
// Your application logic here
}
}
# Output:
# INFO HelloWorld - Hello, Log4j!
This example demonstrates using Log4j’s Logger
class. The logger.info()
method is used to log a message. Ensure that log4j.jar
is included in your classpath. For more comprehensive logging configurations, create a log4j.properties
or log4j.xml
file in your project’s classpath.
This is just a basic way to use Log4j in Java, but there’s much more to learn about configuring and using this powerful logging framework. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
Getting Started with Log4j
To start using Log4j in your Java application, you’ll need to follow a few simple steps. The process involves adding the Log4j library to your project, setting up a configuration file, and then using the logging methods in your code.
Adding Log4j to Your Project
The first step is to add the Log4j library to your project. If you’re using Maven, you can add the following dependency to your pom.xml
file:
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
For Gradle, add this to your build.gradle:
dependencies {
implementation 'log4j:log4j:1.2.17'
}
Alternatively, you can download the JAR file directly from the Apache Log4j Archive.
Setting Up Log4j Configuration
Log4j configuration can be done using a log4j.properties
or log4j.xml
file. Place this file in the classpath of your application. Here’s a simple log4j.properties
example
log4j.rootLogger=DEBUG, stdout, file
log4j.appender.stdout=org.apache.log4j.ConsoleAppender
log4j.appender.stdout.layout=org.apache.log4j.PatternLayout
log4j.appender.stdout.layout.ConversionPattern=%d [%t] %-5p %c - %m%n
log4j.appender.file=org.apache.log4j.RollingFileAppender
log4j.appender.file.File=logs/app.log
log4j.appender.file.layout=org.apache.log4j.PatternLayout
log4j.appender.file.layout.ConversionPattern=%d [%t] %-5p %c - %m%n
Logging with Log4j
Once you’ve set up Log4j, you can start logging messages in your code. Here’s how you can log a simple INFO message:
import org.apache.log4j.Logger;
public class MyApp {
final static Logger logger = Logger.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("This is an info log entry");
logger.error("This is an error log entry");
}
}
In this example, we first import the necessary Log4j
classes. We then create a logger instance using the Logger.getLogger()
method, passing in the class name. Finally, we use the info()
method on the logger instance to log our message. We also use the .error()
method on the logger to simulate an error entry.
Advanced Log4j Features
Once you’ve gotten the hang of basic logging with Log4j, it’s time to explore some of its more advanced features. These features allow you to customize your logging to suit your specific needs, providing greater control and flexibility.
Different Logging Levels
Log4j supports several logging levels, which determine the severity of the messages being logged. The levels, in order of severity, are: FATAL, ERROR, WARN, INFO, DEBUG, and TRACE
. You can set the level in your configuration file or in your code.
Here’s how you can log messages of different levels:
logger.fatal('Fatal log message');
logger.error('Error log message');
logger.warn('Warn log message');
logger.info('Info log message');
logger.debug('Debug log message');
logger.trace('Trace log message');
# Output:
# FATAL HelloWorld - Fatal log message
# ERROR HelloWorld - Error log message
# WARN HelloWorld - Warn log message
# INFO HelloWorld - Info log message
# DEBUG HelloWorld - Debug log message
# TRACE HelloWorld - Trace log message
In this example, we use different methods on the logger instance to log messages of different levels. The logged messages include the log level, which can be useful for filtering and analyzing your logs.
Configuring Multiple Appenders
Log4j allows you to log messages to multiple destinations, such as the console, a file, or a remote server. Each destination is represented by an appender. You can configure multiple appenders in log4j.properties
or log4j.xml
to direct log messages to different outputs.
Here’s a configuration file that sets up both a console appender and a file appender:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
<File name="File" fileName="logs/app.log">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</File>
</Appenders>
<Loggers>
<Root level="all">
<AppenderRef ref="Console"/>
<AppenderRef ref="File"/>
</Root>
</Loggers>
</Configuration>
In this configuration, we’ve added a file appender that logs messages to a file named app.log
in the logs
directory. The root logger uses both the console appender and the file appender, so messages will be logged to both the console and the file.
Using Layout Patterns
Log4j allows you to customize the format of your log messages using layout patterns. These patterns can include elements like the timestamp, thread name, log level, logger name, and the actual message.
Here’s an example of a layout pattern that includes the timestamp, log level, and message:
<PatternLayout pattern="%d{ISO8601} %-5level - %msg%n"/>
This pattern uses the ISO8601
format for the timestamp, followed by the log level and the message. The output might look something like this:
2022-01-01T12:34:56,789 INFO - Hello, Log4j!
These advanced features of Log4j provide a powerful and flexible framework for logging in Java. By leveraging these features, you can tailor your logging to your specific needs, making your logs more informative and easier to analyze.
Exploring Alternatives to Log4j
While Log4j is a powerful and flexible logging framework, it’s not the only game in town. Let’s take a look at two other popular logging frameworks in the Java ecosystem: Logback and java.util.logging.
Logback: The Successor to Log4j
Logback is often seen as the successor to Log4j, designed by the same author. It’s designed to be faster and more flexible than Log4j, with a cleaner codebase and a more modern API.
Here’s a simple example of logging with Logback:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class HelloWorld {
private static final Logger logger = LoggerFactory.getLogger(HelloWorld.class);
public static void main(String[] args) {
logger.info('Hello, Logback!');
}
}
# Output:
# 12:34:56.789 [main] INFO HelloWorld - Hello, Logback!
In this example, we use the LoggerFactory.getLogger()
method to create a logger instance, similar to Log4j. The info()
method is used to log a simple message.
java.util.logging: The Java Standard
java.util.logging, also known as JUL, is the standard logging framework that comes with the Java Development Kit (JDK). It’s not as powerful or flexible as Log4j or Logback, but it’s always available without adding any external libraries.
Here’s a simple example of logging with JUL:
import java.util.logging.Logger;
public class HelloWorld {
private static final Logger logger = Logger.getLogger(HelloWorld.class.getName());
public static void main(String[] args) {
logger.info('Hello, JUL!');
}
}
# Output:
# Jan 01, 2022 12:34:56 PM HelloWorld main
# INFO: Hello, JUL!
In this example, we use the Logger.getLogger()
method to create a logger instance. The info()
method is used to log a simple message. The output format is different from Log4j and Logback, showing the date, time, class name, method name, log level, and message.
Choosing the Right Framework
When choosing a logging framework, consider your specific needs. If you need a powerful, flexible framework with many features, Log4j or Logback might be the right choice. If you’re working on a small project or just need basic logging capabilities, JUL might be sufficient.
Keep in mind that you can also use the Simple Logging Facade for Java (SLF4J) to abstract away the logging framework. This allows you to switch between Log4j, Logback, and JUL without changing your application code.
Resolving Common Log4j Issues
While using Log4j, you may encounter some common issues. Let’s discuss these problems and their solutions to help you troubleshoot effectively.
Log4j Not Logging
One of the most common issues you might face is Log4j not logging anything. This can occur due to several reasons, such as incorrect configuration, missing or misplaced configuration file, or incorrect logging level.
Ensure that your configuration file is correctly set up and located in a place where Log4j can find it (typically the src/main/resources
directory in a Maven project). Also, check that the logging level is not set too high. For instance, if the logging level is set to ERROR, INFO messages will not be logged.
Logging in the Wrong Format
If your logs are not in the expected format, check your layout pattern in the configuration file. Ensure that the pattern matches your desired format. Here’s an example of a pattern that includes the timestamp, log level, logger name, and message:
<PatternLayout pattern="%d{ISO8601} %-5level %logger{36} - %msg%n"/>
In this pattern, %d{ISO8601}
represents the timestamp, %-5level
is the log level, %logger{36}
is the logger name, and %msg
is the message. The %n
at the end inserts a newline character after each message.
Log File Not Created or Updated
If Log4j is not creating or updating the log file, check your file appender configuration. Make sure the fileName
attribute points to the correct path and that your application has the necessary permissions to create and write to the file.
Here’s an example of a file appender configuration:
<File name="File" fileName="logs/app.log">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</File>
In this example, the fileName
attribute is set to logs/app.log
, which means Log4j will create a file named app.log
in the logs
directory.
By understanding these common issues and their solutions, you can effectively troubleshoot your Log4j setup and ensure your logging is working as expected.
The Importance of Logging in Software Development
In software development, logging is akin to a black box in an aircraft. It records the events and interactions that occur during the execution of a program. This information is crucial for debugging and troubleshooting, understanding system behavior, and even for security audits.
logger.info('User login successful.');
logger.error('Failed to connect to the database.');
# Output:
# INFO Main - User login successful.
# ERROR Main - Failed to connect to the database.
In this code snippet, we log an informational message when a user logs in successfully, and an error message when the system fails to connect to the database. These logs can help us understand what the system is doing and quickly identify any issues.
Log4j: A Powerful Tool for Java Logging
Log4j comes into play as a powerful tool for handling logging in Java. It’s a flexible and extensible API, allowing developers to log messages according to message type and level, and to control output format and destination.
Architecture of Log4j
The architecture of Log4j is built around three main components: loggers, appenders, and layouts.
- Loggers: These are named entities that developers use to log messages. They have different levels (FATAL, ERROR, WARN, INFO, DEBUG, TRACE) which can be used to filter out logs below a certain severity.
Appenders: These are output destinations for the logs. Log4j provides several appenders out of the box, such as console, file, and socket appenders, and you can also create your own.
Layouts: These determine the format of the output. Log4j provides several layouts, such as the simple layout (which outputs the level and the message), and the pattern layout (which is highly configurable).
By understanding these components and how they interact, you can effectively use Log4j to handle logging in your Java applications.
Log4j in Larger Projects
As your projects grow in size and complexity, the role of logging becomes even more critical. Log4j’s flexibility and adaptability make it an ideal choice for large-scale projects. With its ability to handle multiple loggers and appenders, you can easily segregate logs based on their source and importance.
Moreover, Log4j’s integration with other Java frameworks, such as Spring and Hibernate, can help streamline your logging process. For instance, you can use Aspect-Oriented Programming (AOP) in Spring to log method calls, providing valuable insights into your application’s behavior.
Transitioning to Log4j2
Log4j2, the successor to Log4j, offers several enhancements, including improved performance, better support for multi-threaded applications, and an advanced plugin system. If you’re already familiar with Log4j, transitioning to Log4j2 can be a worthwhile investment.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class HelloWorld {
private static final Logger logger = LogManager.getLogger(HelloWorld.class);
public static void main(String[] args) {
logger.info('Hello, Log4j2!');
}
}
# Output:
# INFO HelloWorld - Hello, Log4j2!
In this example, the code remains largely the same when transitioning from Log4j to Log4j2. The output shows the logged message, along with the log level and logger name.
Further Resources for Mastering Log4j
To deepen your understanding of Log4j and its related technologies, here are some valuable resources:
- Advanced Javadoc Techniques – Learn how to publish and distribute Javadoc documentation for your Java projects.
Exploring SLF4J Logging Library – Master SLF4J for standardized logging across Java projects
Log4j2: Overview – Explore Log4j 2 for improved logging in Java.
Apache Log4j User’s Guide provides a comprehensive guide to using Log4j2, complete with examples and explanations.
Log4j Tutorial by Sematext by Sematext covers various concepts and features of Log4j, including configuration, logging levels, and loggers.
Log4j Example by JavaTpoint JavaTpoint explains how to configure Log4j, create loggers, and log different types of messages with different levels of severity.
Wrapping Up: Java Logging with Log4j
In this comprehensive guide, we’ve delved into the depths of Log4j, a popular and powerful logging utility in the Java ecosystem.
We began with the basics, learning how to set up Log4j and use its logging methods in our Java code. As we progressed, we ventured into more advanced territory, exploring different logging levels, configuring multiple appenders, and using layout patterns for custom log formats.
Along our journey, we tackled common challenges that you might encounter when using Log4j, such as Log4j not logging, logging in the wrong format, and issues with log file creation or updates. For each challenge, we provided solutions and workarounds to help you get back on track.
We also looked at alternative approaches to Java logging, comparing Log4j with other logging frameworks like Logback and java.util.logging. Here’s a quick comparison of these frameworks:
Framework | Flexibility | Ease of Use | Speed |
---|---|---|---|
Log4j | High | Moderate | Fast |
Logback | High | High | Fast |
java.util.logging | Moderate | High | Moderate |
Whether you’re just starting out with Log4j or you’re looking to level up your Java logging skills, we hope this guide has given you a deeper understanding of Log4j and its capabilities.
With its balance of flexibility, ease of use, and speed, Log4j is a powerful tool for logging in Java. Now, you’re well equipped to handle any logging needs in your Java applications. Happy coding!